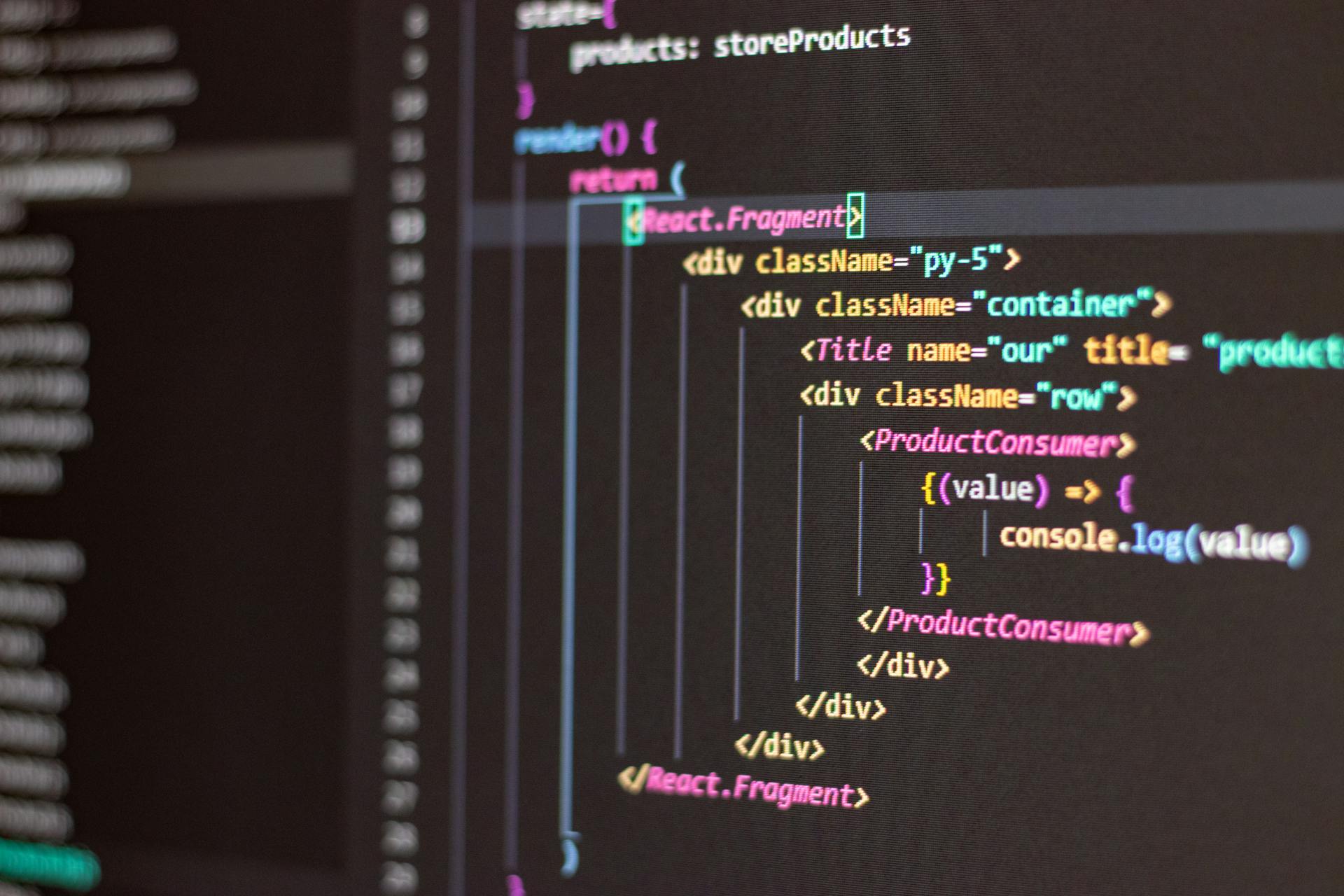
Next.js is a popular React framework that allows you to build server-side rendered (SSR) and statically generated websites and applications.
It's built on top of React and provides a lot of features out of the box, such as automatic code splitting and optimization, support for internationalization, and more.
One of the key benefits of Next.js is its ability to pre-render pages at build time, which can improve performance and SEO.
This is achieved through Next.js's built-in support for static site generation (SSG), which allows you to generate static HTML files for your pages.
Next.js Fundamentals
Next.js is a popular React-based framework for building server-rendered, statically generated, and performance-optimized applications.
To get started with Next.js, you'll want to learn about its introduction, which covers the basics of the framework. This is a great place to begin your journey with Next.js.
Next.js can be installed and set up with Tailwind CSS, a utility-first CSS framework, to give your application a sleek and modern look. You can follow the steps outlined in the "Install & Setup Tailwind CSS with NextJS" section to achieve this.
To understand the differences between Next.js and React, check out the "Next VS React" section. This will give you a clear idea of what sets Next.js apart from the popular React library.
To import images in your Next.js application, use the `Image` component, which can be found in the "How to import image in NextJS?" section. This is a straightforward process that requires minimal setup.
Here are some key differences between Next.js and React:
- Server-side rendering
- Static site generation
- Performance optimization
Basic
Next.js is a powerful framework for building server-side rendered (SSR) and statically generated websites and applications. Next.js Basic covers the essential concepts you need to know to get started.
You can create routes in Next.js by creating a file within the pages folder, which automatically makes that into a route. For example, creating a file called about.js will create a /about page.
To generate static pages for a template in Next.js, you need to define a getStaticPaths function. This function returns an object with fallback set to false, which makes it so that if you go to a route not in your array, you'll get a 404 page.
The Next.js Pages Router was introduced in 2016 and has a design choice that feels closer to Node.js than web APIs. However, frameworks must evolve over time, and Next.js 12 introduced Middleware, which is built on the Web Request, Response, and fetch.
To create a page in Next.js, you need to define a getStaticProps function. This function gets the params passed to it, in your case, the color name, and returns the data nested within { props }. For example, for the page /Marsala, it would return { "name": "Marsala", "hex": "#B57170" }.
Here's a quick rundown of the basic concepts you need to know:
- Install & Setup Tailwind CSS with NextJS
- How to import image in NextJS?
- How to add stylesheet in NextJS?
- How to start NextJS server?
Is a Language?
Next.js is not a coding language, it's an open-source framework designed to simplify building interactive web applications using React.
Next.js was created by Vercel, a company that specializes in web development tools.
It offers features for both server-side rendering (SSR) and static site generation (SSG), which enables you to create performant and SEO-friendly web experiences.
These features are particularly useful for building web applications that require fast loading times and good search engine rankings.
Next.js is built on top of React, a popular JavaScript library for building user interfaces.
Backend?
Next.js is often viewed as a frontend framework, but it's also capable of handling server-side logic. This is a key point to consider when deciding whether to use Next.js for your project.
Next.js backend capabilities are often overshadowed by its frontend features, but it’s equally competent in handling server-side logic.
Page Structure
In Next.js, the props object created in getStaticProps is passed to the component by the framework itself. This allows us to render dynamic data on the page.
The props object is used to pass data from getStaticProps to the component, making it possible to create a dynamic page. Next.js handles this process seamlessly.
To add a background color to the page, we can use the hex code passed through the props object. This is done by rendering the hex code in the component.
Deployment and Prerequisites
To deploy your Next.js app, start by creating an AWS account if you don't already have one. You can then navigate to the Amplify Console and follow the prompts to connect your app.
You'll need to choose GitHub as the source of your code, and the build settings will auto-populate. From there, you can simply click "Save and deploy."
To get started with Next.js, you'll need to have a good grasp of HTML and CSS, as well as JavaScript and ES6. React and Node.js with npm are also essential prerequisites.
Deployment
To get your Next.js app live online, you need to deploy it. AWS Amplify supports deploying both statically generated and server-side rendered Next.js apps without any additional configuration.
First, you need to create an AWS account if you don't already have one. This will give you access to the Amplify Console, where you'll be able to deploy your app.
To deploy your app, navigate to the Amplify Console and click on the orange "Connect app" button. You'll then be prompted to choose your existing code repository, which in this case is GitHub.
You'll need to create a build script in your package.json file if you're creating a statically generated Next.js app. Change your build script to "next build && next export" to prepare your app for deployment.
Here are the steps to connect your GitHub repository to the Amplify Console:
- Create an AWS account if you don't already have one.
- Navigate to the Amplify Console
- Click on the orange connect app button.
- Choose GitHub in the From your existing code menu, and click continue
Once you've connected your repository, you'll need to configure your build settings. The build settings will auto-populate, so you can simply click "Next" on the Configure build settings page.
Finally, click "Save and deploy" to deploy your app to AWS Amplify.
Prerequisites to Learn
To learn about deployment and prerequisites for Next.js, you'll need to have a solid foundation in several areas.
First and foremost, you should be comfortable with HTML and CSS, which are the building blocks of the web.
Next, you'll want to have a good grasp of JavaScript and ES6, which are essential for dynamic web development.
You should also have experience with React, which is a popular JavaScript library for building user interfaces.
Lastly, you'll need to know your way around Node.js and npm, which are used for server-side development and package management.
Here's a quick rundown of the prerequisites in a list:
- HTML and CSS
- JavaScript and ES6
- React
- Node.js and npm
Why Learn?
Learning Next.js is a no-brainer, especially if you're already familiar with React. Its built-in routing and server-side rendering capabilities make it a game-changer for developers.
Here are just a few reasons why Next.js stands out:
- Built-in Routing and SSR: Next.js provides seamless routing functionality out of the box, unlike traditional React development.
- Faster Development: Next.js accelerates development by offering built-in features and conventions, allowing developers to focus on building features rather than configuring complex setups.
- SEO Optimization: Next.js enhances SEO by addressing slow rendering and loading times associated with client-side rendering, and its SSR capabilities ensure that search engines can efficiently crawl and index your content.
With Next.js, you can say goodbye to slow rendering and loading times, which are a major concern for SEO performance. Its integration of server-side rendering out of the box is a huge time-saver for developers.
Frequently Asked Questions
What is the use of Next.js in React?
Next.js is a React framework that simplifies web application development by handling tooling and configuration, and providing additional structure and optimizations. It gives you the building blocks to create robust and efficient web applications with ease.
Is Next.js beginner friendly?
Yes, Next.js is beginner-friendly, with a step-by-step course that guides you through building a simple project from a JavaScript application to a React and Next.js application. This hands-on approach makes it easy to learn and master Next.js.
Featured Images: pexels.com