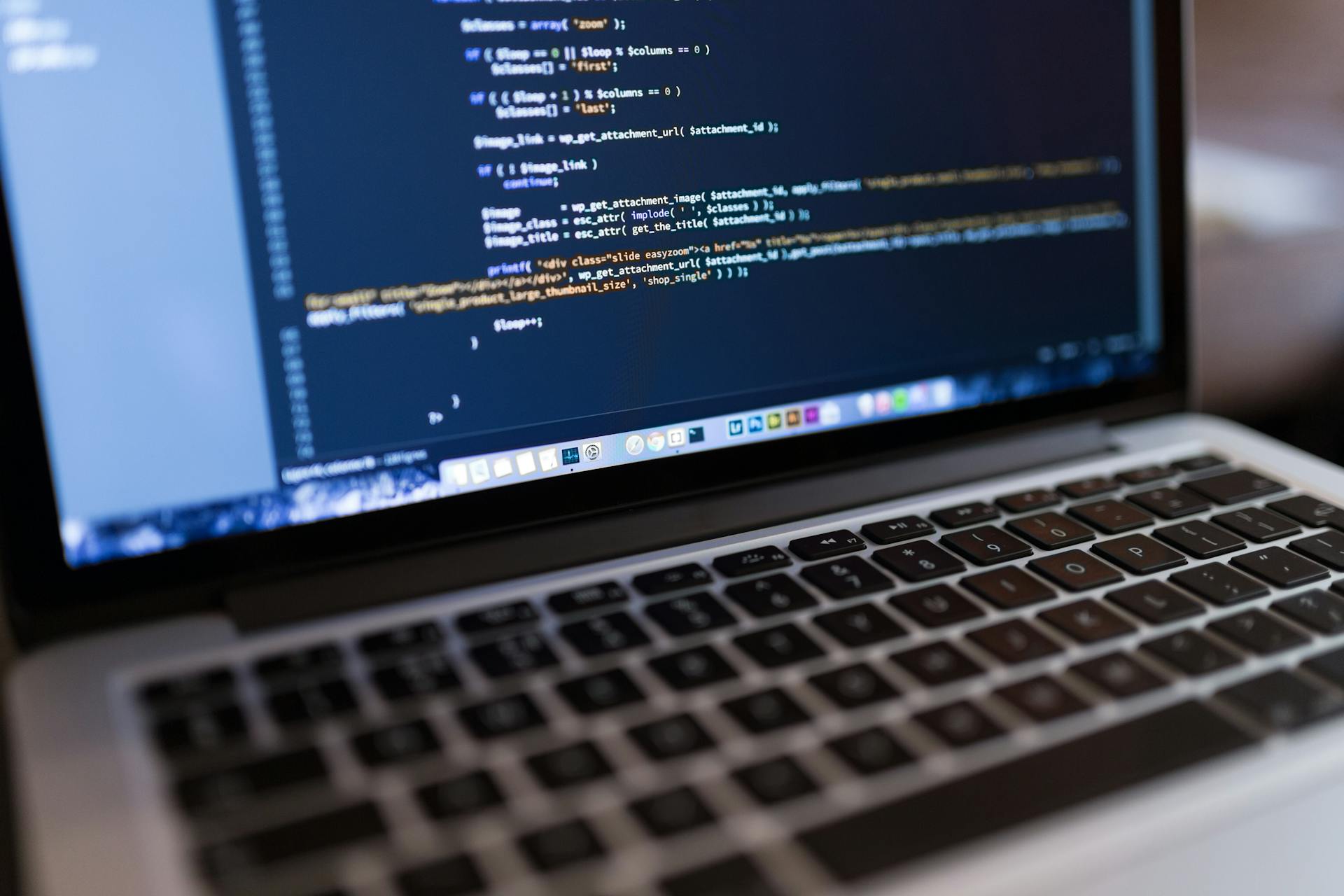
Nextjs element parameters allow you to dynamically generate static sites by passing values to components.
You can pass parameters to elements using the `props` object in Nextjs.
To make the most of element parameters, it's essential to understand how they work with static site generation.
Nextjs element parameters can be used to fetch data dynamically, making your site more interactive and engaging.
Generating Static Site Parameters
Generating static site parameters is a crucial step in Next.js, and it's essential to understand how it works. You can use the generateStaticParams function to statically generate routes at build time instead of on-demand at request time.
The generateStaticParams function returns an array of objects, where each object represents the populated dynamic segments of a single route. For example, if you have a route like /product/[id], the generateStaticParams return type would be { id: string }[].
To statically render all paths at build time, you can supply the full list of paths to generateStaticParams. However, if you want to statically render a subset of paths at build time and the rest at runtime, you can return a partial list of paths from generateStaticParams.
Here are some key points to keep in mind when working with generateStaticParams:
- You must return an empty array from generateStaticParams or utilize export const dynamic = 'force-static' to revalidate (ISR) paths at runtime.
- During next dev, generateStaticParams will be called when you navigate to a route.
- During next build, generateStaticParams runs before the corresponding Layouts or Pages are generated.
- generateStaticParams replaces the getStaticPaths function in the Pages Router.
Static Site Generation with Next.js
Static Site Generation with Next.js is a powerful feature that allows you to pre-render your website at build time, improving performance and SEO. You can use the generateStaticParams function to statically generate routes at build time instead of on-demand at request time.
This function can be used in combination with dynamic route segments, allowing you to control what happens when a dynamic segment is visited that was not generated with generateStaticParams. You must return an empty array from generateStaticParams or utilize export const dynamic = 'force-static' in order to revalidate (ISR) paths at runtime.
During next dev, generateStaticParams will be called when you navigate to a route, and during next build, it runs before the corresponding Layouts or Pages are generated. During revalidation (ISR), generateStaticParams will not be called again.
The options.params object contains the populated params from the parent generateStaticParams, which can be used to generate the params in a child segment. This allows you to generate multiple dynamic segments from the child route segment.
Here's an example of how to use generateStaticParams:
To statically render all paths at build time, supply the full list of paths to generateStaticParams. To statically render a subset of paths at build time, and the rest the first time they're visited at runtime, return a partial list of paths.
You can also use the dynamicParams segment config option to control what happens when a dynamic segment is visited that was not generated with generateStaticParams. If you want to prevent unspecified paths from being statically rendered at runtime, add the export const dynamicParams = false option in a route segment.
Note that you must always return an array from generateStaticParams, even if it's empty, otherwise the route will be dynamically rendered.
Benefits of Static Site Generation
Static Site Generation (SSG) is a game-changer for website performance.
With SSG, your website loads instantly, thanks to the pre-built HTML files that are generated at build time. This is a huge improvement over traditional dynamic sites that load content on the fly.
The average page load time for a static site is around 200-300 milliseconds, making it virtually indistinguishable from a desktop application.
In contrast, dynamic sites can take up to 3 seconds to load, which can lead to a higher bounce rate and lower conversion rates.
Static sites are also incredibly scalable, as they don't require server-side processing to render content. This means you can handle a large number of visitors without any issues.
Additionally, SSG makes it easier to deploy and maintain your website, as you don't need to worry about server-side configuration or database management.
Using Query Parameters in Next.js
In Next.js, query parameters are automatically parsed from the URL and made available in the req.query object. This allows you to access and use them in your GET request handlers.
You can extract query parameters from the req.query object and include them in your API responses. For example, if you access an API route with a query parameter, such as /api/users?id=123, the response would be customized based on the query parameter.
Next.js makes it easy to customize your API responses by allowing you to access query parameters using the req.query object. This is a powerful feature that can help you create more dynamic and interactive APIs.
Sources
- https://nextjs.org/docs/app/api-reference/functions/generate-static-params
- https://nextjs.org/docs/pages/api-reference/functions/use-router
- https://caisy.io/blog/nextjs-iframe-implementation
- https://nextjs.org/docs/pages/building-your-application/data-fetching/get-static-props
- https://nextjsstarter.com/blog/nextjs-api-routes-get-and-post-request-examples/
Featured Images: pexels.com