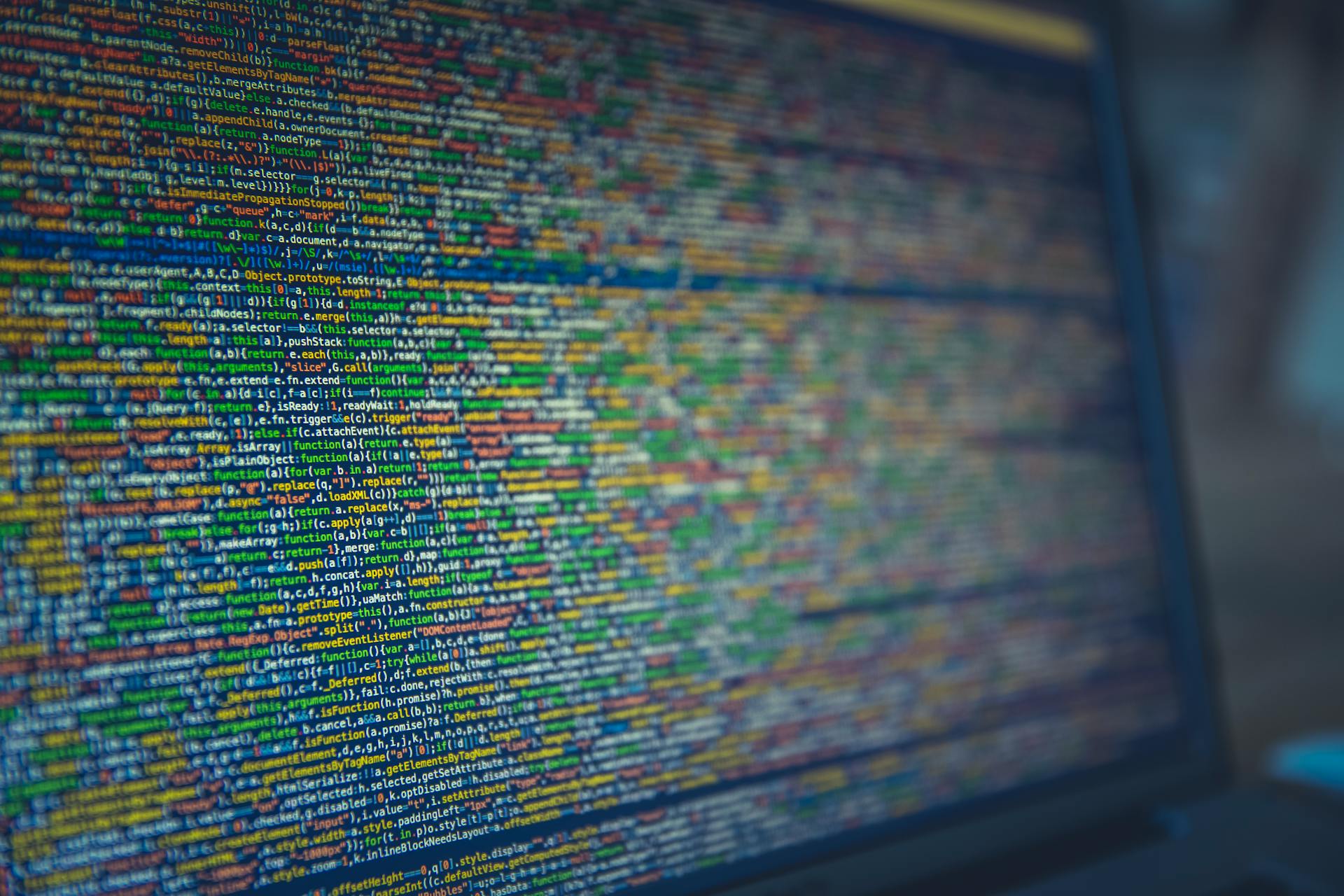
Adding a backend to Next.js is a crucial step in building a robust and scalable application. Next.js provides a straightforward way to integrate a backend with its built-in API routes.
To get started, you can create API routes using the `pages/api` directory, which allows you to define server-side routes that handle HTTP requests. This is a powerful feature that enables you to create custom APIs without needing to set up a separate backend server.
Next.js also supports serverless functions, which can be used to create scalable and cost-effective backend logic. By using serverless functions, you can offload compute-intensive tasks to the cloud, reducing the load on your application.
To integrate a backend with Next.js, you'll need to set up a database to store and retrieve data. This can be done using a variety of databases, including MongoDB, PostgreSQL, and SQLite.
On a similar theme: Nextjs Usecontext
Getting Started with Parse
Using Parse Server together with Next.js is a powerful combination that allows you to focus on critical points of your product and achieve maximum productivity.
To get started, you'll need to install the Parse Javascript SDK to your application. Open your terminal in your project's folder and type the following command to install it.
After that, create a new folder in your project, called services, and inside this folder, create a file called parse.js. This file will hold the Parse configuration and initialization code.
For another approach, see: Next Js Install
Bootstrapping a Parse Application
To bootstrapping a Parse application, you need to make sure Node.js is installed on your computer.
You can create the project by typing a command in your terminal. The project structure is pretty straightforward, with most folders and files being self-explanatory.
The pages folder is responsible for application routing, so every file created inside this folder creates a new route in your app.
To get started with Parse, you'll need to install the Parse Javascript SDK to your application. This can be done by typing a command in your terminal.
For more insights, see: Next Js Spa
Next, you'll need to create a new folder called services and a file called parse.js inside it.
Make sure to use the correct syntax, such as parse instead of parse/node, to avoid errors.
You can retrieve the application id and javascript key by creating an app at Back4App and retrieving its keys.
Once you have your keys, you can use them inside your application by editing the next.config.js file.
Finally, replace the placeholders in the services/parse.js file with the key's path and export Parse from this file.
You might like: Nextjs Server Actions File Upload
Overview
Getting Started with Parse requires a solid understanding of Next.js. Next.js is a robust React framework that optimizes server-side rendering (SSR) and client-side rendering (CSR) capabilities.
Delivering flawless user experience and structured data retrieval is crucial in web development, and Next.js is the go-to choice for developers seeking to achieve this. Its comprehensive toolkit for data fetching and server actions makes it an ideal choice.
To harness the power of Next.js, you need to understand its fundamentals, including data fetching. In fact, understanding the basics of Next.js is essential to building custom server endpoints that handle specific actions and data operations.
Explore further: Nextjs Server Rendering Tailwind
Server actions are an alpha feature in Next.js that enable you to build custom server endpoints. Next.js API routes offer a robust foundation to implement server actions effectively, bridging the gap between the client and server seamlessly.
Here are the key aspects of Next.js that you need to know:
- Server actions are an alpha feature in Next.js.
- Next.js API routes offer a robust foundation to implement server actions effectively.
- Next.js is a robust React framework that optimizes SSR and CSR capabilities.
By mastering these aspects of Next.js, you'll be well on your way to building robust web applications that deliver seamless user experiences.
Prisma and Next Integration
Prisma is the perfect companion for your Next.js app if you need to work with a database. It supports pre-rendering pages at build time or request time, allowing you to decide where to access your database.
You can use Prisma inside Next.js API routes, getServerSideProps, or getStaticProps for full rendering flexibility and top performance. Next.js will pass the props to your React components, enabling static rendering of your page with dynamic data.
Prisma Accelerate can speed up your database queries, especially in Serverless or Edge environments. Its scalable connection pool ensures that your database doesn't run out of connections, even during traffic spikes.
Worth a look: Next Js Client Side Rendering
Next.js and Prisma are a powerful combo for React apps, offering a full stack development experience with end-to-end type safety. You can query your database with Prisma in Next.js API routes, getServerSideProps, or getStaticProps.
t3 is a web development stack that includes Next.js, Prisma, and other tools for building robust web applications. Blitz.js is another framework built on top of Next.js and Prisma, offering simplicity and conventions similar to Ruby on Rails.
CoDox is a starter template for modern web development that includes Next.js 13, Prisma, and other tools to save you time on boilerplate setup. A comprehensive tutorial teaches you how to build a fullstack form application using Prisma and Next.js.
If this caught your attention, see: Next Js Stack
API Routing and Security
To create an API route in Next.js, you need to create a file inside the pages/api directory. This file should be named based on the desired endpoint, for example, pages/api/users.js. Within this file, you can define your server action logic.
Curious to learn more? Check out: Next Js Project Structure
API routes in Next.js make it seamless to communicate with the client-side code. You can use the fetch API or any other HTTP library to make requests to your API endpoints.
Next.js automatically routes requests to the appropriate API route, so you can use relative URLs like /api/users when making requests to API routes from the client-side code.
To protect API endpoints from unauthorized access, you need to ensure that API routes are kept safe. One way to do this is to use authentication and authorization mechanisms, such as those discussed in the article.
Server actions in Next.js are an integral part of web applications, allowing you to handle server-side logic and perform operations such as data manipulation, authentication, and more.
Expand your knowledge: Next Js Use Client
Client-Side Communication with API
Next.js makes it seamless to communicate with API routes from the client-side code, allowing you to fetch data, perform data mutations, or handle authentication.
You can use the fetch API or any other HTTP library to make requests to your API endpoints. For example, you can use the fetch API to fetch users from the /api/users endpoint, as shown in the code snippet above.
Curious to learn more? Check out: Nextjs Code Block
To make requests to API routes from the client-side code, you can use relative URLs like /api/users since Next.js automatically routes requests to the appropriate API route.
API routes provide a powerful mechanism to handle server actions seamlessly within your application. Whether you need to fetch data, perform data mutations, or handle authentication, API routes enable you to define custom server endpoints with ease.
Here are some key points to keep in mind when using API routes for client-side communication:
- Use relative URLs like /api/users to make requests to API routes.
- Use the fetch API or any other HTTP library to make requests to API endpoints.
- Use the useEffect hook with an empty dependency array [] to ensure data fetching only occurs once when the component mounts.
Frequently Asked Questions
Should you have a separate backend for NextJS?
For simpler projects, Next.js API routes might be enough. However, for complex applications or future expansions, a separate backend offers more flexibility and scalability.
Featured Images: pexels.com