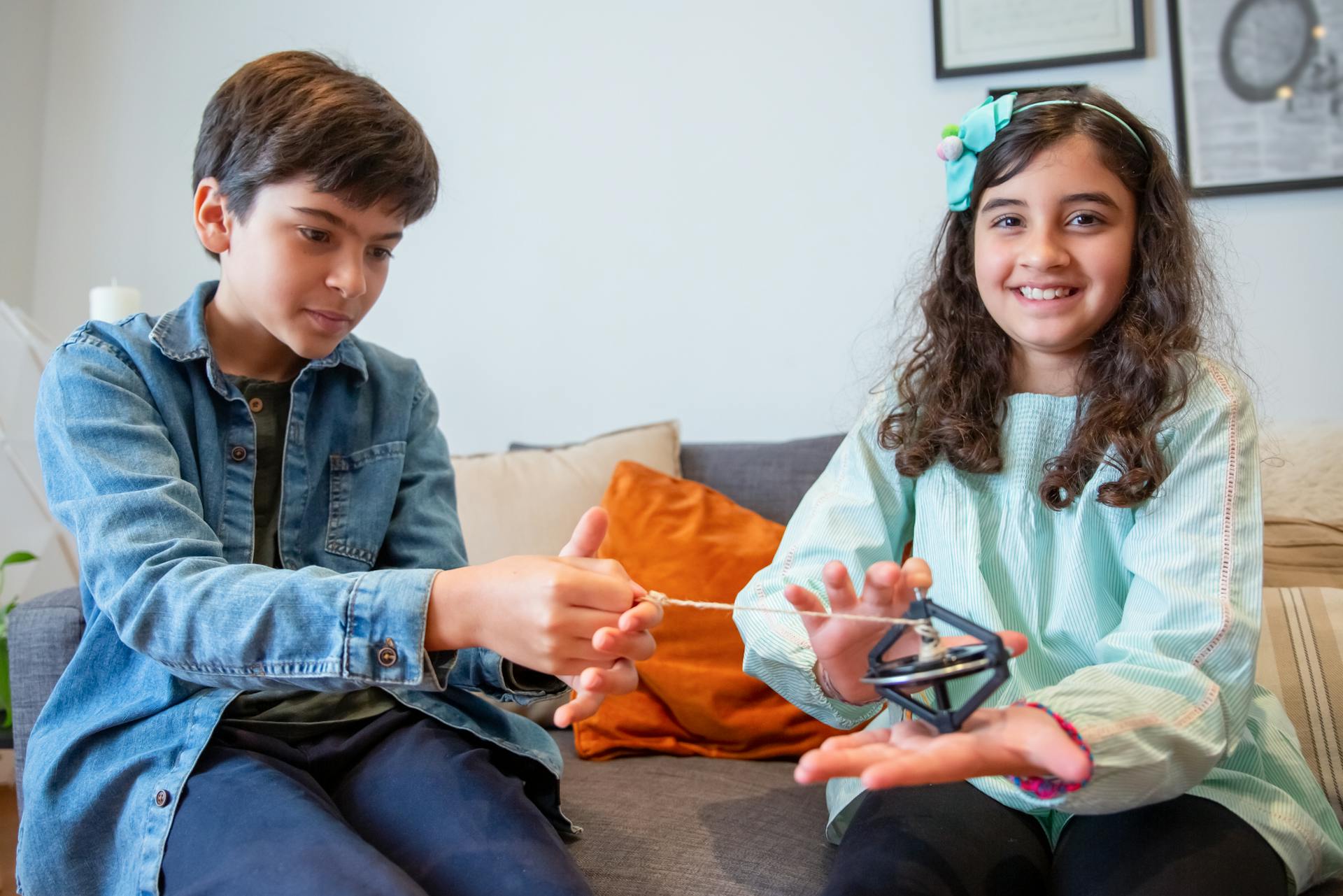
Creating a loading page with Next.js and Tailwind CSS is a great way to enhance the user experience by providing a clear indication of what's happening behind the scenes. This approach helps to prevent frustration and improves engagement.
To get started, you'll need to set up a new Next.js project using the command `npx create-next-app my-app --experimental-app`. This will create a basic Next.js project with the necessary configuration files.
Tailwind CSS is a utility-first CSS framework that allows you to write highly customizable, responsive UI components using a concise syntax. By integrating Tailwind CSS with Next.js, you can create a seamless and visually appealing loading page.
In the next section, we'll dive into the specifics of creating a loading page with Next.js and Tailwind CSS, including how to use the `useState` hook to manage the loading state and how to style the loading animation using Tailwind CSS classes.
Consider reading: Tailwind Classes Not Working Next Js
Getting Started
To get started with creating a loading spinner in Next.js using Tailwind CSS, you'll want to configure your Next.js project with Tailwind. This will allow you to use Tailwind's utility classes to style your spinner.
Curious to learn more? Check out: Nextjs App Router Tailwind Loading Page
First, create a new file called Spinner.js in your components folder. This is where you'll write the code for your spinner. In this file, you'll use Tailwind's utility classes to style the spinner.
To create the basic spinner, you'll need to use the following utility classes: `animate-spin`, `rounded-full`, `h-32`, `w-32`, `border-t-2`, `border-b-2`, and `border-blue-500`. These classes will rotate the div, create a circular effect, and set the size and color of the spinner.
For another approach, see: File Upload Next Js Supabase
Installing
Installing Tailwind CSS is a breeze. You can install it by running the command `npm install -D tailwindcss postcss autoprefixer` in your terminal.
Here are the steps to follow:
- Run `npx tailwindcss init -p` to set up your Tailwind configuration.
- Update your `tailwind.config.js` file to add the paths where Tailwind will be used.
- Create a `globals.css` file inside the styles directory and add the following Tailwind imports: `@tailwind base; @tailwind components; @tailwind utilities;`
- Ensure your project imports `globals.css` in `_app.js` like this: `import "../styles/globals.css";`
By following these steps, you'll be able to style your spinner efficiently with Tailwind's utility classes.
Creating a Basic
Creating a Basic Loading Spinner with Tailwind CSS is a great way to get started with customizing your UI.
You can create a basic loading spinner by following these steps: create a new file called Spinner.js in your components folder.
Check this out: Create Next Js App
The spinner will use simple Tailwind utility classes to make it highly customizable.
To style the spinner, use Tailwind's utility classes, such as the animate-spin class, which rotates the div.
You can also use the border classes to create a circular effect, like border-t-2, border-b-2, and border-blue-500.
The size, speed, and color of the spinner can all be easily modified using Tailwind's utility-first approach.
Here are some key classes to get you started:
- animate-spin: rotates the div
- border-t-2, border-b-2, border-blue-500: creates a circular effect
- h-32, w-32: sets the size of the spinner
Customizing the Appearance
Customizing the spinner's appearance is a breeze with Tailwind CSS. You can change the size, colors, and even add text to make it fit seamlessly into your application's design.
The spinner size can be easily modified using Tailwind's utility classes, such as changing the height and width. For example, you can make the spinner smaller by setting the height and width to 16px.
Tailwind's color system makes it simple to set custom colors for the spinner. You can choose from a wide range of colors to match your application's aesthetic. For instance, you can set the border color to blue-500 to give it a distinctive look.
Suggestion: Nextjs Server Rendering Tailwind
Adding text next to or below the spinner provides additional feedback to the user. You can use Tailwind's text utility classes to style the text, such as setting the text color to gray-500 and adding a margin top of 2px.
Here are some customization options for the spinner:
By customizing the spinner's appearance, you can create a more cohesive user experience that aligns with your application's design.
Animations and Transitions
Animations and Transitions can greatly enhance the user experience on your Next.js Tailwind spinner loading page. Tailwind CSS provides excellent utility classes for transitions and animations that can make the loading experience feel polished.
You can add smooth transitions and animations to your spinner by using Tailwind's animation utilities. For example, you can add the animate-spin class to your spinner to create a smooth, continuous rotation effect.
The bounce utility class is another helpful animation utility that comes out-of-the-box with Tailwind CSS. It can be used to create a skeleton loader animation when the page loads or to get the attention of the user if there is an update available.
Check this out: Is Next Js Backend
Tailwind also provides the pulse class, which provides a smooth fade in and fade out animation on the element. This can be useful in situations such as loading states or creating a skeleton loader animation.
Here are the primary Tailwind animation utility classes:
The spin utility class rotates an element with a linear animation, making it ideal for crafting loading spinners that visually communicate to users that an action is currently being processed.
Best Practices and Implementation
Implementing a spinner in your Next.js application with Tailwind CSS can be a great way to enhance the user experience during loading times. However, it's essential to follow some best practices to ensure it doesn't detract from the user experience.
Use spinners sparingly, as they should only be visible when necessary. This will prevent overwhelming users with too much visual feedback.
Limit the duration of the spinner to avoid frustration. If the process takes too long, consider offering users an alternative or explanation instead of just a spinner.
The size and colors of the spinner are also crucial. Ensure it's visible but not overpowering, so it doesn't compete with other elements on the page.
Combine spinners with text-based feedback, such as "Loading your data...", to reassure users that something is happening. This will help build trust and keep users engaged.
Here are the best practices summarized in a list:
- Use spinners sparingly
- Limit spinner duration
- Use appropriate size and colors
- Provide feedback
By following these best practices, you can create a responsive and lightweight loading page that adds polish to your Next.js application.
Displaying the Loader
You can display a skeleton loader on the article page to show a loading effect before the article is fetched from the API. This can be achieved by adding the SkeletonCard component to the article page and using the useState hook to set the loading state to true initially.
To simulate a longer loading time, you can use the setTimeout function to delay setting the loading state to false for 3 seconds. This will make the skeleton loading effect appear for a longer time.
You might like: Nextjs State Management
You can also create a full-page loader that covers the entire screen until the main content has fully loaded. This is particularly useful for scenarios where the entire application depends on certain data, and you want to ensure a smooth transition once the content is ready.
Here are the steps to create a full-page loader:
- Wrap your content in a div that conditionally shows the loader.
- Add the fixed class to ensure the loader stays on top of the content, covering the entire screen.
You can also display a spinning or loading animation within a button to indicate that an action is in progress. This is useful for providing visual feedback to the user while the button is being processed.
For more insights, see: Nextjs Button
Displaying a Loader in a Button
Displaying a loader in a button is a great way to show that an action is in progress. This is typically done with a spinning or loading animation within the button.
You can use Tailwind CSS to make a loading spinner element, as shown in a tutorial video that's available to watch.
Showing a loader in a button is useful for indicating that something is happening, like when a user submits a form or clicks a link.
It's a simple way to keep users informed and engaged, and it can be especially helpful for tasks that take a few seconds to complete.
Take a look at this: Next Js Page Transition Animation Loader
Full Page Loader
A full-page loader can be a lifesaver when dealing with slow-loading content. This technique involves wrapping your content in a div that conditionally shows the loader.
You can achieve this by using a div with a fixed class to ensure it stays on top of the content, covering the entire screen. The z-50 class ensures it has a higher stacking order than other elements.
To create a full-page loader, you'll need to add a Spinner component inside the div. This will display the loading animation to the user.
Here are the steps to create a full-page loader:
- Wrap your content in a div that conditionally shows the loader.
- Add the fixed class to ensure the loader stays on top of the content.
The full-page loader approach is particularly useful for scenarios where the entire application depends on certain data, and you want to ensure a smooth transition once the content is ready. This is especially true when dealing with huge or heavy content articles that take a while to load.
Sources
- https://www.cloudzilla.ai/dev-education/skeleton-loading-in-nextjs-with-tailwindcss/
- https://prateekshawebdesign.com/blog/creating-a-tailwind-spinner-loading-page-in-nextjs
- https://www.braydoncoyer.dev/blog/how-to-create-an-animated-loading-spinner-with-tailwind-css
- https://headlessui.com/react/transition
- https://blogs.purecode.ai/blogs/tailwind-spinner/
Featured Images: pexels.com