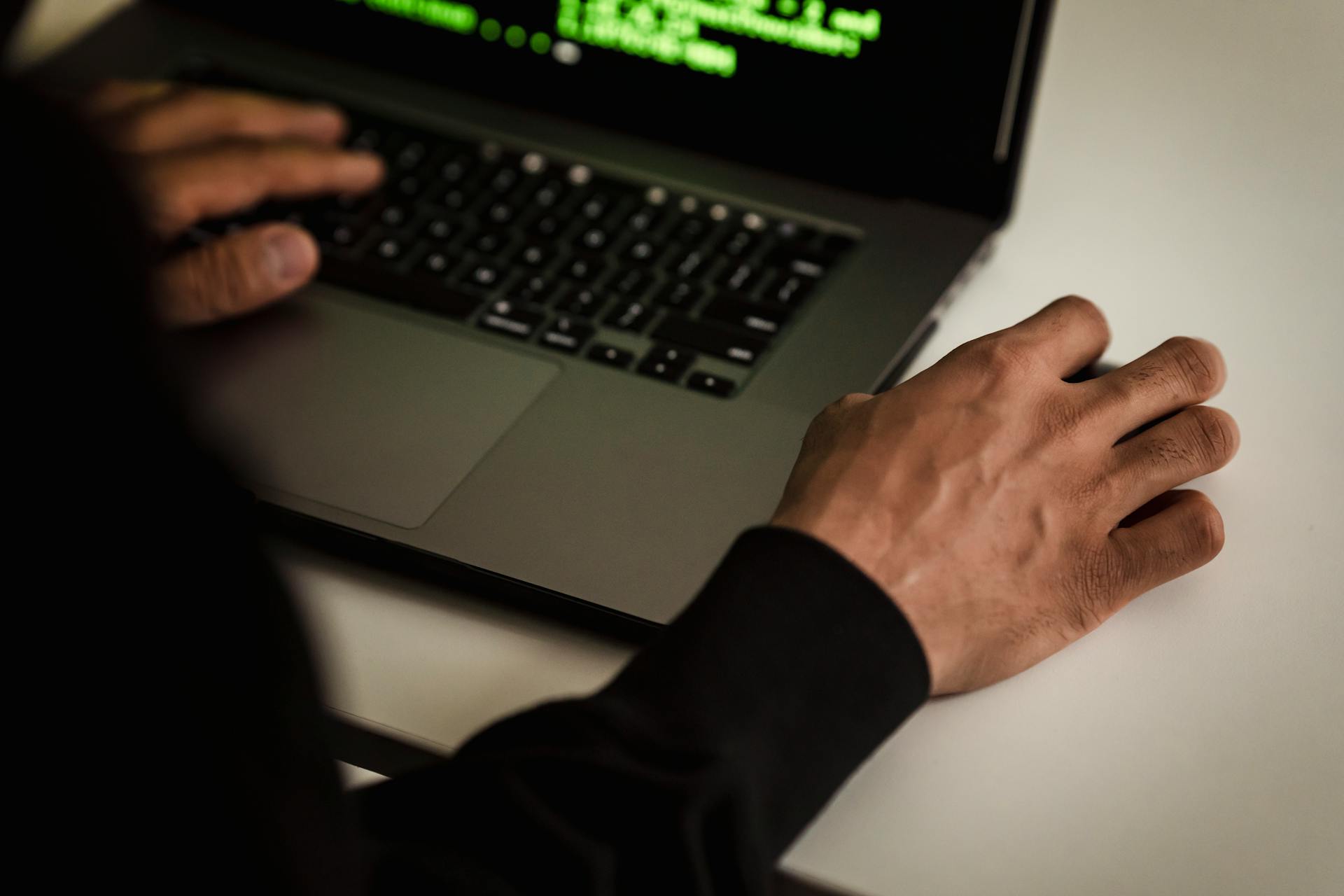
Next.js is an excellent choice for full-stack web development, and mastering its stack can significantly boost your productivity. Next.js uses a server-rendered architecture, which means that the server generates the initial HTML of the page, improving SEO and user experience.
The Next.js stack includes Next.js itself, along with other tools like TypeScript, React, and Redux. This combination provides a robust foundation for building scalable and maintainable web applications.
By using Next.js, you can take advantage of its built-in features, such as automatic code splitting and static site generation. This allows you to create fast and efficient web applications that can handle large amounts of traffic.
Setting Up Next.js
To set up Next.js, you need to have Node.js and npm/yarn installed on your computer. You can install them from their official websites: Node.js and npm.
If you don't have Node.js and npm installed, you're in luck because npm is included when you install Node. This means you only need to install Node.js once.
For more insights, see: Install Nextjs
To create a new Next.js project, launch your terminal and run the following command: `npx create-next-app@latest --experimental-app`. This will create a new directory with the necessary files.
Enter your project name and click enter, then wait for it to install. A new directory with your project name will be created.
Once you're in the new directory, you can start the development server by running the following command: `yarn run dev` (if you're using yarn) or `npm run dev` (if you're using npm).
You can see your Next.js app running on http://localhost:3000.
Check this out: Create Cookies Nextjs
Authentication and Authorization
In Next.js, you can create new pages in the app directory, each with a page.js file. This is where you'll add the logic for your sign up and sign in pages.
To create the sign up page, you'll add a new signup > page.js file in your app directory and tell Next.js that you want a Client component by adding the following code at the top of the file. This will allow you to add client-side interactivity like adding an onSubmit() to a form element.
For your interest: Next Js Backend
The sign up page will have a function like signUp() that uses the Firebase createUserWithEmailAndPassword() method to sign up new users. This function can be used anywhere in your app.
In the same manner, you can create your login page by adding a new signin > page.js file in your app directory. If the user is null, you'll redirect them to the homepage, and if the user is not null, you'll show them the protected page.
To set up authentication, you'll create a new directory called auth in your src > firebase directory. In this directory, you'll add your Firebase authentication related code, including the signUp() and signIn() functions. The signUp() function will use the Firebase createUserWithEmailAndPassword() method to sign up new users.
Recommended read: New Nextjs Project Typescript
Next.js Concepts
Next.js is a full-stack open-source React framework created by Vercel in 2016. It has grown to become one of the top choices for building websites and applications.
Next.js has gained widespread adoption from the web development community and is used by everyone from small businesses and startups to large corporations and Fortune 500 companies like Stripe, The Washington Post, Nike, OpenAI, and Netflix.
Next.js is a React meta-framework that builds on top of React and extends its functionalities to offer a more comprehensive development experience. It inherits some of its core properties from React, like the virtual DOM, component-based architecture, and the use of JSX for defining UI elements.
Suggestion: Next Js React Fundamentals
What Is?
Next.js is a full-stack open-source React framework created by Vercel in 2016. It provides the tools needed to handle the front-end and back-end aspects of building web applications.
Next.js is built on top of React and extends its functionalities to offer a more comprehensive development experience. It inherits some of its core properties from React, like the virtual DOM, component-based architecture, and the use of JSX for defining UI elements.
For more insights, see: React + Next Js
Next.js supports multiple rendering strategies, including Server-side rendering (SSR), Client-side rendering (CSR), Static site generation (SSG), and Incremental static regeneration (ISR). These strategies allow you to choose the most suitable rendering method for different parts of your application.
Here are the primary rendering strategies Next.js supports:
- Server-side rendering (SSR): Generates HTML files on each request, allowing for dynamic content generation.
- Client-side rendering (CSR): Generates HTML on the client side after the initial page load.
- Static site generation (SSG): Generates HTML files at build time to create static pages that can be served quickly to users.
- Incremental static regeneration (ISR): Combines SSG and SSR by allowing static pages to be regenerated in the background as new requests come in.
Next.js blurs the lines between client and server, allowing you to decide whether to access your database with Prisma at build time (getStaticProps), at request time (getServerSideProps), using API routes, or by entirely separating the backend out into a standalone server.
Custom Link Component
The custom Link component in Next.js is a game-changer for client-side navigation between pages.
Unlike traditional HTML anchor tags, which cause a full page reload, Next.js Link component leverages client-side routing and JavaScript-based navigation to avoid full-page refreshes.
This means that when a user clicks on a link, Next.js intercepts the click event and uses the browser's History API to update the URL without refreshing the page.
If this caught your attention, see: Next Js Use Client
The Link component offers additional features like prefetching, which preloads the data needed to display a page in the background before a user clicks on a link.
This ensures that the page loads almost instantly when the user clicks on the link, resulting in faster page transitions and a smoother browsing experience for users.
Component Lazy Loading
Component lazy loading is a powerful feature in Next.js that allows you to display components as needed, rather than loading all of them simultaneously.
This on-demand strategy can significantly improve the initial page load time and perceived performance by reducing the amount of code that needs to be executed. By loading components only when they're actually needed, you can create a snappier user experience.
Next.js uses component lazy loading to optimize the rendering of components. This means you can focus on building your application without worrying about the performance implications of loading multiple components at once.
A unique perspective: Nextjs App Router Loading
The benefits of component lazy loading are numerous, including faster page load times and improved user experience.
Here are the different strategies for loading components in Next.js:
- beforeInteractive: loads scripts before any Next.js code and page hydration occurs
- afterInteractive: loads scripts after the initial critical page content is rendered
- lazyOnload: loads scripts after all other resources on the page have been fetched
These strategies can be applied to component lazy loading as well, allowing you to control when and how components are loaded.
Implementation and Deployment
Implementation and deployment of a Next.js project is a straightforward process. You can use the `getStaticProps` method to tell Next.js that this is a Static Site Generation (SSG) page and should fetch the data at build time.
To start, you'll need to adjust the build command in your `package.json` file to `next build && next export`. This tells the Next.js CLI to output all pages as static sites and place them in the `out/` folder.
You can then build your SSG project via `npm run build`, and Next.js will show you which types it's using in the build process.
Here are the key types used in the build process:
If you want to test your result, you can start a local HTTP server using `http-server` and access the URL `http://127.0.0.1:8080/ssg`. This will show you the finished HTML with all the content of the rest of the endpoint.
Setting Up Firebase
To set up Firebase, start by going to the Firebase console and logging in with your Google account. You'll need to create a new project and give it a name, and then you can choose whether or not to enable analytics for your project.
To create a web app, click on the web icon on your project homepage and give your app a name. You'll also need to copy the configuration file, which you'll use later.
Once you've set up your project, you can add products to your app, such as Authentication and Cloud Firestore. For the sake of this tutorial, let's add only Authentication and Cloud Firestore.
Here's a list of the Firebase configuration files you'll need to set up:
To use Firebase with your Next.js app, you'll need to install the latest Firebase SDK by running the command `yarn add firebase` or `npm install firebase` in your terminal.
A fresh viewpoint: Install Next Js 13
Implementation
To implement a Static Site Generation (SSG) approach with Next.js, you need to use the `getStaticProps` method, which fetches data at build time. This method is called at build time, and it's used to tell Next.js that this is an SSG page.
A fresh viewpoint: Nextjs Ssg App Router
You'll also need to adjust the build command in your `package.json` file to `next build && next export`. The `next export` command tells the Next.js CLI to output all pages as Static Sites and place them in the `out/` folder.
The build process will show you which types of pages Next.js is generating, including SSG pages. To test your result, you'll need to start a local HTTP server after the build & export, using a command like `http-server -p 8080 -c-1 out/ -o`.
Here are some key points to keep in mind when implementing SSG with Next.js:
- All pages of the project must be generated in the SSG approach at build time.
- This approach is great for projects where the content doesn't change often, such as a blog.
- You can use the Hydration process to load a frontend framework at runtime and only load individual data.
For example, if you're building a product detail page that's assembled using an ID parameter, you'll need to use the SSG approach to generate the page at build time.
Deploy Docker
Deploying a NextJS application via Docker is a straightforward process. You can find the Dockerfile for this example on the branch ssr.
To build the Docker image, use the command `docker build . -t my-next-js-app:1.0.0`. This command tells Docker to build an image with the tag `my-next-js-app:1.0.0` from the current directory.
The Dockerfile is inspired by the one found at https://github.com/vercel/next.js/blob/canary/examples/with-docker/Dockerfile.
Once the image is built, you can start it with `docker run -p 3000:3000 my-next-js-app:1.0.0`. This command maps port 3000 on the host machine to port 3000 in the container.
Your application can then be accessed at `localhost:3000`.
See what others are reading: Next Js Single Page Application
Static Site Generation with Prisma
Static Site Generation (SSG) is a technique used in Next.js to pre-render pages at build time, making it ideal for static pages like blogs and marketing sites.
To use Prisma with SSG, you can use the getStaticProps function in Next.js, which is executed at build time. This function allows you to send queries to your database using Prisma.
Next.js will pass the props to your React components, enabling static rendering of your page with dynamic data.
Here are some key benefits of using Prisma with SSG:
- Improved performance: With Prisma, you can query your database at build time, reducing the load on your database at runtime.
- Flexibility: You can use Prisma in API routes, getServerSideProps, or getStaticProps for full rendering flexibility.
- Top performance: Prisma's connection pooler and global cache make your database queries faster, especially in Serverless and Edge environments.
To get started with Prisma and SSG, you can use the getStaticProps function in Next.js to send queries to your database. Next.js will pass the props to your React components, enabling static rendering of your page with dynamic data.
Prisma-powered Next.js projects can be deployed on Vercal, a platform built for Next.js apps, making it easy to deploy your project.
By using Prisma with SSG, you can create fast, flexible, and scalable web applications that meet the needs of your users.
Check this out: Nextjs Prisma Docker
Frequently Asked Questions
Is NextJS front-end or full-stack?
NextJS is a full-stack framework, meaning it handles both front-end and back-end development, making it a versatile choice for building complex applications. Its full-stack capabilities set it apart from traditional front-end frameworks like React.
Is NextJS a mern stack?
NextJS is not a part of the traditional MERN stack, but can be used in conjunction with it to build scalable server-side rendered applications. It's often used to enhance the MERN stack, particularly for server-side rendering and static site generation.
Is NextJS better than node?
Next.js offers built-in server-side rendering and static site generation capabilities, setting it apart from Node.js, which requires additional frameworks like Express for similar functionality. This built-in support makes Next.js a more streamlined choice for certain web development needs.
Does Next.js have a backend?
Yes, Next.js has a robust backend capability, allowing you to build server-side logic and dynamic user interfaces with ease. Its powerful backend features make it a versatile tool for modern web development.
Featured Images: pexels.com