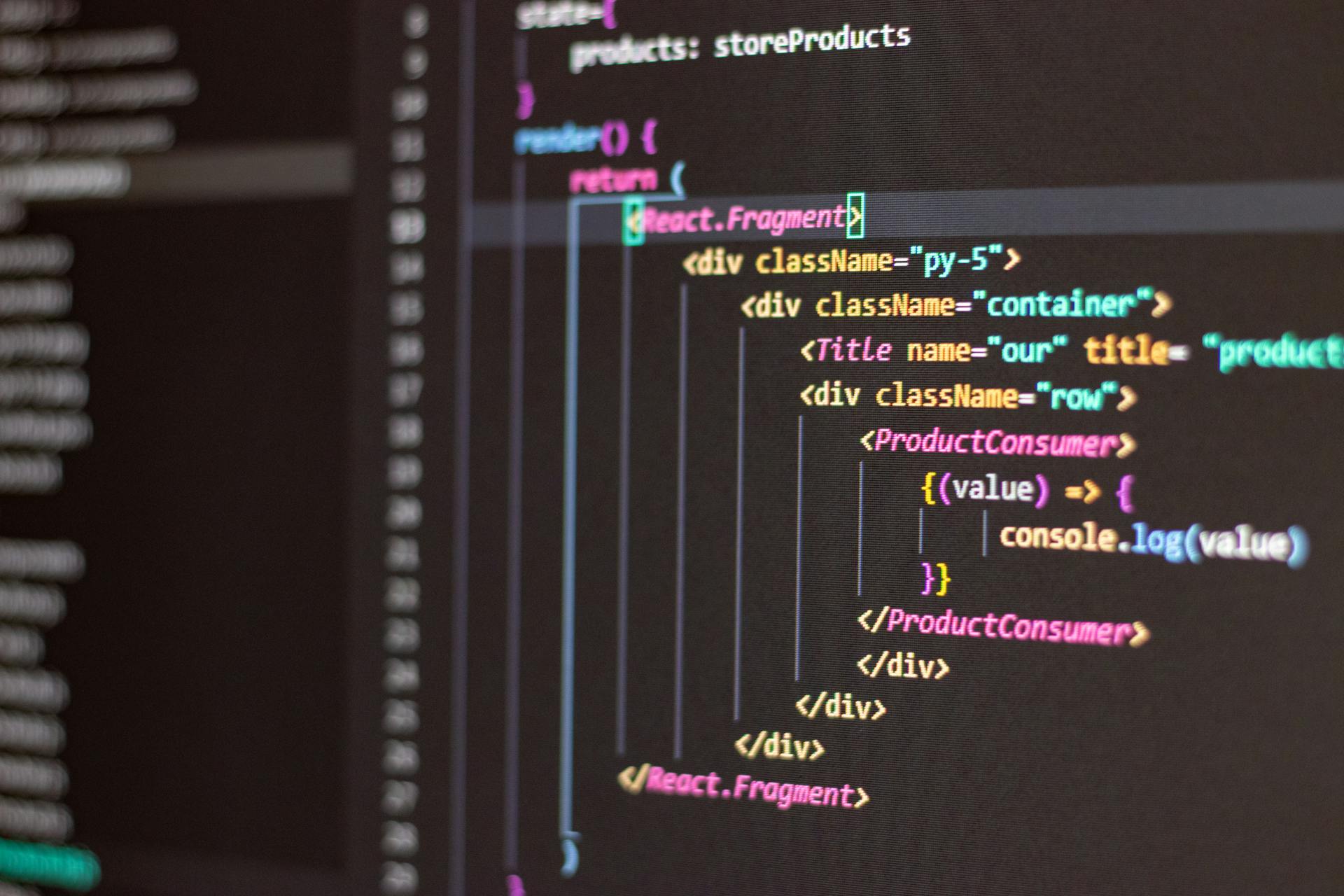
Building Next JS Forms with Server Actions is a powerful way to create dynamic and interactive forms. You can use the `useForm` hook from the `next-form` library to create a form with server-side validation.
To get started, you'll need to install the `next-form` library and set up a server action to handle form submissions. This involves creating a new API route using Next.js's built-in API routes feature.
Server actions allow you to define a custom API endpoint that handles form submissions, making it easy to validate and process form data on the server-side. This approach provides a more robust and secure way to handle form submissions compared to client-side validation alone.
Curious to learn more? Check out: Next Js Client Side Rendering
Form Basics
Next.js forms are built on top of the HTML form standard, which means you can create forms using the standard HTML form elements, such as input, select, and textarea.
To create a form in Next.js, you need to wrap your form elements in a form tag with an action attribute that points to a server-side endpoint to handle the form submission.
You can also use the built-in useForm hook to handle form state and submission in a more declarative way, which can be especially useful for complex forms.
Broaden your view: Html to Json Parser Nextjs
What Are Forms?
Forms are essentially templates that collect and organize information from users in a structured way.
A form can be as simple as a piece of paper with a few lines for someone to write on, or it can be a complex digital application with multiple fields and validation rules.
Forms are used in various contexts, including online applications, surveys, and even everyday situations like job interviews.
The primary purpose of a form is to gather specific information from users, which can then be used for various purposes, such as processing a loan application or creating a customer database.
A well-designed form should be easy to understand and navigate, with clear labels and minimal errors.
If this caught your attention, see: Next Js Spa
Prerequisites
To get started with form basics, you'll need a few things. You'll need a Formspree account, which you can sign up for free.
To use Formspree, you'll also need an existing web project built with Next.js. If you don't have one yet, you can create one by running a command in your terminal.
Next.js has an official documentation that's worth checking out if you're not familiar with it.
Creating a Form
To create a form in Next.js, start by creating a new form with the +New form button and call it Contact form.
Update the recipient email to the email where you wish to receive your form submissions and then click Create Form.
You'll then be presented with the integration options for your new Formspree form, which provides a wide range of implementation examples such as React, Ajax, and regular HTML.
The code for creating a contact-form.js component is almost identical to the React example on the integration page, so you can use that as a starting point.
To customize your form, create a special file named ContactForm.js in the components folder of your Next.js project.
With this piece of code, you've created a form that listens to every keystroke and sends the important stuff over to your route when users hit that Submit button.
Worth a look: Create Next Js App
Creating with Vercel
Creating with Vercel is a great option for form creators. You can use the Formspree Vercel integration to create forms quickly.
If this caught your attention, see: Nextjs by Vercel Meaning
To get started, go to the Formspree Vercel integration page and click Add Integration. You'll be asked to log into Formspree.
After logging in, you'll see a list of your Vercel projects that you can connect to Formspree. Connect the projects you want to use with Formspree.
For this tutorial, pick dashboard projects. Once you've connected your projects, don't forget to click Done to complete the integration setup.
The integration will create an initial form and store its ID in the NEXT_PUBLIC_FORM environment variable.
Check this out: Nextjs Open Source Personal Projects
Steps to Build
To build a form, start by creating a new form with the +New form button and call it Contact form. Update the recipient email to the email where you wish to receive your form submissions.
First, create a new folder named api inside the pages folder of your Next.js project. This is where you'll put the special route that will handle form submissions.
Copy the 8 character "hashid" from the new form's endpoint URL and replace the YOUR_FORM_ID placeholder in your contact form component. This will allow the form to submit successfully.
Check this out: What Is .next Folder in Next Js
Open contact-form.js and replace the YOUR_FORM_ID placeholder with the environment variable like so: NEXT_PUBLIC_FORM. This will automatically set up environment variables in your Vercel projects.
Create a new file called form.js inside the api folder and add the code for the special route. This route will handle any information submitted through the form.
To customize the form itself, create a special file named ContactForm.js in the components folder of your Next.js project. This will allow you to make the form look and work just the way you want it to using React components.
Explore further: Next Js Cookies
Form Structure
In Next.js, forms can be structured in a few different ways, but one common approach is to use a combination of HTML elements and JavaScript to create a dynamic form.
The `input` element is a fundamental building block of forms in Next.js, and it can be used to create a wide range of form fields, from simple text inputs to more complex date pickers.
A `form` element is required to wrap the `input` element and submit the form data to the server.
The `name` attribute is used to identify the form field and associate it with a specific value in the form data.
In Next.js, forms can be submitted using the `fetch` API or the `axios` library, both of which are supported out of the box.
By using a combination of HTML elements and JavaScript, you can create a robust and dynamic form in Next.js that meets the needs of your application.
Related reading: Next Js Get Url Params
Validation and Error Handling
Form validation is crucial in Next.js forms, and you can validate on the server using Zod. This means you won't need to validate on the client side with React Hook Form or Formik.
You can use Zod's object function to create a schema for the form data, which will have a single field text that is a string, is required, and has a max length of 191 characters.
Broaden your view: Next Js Formdata
Zod's parse method will throw an error if the form data does not match the schema, allowing you to catch and display errors to the user.
To handle errors, you can add a try-catch block to the server action to catch any errors that may occur during form submission.
You can use a utility function to create a form state object that will be used to display user feedback in the form component.
Zod's error object is nested, so you'll need to flatten it to get all error messages per form field.
Client-side validation and error messages can be implemented in Next.js form components using JavaScript.
Built-in Next.js form validation rules include required, type, minLength, and maxLength, which can help users enter information correctly.
To analyze JavaScript errors in Next.js forms, you can look for event listeners attached to form elements and identify potential errors using code editors or IDEs.
JavaScript-based Next.js form validation can be used alongside HTML form field rules to provide an extra layer of checking.
Take a look at this: Next Js Using State Context
Submission and Server Actions
In Next.js, you can use the useFormState Hook to send custom information between server action and client component. This allows for seamless communication between the client and server.
To handle form submissions, you can create a new folder named api inside the pages folder, and then create a fresh new file called form.js within it. This special route will take care of the form submissions and handle any information you send from the form.
When submitting a form, you can use the bind method to create a new function with additional arguments, such as a date, to be sent to the server action. This can be useful when working with components like DatePicker.
You can also use a hidden form field to send additional information to the server action without adjusting the server action itself. This approach can be useful when receiving data in the formData in the server action.
Explore further: New Nextjs Project Typescript
To make your form work even if someone's turned off their JavaScript, you can create a new file called no-js-form.js inside the pages directory. This allows the form to send data to the server via the /api/submit route without the need for JavaScript.
To implement submission so that the backend is triggered, you can code up a handleMySubmission function. This function will be responsible for handling the form submission and sending the data to the server.
You can use Next.js elements and make a few validation functions to create a form. The validation will be taken care of through your application's backend, i.e., the server.
To create a form endpoint, you can use a service like Formspree, which provides you with a wide range of implementation examples, such as React, Ajax, and regular HTML. This allows you to easily integrate the form with your Next.js application.
Here's an interesting read: Next Js Fetch Data save in Context and Next Route
Featured Images: pexels.com