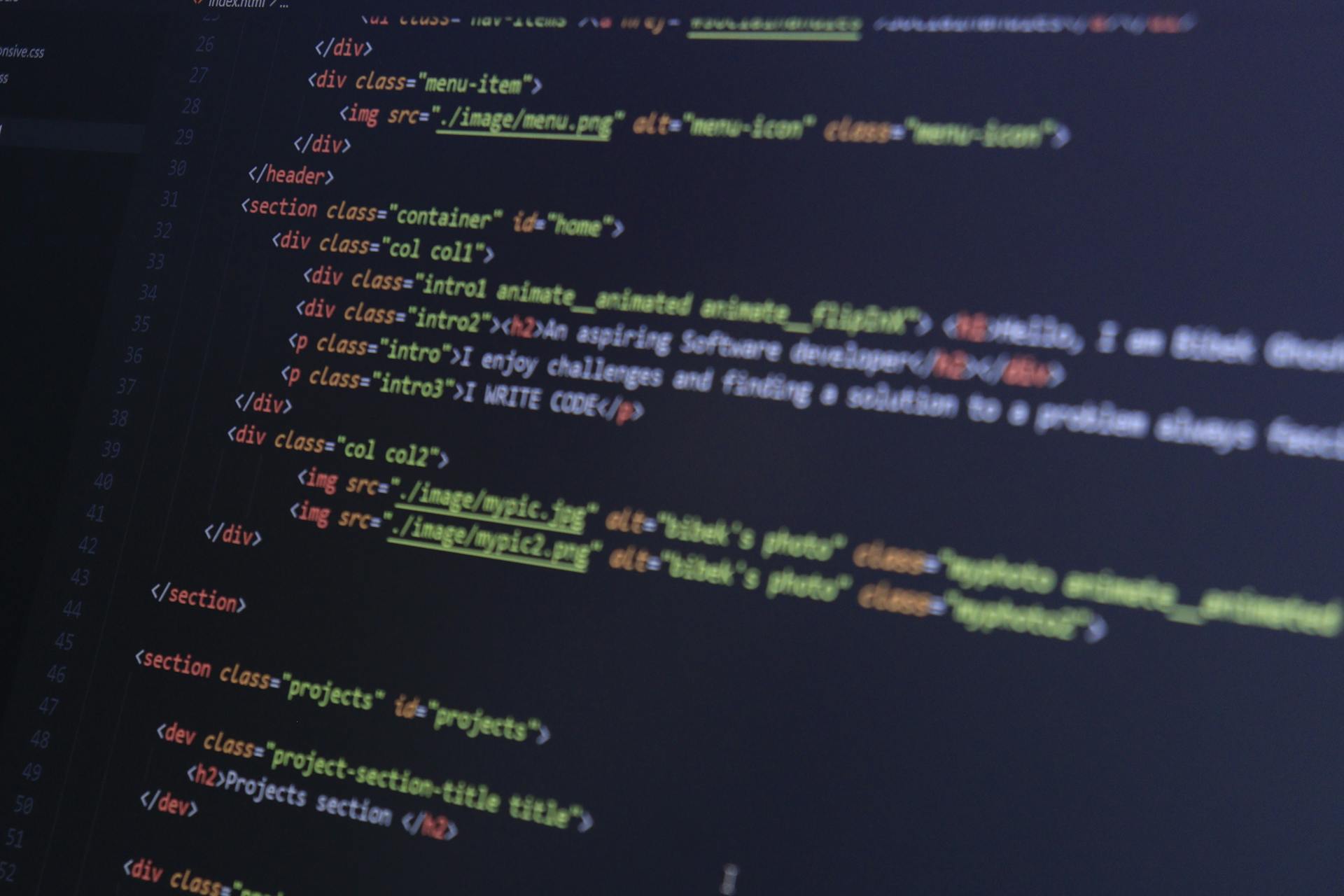
Adding images to your Next.js project is a crucial step in making it visually appealing and engaging. To start, you need to create an image component that can handle different image formats and sizes.
You can use the Image component provided by Next.js, which automatically optimizes images for web use. This component is a great starting point for adding images to your project.
To optimize images for web use, you can use techniques like compressing images using tools like ImageOptim or ShortPixel. By compressing images, you can reduce their file size without sacrificing quality.
Remember to use the right image format for the job. For example, use JPEG for photos and PNG for graphics and logos.
For your interest: Nextjs Use Server
Optimization Techniques
The Next.js Image component automatically optimizes images on-demand as they are requested by users, serving them in the most efficient format based on the user's device and browser capabilities.
To further optimize your images, consider using a CDN like Amazon Cloud front or Google CDN to host your images, especially if you have a large amount of dynamic images.
Before serving your images, choose the appropriate image format, optimize the size, and compress the quality using appropriate dimensions.
Here are some key points to consider when optimizing your images:
- Choosing the right image format
- Optimizing image size
- Compressing image quality
- Using image compression tools
By following these optimization techniques, you can ensure that your images are delivered to users in the shortest possible time, improving the overall performance of your Next.js app.
Built-in Image Optimization
The Next.js Image component automatically optimizes images on-demand, serving them in the most efficient format based on the user's device and browser capabilities.
Lazy loading is also enabled by default, meaning images are only loaded when they enter the viewport, significantly improving loading times.
To ensure responsive images, set the layout attribute to responsive, and the Image component will adapt to different screen sizes.
The component also supports placeholders, displaying a placeholder until the image is fully loaded, improving the perceived performance of your Next.js app.
Automatic image optimization solves image size, web formats, and responsiveness issues, making it a comprehensive solution for image optimization.
Here's an interesting read: Nextjs App Router Loading
The Next.js built-in image optimization API, next/image, provides a canonical form for native automatic image optimization.
When adding the width and height of an image, ensure to add the correct dimension in pixels, as incorrect dimensions can result in a skewed image.
Here are the benefits of using the Next.js Image component for optimization:
- Automatic Image Optimization
- Lazy Loading
- Responsive Images
- Placeholder Support
Choosing the Right Image Format
Choosing the right image format is crucial for optimizing your website's performance. The three most popular image formats on the web are JPEG, PNG, WebP, and Avif.
WebP and Avif are highly recommended due to their many advantages and performance benefits. WebP provides superior lossy and lossless image compression for web images without compromising quality.
Avif, on the other hand, provides better compression and faster loading times compared to WebP after the first load. Using a tool like WebP-Converter/Avif converter can help you convert your images to WebP or Avif format, which is supported by most modern browsers.
A unique perspective: Nextjs Performance
The Image Optimization API in NextJs supports multiple formats, and you can specify the formats that are supported by this API. The default value is "image/webp", but you can also enable AVIF support by adding "image/avif" to the list of formats.
Here are the supported formats in NextJs, in the order they are used:
- image/webp
- image/avif
If there is no match or the source image is animated, the Image Optimization API falls back to the original image's format. Keep in mind that AVIF generally takes 20% longer to encode compared to WebP, but it compresses 20% smaller.
Suggestion: Image Component Nextjs
Device-Specific Image Sizes
Serving the right images for the right device sizes is crucial for optimizing image performance on the web. This is because serving a huge image for users with small device sizes can lead to unnecessary bandwidth usage, slowing down page loads and affecting performance metrics.
Consider using a CDN like Amazon Cloud front, Google CDN, or Cloudinary to host your images, especially if you have a large amount of dynamic images. This can help reduce the load on your server and improve page loads.
To optimize image performance, choose the appropriate image format, optimize the size, and compress the quality using appropriate dimensions. Using image compression tools can also help reduce the file size of your images.
To provide different images according to device sizes, use the "source" elements to provide different image sources for different screen sizes. For example, you can use the first "source" element if the screen width is less than or equal to 500px, which will provide a smaller image.
The Responsive Breakpoints Generator tool by various CDN providers is a good tool for generating multiple image file sizes for different screen sizes. This tool also provides options for serving images in different formats, including WebP and AVIF, which can further improve performance.
Here's an example of how to use the "next/image" component to optimize images for different screen sizes and formats:
By using device-specific image sizes, you can reduce the amount of unnecessary bandwidth usage and improve performance metrics.
Using Next.js for Image Optimization
The Next.js Image component automatically optimizes images on-demand, serving them in the most efficient format based on the user's device and browser capabilities.
Lazy loading is enabled by default, meaning images are only loaded when they enter the viewport, significantly improving loading times.
To provide an optimal experience across devices, the Image component ensures images adapt to different screen sizes by setting the layout attribute to responsive.
A placeholder can be displayed until the image is fully loaded, improving the perceived performance of your Next.js app.
The golden rule for performance optimization is giving users what they want in the shortest possible time, and Next.js provides a built-in image optimization API to achieve this.
Automatic image optimization solves image size, web formats, and responsiveness issues with a single usage.
The width and height of the image should be in pixels, and when adding dimensions, ensure they are correct to avoid skewed images.
Expand your knowledge: Next Js Tailwind Spinner Loading Page
Serving the right images for the right device sizes is crucial for optimizing image performance on the web.
To achieve this, you can use the Responsive Breakpoints Generator tool to generate multiple image file sizes for different screen sizes.
The three most popular image formats on the web are JPEG, PNG, Avif, and WebP, with WebP and Avif recommended for their many advantages and performance benefits.
WebP provides superior lossy and lossless image compression for web images without compromising quality, while Avif provides better compression and faster loading times compared to WebP after the first load.
To convert your images to WebP or Avif format, you can use a tool like WebP-Converter/Avif converter, which is supported by most modern browsers.
The formats supported by Next.js include WebP, Avif, and JPEG, with the order of formats in the array mattering if the Accept header matches more than one format.
AVIF generally takes 20% longer to encode compared to WebP but compresses 20% smaller, making it a good choice for optimizing image performance.
To make a single image responsive for all viewports, you can use the sizes attribute provided by Next.js, which automatically generates custom srcsets according to the defined sizes.
Additional reading: Next Js Use Client
Serving External Images
Fetching and rendering images from a server or CMS in Next.js isn't as straightforward as passing the URL of the referenced image into the src property.
You need to specify which domains are allowed to be optimized in your project, this is done by adding an image object with a domain to the next.config.js module.
Add the following code to your next.config.js module: module.exports={ Images:{ domains:['example.com'], }, }
Sources
- https://www.dhiwise.com/post/how-to-add-a-background-image-in-nextjs-a-comprehensive-guide
- https://webkul.com/blog/nextjs-image-component/
- https://blog.abdulqudus.com/image-optimization-with-nextjs
- https://dev.to/david4473/working-with-images-in-next-js-48d6
- https://rhnmht30.dev/blog/next-image-with-netlify
Featured Images: pexels.com