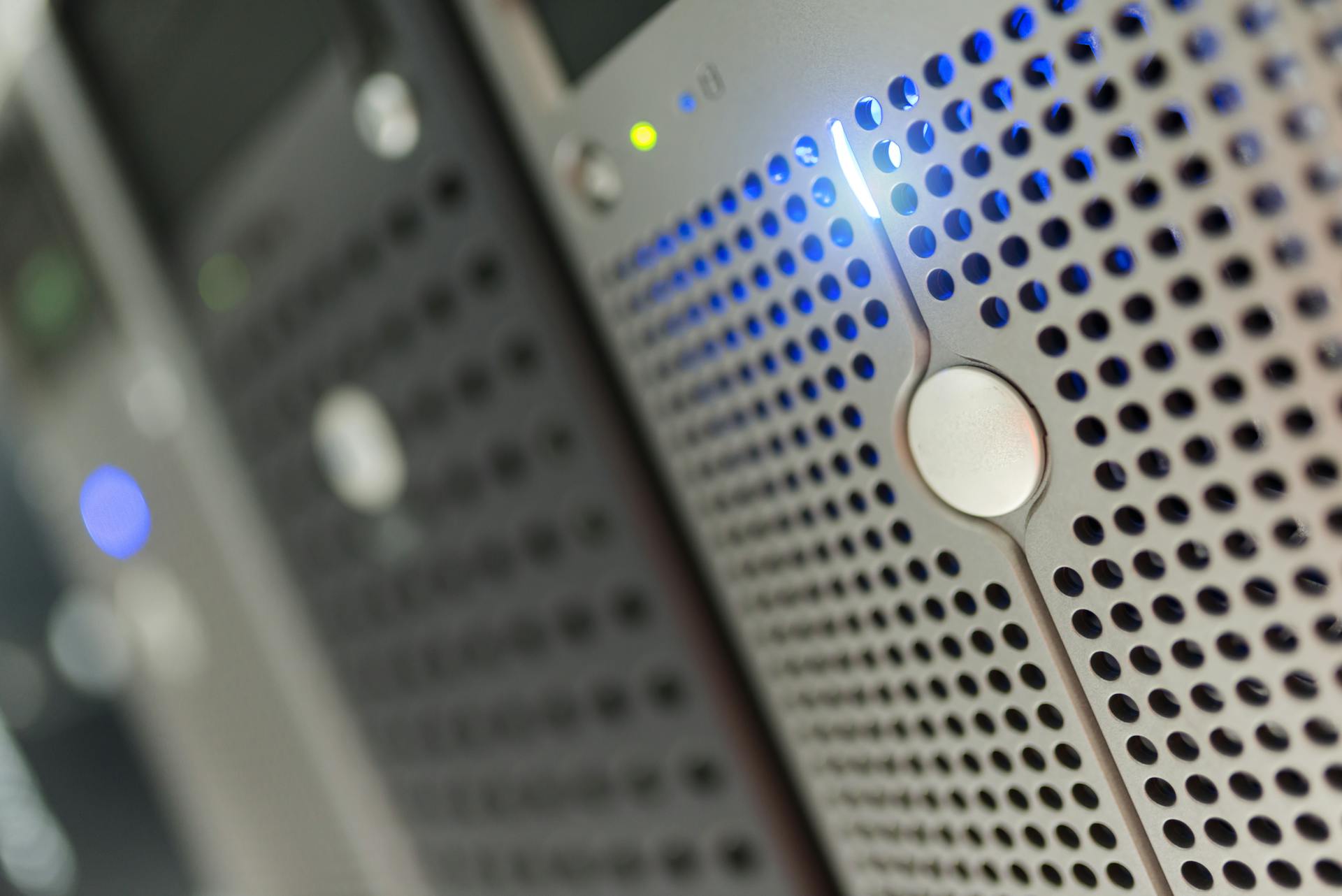
Next.js Cache Route is a powerful feature that can significantly improve the performance of your application. It enables caching of routes, which can reduce the number of requests made to the server and improve page load times.
By caching frequently accessed routes, Next.js Cache Route reduces the load on the server and speeds up page rendering. This is particularly beneficial for applications with high traffic or complex routes.
Next.js Cache Route uses a simple and efficient caching mechanism that stores the rendered pages in memory. This allows the application to serve cached pages quickly, without having to re-render the page from scratch.
Caching in Next.js
Caching in Next.js can be a bit tricky, but don't worry, I've got you covered.
The App Router has four separate caches, including fetch request and route caches. The route cache is the most likely to cause issues.
If your application accepts login and renders different data based on the user, you'll need to disable the route cache by using the dynamic export from the route handler. This is because the route cache can get stuck on an outdated version of the route.
After a mutation, you should invalidate the cache by calling revalidatePath or revalidateTag as appropriate. This ensures that the cache is updated and reflects the latest changes.
App Router Optimizes Performance
App Router significantly enhances page speed and user experience by leveraging React Server Components (RSC) to render and cache HTML on the server.
Data fetching is performed on a server, reducing the time it takes to retrieve data from a server located closer to the data source.
Caching results across multiple users reduces the need for repeated fetching and rendering, making the process more efficient.
Rendering components on the server means less JavaScript is sent to a user's browser, which is beneficial for users on slow connections and less powerful devices.
Here are the benefits of App Router's performance optimization:
- Data fetching is performed on a server, reducing the time it takes to retrieve data.
- Caching results across multiple users reduces the need for repeated fetching and rendering.
- Less JavaScript is sent to a user's browser, making it beneficial for users on slow connections and less powerful devices.
Next.js enhances this process further by using static rendering for pages where request time information isn’t needed, rendering content at build time and then caching on a CDN, significantly boosting page speed and efficiency.
Implementing Cache
The App Router has four separate caches, but the most likely one to cause issues is the route cache. You can disable it by using the dynamic export from the route handler.
To invalidate the cache after a mutation, you should call revalidatePath or revalidateTag as appropriate. This ensures that the cache is updated with the latest changes.
Next.js App Router optimizes frontend performance by leveraging React Server Components (RSC) to render and cache HTML on the server. This significantly enhances page speed and user experience.
Data fetching is performed on a server, reducing the time it takes to retrieve data. Since data fetching is performed on a server, it's possible to cache results across multiple users, reducing the need for repeated fetching and rendering.
Here are the benefits of using server-side rendering:
- Data fetching: Server-side rendering enables fetching data from a server located closer to the data source, reducing the time it takes to retrieve data.
- Caching: Since data fetching is performed on a server, it's possible to cache results across multiple users, reducing the need for repeated fetching and rendering.
- Bundle size: Rendering components on the server means less JavaScript is sent to a user's browser.
Next.js enhances this process further by using static rendering for pages where request time information isn’t needed. This means the content is rendered at build time and then cached on a CDN, significantly boosting page speed and efficiency.
Verifying Cache
The route cache is the most likely cache to cause issues, especially if your application has routes that render different data based on the user.
To disable the route cache, you'll need to use the dynamic export from the route handler. This will prevent caching issues.
After a mutation, you should invalidate the cache by calling revalidatePath or revalidateTag as appropriate.
You can check your work by verifying that the data in the Redux store is present in the server-side rendered output.
Here are three key areas to check:
- Server Side Rendering: Check the HTML output of the server to ensure that the data in the Redux store is present.
- Route Change: Navigate between pages on the same route as well as between different routes to ensure that route-specific data is initialized properly.
- Mutations: Check that the store is compatible with the Next.js App Router caches by performing a mutation and then navigating away from the route and back to the original route.
Frequently Asked Questions
Does Next.js cache dynamic routes?
No, Next.js does not cache dynamic routes by default. However, you can configure caching for dynamic routes in certain situations
What is the difference between React cache and Next.js cache?
Next.js cache stores responses on disk, reducing backend queries, whereas React cache relies on client-side deduplication
How do you clean the cache in next?
To clean the cache in Next.js, delete the contents of the .next directory and rebuild your application. This will generate fresh build artifacts and restart your application.
Sources
- https://nextjs.org/docs/app/api-reference/directives/use-cache
- https://nextjs.org/docs/app/building-your-application/routing/linking-and-navigating
- https://redux.js.org/usage/nextjs
- https://www.contentful.com/blog/integrate-contentful-next-js-app-router/
- https://nextjs.org/docs/app/building-your-application/caching
Featured Images: pexels.com