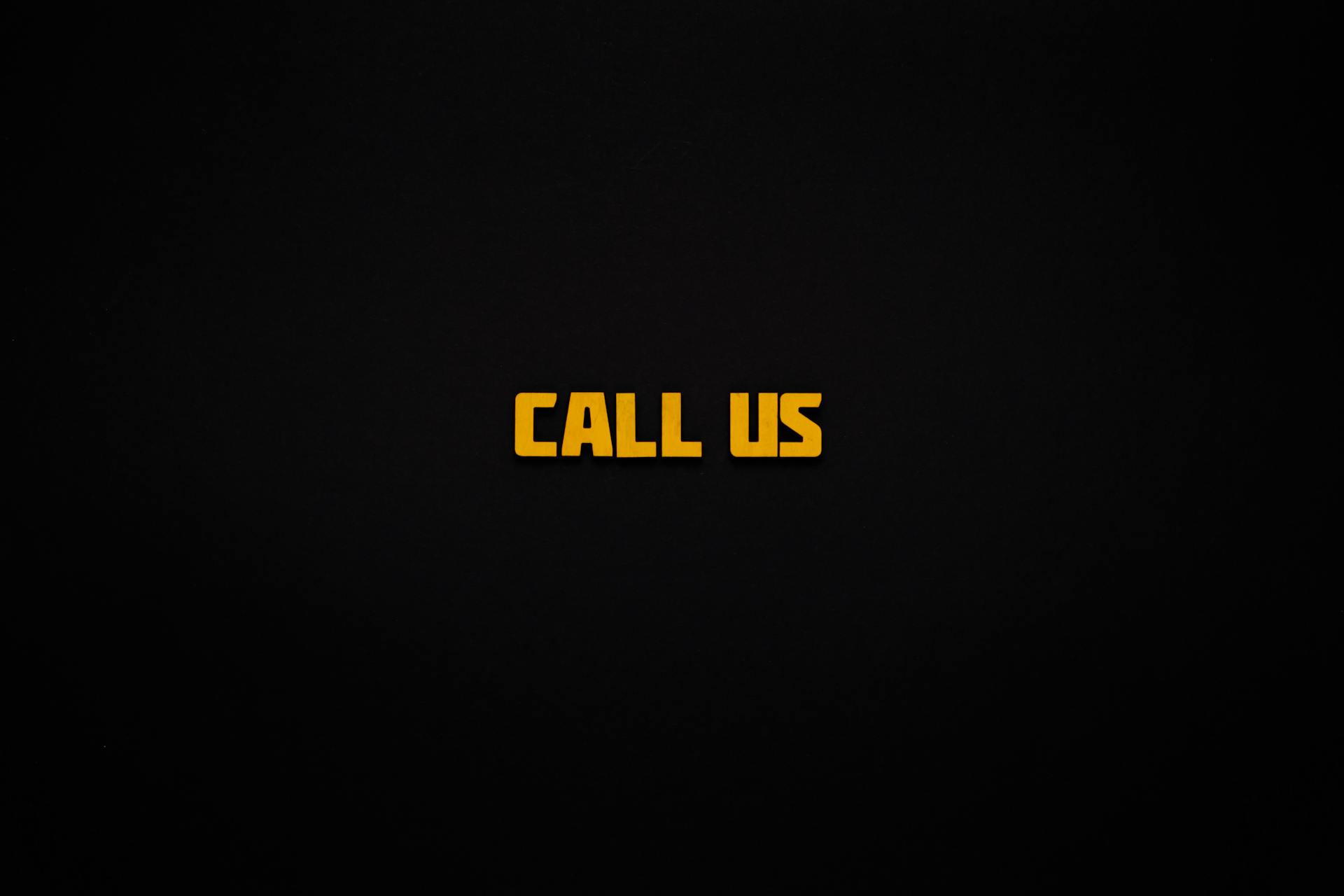
Creating a PHP contact form that sends an email is a straightforward process. You can achieve this by combining HTML and PHP code.
First, you need to set up the HTML structure for your contact form. This includes creating input fields for the user's name, email, and message.
To send the email using PHP, you'll need to use the mail() function, which is a built-in PHP function. This function takes three main parameters: the recipient's email address, the subject of the email, and the email body.
The recipient's email address is typically set to a static value, such as a company email address, while the subject and email body are dynamically generated from the form data.
HTML Structure
The HTML structure of a contact form is crucial for sending form data to a PHP script.
The action attribute in the form tag determines where the form data will be sent when submitted, typically to a PHP file like contact.php.
To create a basic form, you'll need to include the action attribute, specifying the destination of the form data.
The method attribute in the form tag determines which HTTP method to use when sending form data.
Here are the key attributes to consider:
For example, a simple HTML form with three inputs (Name, Email, Message) might look like this:
Making the Form Functional
In a PHP contact form, the form fields are typically defined using HTML elements such as input, textarea, and select.
To make the form functional, we need to add a submit button that allows users to send the form data. According to the code example, the submit button is defined as a button element with the type attribute set to submit.
The form action attribute is also crucial, as it specifies the URL where the form data will be sent. In the code example, the form action attribute is set to a PHP script that handles the form submission.
We can also add validation to the form fields to ensure that users enter valid data. The code example uses the required attribute to specify which fields are mandatory.
Validating on Both Client and Server
Client-side validation is a nice way to provide users with feedback before submission, but it's not required and can be easily bypassed by disabling Javascript.
Server-side validation is crucial because we have no idea what kind of data we'll get from a contact form submission, and even with client-side validation, users can still submit any kind of data by disabling Javascript or editing the web page source locally.
Important! Validation isn't sanitization, it's just a confirmation that the information we're getting is in a valid format and meets the criteria we expect.
If you're using PHP, you can use the same filter_var function for validation as you would for sanitization, but this time apply a validation filter instead of a sanitization filter.
Here are some validation filters you can use in PHP:
- FILTER_VALIDATE_INT
- FILTER_VALIDATE_IP
- FILTER_VALIDATE_URL
- FILTER_VALIDATE_BOOL
Remember, validation is just a confirmation that the data is in the right format, it doesn't remove any malicious data.
Sending Form Data
Sending form data is a crucial step in creating a PHP contact form that sends email. To do this, you need to collect all the form data using $_POST variables.
You'll want to sanitize the data to remove anything malicious. This involves using flags like FILTER_SANITIZE_STRING and FILTER_SANITIZE_NUMBER_INT to remove unwanted characters.
Once you have the sanitized data, you can start preparing your email headers. By including Content-type: text/html in the email headers, you can use HTML tags inside your email.
The mail() function in PHP is used to send the email, and you'll need to specify the recipient's email address, the subject line, and the email content.
Here's a breakdown of the code:
- The mail() function sends the email to the recipient's address.
- The subject line is specified after the mail() function.
- The email content is the message that will be sent to the recipient.
Here's a simple example of how to use the mail() function:
* mail($recipient, "Subject Line", $email_content, $headers);
Note that the $recipient variable should be replaced with the actual email address you want to send the email to.
Managing Your Own Backend
Managing your own form backend can be a technical and time-consuming process that requires specific skills. This approach involves using a backend framework like PHP to handle form submissions and send emails to your mailbox.
To make your form work with your email server, you need to use the "action" attribute of the form tag to send the form data to a specific URL. The browser will then send the information to the backend using this link.
Creating a form backend platform like Getform can be a great alternative to managing your own backend. This approach doesn't require any framework or language dependency and works well with static sites like Hugo, Gatsby, 11ty, and Jekyll.
Here are the steps to create a form on Getform:
- Getform automatically creates a unique form endpoint and shows a simple HTML form code with it.
- You can change the HTML to make it usable on any HTML page.
- Getform creates a unique form endpoint and shows a simple HTML form code with it.
To send an email from your HTML form using Getform, you need to set up an email notification. This can be done by filling out the sample form to start receiving submissions and then setting up an email notification.
Here's a step-by-step guide to setting up an email notification on Getform:
1. Fill out the sample form to start receiving submissions.
2. Set up an email notification by entering the email addresses you want to receive the submission data.
3. Send another submission to your form and see if the email addresses receive the submission data as an email.
If you want a full control of the email you're sending from your HTML form, Getform has a "Custom Email Notification" option. This allows you to use double brackets with the related fields to inject their values into the email template.
For example, if you have an input named as email, you can inject its value by writing {{email}} to the template. You can also embed {{@form_name}} within your template to get the name of your form.
Getform also allows you to set up autoresponders for form submitters. This involves creating an autoresponder email template section that has email, name, and message form fields as embeddable tags. You can then send an autoresponse email to your form submitter when you receive a new submission.
Here's an example of a sample autoresponder email:
- The autoresponder email will be sent to the form submitter when you receive a new submission.
- The autoresponder email will contain the email, name, and message form fields as embeddable tags.
Building a backend with PHP involves creating a submit.php file that handles form submissions, sanitizes and validates input, and sends an email. This file is responsible for ensuring a secure and stable contact form.
Here are the key components of the submit.php file:
- submit.php: PHP file for handling backend.
- index.html: HTML structure containing the contact form.
- style.css: CSS for styling the form.
To make sure everything is working correctly, you can simply display all data you get from the contact form in an array format.
Simple
Simple contact forms are a great place to start. You can find professional quality custom contact form PHP templates on CodeCanyon.
Learning how to create a simple PHP contact form is a useful skill. Check out the tutorial on CodeCanyon for a step-by-step guide.
Quform is a simple and responsive PHP email contact form example. It's easy to use and works on multiple device screen sizes.
Creating a basic contact form using HTML, CSS, and PHP is a great way to get started. You can add your own details such as an address, phone number, and email address as per your application's need.
The Quform template is a step that saves your users time, making it easier for them to fill out your form. It's a sleek and responsive contact form that works without needing to reload.
To add functionality to your form, you can use phpmailer. A separate php file is created and the name of the file is added in the HTML file in the form action field.
Simple contact forms can be created in multiple sections and HTML divs. This makes it easier to add styles later using CSS.
Sources
- https://code.tutsplus.com/create-a-contact-form-in-php--cms-32314t
- https://code.tutsplus.com/create-a-php-email-script-and-form--cms-32487t
- https://blog.getform.io/how-to-create-an-html-form-that-sends-you-an-email/
- https://www.geeksforgeeks.org/simple-contact-form-using-html-css-and-php/
- https://www.oopspam.com/blog/contact-form-with-PHP
Featured Images: pexels.com