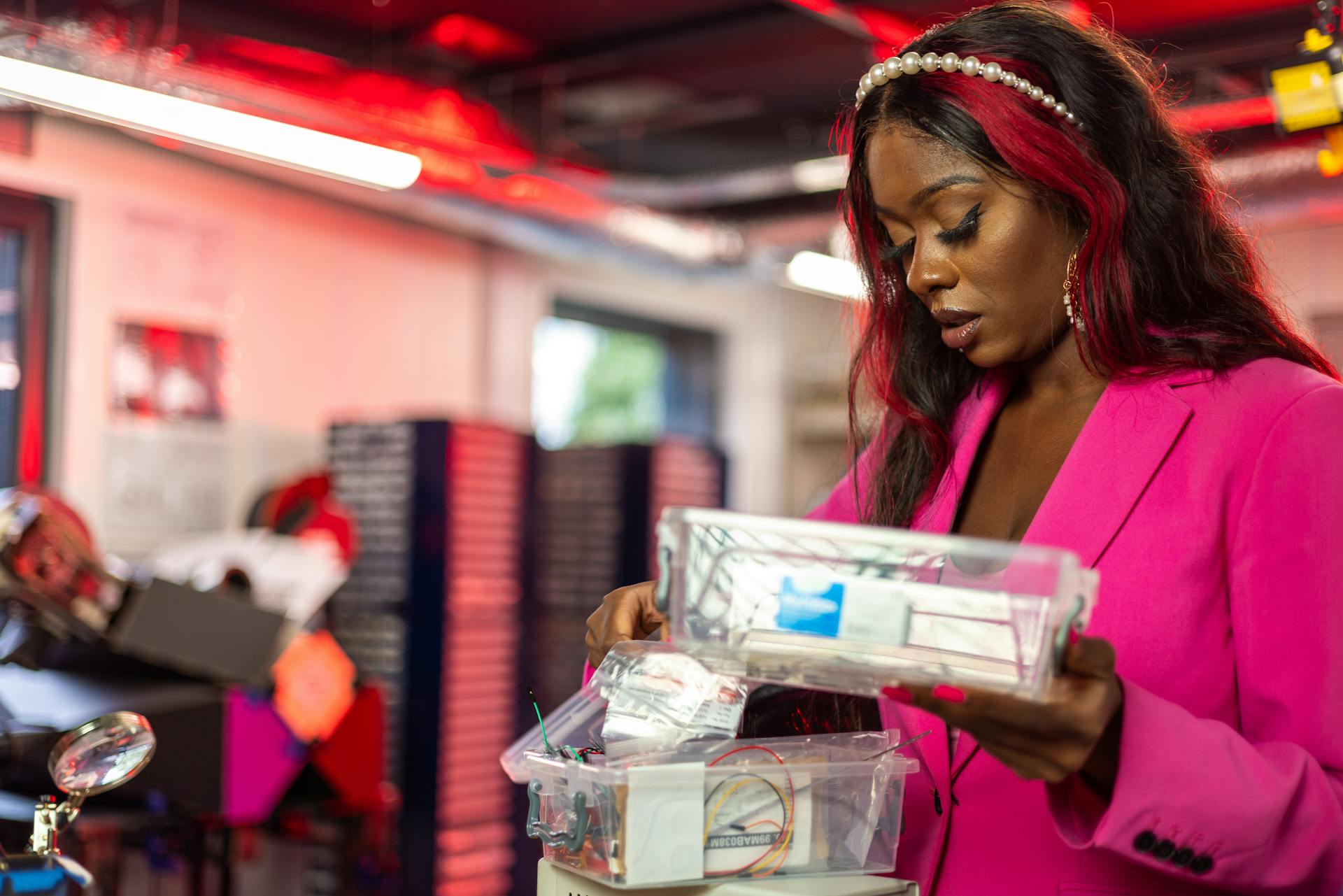
Creating a new Next.js project is a straightforward process that can be completed in just a few minutes.
You can create a new Next.js project by running the command `npx create-next-app my-app` in your terminal.
This command will scaffold a basic Next.js project with the necessary files and folders.
The `package.json` file will be created automatically, listing the dependencies and scripts for the project.
The project will also come with a basic `pages` directory, where you can create your application's routes.
Next.js uses a file-based routing system, where each file in the `pages` directory represents a route in your application.
Expand your knowledge: Next Js App Folder
Getting Started
To get started with Next.js, I recommend using create-next-app, a CLI tool that sets up a new project with everything configured for you. This tool is the easiest way to start building a Next.js application.
You can create a new app using the default Next.js template by running a command in your terminal. This will install dependencies and set up the project for you.
Worth a look: New Nextjs Project Typescript
Once create-next-app finishes, you'll have a production-ready Next.js app configured with React, ESLint, TypeScript, a basic App Router, and development and production builds.
Some key features of create-next-app include setting up Webpack, Babel, and ESLint automatically, creating optimal production and development builds, and making it easy to customize plugins and styling tools like Sass.
To get started quickly, create-next-app also offers a range of official examples, including pre-built templates for common use cases like blog sites, e-commerce stores, and dashboards.
Here are some of the key advantages of using create-next-app:
- Sets up Webpack, Babel, ESLint automatically
- Creates optimal production and development builds for you
- Easy customization for plugins, styling tools like Sass
- Gets you from zero to deploying an app quickly
Project Setup
To initialize a Next.js project, you can use a suitable starter project from GitHub or npm.
You can run `create-next-app` via npm or Yarn to scaffold project files from the starter, and then install dependencies using `npm install` or `yarn`.
The starter should export all necessary build scripts, folder structure, configs, etc, so you can focus exclusively on writing business logic.
Broaden your view: Production Ready Starter Next Js
Here are some key steps to get started:
- Run `create-next-app` via npm or Yarn
- Install dependencies using `npm install` or `yarn`
- Start local development environment using `npm run dev`
- Modify code in `pages/` directory to build application views
- Add additional libraries, features when needed
Some other handy `create-next-app` commands include setting up Webpack, Babel, ESLint automatically, creating optimal production and development builds, and easy customization for plugins and styling tools like Sass.
Selecting a Starter Kit
Create-next-app is a CLI tool that enables you to quickly start building a new Next.js application, with everything set up for you. It handles all the complex configurations, so you can start coding your app right away!
Some key advantages of using create-next-app include setting up Webpack, Babel, ESLint automatically, creating optimal production and development builds for you, and easy customization for plugins and styling tools like Sass.
When evaluating starters, consider aspects like integrations, styling solutions, state management, SEO optimizations, testing setup, UI framework, and deployment platforms. An ideal starter should require minimal ejecting or overwriting just to add basic functionality later.
Here are some key aspects to consider when selecting a starter:
Resist the temptation to choose a complex starter packed with unnecessary features. Start simple, then progressively enhance your app.
Manual Setup
To manually set up a new Next.js project, you'll need to create an empty directory where you want to store your project's files. Then, navigate into this directory in your terminal and run `npm init -y` to initialize a `package.json` file.
You'll need to install some dependencies required for your Next.js project by running `npm install next react react-dom`. Once these dependencies are installed, you can set up your basic folder structure and files.
Create two additional folders named `pages` and `public` inside your root directory. The `pages` folder will contain all of your application's pages, while any static assets such as images or fonts should be placed inside the `public` folder.
To start creating your first page, create a file named `index.js` in the `pages` folder and add the following code: `import { AppProps } from 'next/app';`. You'll also need to add a script to `package.json` so that you can run your project locally.
Expand your knowledge: Create Next Js App
Inside the `scripts` object, add a new property called `dev` and set its value to `next dev`. Now, in your terminal, run `npm run dev` to start your Next.js project on your local server. You can access it by navigating to `http://localhost:3000` in your browser.
Here's a quick rundown of the steps:
- Create an empty directory for your project
- Initialize a `package.json` file with `npm init -y`
- Install dependencies with `npm install next react react-dom`
- Set up your basic folder structure with `pages` and `public` folders
- Create your first page in the `pages` folder
- Add a script to `package.json` for local development
- Run your project locally with `npm run dev`
Global Stylesheets
You can keep your styles separate from your components by using global stylesheets in Next.js. This is a great way to organize your code and make it more maintainable.
To use global stylesheets, create a new folder called styles at the root of your project and place all your CSS files inside it.
This allows you to import styles into your components using the import statement at the top of your component file.
Worth a look: Using State in Next Js
Project Configuration
So you've initialized your Next.js project, and now it's time to configure it. Most starters come with detailed documentation on the setup and initialization process.
Typically, you'll find that the starter exports all necessary build scripts, folder structure, and configs, which you can focus on using to write business logic.
To get started with Next.js, you can use the Create Next App command line tool or manually set up a new Next.js project. This will give you a solid foundation to build upon.
You'll want to run `create-next-app` via npm or Yarn to scaffold project files from the starter. This will create the necessary project files for you.
Next, install dependencies using `npm install` or `yarn`. This will ensure you have all the necessary packages to build and run your application.
You can start the local development environment using `npm run dev`. This will allow you to see your application in action and make changes as needed.
To build application views, modify code in the `pages/` directory. This is where you'll create the views and layouts for your application.
Here's a quick rundown of the typical steps to initialize a Next.js project:
- Run `create-next-app` via npm or Yarn to scaffold project files from the starter
- Install dependencies using `npm install` or `yarn`
- Start local development environment using `npm run dev`
- Modify code in `pages/` directory to build application views
- Add additional libraries, features when needed
CLI and Scripts
Familiarity with the Command Line Interface (CLI) is a must when setting up a Next.js project locally, as most steps require using commands in your terminal or command prompt.
Navigating through directories and executing commands using CLI is a crucial skill to have, especially when working with Next.js.
To run your Next.js application, you'll need to add scripts to your project's package.json file.
These scripts will allow you to run your development server, build your application for production, and start the built application.
A different take: How to Run Nextjs to Build
Adding Scripts to pkg.json
Adding scripts to package.json is a crucial step in setting up your application. To do this, open the package.json file in any text editor of your choice.
In this file, you'll need to add some scripts that will allow you to run your development server, build your application for production, and start the built application. These scripts can be added under the "scripts" section of the file.
Suggestion: Next Js Single Page Application
The scripts you'll want to add are for running the development server, building the application for production, and starting the built application. These scripts are essential for getting your application up and running.
For example, you can add a script to run your development server by adding the following code: "dev": "next". This script will allow you to start your development server with a single command.
Worth a look: Running Json Nextjs
CLI Familiarity
CLI familiarity is a must-have skill for navigating through directories and executing commands using the Command Line Interface (CLI).
Most steps in setting up a Next.js project locally require using commands in your terminal or command prompt.
Familiarity with CLI can save you a lot of time and frustration in the long run.
Broaden your view: Nextjs Usecontext
Overview
Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized web applications.
It's created by Vercel, a platform that provides a suite of tools for building, deploying, and managing web applications.
Next.js is built on top of Node.js and supports both JavaScript and TypeScript.
The framework has a large and active community, with thousands of developers contributing to its ecosystem.
Next.js provides a number of features out of the box, including server-side rendering, static site generation, and internationalization support.
These features make it an ideal choice for building complex, data-driven web applications.
Next.js also supports a number of third-party libraries and tools, including popular frameworks like Redux and React Query.
This makes it easy to integrate additional functionality into your application.
With Next.js, you can build fast, scalable, and maintainable web applications that meet the needs of your users.
Sources
- https://stackoverflow.com/questions/77039686/in-next-js-how-to-init-the-app-no-matter-the-entering-routes
- https://github.com/vercel/next.js/discussions/50198
- https://nextjsstarter.com/blog/next-js-starter-project-guide-from-init-to-deployment/
- https://dev.to/nnnirajn/step-by-step-tutorial-setting-up-a-nextjs-project-locally-for-beginners-4ep3
- https://docs.sst.dev/start/nextjs
Featured Images: pexels.com