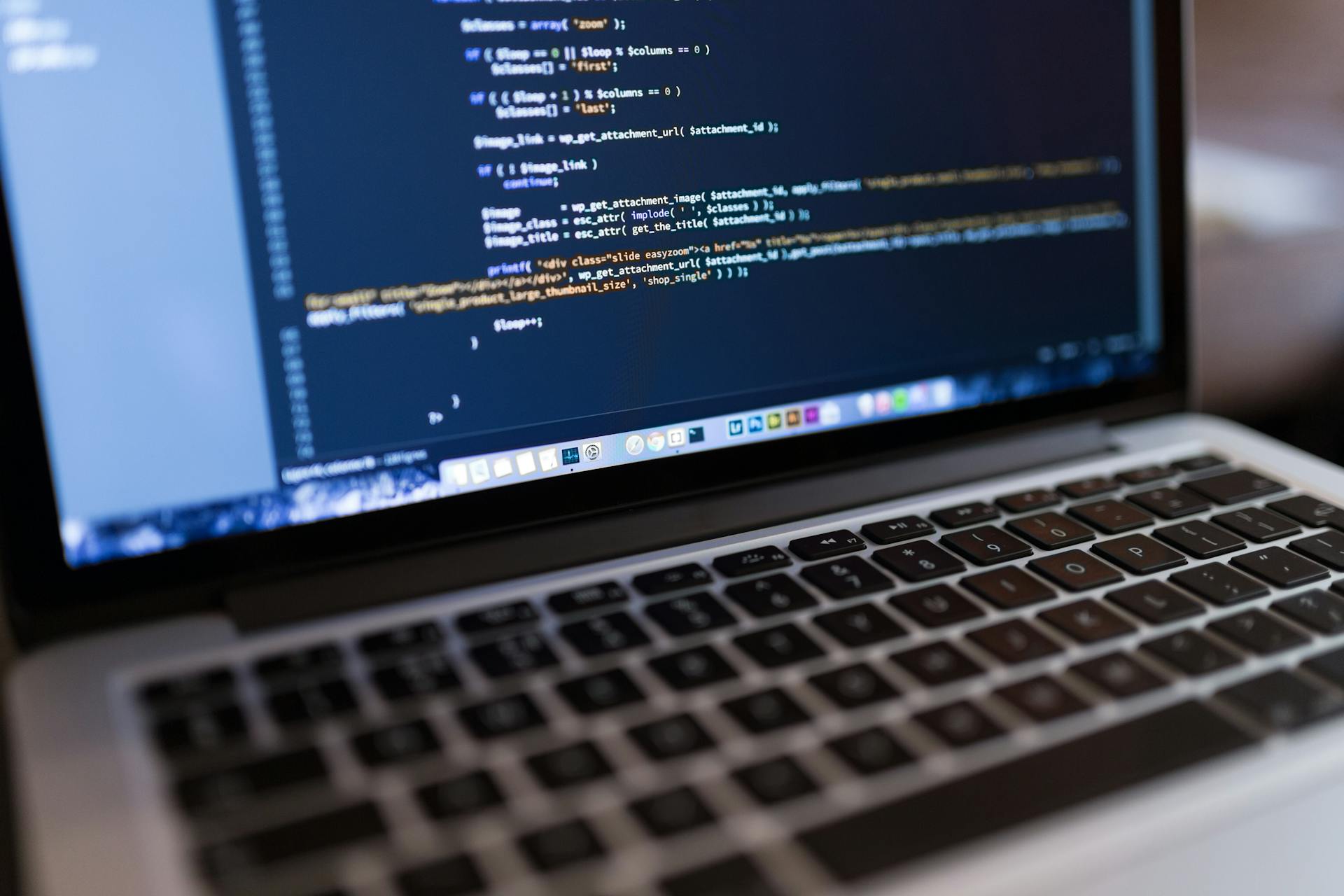
Next.js params are a powerful tool that allows you to dynamically render pages based on user input.
They can be used to create custom URLs, handle complex routing scenarios, and improve user experience.
With Next.js params, you can easily create routes that are parameterized, making it easy to handle different scenarios.
In Next.js, you can use the `getStaticProps` method to pre-render pages at build time, and the `useParams` hook to access params in a dynamic component.
Consider reading: Router Query Params Nextjs 14
Dynamic Routing
Dynamic Routing is a powerful feature in Next.js that allows you to create pages that can match a variety of URL structures. This is accomplished by placing square brackets around a file or folder name within the 'pages' directory.
Dynamic routes in Next.js are defined by placing square brackets around a file or folder name within the 'pages' directory, such as [id].js, which would match any route like /posts/1 or /posts/abc.
You can access the dynamic segments of the URL as query parameters using the useRouter hook or the context object provided to getServerSideProps or getStaticProps. This is useful when you want to create pages that adapt to different content based on the URL parameters provided.
Here's an interesting read: Next Js Dynamic Routes
To create a dynamic page that responds to query parameters, you first define the dynamic route using the file naming convention mentioned above, and then within the page component, you can access the dynamic segments of the URL as query parameters.
Here are some examples of dynamic routes:
- pages/[uid].js: matches any URL with a single path segment, such as /apples or /carrots
- pages/[category].js: matches routes like /shoes or /accessories, and the dynamic segment is available as a query parameter under the key category
- pages/[category]/[uid].js: matches routes like /clothing/t-shirt or /dogs/doberman, and returns a file for the /*/* URL
These are just a few examples of how you can use dynamic routing in Next.js to create flexible and adaptable pages. By using the useRouter hook and the context object, you can access the dynamic segments of the URL and create pages that respond to different content based on the URL parameters provided.
Broaden your view: Nextjs 14 Enviar Parametros En La Url
Using Next.js Features
Dynamic routes in Next.js allow you to create pages that can match a variety of URL structures.
These routes are defined by placing square brackets around a file or folder name within the 'pages' directory.
You can create a file named [id].js to match any route like /posts/1 or /posts/abc.
Discover more: Next Js Nested Routes
This flexibility is particularly useful when you want to create pages that adapt to different content based on the URL parameters provided.
For instance, a file named [id].js would match any route like /posts/1 or /posts/abc.
This means you can use the same code to handle different URLs with different parameters.
Next.js allows you to create pages that adapt to different content based on the URL parameters provided.
This is especially useful when working with dynamic data that needs to be displayed based on the URL.
If this caught your attention, see: Pages Router Next Js
Next.js Routing
Next.js Routing is a powerful feature that allows you to create pages that adapt to different content based on the URL parameters provided. You can define dynamic routes by placing square brackets around a file or folder name within the 'pages' directory.
For example, a file named [id].js would match any route like /posts/1 or /posts/abc. This flexibility is particularly useful when you want to create pages that adapt to different content based on the URL parameters provided.
To create a dynamic route, you can use the useRouter hook to access the category and any additional query parameters. For instance, in an e-commerce application, you could create a dynamic route to handle products based on their category and optional filters like price range or brand.
Here are some strategies to preserve query parameters during navigation:
- Passing Query Parameters Manually: You can manually include the query parameters you want to preserve in the new URL when using router.push or router.replace.
- Using a Global State Management Library: Libraries like Redux or Zustand can help manage the application state globally, including the state represented by query parameters.
- Storing in Local Storage or Session Storage: You can store query parameters in local storage or session storage and retrieve them when needed.
- Custom Hooks: Creating custom hooks to manage query parameters can abstract the logic of preserving parameters during navigation, making your components cleaner and more reusable.
Dynamic paths in Next.js's filesystem-based routing are defined inside square brackets: pages/[uid].js or app/[uid]/page.js. This route handles any URL with a single path segment, such as /apples or /carrots.
Static Generation and Hooks
Static generation is a powerful feature in Next.js that allows you to pre-render pages at build time. This is achieved through the use of getStaticProps and getStaticPaths.
getStaticProps is used to fetch data at build time, making it possible to render pages with dynamic data. getStaticPaths, on the other hand, is used to specify dynamic routes that should be pre-rendered.
In getStaticPaths, you define the paths that include the query parameters you want to pre-render. The params object provided by the context allows you to access these parameters in getStaticProps.
Discover more: Dynamic Route Nextjs 13
Static Generation with GetStaticProps
Static Generation with GetStaticProps is a powerful tool in Next.js. It allows you to fetch data at build time.
You can use getStaticProps to fetch data that doesn't change often, such as product information or blog posts. This approach can improve performance and reduce server load.
In Next.js, getStaticProps is used to fetch data at build time, which means it runs on the server during the build process. This allows you to pre-render pages with dynamic data.
For example, if you want to pre-render pages with query parameters, you can use getStaticPaths in conjunction with getStaticProps.
Here's an interesting read: Why Use Nextjs
Using the UseRouter Hook
The useRouter hook is a client-side hook that allows you to access the router object within your page components.
It's part of the Next.js router library, and it's incredibly useful for accessing query parameters of the current URL.
The router object contains the query object, which holds the query parameters of the current URL.
To use the useRouter hook, you import it from the Next.js router library and use it to retrieve the router object.
You then destructure the query object from router to access the query parameters.
Here's an interesting read: Nextjs App Router Query Params
Frequently Asked Questions
What does useParams() return?
useParams() returns an object with dynamic route parameters, where each property represents a filled-in segment with its corresponding name and value. This object helps you access and utilize the dynamic parts of your current route.
Sources
- https://prismic.io/docs/define-paths-nextjs
- https://www.dhiwise.com/post/navigating-nextjs-query-params-a-comprehensive-guide
- https://birdscoders.com/articles/how-to-add-query-parameters-to-the-url-in-nextjs/
- https://egghead.io/lessons/next-js-access-route-params-from-props-inside-a-next-js-dynamic-app-router-route
- https://authjs.dev/reference/nextjs
Featured Images: pexels.com