
Nextjs uses Server Side Rendering (SSR) to load pages, which means the server generates the HTML for each page on every request. This approach provides faster page loads and better SEO.
One of the key benefits of Nextjs is its ability to handle complex layouts and data fetching, making it an ideal choice for large-scale applications. Nextjs also provides a built-in mechanism for handling errors and exceptions, ensuring a smooth user experience.
By leveraging the power of SSR, Nextjs can render pages on the server before sending them to the client, resulting in faster page loads and a more seamless user experience. This is especially important for applications that require fast and reliable performance.
You might enjoy: Nextjs App vs Pages
Server-Side Rendering (SSR)
Server-Side Rendering (SSR) is a technique used in Next.js to pre-render pages on the server, which can improve performance and SEO. This is achieved through the use of server-side components and route handlers.
To make authenticated requests to Convex during server rendering, you need to pass a JWT token to preloadQuery or fetchQuery in the third options argument. The implementation of getAuthToken depends on your authentication provider, which can be Clerk or Auth0.
Detecting whether Next.js applications are running on the server side or the client side is crucial to avoid hydration errors. This can be done by using the context parameter in getInitialProps or getServerSideProps.
For your interest: Next Js Component Rendering Analyzer
Client Components
Client Components are an important part of Next.js applications, and lazy loading them can improve performance. You can lazy load client components using the dynamic function from the next/dynamic module.
To do this, you need to set the ssr option to false, which disables server-side rendering for the component. This means the component won't be rendered on the server side, which can improve performance for server-rendered pages.
Lazy loading client components can be especially useful when interacting with the browser's API or making API calls. This approach can help reduce the initial page load time and make your application feel more responsive.
By using the dynamic function and setting the ssr option to false, you can effectively lazy load client components in your Next.js application.
For another approach, see: Page Load Time Bounce Rate
Server vs Client-Side Rendering
Server-side rendering is a powerful technique, but it requires careful consideration of how it affects your application. Detecting whether Next.js applications are running on the server side or the client side is indeed an essential task to avoid unnecessary hydration errors in our application.
Hydration errors occur when there is a mismatch between the server-rendered content and the content rendered on the client side. This can happen when the server and client have different versions of the application, leading to inconsistencies and errors.
Knowing whether the application is running on the client or server side is crucial for preventing such errors. By detecting the rendering environment, you can take measures to ensure that your application behaves correctly in both scenarios.
Hydration errors can be frustrating and difficult to debug, so it's essential to take proactive steps to avoid them.
You might like: Hydration Issue Nextjs
Choosing Between GetInitialProps and GetServerSideProps
getInitialProps is an asynchronous function used for fetching data on the server and pre-rendering the resulting data in Next.js page components.
getInitialProps runs only on the server at the initial page load, but can also run in the browser if you make client-side navigation to other parts of your application and come back to the page.
Explore further: Getinitialprops Next Js
The context parameter is an object containing various keys, but its exact composition is not specified in the article.
getServerSideProps, on the other hand, is also an asynchronous function used for fetching data on the server, but it's not limited to initial page loads.
getServerSideProps returns an object that can be used to populate the props parameter in a page component, just like getInitialProps.
Automatic caching capabilities are mentioned as a feature of getServerSideProps, but it's unclear what this means in practice.
Here's a summary of the key differences between getInitialProps and getServerSideProps:
Ultimately, the choice between getInitialProps and getServerSideProps depends on your specific use case and requirements.
Optimizing Performance
Automatic caching capabilities in Next.js help improve server response times by reducing the number of requests to external services. This is achieved through server-side rendering with caching capabilities.
Next.js automatically adds caching headers to static assets served from /_next/static, including JavaScript, CSS, and static images. This means you don't have to worry about setting up caching manually.
You can set custom headers in getServerSideProps using the response object, but keep in mind that caching doesn't work in development mode.
Automatic Caching Capabilities
Next.js provides a feature called automatic caching capabilities that improve server response times and reduce the number of requests to external services.
This feature is especially useful when dealing with static assets like JavaScript, CSS, static images, and other immutable media.
Next.js automatically adds caching headers to these types of assets served from /_next/static.
You can set a custom header in getServerSideProps using the response object, but keep in mind that caching doesn't work when your application is running in development mode (next dev).
You can learn more about configuration options for the HTTP header field Cache-Control on MDN.
Preloading Client Components
Preloading Client Components is a great way to improve performance in your Next.js application. By preloading data for client components, you can leverage Next.js server rendering while still retaining reactivity after the initial page load.
To achieve this, you can use the preloadQuery function from convex/nextjs. This function takes three arguments: the query reference, an optional arguments object passed to the query, and an optional NextjsOptions object.
Here's a breakdown of the preloadQuery function:
- The query reference: This is the query that you want to preload.
- Optionally the arguments object passed to the query: If your query takes arguments, you can pass them to the preloadQuery function.
- Optionally a NextjsOptions object: This object allows you to customize the behavior of the preloadQuery function.
It's worth noting that preloadQuery uses the cache: 'no-store' policy, which means that any Server Components using it will not be eligible for static rendering.
Advanced Techniques
Next.js provides advanced techniques to enhance your application's performance. Beyond basic component and image lazy loading, you can optimize your pages for faster loading times.
With advanced lazy loading techniques, you can load only the necessary components and images on demand, reducing the initial page load time. This is especially useful for large applications with many components.
By leveraging these advanced techniques, you can create a seamless user experience and improve your application's overall performance.
Here's an interesting read: Lazy Loading Preloader in Nextjs
Custom Flag Approach
You can use a custom flag to lazy load components in Next.js. This approach involves setting a custom flag during server-side rendering and checking for its presence on the client side.
The Intersection Observer API can be used in conjunction with a custom flag to lazy load components more efficiently. This allows you to load resources just before they are needed, improving loading performance.
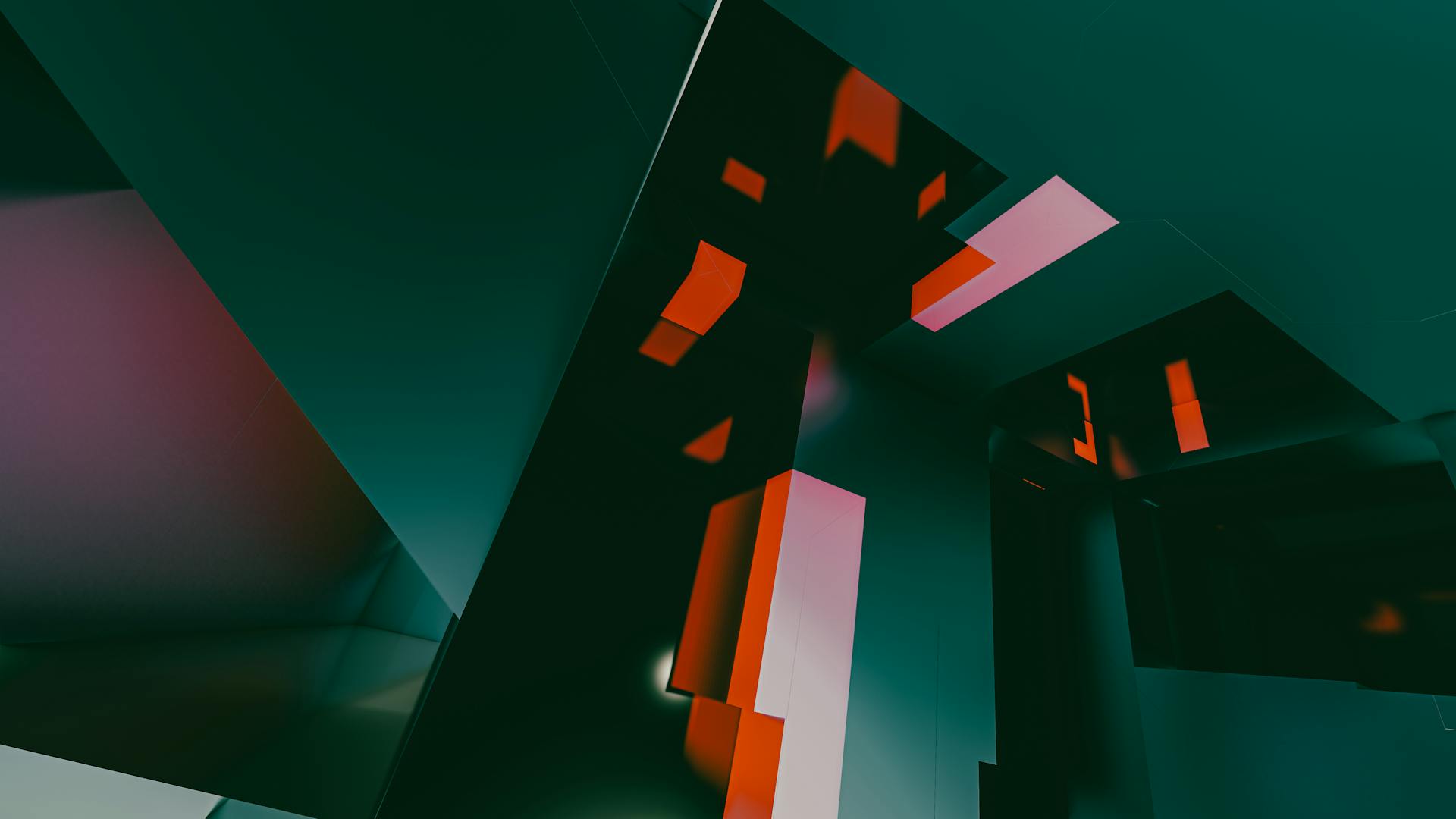
By setting a custom flag, you can control when a component is loaded, reducing the initial bundle size and improving performance. This is particularly useful when dealing with large applications or complex user interactions.
In Next.js, you can use the dynamic import() syntax to split your code into manageable chunks, which can be loaded on demand. This is an example of code splitting, a technique that can be used in conjunction with lazy loading to further improve performance.
Server Components
Server components play a crucial role in handling large volumes of data and traffic.
A server can have multiple CPU cores, with some servers having up to 128 cores, which significantly boosts processing power.
In a cloud environment, a server can be scaled up or down depending on the demand, allowing for more efficient resource allocation.
A load balancer helps distribute incoming traffic across multiple servers, preventing any single server from becoming overwhelmed.
The operating system of a server is responsible for managing hardware resources and providing a platform for running applications.
In a virtualized environment, multiple virtual servers can run on a single physical server, maximizing resource utilization.
A network interface card (NIC) is responsible for connecting a server to a network and facilitating data transfer.
Some servers have a built-in firewall to provide an additional layer of security and protect against unauthorized access.
Server-Side Authentication
Server-Side Authentication is crucial for making authenticated requests to Convex during server rendering. You can pass a JWT token to preloadQuery or fetchQuery in the third options argument to achieve this.
The implementation of getAuthToken depends on your authentication provider. For example, if you're using Clerk or Auth0, you'll need to implement it according to their specifications.
To use preloadQuery, fetchQuery, fetchMutation, and fetchAction in Server Components, Server Actions, and Route Handlers, you must either pass a JWT token to the third options argument or implement getAuthToken correctly.
Here are some authentication providers you can use with Convex:
- Clerk
- Auth0
Note that implementing getAuthToken correctly is key to making authenticated requests to Convex.
Best Practices
To get the most out of Next.js's Server-Side Rendering (SSR), it's essential to follow best practices.
Optimize your components by using the `getStaticProps` method, which allows you to pre-render pages at build time. This can significantly improve page loading times.
Use the `useEffect` hook to handle side effects, like API calls, only when the component is mounted. This ensures that your component is not re-rendered unnecessarily.
Minimize the use of `getServerSideProps` in favor of `getStaticProps` whenever possible, as it can lead to faster page loads and improved SEO.
By following these best practices, you can unlock the full potential of Next.js's SSR and create fast, efficient, and scalable web applications.
Broaden your view: Server Side Rendering Next Js
Conclusion
Next.js is a powerful tool for server-side rendering, and understanding its features is crucial for a smooth development experience.
The Next.js developers recommend using getServerSideProps over getInitialProps since the former has extra features such as automatic caching.
This means that getServerSideProps is a more efficient choice for handling server-side rendering.
Automatic caching is a significant advantage, as it can improve page load times and reduce the load on your server.
By using getServerSideProps, you can handle all use cases for server-side rendering, making it a more versatile option.
Sources
- https://refine.dev/blog/next-js-getinitialprops-and-getserversideprops/
- https://www.dhiwise.com/post/implementing-next-js-lazy-loading-for-optimized-web-apps
- https://nextjs.org/docs/app/building-your-application/rendering/server-components
- https://docs.convex.dev/client/react/nextjs/server-rendering
- https://www.geeksforgeeks.org/detecting-server-vs-client-in-nextjs-application/
Featured Images: pexels.com