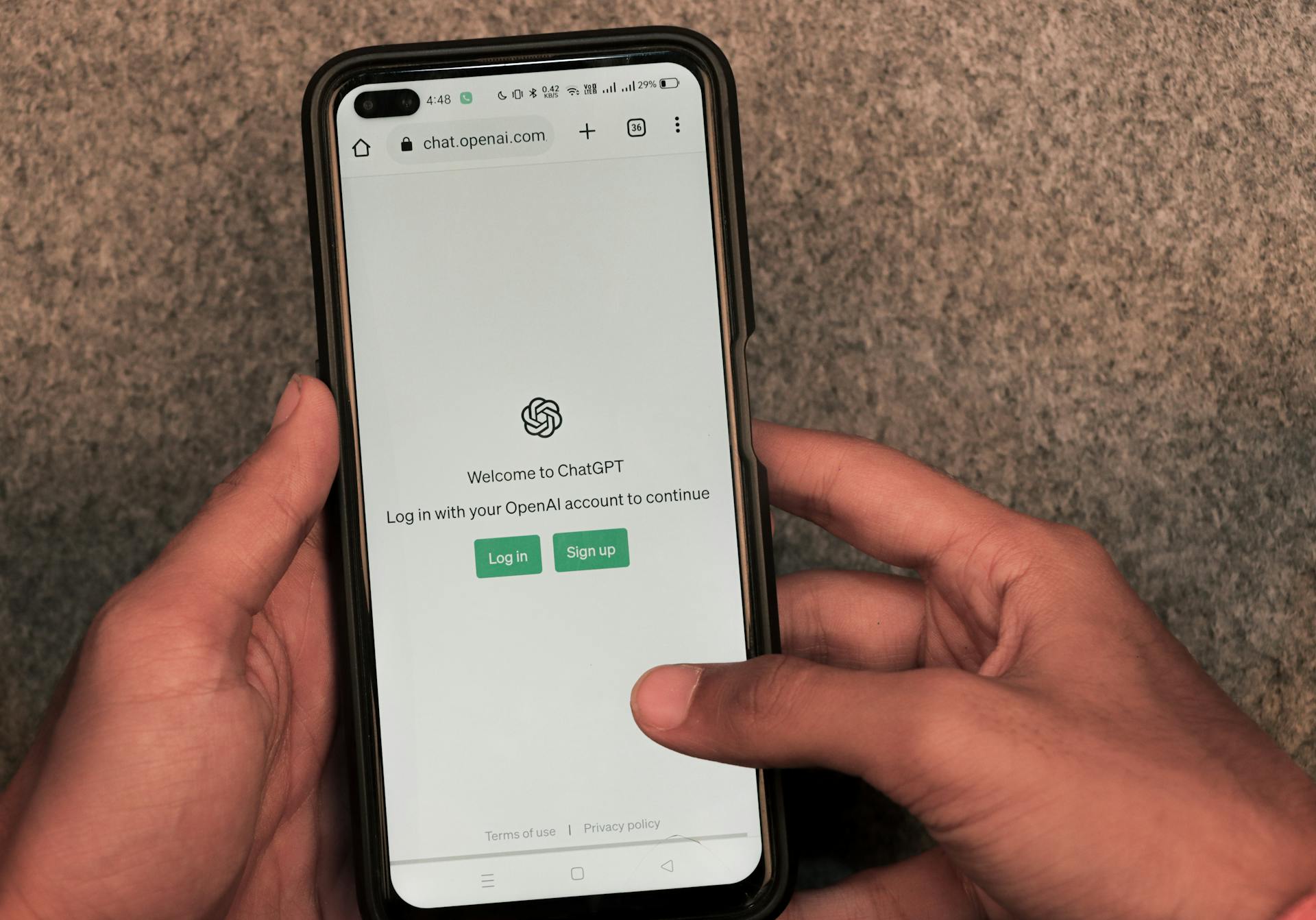
Next.js and TailwindCSS are a powerful combination for building fast and scalable applications. With Next.js, you can create server-side rendered (SSR) pages that load quickly and improve SEO.
TailwindCSS, on the other hand, provides a utility-first approach to styling that makes it easy to create responsive and accessible designs. By using these two frameworks together, you can create a seamless navigation experience for your users.
One of the key benefits of using Next.js and TailwindCSS is that you can easily customize the navigation bar to fit your application's needs. For example, you can change the background color, text color, and padding of the navigation bar using TailwindCSS classes.
In the next section, we'll dive deeper into how to customize the navigation bar using Next.js and TailwindCSS.
If this caught your attention, see: Create Next Js Project
Navbar Setup
To start building a responsive navbar, software engineers first solve the problem, then write the code. This means planning out the steps to create the navbar.
We'll create a component directory, logo, and navbar components, and import relevant components from Next.js. We'll also import the navbar in index.js to view our changes.
We create and export the function Logo, which returns the Link component with an HTML anchor tag inside. This allows us to reuse the Logo component wherever we want in our application.
To style the navbar, we use flexbox to center the contents vertically and space out the components automatically. We also set the background color to black, add padding, and set the text color.
Here are the steps to style the navbar:
- Add the className to the top-level div to set the layout and background color.
- Add the className to the div containing the links to hide them on mobile and center them on larger screens.
- Add the className to the second div with links inside to style the mobile menu.
- Write the onClick function to toggle the "hidden" class on the mobile-menu link section.
We use the JavaScript DOM manipulation to select the mobile-menu link section and toggle the "hidden" class. We also use the onClick property to call the showMenu function to hide and show the menu for respective devices.
To make the navbar appear on all pages, we can import it in the root level file _app.js. This way, we can use a common navbar in every page of our application.
Building the Navbar
First, let's create a responsive and reusable navbar by following a clear strategy. This involves planning how we'll build the navbar, which includes creating a component directory, importing relevant components, and writing the markup code.
We start by creating the component directory and then create two new files: Logo.js and Navbar.js. In Logo.js, we export a function that returns the Link component with an HTML anchor tag inside, making it reusable throughout our application.
To style our navbar, we use Tailwind CSS classes to set the layout using flexbox. We center the contents of the navbar vertically and automatically space out the components. We also set the background color to black, add padding, and set the color of all the text in the navbar.
Here are the Tailwind CSS classes used to style the navbar:
We also add classes to hide the button on screens bigger than 768px and to style the links for the mobile menu.
Breadcrumb Navigation
Breadcrumb navigation is a crucial aspect of any website, and in Next.js, we can easily create a breadcrumb navigation system using Tailwind UI. To start, we create a new directory called components, where we will put our Breadcrumb and BreadcrumbItem components.
The Breadcrumb component accepts one prop, children, which includes the breadcrumb items, or links to the pages. We convert the children into an array using the Children.toArray() function, and then map over it to render each breadcrumb item.
Each breadcrumb item is a list item, or li, and is rendered inside an ordered list, or ol. The space-x-4 class adds a margin of 1 rem between all children elements. The breadcrumb component returns a nav element with the ordered list.
We also create a BreadcrumbItem component, which takes in a children and a href prop, as well as additional props that will be passed into the li prop. This component is used to render each individual breadcrumb item.
A unique perspective: Next Js Using State Context
To add the breadcrumb to our application, we import the Breadcrumb component and add it to our index.js file under pages. We also add the BreadcrumbItem component to render each breadcrumb item.
However, manually passing in the breadcrumb items is not suitable for larger applications. To dynamically create the breadcrumb items, we make some changes to the _app.js file.
We initialize the router and create a state to store our breadcrumbs. We also use an useEffect hook to fire when the page loads and whenever the router.asPath changes. In the useEffect hook, we remove query parameters from the path, split it into an array, and filter out any blank paths.
We then map over the path array to generate a new array of breadcrumb objects, containing the href and label for each breadcrumb item. We save this array to the breadcrumbs state, which we can then use outside the useEffect hook.
To display the dynamic breadcrumb, we add the Breadcrumb component and a BreadcrumbItem for the home route. We then map over the breadcrumbs array and add the other BreadcrumbItem components.
We can also pass a prop to the BreadcrumbItem called isCurrent with a boolean value, which is true for the last breadcrumb item, or the current page. This allows us to style the current breadcrumb item differently if needed.
With these components and dynamic breadcrumb generation, we can easily create a breadcrumb navigation system in Next.js using Tailwind UI.
Here's an interesting read: How to Add Img to Nextjs
Sources
- https://dremukare.hashnode.dev/creating-reusable-navigation-bar-with-nextjs-and-tailwindcss
- https://letmefail.com/react/responsive-mobile-navigation-with-nextjs-and-tailwindcss/
- https://blog.foujeupavel.com/build-and-deploy-a-fullstack-app-with-typescript-next-js-graphql-tailwind-css-and-aws-part-2/
- https://blog.anishde.dev/making-an-accessible-breadcrumb-navigation-using-tailwindcss-and-nextjs
- https://flowbite.com/docs/getting-started/next-js/
Featured Images: pexels.com