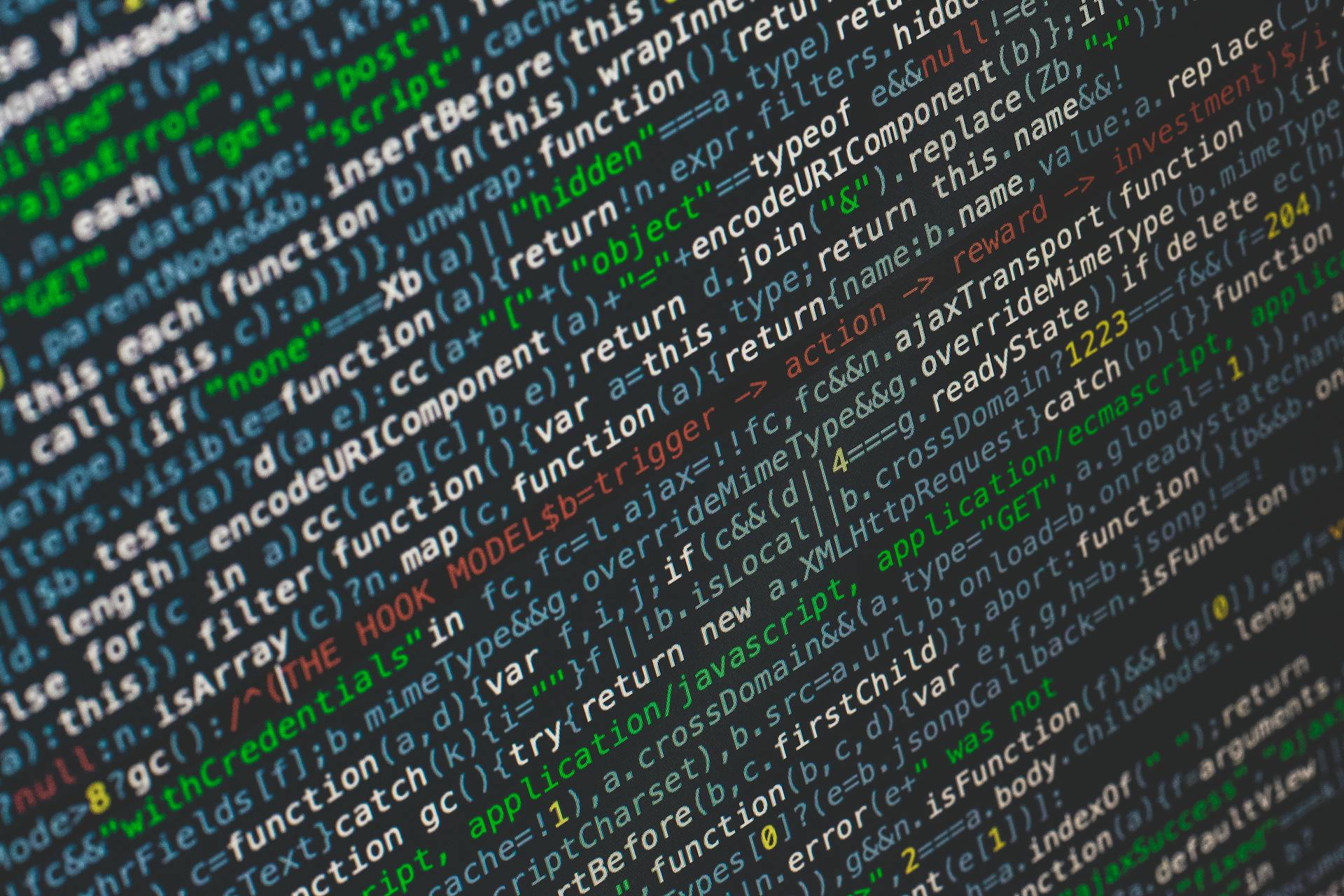
Creating cookies in Next.js is a fundamental aspect of modern web development. You can store user preferences, login information, and other data using cookies.
Next.js provides a built-in way to handle cookies through its API routes. This allows you to create, read, and delete cookies with ease.
To get started, you'll need to create an API route for handling cookies. This can be done by creating a new file in the pages/api directory of your project.
Next.js uses the serverless architecture, which enables fast and efficient cookie handling.
Take a look at this: Creating Shop Page Next Js
Implementing Cookies in Next.js
To implement cookies in Next.js, you'll need to install two packages: cookie and react-cookie. The cookie package allows you to access cookies from the server-side, while react-cookie enables you to set cookies from the client-side.
For server-side cookies, you can use the cookies module on the request/response object. This is useful for accessing cookies within an API route in Next.js. On the client-side, you can use the cookie-cutter module, which has no external dependencies and is efficient.
To set a cookie, you can use the set method, passing the key-value pair and optional options. For example, you can set the Max-Age, Secure, and HttpOnly flags to control the cookie's expiration, accessibility, and access restrictions.
See what others are reading: Next Js Debug
Client Component
To get and set cookies in the client component, we'll use the js-cookie library. Install it first and then you're good to go.
For setting a cookie in the client component, you can use the setCookie hook from react-cookies. This sets the cookie to the default name, which is 'user' in this case.
To access client-side cookies in Next.js, we use the cookie-cutter module. It's efficient and has no external dependencies.
The cookie-cutter module can be used to check if a cookie exists. This is useful for determining whether a user is logged in or not.
To remove a cookie, you can call Cookie.remove(key). This deletes the cookie completely.
A unique perspective: Next Js Use Client
Cookie Strings
Cookies in Next.js can be a bit tricky to work with, but once you get the hang of it, it's a powerful tool for customizing user experiences.
A valid cookie string is simply a string with a name and a value, like viewedWelcomeMessage=true.
However, you can also add additional settings to control how the cookie is stored and accessed. For example, adding Max-Age=600 will make the cookie expire after 10 minutes.
You can also set the Secure flag to ensure the cookie is only accessible on HTTPS sites or localhost.
And finally, you can set the HttpOnly flag to make the cookie only accessible from the server.
Using a library like cookie can make constructing these strings a lot easier.
Cookie Management
To manage cookies in Next.js, you need to install two packages: cookie and react-cookie. The cookie package allows you to access cookies from the server-side, while react-cookie enables you to set cookies from the client-side.
For setting cookies, you can use the server actions in your application. To do this, import the cookies function in your page.tsx file and use the set method to pass the key-value pair and optional options.
Cookies can be stored on the client-side using the cookie-cutter module, which has no external dependencies and is efficient. On the other hand, server-side cookies are accessed using the cookies module on the request/response object.
Here's an interesting read: Next Js React Fundamentals
When setting cookies, you can configure their expiry time, security, and accessibility using options like Max-Age, Secure, and HttpOnly. You can also use a library like cookie to construct the cookie string for you.
To check if a cookie is set on the client-side or server-side, you can use the following code in your application: For client-side cookies, the cookie-cutter module is used, while for server-side cookies, the cookies module is used on the request/response object.
Recommended read: Next Js Client Side Rendering
Setting with Care
To set cookies in Next.js, you need to use server actions or route handlers. This is because cookies are stored on the server-side, and using them in other contexts will throw an error.
When setting multiple cookies, be careful not to overwrite the full Set-Cookie header. Instead, use the `Set-Cookie` header with multiple values, separated by semicolons.
You can use the `next/headers` module to set cookies, or the standard `Response` object and pass cookies in the header. Both methods work, but the `next/headers` module is a more modern approach.
Take a look at this: Nextjs Headers
To set a cookie, you need to use the `set` method and pass the key-value pair, along with optional options to configure the cookie. Refer to the MDN docs for all available options.
Remember to always check the browser's Application tab and cookies tab to verify that cookies are being stored correctly.
App Router and Cookies
The App Router and cookies go hand-in-hand, making it easier to get and set cookies. The App Router introduced a cookies function that simplifies the process.
To use cookies with the App Router, you can rewrite your route handlers to take in NextRequest instead of Request. This adds the cookies field, making it easier to access and set cookies.
Route handlers in the App Router are similar to API routes in the Pages Router. You can use the cookies function to set cookies, and it takes additional options in the set call.
The cookies function from next/headers can also be used to set cookies in route handlers. This provides more flexibility and options for customizing cookies.
Note that cookies can only be set in server actions or route handlers, not in server components. This is an important consideration when working with cookies and the App Router.
For another approach, see: Next Js Route Handlers
Cookie Middleware
Cookie Middleware is a powerful tool in Next.js that allows you to modify incoming requests and outgoing responses. This can be useful for augmenting server components or sharing cookie logic across multiple routes/pages.
You can use middleware to set a cookie on the response, like in Example 4, where a cookie is set on the response before it is returned to the user. This approach is useful for augmenting server components or sharing cookie logic across multiple routes/pages at the same time.
To set a cookie on the response, you can use the `NextResponse.next()` method, which indicates that Next should continue with the request. The middleware can then set a cookie on the response before it is returned to the user.
Here's a breakdown of the flow:
- The middleware runs and calls `NextResponse.next()` indicating that Next should continue with the request.
- The Message component renders, checking if the viewedWelcomeMessage cookie had been set and returning the correct message.
- The middleware continues running and sets a cookie on the response before it is returned to the user.
If you want to modify the cookie before the underlying page/route loads, you can use a technique called "forwarding modified cookies" in middleware, as shown in Example 5. This involves grabbing and modifying the cookie header, or making your own header.
Here are the steps to follow:
- Grab and modify the cookie header.
- Make your own header.
Note that modifying the request in middleware doesn't impact the request anywhere else, so you need to use one of these approaches to modify the cookie before the underlying page/route loads.
Sources
Featured Images: pexels.com