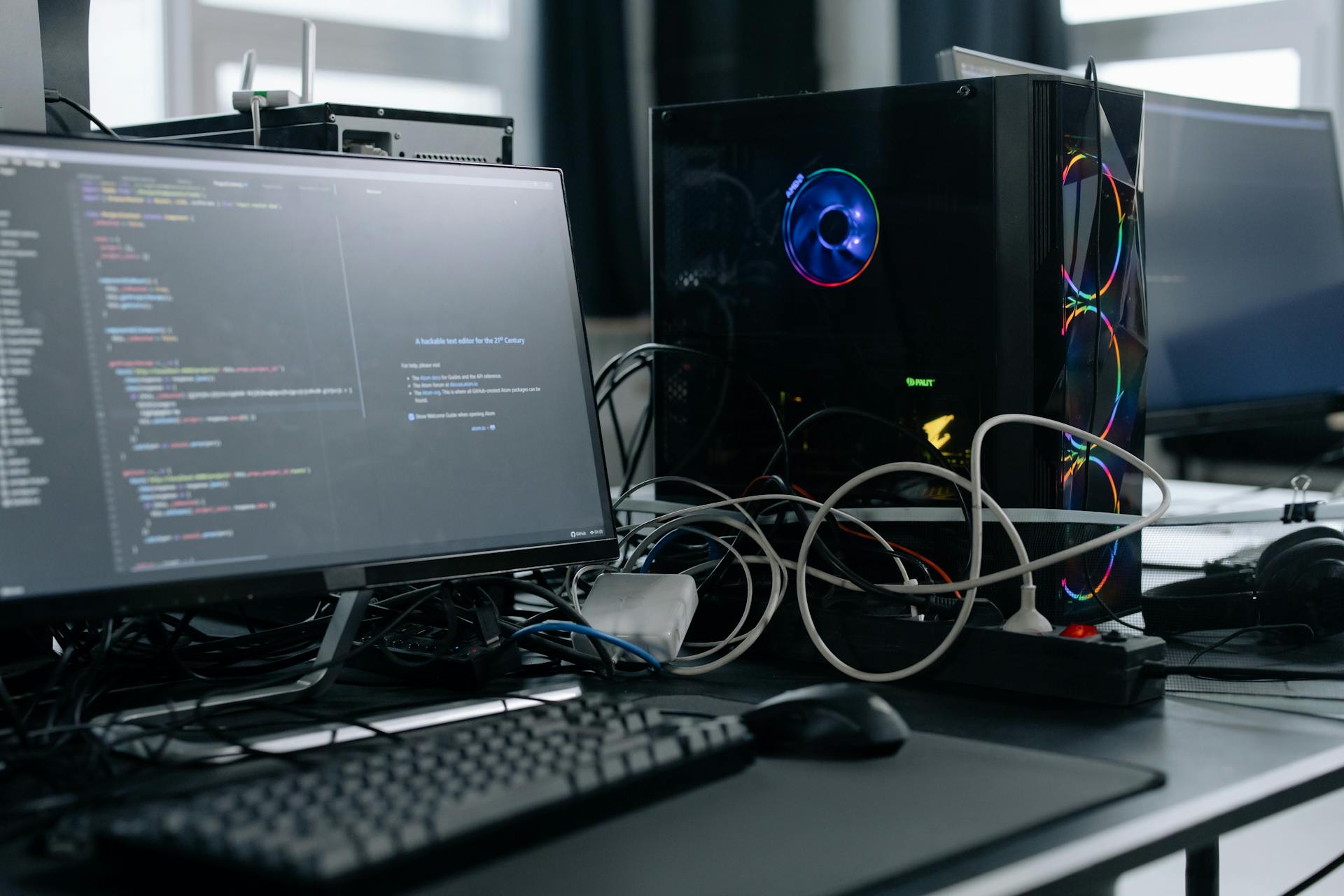
Next.js Prefetching is a powerful technique that helps improve the user experience by loading pages in the background before they're actually needed. This can be especially useful for websites with complex layouts or multiple dependencies.
By using prefetching, you can reduce the time it takes for pages to load, making your website feel faster and more responsive. In fact, prefetching can reduce page load times by up to 50%.
Prefetching works by sending a request to the server for a page before the user clicks on a link to that page. This request is made in the background, so it doesn't interfere with the user's current activity. For example, in the example code, `getStaticProps` is used to prefetch pages in a Next.js project.
Readers also liked: Nextjs Loading Page Ssr
Prefetching in Production
In production builds, Next.js 13 takes prefetching to the next level. It prefetches routes as they become visible in the viewport, which can happen during the initial page load or when the user scrolls through the website.
As soon as a Link comes into view, Next.js proactively fetches and caches the route content. This ensures that when the user clicks on a link to navigate, the page transition is instantaneous.
For your interest: Next Js Build Collecting Page Data
Prefetching Removed
Prefetching is no longer supported in modern browsers. The prefetch keyword for the rel attribute of the link element is a hint to browsers that the user is likely to need the target resource for future navigations.
This technique was used to speed up the loading of web pages by preloading some content in the background before it's actually needed. Prefetching proactively loads the anticipated pages or parts of a website.
Instead of waiting for the user to click on a link and then fetching the content from the server, prefetching loads the content in advance. This way, when the user eventually requests that content, it can be displayed almost instantly.
The browser can likely improve the user experience by preemptively fetching and caching the resource, as hinted by the prefetch keyword.
Consider reading: Next Js Link Component
Production Builds
In production builds, Next.js 13 takes prefetching to the next level by automatically prefetching routes as they become visible in the viewport.
As soon as a Link comes into view, Next.js proactively fetches and caches the route content.
This means that when you scroll through a website, Next.js is working behind the scenes to ensure that the page transition is instantaneous when you click on a link.
In other words, Next.js is prefetching routes even before you click on them, which greatly improves the user experience.
Turning Off
You can disable prefetching for specific links by using the prefetch attribute on the Link component and setting it to false.
This prevents Next.js from prefetching the content when the user hovers over the link, which can be handy for conserving bandwidth.
It can also address privacy concerns related to data access or tracking through prefetching.
Programmatic Prefetching
You can programmatically prefetch routes in Next.js using the prefetch method on the router object returned from the useRouter hook.
By calling router.prefetch() with the full path of a route, you can prefetch that specific route.
Explore further: Nextjs Cache Route
This is particularly useful when you know beforehand that the user is likely to navigate to a particular page.
Prefetching a route in advance ensures a seamless and instant transition without any additional requests.
You can also extend the capabilities of the Link component by introducing a custom component that wraps around it.
This custom component can override prefetch and programmatically prefetch on certain interactions.
Worth a look: Next Js Component Rendering Analyzer
Custom Prefetching Components
You can build a custom component that does link prefetch on hover, similar to how the Link component works in Next.js.
The Link component from next/link accepts an optional prop prefetch, which can be set to true or false. However, these values don't really mean what you might think they do.
Here's a breakdown of what each value means:
To create a custom component that prefetches on hover, you can wrap around the Link component and override the prefetch behavior. This allows you to control prefetching programmatically and prefetch on certain interactions, such as hover.
You can use the prefetch method from useRouter to implement prefetching in your custom routing code. This is especially useful when you want to do something before navigating to a new route, such as submitting a form.
By using custom prefetching components, you can improve the responsiveness of your application by making navigations to new pages quicker. Next.js automatically prefetches the JavaScript needed to render the page, which improves application responsiveness.
Advanced Prefetching Techniques
Prefetching is a technique used to speed up the loading of web pages by preloading some content in the background before it's actually needed.
The prefetch keyword for the rel attribute of the link element is a hint to browsers that the user is likely to need the target resource for future navigations.
By proactively loading anticipated pages or parts of a website, prefetching eliminates any noticeable delay when the user eventually requests that content.
This technique can improve the user experience by preemptively fetching and caching the resource, making it available almost instantly.
Optimizing Navigation
Immediate availability of resources is key to speeding up navigation. With Next.js prefetch, the required resources are loaded in the background, allowing the page to be displayed almost instantly when a user clicks on a prefetched link.
This seamless transition gives the appearance of a very fast, almost instant, navigation experience. Traditional page loads where resources are only fetched after the user clicks the link are significantly improved.
Next.js handles prefetching intelligently, taking into account the user's bandwidth and the priority of resources. It avoids prefetching when it detects a slow network to keep the app responsive.
For your interest: Nextjs Slow Navigation
Fast Internet Only
Prefetching on fast internet only can make a big difference in user experience. By using navigator.connection, you can trigger prefetch only when the user has a stable and fast internet connection. This can help prevent unnecessary delays and improve overall performance.
You can use navigator.connection to check the user's internet speed and quality. This can be a game-changer for users with slow or unreliable internet connections. By disabling prefetching for them, you can prevent unnecessary content downloads and keep their experience smooth.
For example, if you have a link to a page that's only visited occasionally, you can set the prefetch property to false. This way, the content won't be downloaded unnecessarily, saving bandwidth and improving performance.
For more insights, see: Nextjs Performance
Speeding Up Navigation
Immediate availability is key when it comes to user experience, and Next.js delivers just that. With prefetched links, the required resources are loaded in the background, allowing the page to be displayed almost instantly when clicked.
This seamless transition gives the appearance of a very fast, almost instant, navigation experience, which is a significant improvement over traditional page loads.
Next.js handles prefetching intelligently, taking into account the user's bandwidth and the priority of resources. It avoids prefetching when it detects a slow network to keep the app responsive.
Here are some benefits of Next.js prefetching:
- Significantly speeds up navigation as the browser doesn't need to fetch the JavaScript and other resources at click-time.
- Provides a seamless user experience, giving the appearance of a very fast, almost instant, navigation experience.
- Intelligently manages resources, avoiding prefetching when it detects a slow network to keep the app responsive.
Hybrid Approach and Custom Routing
In Next.js, you can use a hybrid approach to prefetch pages, combining server-side rendering and client-side rendering. This allows for more flexibility and control over the prefetching process.
The hybrid approach can be particularly useful for complex pages that require a lot of data to be fetched from the server. By using a hybrid approach, you can render the initial HTML on the server and then use client-side rendering to fetch additional data.
Explore further: Nextjs Rendering
Custom routing is another key feature of Next.js prefetching. By using custom routes, you can define how pages are routed and prefetched. For example, you can use a custom route to prefetch a page only when a specific condition is met.
Custom routing can be achieved using the `getStaticProps` method, which allows you to define how a page should be prefetched. By using this method, you can specify which pages should be prefetched and in what order.
The `getStaticProps` method can also be used to prefetch data for a page, making it easier to manage complex data fetching scenarios. By using this method, you can ensure that the data is prefetched and ready for use when the page is rendered.
For more insights, see: Why Use Next Js
Example and Implementation
In Next.js, prefetching is a powerful feature that allows you to pre-load resources in the background, making your application feel snappier and more responsive.
Next.js starts prefetching pages as soon as the Navbar is rendered, loading resources for /home, /about, and /contact in the background.
This means that when a user clicks on any of these links, the transition to the clicked page is much faster compared to a standard load.
The key benefits of prefetching in Next.js include improved user experience and faster page transitions.
Here's an example of how prefetching works:
- Navbar is rendered, triggering prefetching of pages for /home, /about, and /contact.
- Resources are loaded in the background, making page transitions faster.
By using prefetching, you can make your Next.js application feel more responsive and engaging, which is especially important for users who expect fast and seamless interactions.
Automatic Prefetching
Automatic Prefetching is a technique used to speed up the loading of web pages by preloading some content in the background before it's actually needed. This is done through the Link component in Next.js, which automatically starts prefetching resources for the page that the Link points to when it's included in a Next.js page and rendered into the viewport.
Next.js intelligently detects which links are likely to be clicked by observing which links are in the viewport. Links that are visible on the screen or about to be scrolled into view are considered candidates for prefetching.
Here's how it works:
- Next.js prefetches only links that appear in the viewport.
- It uses the Intersection Observer API to detect them.
- It disables prefetching when the network connection is slow or when users have Save-Data turned on.
Next.js only fetches the JavaScript; it doesn't execute it. That way, it's not downloading any additional content that the prefetched page might request until you visit the link.
Frequently Asked Questions
What does router prefetch do?
Router prefetch optimizes client-side transitions by preloading pages, but it's only useful for Next.js apps without next/link and is a production-only feature
Featured Images: pexels.com