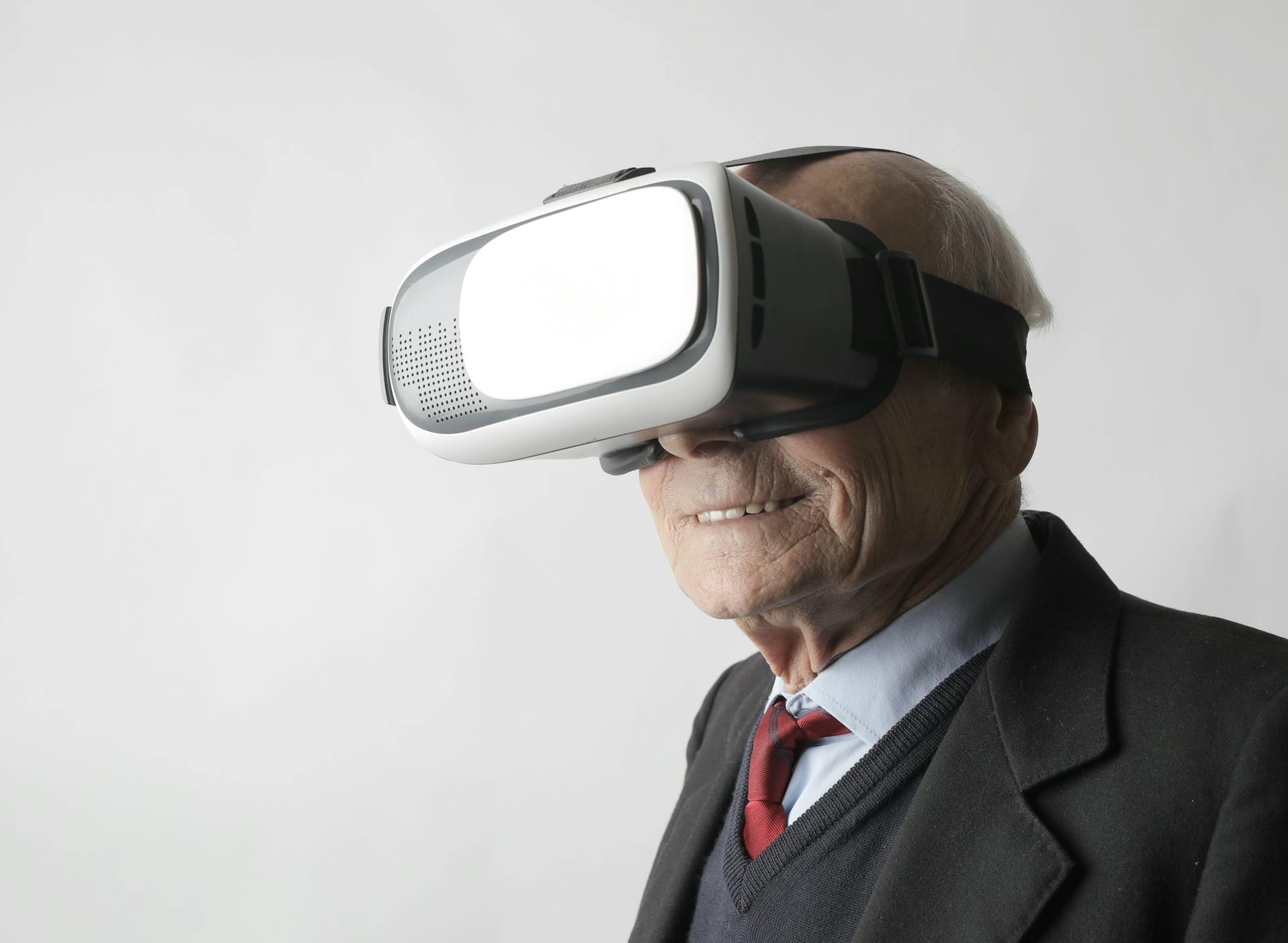
NextJS slow navigation performance issues can be frustrating, especially when you're trying to optimize your app for better user experience. One of the main reasons for this issue is the way NextJS handles routing, which can lead to slow navigation.
To fix this, you need to understand how NextJS handles routes. According to the documentation, NextJS uses a client-side routing system, which can cause delays if not optimized properly.
One simple fix is to use the `getStaticProps` method to pre-render pages. This can significantly improve navigation speed, especially for static pages.
Additionally, you can use the `getStaticPaths` method to pre-render pages at build time, which can also improve navigation speed.
Related reading: Dropbox Upload Speed Slow
Why Is It So Slow?
The navigation in Next.js can be slow due to the page navigation waiting until `getServersideProps` is ran. This is a key issue that needs to be addressed.
The internal routing engine is being used for links, which avoids a full reload of the page. However, this doesn't necessarily speed up the navigation.
Expand your knowledge: Tailwindui Navigation in Nextjs
This change to internal routing is a step in the right direction, but it's not enough to overcome the delay caused by `getServersideProps`. It's a crucial function, but it's also a bottleneck.
The slow navigation is a trade-off for the benefits of using a next-gen routing engine. It's a compromise that developers need to be aware of.
Using internal routing for links is a good start, but it's just one piece of the puzzle. The real solution lies in optimizing `getServersideProps` and other server-side rendering functions.
Optimizing Next.js Performance
Avoiding unnecessary fetches can significantly speed up navigation. This can be achieved by storing the navigation data in a local state and checking if the request URL starts with /_next/data.
Storing navigation data in a local state is a good practice. This can be done by setting a variable, such as stateNavigation, once the page component is mounted.
Checking the request URL is a simple way to determine if the navigation data has already been fetched. If the URL starts with /_next/data, it means the data has already been fetched.
Intriguing read: Next Js Build Collecting Page Data
Skipping unnecessary calls can save a lot of time. By trusting that the first fetched data is still valid, we can avoid refetching the navigation data.
Navigating internally within the app can result in a URL that starts with /_next/data. This is because the navigation data has already been fetched once.
Trusting that the first fetched data is still valid is a good strategy. This can save a call and make page transitions much faster.
Next.js App Router Migration
The Next.js App Router is a significant improvement over the traditional Page Router, offering better performance and scalability.
It's designed to handle complex routing scenarios and provides a more flexible way to manage routes.
One of the key benefits of the App Router is its ability to render routes on demand, reducing the initial page load time.
This is achieved through the use of a new routing system that uses a combination of routes and layouts to render pages.
For your interest: Next Js Parallel Routes
To migrate to the App Router, you'll need to update your `next.config.js` file to use the `app` router.
This involves changing the `router` option to `app` and configuring the `appDir` option.
By using the App Router, you can take advantage of features like automatic code splitting and improved performance.
These benefits can lead to a significant reduction in page load times and a better overall user experience.
In addition to performance improvements, the App Router also provides a more intuitive way to manage routes.
This is achieved through the use of a new API that allows you to define routes using a more declarative syntax.
To get started with the App Router, you'll need to create a new `app` directory in your project root.
This directory will contain the new routing configuration and layout files.
The App Router also provides support for server-side rendering (SSR) and static site generation (SSG).
These features allow you to render pages on the server and generate static HTML files, respectively.
By following these steps and updating your project to use the App Router, you can take advantage of its many benefits and improve the performance of your Next.js application.
Worth a look: Why Use Next Js
Frequently Asked Questions
Is Next.js 14 slow?
Upgrading to Next.js 14.1 may cause compilation slowdowns in development mode, affecting productivity. Learn how to optimize your project for faster development times
Sources
- https://rasmussendev.medium.com/avoiding-refetching-data-in-nextjs-when-navigating-df673ef491f
- https://www.flightcontrol.dev/blog/nextjs-app-router-migration-the-good-bad-and-ugly
- https://www.radix-ui.com/primitives/docs/components/navigation-menu
- https://mariogiancini.com/implementing-smooth-scroll-behavior-with-tailwind-css-and-nextjs
- https://nextjs.org/docs/app/building-your-application/routing/loading-ui-and-streaming
Featured Images: pexels.com