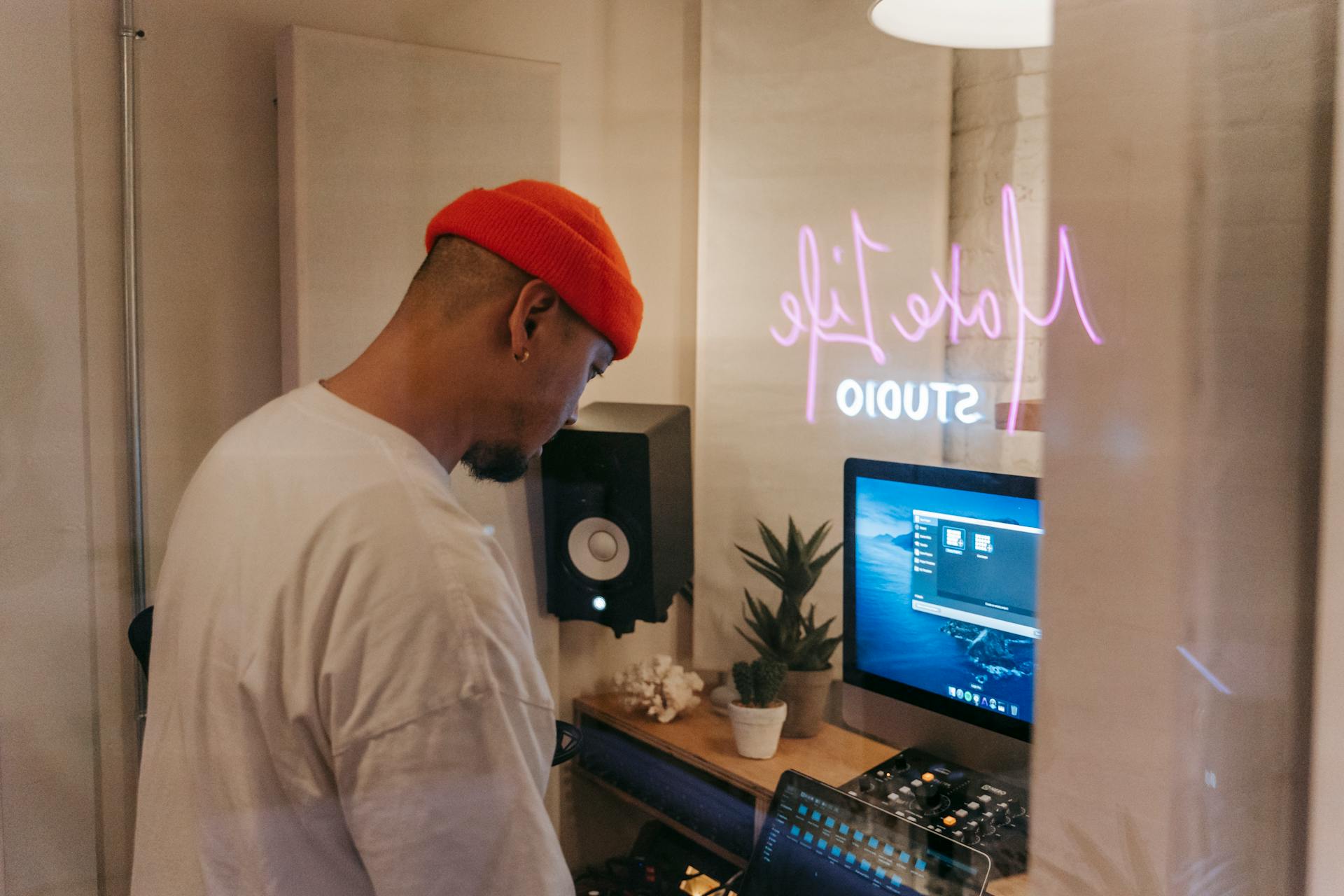
File upload in Next.js with Supabase can be a breeze, especially with the right tools and setup. You can create a file upload system in Next.js using Supabase's API endpoint.
To start, you'll need to create a Supabase project and enable the Storage feature, which allows you to store and manage files. This feature provides a simple and secure way to handle file uploads.
Supabase's Storage feature allows you to upload files up to 5 GB in size, and you can also set custom upload policies to control how files are stored and accessed. With the right configuration, you can ensure that your file uploads are secure and efficient.
Take a look at this: Nextjs Client Side Cookies
Benefits and Usage
Supabase Storage offers several benefits that make it a great choice for file uploads. It has a simple API for file uploads and downloads.
One of the main advantages of Supabase Storage is its ease of use. Supabase has a simple API for file uploads and downloads. This makes it easy to integrate into your Next.js application.
Broaden your view: Next Js Drizzle Supabase
Supabase Storage is also secure, with access control and permission settings to keep your data safe. Scalability is another key benefit, as Supabase is built with scalability in mind to handle growing amounts of data efficiently.
To integrate Supabase Storage with your Next.js application, you'll need to install the Supabase client library.
A different take: Nextjs App Route Get Ssr Data
Next.js and Supabase Overview
Next.js and Supabase are two powerful tools that can help you build fast, scalable, and secure web applications. Supabase is an open-source alternative to Firebase, providing a PostgreSQL database, authentication, and APIs.
Next.js is a popular React framework for building server-side rendered (SSR) and statically generated websites and applications. It allows for seamless client-side routing and server-side rendering.
Supabase's PostgreSQL database offers features like data encryption, row-level security, and support for SQL queries. With Next.js, you can easily integrate Supabase's APIs into your application.
Next.js also provides built-in support for internationalization (i18n) and server-side rendering (SSR), making it easier to build complex web applications.
Explore further: Next Js Client Side Rendering
Benefits of File Upload
Uploading files can be a breeze with Supabase. You can easily upload files with their simple API, making it a great option for developers.
Supabase's API is designed to be easy to use, so you can focus on building your application without worrying about the complexities of file uploads.
One of the key benefits of using Supabase for file uploads is its scalability. This means you can handle growing amounts of data efficiently, without any hiccups.
Here are some key benefits of Supabase's file upload feature:
- Easy to use API for file uploads and downloads
- Access control and permission settings for secure data storage
- Scalable design to handle growing amounts of data efficiently
Common Use Cases
Supabase Storage is a powerful tool that can be used in a variety of ways, but one of its most common use cases is integrating it with a Next.js application.
We can set up a project, connect to Supabase, and implement file upload and retrieval functionality with ease. This includes doing a simple image upload and retrieval.
Supabase Storage can be used to store and manage files, making it a great solution for applications that require file uploads and downloads.
To get started, we can install the Supabase client library, which will allow us to connect to our Supabase instance and start using the Storage feature.
A different take: Connect Rpc Nextjs
Setting Up File Upload
Setting up file upload in a Next.js app with Supabase involves creating a storage bucket and setting up the Supabase client. You can create a storage bucket in Supabase by going to the Supabase Storage page in the Dashboard and clicking New Bucket.
To create a storage bucket, you'll need to enter a name for the bucket, such as "images", and then click Create Bucket. It's also important to set up an access policy that allows your application to insert and read data in the storage bucket.
To set up the access policy, you'll need to follow these steps: go to the Storage tab on the left-hand sidebar, select your storage bucket, and click on the Access tab. Then, under Bucket policies, click the New policy button and select the For full customization option to create a policy from scratch.
Explore further: New Nextjs Project Typescript
Creating a Bucket
To create a bucket in Supabase, you can use the dashboard or the client library. You can name your bucket anything you want, but it's a good idea to choose a name that reflects the type of files you'll be storing in it.
For example, you can name your bucket "images" if you'll be storing images in it. To create a bucket using the dashboard, follow these steps:
- Go to the Supabase Storage page in the Dashboard.
- Click New Bucket and enter a name for the bucket.
- Click Create Bucket.
You can also play around with the bucket settings, such as setting permissions and making the bucket public.
Client Setup
To set up the Supabase client, start by installing the supabase-js client library via the terminal. This is the first step in integrating Supabase storage into your Next.js app.
You'll need to create a new folder named lib at the root of your application or in the src folder if you're using it. This is where you'll store the Supabase client configuration.
In this folder, add a new file named supabase.js and use the following code to initialize the Supabase client. Replace the project URL and the anon key with your own details from the Supabase project settings.
You can copy the details in the .env file and reference them from there. This will help keep your project organized and secure.
With the Supabase client set up, you're now ready to move on to the next step: uploading images to the storage bucket. This is where things get really interesting, and you'll learn how to make it happen in the next section.
Here's an interesting read: What Is .next Folder in Next Js
Creating an Image Form
To create an image form, you need to create a new page in your Next.js app. This can be done by creating a subfolder named upload and adding a new file named page.js. If you're using Next.js 12, create upload.js in the pages folder instead.
Inside the upload page, add the following code to create the form. This code will create a form that accepts images.
To handle the form submission, you'll need to create a handleSubmit function. This function will be called when the user selects a file and clicks the submit button.
To create a unique file name, you can use the uuid package. This is recommended to avoid overwriting files that share the same name. To install the uuid package, run the command npm install uuid in the terminal.
Here's an example of how to import version 4 of uuid at the top of your file:
```html
Related reading: Next Js Npm Install
import { v4 as uuidv4 } from 'uuid';
```
To upload the image to the storage bucket, you'll need to use the Supabase client. This involves passing the path to the image and the image itself to the client. The path works the same as it does in the file system.
Here's an example of how to use the Supabase client to upload the file:
```html
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = 'https://your-supabase-url.supabase.co';
const supabaseKey = 'your-supabase-key';
const supabaseSecret = 'your-supabase-secret';
const supabase = createClient(supabaseUrl, supabaseKey, supabaseSecret);
const file = new File([image], fileName, { type: 'image/jpeg' });
const { data, error } = await supabase.storage.from('images').upload(file.path, file);
```
Note that you'll need to replace 'your-supabase-url', 'your-supabase-key', and 'your-supabase-secret' with your actual Supabase URL, key, and secret.
A unique perspective: Loading Page Nextjs
Uploading and Serving Images
Uploading images to a Supabase storage bucket is a straightforward process. You can create a form to accept images and handle the upload in the handleSubmit function using the Supabase client.
To handle the upload, you'll need to create a unique file name by concatenating the file name and a UUID generated by the uuid npm package. This is recommended to avoid overwriting files that share the same name.
You'll need to install the uuid package via npm and import version 4 of uuid at the top of your file. The Supabase client will then upload the file to the storage bucket called "images".
Here are the steps to create an access policy that allows your application to insert and read data in a Supabase storage bucket:
- On your project dashboard, click on the Storage tab on the left-hand sidebar.
- Select your storage bucket and click on the Access tab.
- Under Bucket policies, click the New policy button.
- Select the For full customization option to create a policy from scratch.
- In the Add policy dialog, enter a name for your policy (e.g. "Allow Insert and Read").
- Select INSERT and SELECT permissions from the Allowed operations dropdown menu.
- Click the Review button to review the policies.
- Click the Save button to add the policy.
After uploading the image, you can retrieve the public URL of the uploaded image using the `getPublicUrl` method on the same storage bucket. You can then set the URL in the `imageUrl` state, triggering a re-render of your component to display the uploaded image.
To serve the image on your site, you can use the Supabase client to retrieve the public URL of the uploaded image. Alternatively, you can manually concatenate the bucket URL with the file path.
Conclusion and Next Steps
You've successfully set up a Next.js 14 project and created a React form for file uploads using Server Actions. This approach simplifies state handling with direct access to form data.
To take your project to the next level, consider implementing the following recommendations:
- Only allow unique visitors by storing IP hashes or using localStorage, which could be accomplished with Supabase Edge functions.
- Build a visualization page for interacting and querying the results.
- Expand your analytics to include tracking the referer value.
- Ignore certain user agents to reflect a more accurate view count.
By following these next steps, you'll be able to enhance your file upload functionality and gain more insights into your users' behavior.
Conclusion
Setting up a Next.js 14 project is a great starting point for building dynamic applications.
You can create a React form for file uploads, which is a crucial step in handling file uploads in Next.js.
Server Actions provide a simpler and more integrated approach than traditional API routes, eliminating the need for separate API management.
Direct access to form data simplifies state handling, making it easier to manage complex application logic.
Writing server-side logic directly alongside your front-end code streamlines development and reduces the need for separate API management.
This approach can significantly reduce development time and improve overall efficiency in building dynamic applications.
Consider reading: Nextjs Forms
Next Steps
Now that you've got a solid foundation, it's time to think about how to take your project to the next level. You can only allow unique visitors by storing IP hashes or using localStorage, which could probably be accomplished with Supabase Edge functions.
There are several ways to expand your analytics, such as keeping track of the referer value. This will give you a more accurate view of how users are finding your project.
Here are some specific recommendations for next steps:
- Only allow unique visitors, perhaps by storing IP hashes or using localStorage.
- Build a visualization page for interacting and querying the results.
- Expand your analytics to include things like keeping track of the referer value.
- Ignore certain user agents to reflect a more accurate view count.
Frequently Asked Questions
How to load data from a file in next js?
To load data from a file in Next.js, import the file system library and use it to read the file, then parse its contents as JSON data. Follow these steps to get started: import fs, create a server component, read the file, and parse its contents.
Sources
- https://kodaschool.com/blog/next-js-and-supabase-how-to-store-and-serve-images
- https://www.makeuseof.com/next-js-upload-images-supabase-storage-bucket/
- https://akoskm.com/file-upload-with-nextjs-14-and-server-actions/
- https://maxleiter.com/blog/supabase-next-analytics
- https://supabase.com/docs/reference/javascript/v1/storage-from-upload
Featured Images: pexels.com