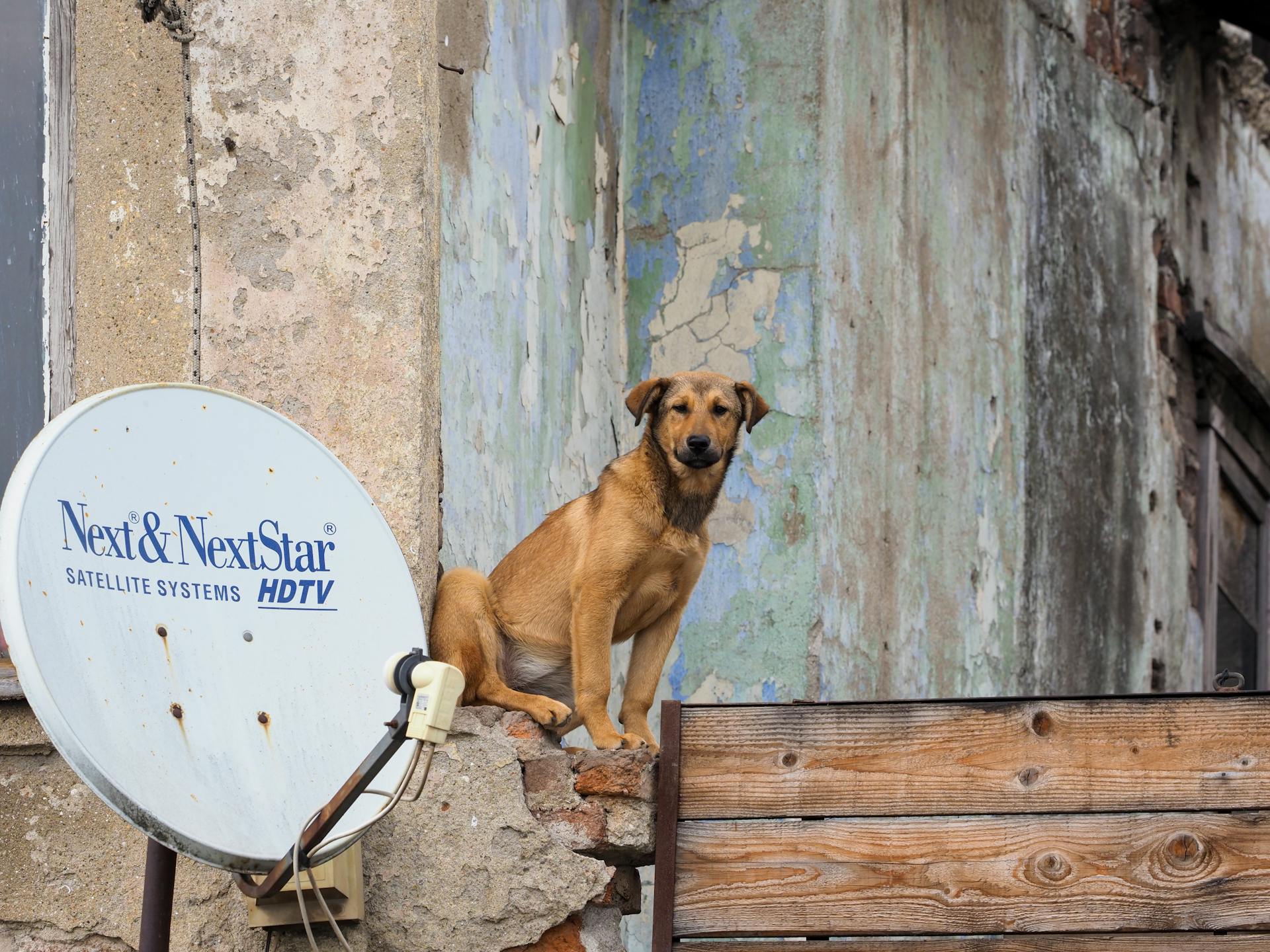
Next.js is a popular React framework for building fast and efficient websites. It's known for its server-side rendering capabilities.
One of the key benefits of Next.js is its ability to pre-render pages at build time, which can significantly improve page load times. This is especially useful for large websites with many pages.
With Next.js, you can also use its built-in internationalization (i18n) features to easily support multiple languages on your website.
A different take: Nextjs App vs Pages
Getting Started
To start with Next.js, you can use the CLI to create a brand new project using the create-next-app package. This will scaffold a new Next.js project with a src folder and a prompt to ask you some questions.
Next.js offers a lot of features with zero configuration, making it easy to get started. For example, page routing is built-in, so you don't need to write any code to create a route in your app.
Here are some basic steps to get started with Next.js:
- Create a Next.js project using the CLI or manual installation.
- Choose to use the App Router or Pages Router, depending on your needs.
- Set up Tailwind CSS in your Next.js project.
By following these steps, you'll be able to create a basic Next.js project and start building your app.
Prerequisites to Learn
To get started with Next.js, you'll need to have a solid foundation in some key areas.
HTML and CSS are essential for building the structure and layout of your web application.
You'll also need to have a good grasp of JavaScript and ES6, which are the building blocks of interactive web pages.
React is another crucial skill to have, as it's a popular JavaScript library for building user interfaces.
Node.js and npm are also required, as they provide the tools you need to build and deploy your Next.js application.
Here are the specific skills you'll need to learn Next.js:
- HTML and CSS
- JavaScript and ES6
- React
- Node.js and npm
Basic
To get started with Next.js, you'll need to have some prerequisites covered. You'll need to know HTML and CSS, JavaScript and ES6, React, and Node.js and npm.
Next.js is a framework that builds on top of React, so it's essential to have a good grasp of React fundamentals. If you're new to React, don't worry – there are plenty of resources available to help you get up to speed.
To start building with Next.js, you'll need to install and set up Tailwind CSS. This will give you a solid foundation for styling your app.
Here are some basic features you can expect from Next.js:
- NextJS Introduction: This will give you a brief overview of what Next.js is and what it can do.
- Install & Setup Tailwind CSS with NextJS: As mentioned earlier, this is essential for styling your app.
- Next VS React: This will help you understand the differences between Next.js and React.
- How to import image in NextJS?: This will show you how to import images into your Next.js app.
- How to add stylesheet in NextJS?: This will walk you through the process of adding stylesheets to your app.
- How to start NextJS server?: This will show you how to start the Next.js server.
Create a Project
To create a Next.js project, you can use the CLI or a manual installation. Follow the next steps to create a brand new Next.js project using the CLI or a manual installation.
You can find starter templates on GitHub or StackBlitz to get you started with the Tailwind CSS, React, Next.js, and Flowbite stack. These templates are a great way to jumpstart your project and save time.
To create a new Next.js project, use the create-next-app package to create a new Next.js project called next-admin. A prompt will ask you some questions, feel free to choose answers according to your needs.
Here are the steps to create a Next.js project:
- Use the create-next-app package to create a new Next.js project.
- Follow the prompts to answer questions about your project.
- Choose to use the App Router or Pages Router, or both.
You can also use the CLI to scaffold a new Flowbite React project using Next.js. Running the following command will automatically set up Flowbite React in a Next.js project environment.
Run the following command to build the project files:
- Run the following command to scaffold a new Flowbite React project using Next.js.
- Build the project files using the command.
That's it! You now have a basic Next.js project set up and ready to go.
Components and Routing
In Next.js, routing is a crucial aspect of building a React application, and it's essential to understand how it works.
To get the current route in Next.js, you can use a specific method, but unfortunately, that information is not provided in the article section. However, you can catch all routes in Next.js using a particular approach, which is more like a workaround than a straightforward solution.
Here are some key aspects of routing in Next.js:
- Catch all routes can be achieved using a specific method, but the details are not provided.
- Nested routes are another important aspect of Next.js routing.
- Dynamic API routes can be used to create flexible and reusable routing logic.
Functions
In Next.js, you can use server-side rendering to fetch data and render pages on the server. This is achieved through the getServerSideProps() function.
The getServerSideProps() function is a powerful tool that allows you to pre-render pages at build time, but also fetch data on the server-side for each request. This is particularly useful for pages that require dynamic data.
To handle dynamic routes, you can use the getStaticPaths() function. This function generates the paths for static pages at build time.
Expand your knowledge: Next Js Client Side Rendering
Next.js also provides a way to generate image metadata using the generateImageMetadata function. This function is useful for optimizing images and reducing page load times.
Fetch is another key function in Next.js that allows you to make HTTP requests to external APIs or services. This function is useful for retrieving data from external sources.
Here are some key functions in Next.js that you should know about:
- getServerSideProps()
- getStaticPaths()
- generateImageMetadata
- fetch
- generateStaticParams
- NextResponse
- NextRequest
Routing
Routing is a crucial aspect of building a Next.js application. You can get the current route in NextJS using the `useRouter` hook.
Next.js provides a built-in way to create your own APIs, called API routes. With API routes, you can create your own endpoints and handle incoming requests however you want. This is useful for creating a custom backend for your Next.js application or exposing data from your database to the front end.
To catch all routes in NextJS, you can create a new file at the following location: `src/app/api/admin/[...slug]/route.ts` for the App Router and `src/pages/api/admin/[[...slug]].ts` for the Pages Router.
Explore further: Nextjs Spa
Next.js's routing system is helpful, but it comes at a cost. By tightly coupling your routing logic to your pages, you make your pages heavier and more difficult to maintain.
To create a new page in the Next.js app, you can create a new file at the following location: `src/app/admin/page.tsx` for the App Router and `src/pages/admin/index.tsx` for the Pages Router.
Here is a list of ways to create routes in Next.js:
- File system routing: When you add a file to the page’s directory, it is automatically available as a route.
- Dynamic API Routes: You can create a catch-all API route by adding a new file at the following location: `src/app/api/admin/[...slug]/route.ts` for the App Router and `src/pages/api/admin/[[...slug]].ts` for the Pages Router.
- Nested Routes: You can create a new page in the Next.js app by creating a new file at the following location: `src/app/admin/page.tsx` for the App Router and `src/pages/admin/index.tsx` for the Pages Router.
Components
Components are a fundamental part of building web applications, and Next.js provides several features to make working with components easier.
Next.js uses React, which has its own set of features that make building components a breeze. React's JavaScript Syntax Extension (JSX) allows you to write HTML-like code in your JavaScript files, making it easy to create reusable components.
One of the key features of React is its Virtual DOM, which improves performance by only updating the parts of the DOM that need to be changed. This is especially useful when building complex web applications.
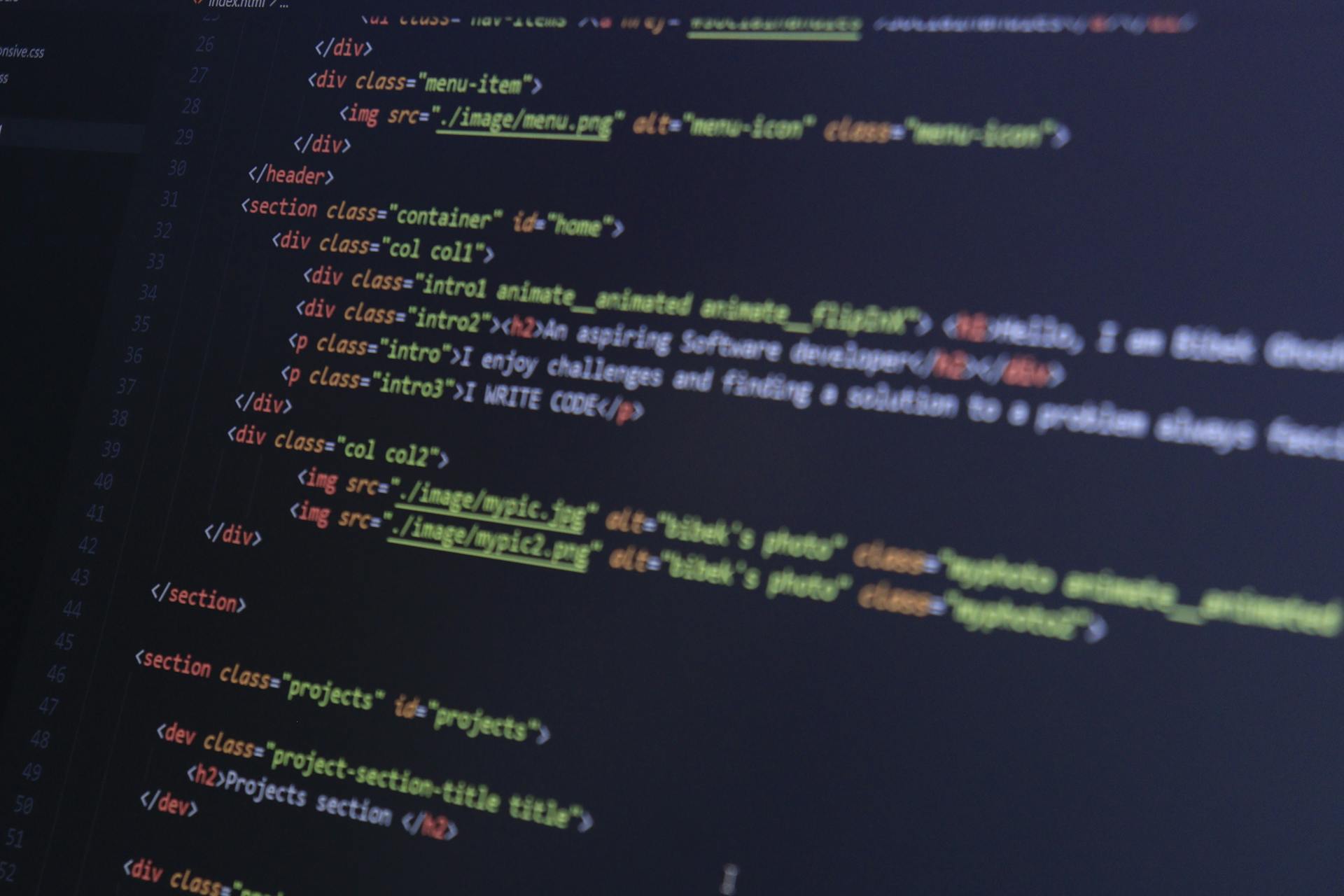
In Next.js, components can be created as separate files, and the framework will automatically handle the routing for you. This is thanks to Next.js' file system routing feature.
Here are some of the key features of React components:
- JavaScript Syntax Extension (JSX)
- Component
- Virtual DOM
- One-way data binding
These features, combined with Next.js' own features, make building web applications a much more efficient and enjoyable process.
Flowbite Components
Flowbite components are a great way to create reusable UI elements. You can import them from flowbite-react to use in your app.
To use Flowbite components, you can import them from flowbite-react. This makes it easy to add UI elements to your app.
Flowbite components can be used to create custom alerts and other UI elements. This helps to make your app more interactive and engaging.
React is a powerful JavaScript library that enables developers to create reusable UI elements, including Flowbite components. This makes it easy to build your app from small, reusable pieces.
A different take: Next Js vs Create React App
Data Provider
Next.js provides a built-in way to create your own APIs, called API routes, which give you a lot of flexibility in how you build your Next.js application.
API routes can be used to create a custom backend for your Next.js application or to expose data from your database to the front end.
To use API routes, you can create your own endpoints and handle incoming requests however you want.
You can use API routes to fetch and update data, as shown in Example 2, where the react-admin data provider is updated to use the Supabase adapter.
API routes are a powerful tool for building custom backends and exposing data to the front end.
Supabase provides a PostgREST endpoint, which is used with the ra-data-postgrest adapter to fetch and update data in the react-admin app.
This setup allows for a seamless data flow between the front end and the back end.
Additional reading: How to Update Nextjs
JavaScript Syntax Extension
JSX is a combination of JavaScript and HTML, used to create React elements. This syntax extension makes writing HTML in React much easier, eliminating the need to call React.createElement(component, props, ...children) every time.
Writing HTML directly in React can be quite a pain, but JSX simplifies this process by allowing you to write HTML as you're most familiar with it. Babel then transpiles the code to JavaScript during runtime, making it work seamlessly.
On a similar theme: Html to Json Parser Nextjs
Virtual DOM
The Virtual DOM is a concept created by the React team to improve rendering performance.
It's essentially a second instance of the actual DOM, created with JavaScript, which is much faster than the browser's DOM.
This virtual DOM makes a comparison between itself and a new virtual DOM whenever a state change occurs.
If the two DOMs are equal, the actual DOM remains untouched.
The actual DOM only gets updated when the virtual DOM detects a difference between the two instances.
This approach significantly reduces the number of DOM manipulations, making the application more efficient.
Automatic Code Splitting
Automatic code splitting is a game-changer for Next.js applications. As your app grows, the size of third-party libraries, CSS, and JavaScript files increases, making page loads slower.
This is especially true for large files that need to be downloaded on page load. Next.js solves this issue by automatically splitting code into smaller units.
With automatic code splitting, scripts are only downloaded when required, significantly improving performance. This means users get a faster experience, and your app's load times are reduced.
As a result, users are more likely to stay engaged, and your app's overall performance improves.
Take a look at this: Nextjs Code Block
Frequently Asked Questions
Is NextJS better than ReactJS?
NextJS is a more feature-rich and opinionated alternative to ReactJS, particularly suitable for SEO-focused websites. Its added capabilities make it worth considering for projects requiring pre-rendering and search engine optimization.
Is NextJS frontend or backend?
NextJS excels as a frontend framework, with a focus on client-side rendering and development. Its backend capabilities are secondary, but still available for server-side rendering and basic functionality.
Should I use React or NextJS in 2024?
For projects requiring high-performance optimizations, consider React. For content-heavy websites and e-commerce platforms needing SEO optimization, Next.js is a better fit.
Featured Images: pexels.com