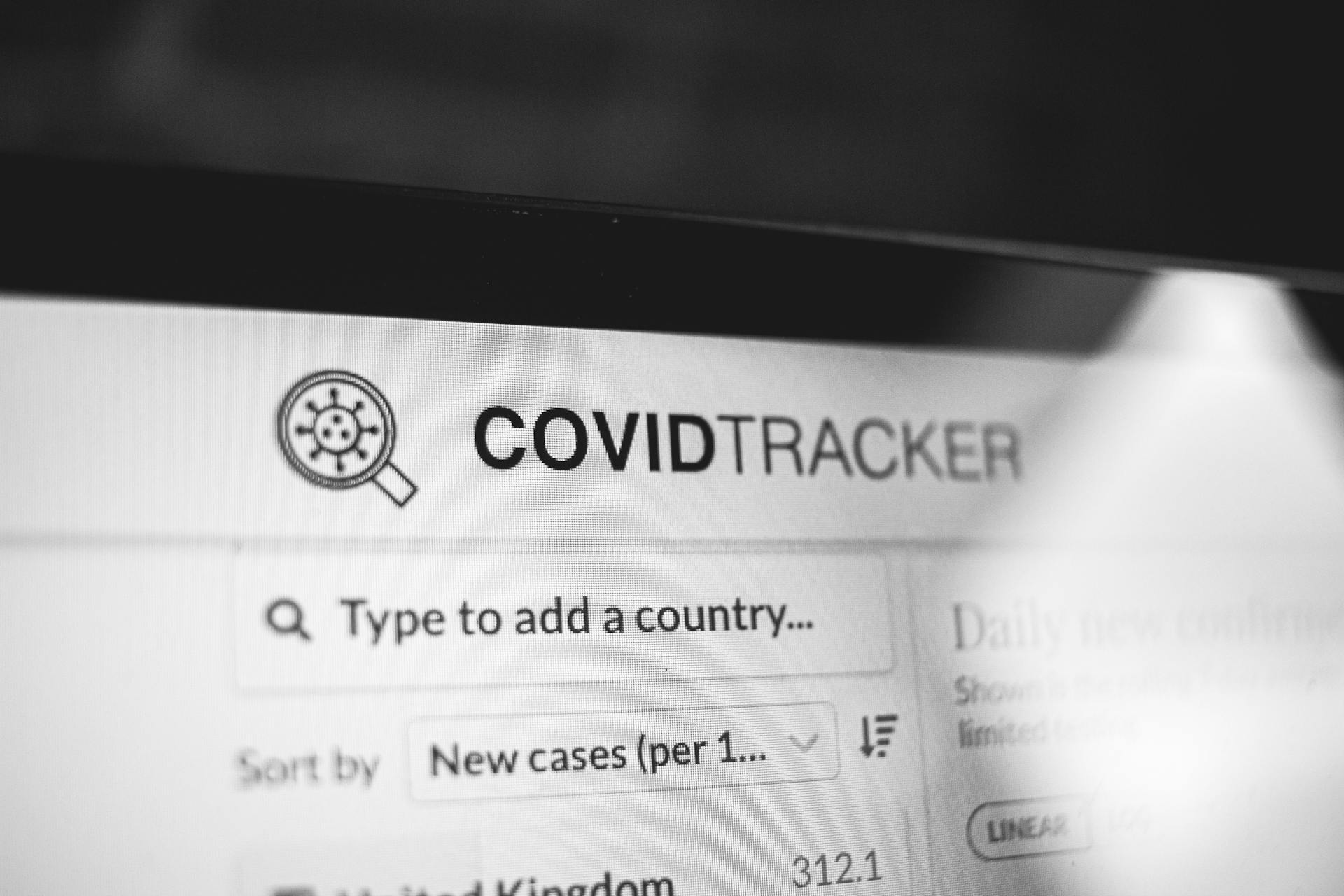
Streamlining your Next.js app with TailwindUI navigation can be a game-changer. By using TailwindUI, you can create a consistent and visually appealing navigation system that enhances user experience.
TailwindUI provides pre-designed navigation components that can be easily integrated into your Next.js app, saving you time and effort. One such component is the navigation bar, which can be customized to fit your app's branding.
A well-designed navigation bar is crucial for user engagement, as it helps users quickly find what they're looking for. In our example, we customized the navigation bar to include a search icon, which improved user experience by providing an additional way to navigate the app.
With TailwindUI, you can create a navigation system that adapts to different screen sizes and devices, ensuring a seamless user experience across all platforms.
Discover more: Nextjs Tailwindui Nav
Navbar Components
Creating a navbar component in Next.js is a crucial step in building a responsive and user-friendly interface. You can't directly style the Link component, so you'll use an anchor tag instead.
Props are a powerful feature in React that allow you to pass data between components, making it easier to manage your application's state. This is especially useful when creating reusable components like the navbar.
To pass data through props, you can use destructured attributes when defining the component, like we did in the Navbar component. This allows you to customize the links and their destinations when importing the navbar.
The Navbar component is designed to be responsive, with a mobile menu button that appears only on smaller screen sizes. We use Tailwind CSS classes to center the navbar's contents and add padding to create a visually appealing layout.
The logo component can be styled with a className to add a hover effect, increase font size, and make the font bold. This gives your navbar a professional and modern look.
The navbar's layout is set using flexbox, which centers the contents and spaces out the components automatically. We also set the background color, padding, and text color using Tailwind CSS classes.
To hide the mobile menu button on larger screens, we use a media query to toggle the display based on the screen size. This ensures a seamless user experience across different devices.
Finally, we add a function to the menu button's onClick prop to toggle the visibility of the mobile menu links. This is done using JavaScript DOM manipulation to select the mobile-menu link section and toggle the "hidden" class.
Expand your knowledge: Next Js Button
Navbar Features
The Navbar Features section is where the magic happens, and we get to explore the various styles and functionalities that make our navigation bar truly shine.
You can create a nice subtle hover effect on the logo by adding a specific className to the anchor tag, which will increase the font size, make it bold, and add some letter spacing.
The bulk of the work for styling the navbar actually happens in the Navbar.js file, where you can set the layout using flexbox, center the contents vertically, and add some padding and margin to create a clean and modern look.
The Navbar.js file also includes a className that hides the mobile nav links on any screen bigger than 768px, making the menu button only appear on mobile viewports.
Navbar Component Styling
The Navbar Component Styling is a crucial aspect of creating an engaging and user-friendly navbar. You can add a nice subtle hover effect to the logo by adding a specific className to the anchor tag in your Logo.js file.
Explore further: Scrolling Logo Bar Next Js
This className not only creates a nice hover effect but also increases the size of the font, makes the font bold, and increases the letter spacing. The result is a visually appealing logo that grabs the user's attention.
To style the navbar itself, you need to add a className to the top-level div in Navbar.js. This className sets the layout of the navbar using flexbox, centers the contents vertically, and spaces out the components automatically.
It also sets the background color of the navbar to black, adds a padding of 32px or 2rem to the left and right of the navbar, sets the left and right margin of the navbar to auto, and sets the color of all the text in the navbar. This creates a clean and modern look for your navbar.
You can also hide the mobile nav links on larger screens by adding a className to the second div with Links inside. This className will only show the links on mobile viewports, providing a better user experience on smaller screens.
To implement the toggle functionality for the menu button, you need to add an onClick function that toggles the "hidden" class from the mobile-menu link section. This is done by selecting the mobile-menu link section and toggling the "hidden" class using JavaScript DOM manipulation.
Worth a look: Nextjs Link
Responsive and Reusable Navbar Strategy
Creating a responsive and reusable navbar is a great way to enhance the user experience of your application. It's essential to plan out the steps before diving into the code.
We create and export the function Logo, which returns the Link component with a HTML anchor tag inside, to make it available in other files and reusable throughout the application.
The reason we have an anchor tag inside the Link is because you can't directly style the Link component, so we will style the anchor tag instead.
To make our navbar responsive, we use tailwind's mobile-first orientation, which means the link section will be hidden on mobile and centered and spaced out on screens bigger than 768px.
Here are the key steps to create a responsive and reusable navbar:
- Creating the component directory
- Creating Logo.js
- Creating Navbar.js
- Importing the relevant components (Logo and Link from Next.js)
- Importing Navbar in index.js
- Writing the markup code
- Styling the markup
Props are an integral feature of React, making it possible to pass data between components, which makes our lives easier. We can pass data through props by giving values to the attributes that we destructured when we defined the component.
To add a nice subtle hover effect to the logo, we add the className "hover:text-blue-600 hover:text-lg hover:font-bold hover:leading-loose" to the anchor tag in the Logo.js file.
To style the navbar component, we add the className "flex justify-between items-center py-4" to the top-level div in Navbar.js, which sets the layout of the navbar using flexbox and centers the contents vertically.
Here are the key classes used to style the navbar:
Customization and Configuration
Tailwind CSS is a composable, utility-first CSS framework that can be configured and used to build any design. It's very useful for mobile screen-size development since it uses a mobile-first approach by default.
Tailwind CSS has advantageous features like enabling faster CSS styling since developers have to write relatively less CSS, and being highly customizable. This makes it easier to build responsive and adaptable user interfaces.
To configure Tailwind CSS, we need to create a new file named postcss.config.js in the project's root directory and add the necessary code to set up Tailwind CSS and include PurgeCSS to remove unused CSS in production builds.
Here are some of the key configuration options for Tailwind CSS:
- Mobile-first approach by default
- Enables faster CSS styling
- Highly customizable
- Uses a common utility classes pattern
By configuring Tailwind CSS correctly, we can take advantage of its benefits and create a robust and responsive navigation system using Tailwind UI in our Next.js project.
Installation and Configuration
Installation and configuration of Tailwind CSS is a straightforward process. You can install it and its dependencies using npm or yarn, and then configure it to work with Next.js.
Tailwind CSS is a composable, utility-first CSS framework that can be configured and used to build any design. It has a mobile-first approach by default, making it very useful for mobile screen-size development.
Here are some advantageous features of Tailwind CSS:
- Mobile-first approach by default
- Enables faster CSS styling
- Common utility classes pattern
- Highly customizable
To configure Tailwind CSS, you'll need to create a new file named postcss.config.js in your project's root directory. This file will set up Tailwind CSS, add necessary PostCSS plugins, and include PurgeCSS to remove unused CSS in production builds.
After installing Tailwind CSS, you'll need to configure it to work with Next.js. You can do this by initializing Tailwind CSS using the following command: `npx tailwindcss init`. This will create a new file named tailwind.config.js, which you can use to configure Tailwind CSS to fit your specific needs.
The tailwind.config.js file is where you can customize your Tailwind CSS installation. You can replace the default contents of this file with custom code to suit your project's needs.
To complete the configuration process, you'll need to add some code to your globals.css file. This file is located in your styles directory, and you can add the following lines of code at the top of the file:
Dark Navbar
The Dark Navbar is a great way to create a cohesive design in your application. It uses a gradient background styled with Tailwind CSS (bg-slate-900) to achieve a dark mode design.
This design element incorporates a search input field with a unique label animation. The label ("Type here...") is styled to appear within the search field when it's not focused and moves above the search field when it is focused, providing a clear, interactive cue for users.
By using a gradient background, you can create a visually appealing and professional-looking navbar that sets the tone for your application's design.
Layout Planning
Layout planning is a crucial step in building a website. It involves sketching out the desired layout of your website before diving into the implementation.
Consider the placement of elements such as the header, navigation, main content, sidebar, and footer. These elements will be present across different pages, so it's essential to get their placement right.
To create a layout component in your Next.js project, simply create a new component that will serve as the layout for your website. This component will be responsible for rendering the header, footer, and other common elements.
In your layout component, use the grid class to define the overall structure. Tailwind CSS provides a responsive grid system that simplifies creating flexible layouts.
To set up the grid system, define the overall structure within your layout component using the grid class.
Sources
- https://dremukare.hashnode.dev/creating-reusable-navigation-bar-with-nextjs-and-tailwindcss
- https://flowbite.com/docs/getting-started/next-js/
- https://www.material-tailwind.com/docs/html/navbar
- https://www.freecodecamp.org/news/how-to-set-up-tailwind-css-with-next-js/
- https://webkul.com/blog/tailwind-css-with-material-ui/
Featured Images: pexels.com