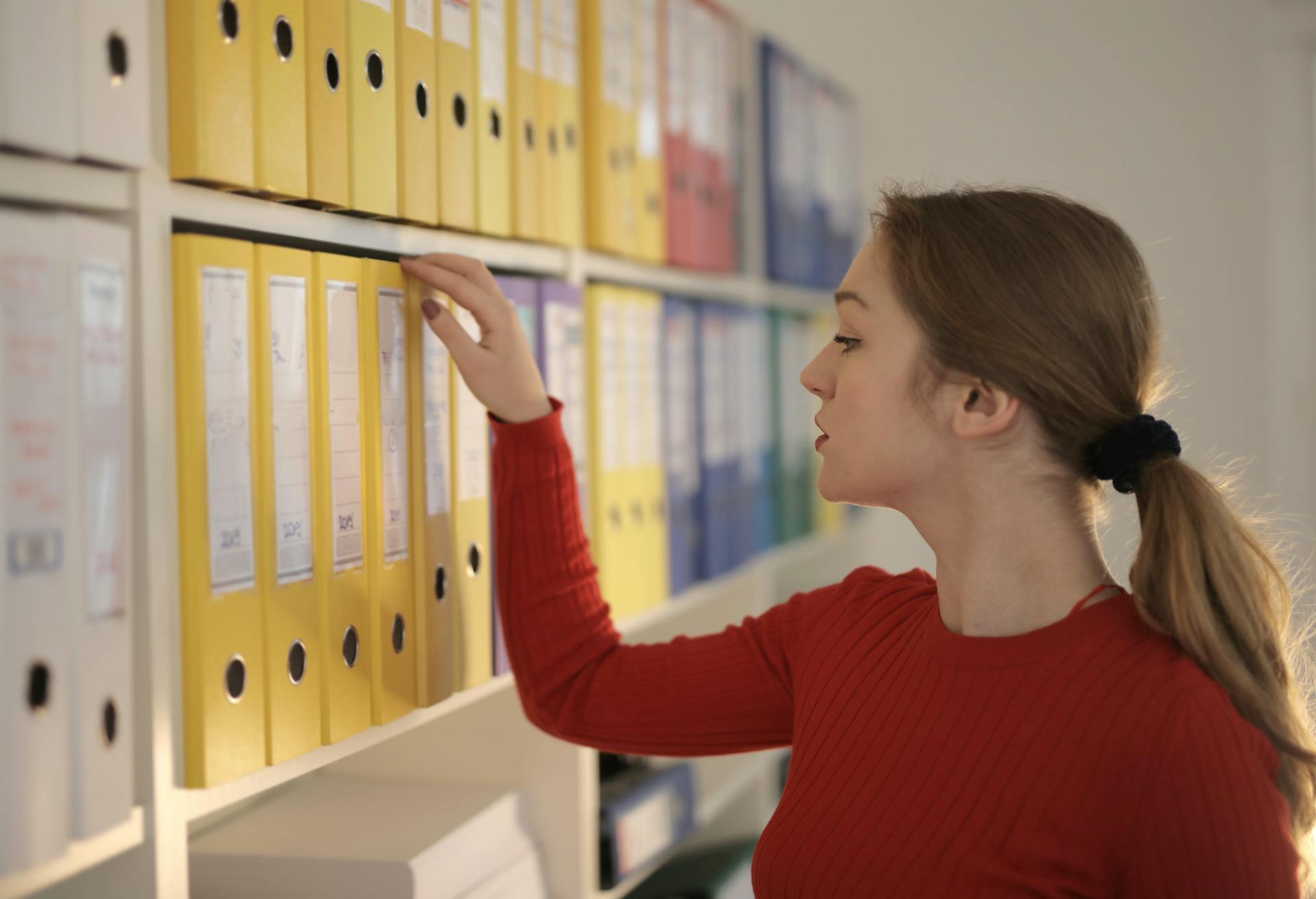
Uploading multiple files to a website can be a real challenge, but it's a crucial feature for many applications. HTML5 introduced a new attribute called "multiple" that allows users to select multiple files at once.
To make use of this feature, you'll need to add the "multiple" attribute to the file input field in your HTML code. This attribute is what enables the user to select multiple files.
Using the "multiple" attribute can greatly enhance the user experience, making it easier and more convenient for them to upload multiple files at once.
File Upload Options
When it comes to uploading multiple files in HTML, you have several options to choose from. The most common method is using the HTML input element with the multiple attribute, allowing users to select and upload multiple files at once.
The HTML input element can be styled to fit your application's design, making it a versatile option. You can use CSS to change the input element's appearance, such as its size, color, and shape.
Discover more: Html Text Element
The multiple attribute can also be combined with other attributes, like the accept attribute, to specify the types of files that can be uploaded. For example, you can use accept="image/*" to allow only image files to be uploaded.
In some cases, you may want to provide a default file to be uploaded when the user submits the form. This can be achieved by setting the value attribute of the input element to the default file path.
It's worth noting that older browsers may not support the multiple attribute, so it's essential to provide a fallback solution, such as a separate input element for each file upload.
Customizing Upload
You can customize your file uploads by using a list of bytes or UploadFile. This is achieved by declaring a list of bytes or UploadFile.
Using a list allows you to receive multiple files associated with the same form field, sent using form data. This is a convenient way to handle multiple file uploads at once.
You can also use the File() function to set additional parameters, including metadata for each uploaded file. This can be especially useful if you need to store extra information about the uploaded files.
Worth a look: How to Upload File to Google Cloud Storage Using Reactjs
Limiting Accepted Types
You can restrict the types of files users can select by using the accept attribute, which will streamline the file selection process for users.
This attribute is a guideline for the file picker and relies on file MIME types, so users can still manually choose to display all file types and select files that don't match the specified criteria.
To allow only images, use the accept="image/*" attribute, which limits the file picker to all image file types, including JPEG, PNG, GIF, and others.
You can specify multiple types by separating them with a comma, like this: accept="image/*, application/pdf".
It's essential to note that the accept attribute should not be the sole line of defense, as users can still find ways to bypass it. Always pair it with robust server-side validation to ensure that the uploaded files are indeed the types that your application expects and can safely handle.
If this caught your attention, see: Itext Insert Image in Header File from Html
Button Customization
You can create a more visually appealing and consistent user interface by hiding the default file input and using a custom-styled label as a proxy button for file selection.
Setting the file input's opacity style to 0 is a more accessible approach compared to using visibility: hidden or display: none, ensuring the file input remains functional for users relying on assistive technologies.
To hide the file input, you can set its opacity style to 0, effectively removing it from the user interface.
You can then create a label element and associate it with the file input using the for attribute, which should match the id of the input element.
Styling this label as you would any button allows you to create a visually appealing and consistent user interface.
Using opacity:0, you can also use position: absolute; z-index: -1; to remove the native input element from the normal flow of the document and prevent it from taking up space or blocking other elements.
If this caught your attention, see: Html Styling Text
Getting Selected Files
You can retrieve information about the selected files using JavaScript. This is useful for validation and displaying file names, sizes, or types.
The HTMLInputElement.files property provides access to the selected files. This property is a FileList object, which is essentially a collection of File objects.
Each File object within this list provides several pieces of information about the file, including its name, last modified date, size, and type.
Here are the details you can get from each File object:
- name: a string representing the file's name
- lastModified: a long integer indicating the time the file was last modified, measured in milliseconds since January 1, 1970
- size: the file's size in bytes, useful for validating file size before uploading
- type: a string indicating the file's MIME type, which can be used to validate the file format
The lastModified property returns a timestamp measured in milliseconds since the UNIX epoch, which is January 1, 1970.
Upload Process
Uploading multiple files is a breeze with the right tools. You can use the File() function to set additional parameters, even for UploadFile.
To get started, you'll need to create a File() object for each file you want to upload. This will allow you to customize the upload process for each file individually.
The File() function can take various parameters, including the file itself, its metadata, and even custom parameters. This flexibility makes it easy to handle different file types and upload scenarios.
You can use the File() function to set additional parameters for UploadFile, just like you would for a single file upload. This allows you to maintain consistency in your code and make it easier to manage multiple file uploads.
By using the File() function, you can streamline your upload process and make it more efficient. This is especially useful when dealing with large numbers of files or complex upload scenarios.
Uploads
You can upload multiple files at once, just like you would with a single file. This is done using the File() method.
The File() method can be used to set additional parameters, even for UploadFile. This is a convenient feature that allows for more flexibility in file uploads.
To upload multiple files, you can use the same method as before, without any changes. The File() method will take care of the rest.
You can also use the File() method to add extra metadata to your uploaded files. This is a useful feature that can help with file organization and management.
Frameworks and Adapters
FilePond is a versatile tool that can be integrated with various frameworks to make uploading multiple files a breeze.
If you're using React, Vue, Svelte, Angular, or jQuery, you're in luck – FilePond has adapters for all of these popular frameworks.
These adapters make it easy to use FilePond with your existing code, so you can focus on what matters most – building a great user experience.
Here are some of the frameworks and adapters you can use with FilePond:
- React
- Vue
- Svelte
- Angular
- jQuery
Examples and Demos
You can create a multi-file drop area by adding the multiple attribute to a file input. This allows users to upload multiple files at once.
To limit the maximum amount of files that can be uploaded, use the data-max-files attribute. This prevents malicious users from uploading a larger number of files than expected.
Files can be reordered by grabbing a file and dragging it to a new location. This feature is useful for organizing uploaded files.
Image editing is powered by Pintura, which integrates beautifully with FilePond. This provides a seamless image editing experience for users.
The following examples demonstrate multiple file upload in a component. InputFileChangeEventArgs.GetMultipleFiles allows reading multiple files, while InputFileChangeEventArgs.File allows reading the first and only file if the file upload doesn't support multiple files.
Here are some key points to note about InputFileChangeEventArgs:
To use these examples in a test app, you'll need to register controller services and map controller endpoints. This is necessary for routing to controller actions in ASP.NET Core.
Overview and Basics
FilePond is a powerful tool for uploading multiple files to your HTML page. It accepts a wide range of file types, including directories, files, blobs, local URLs, remote URLs, and Data URIs.
You can interact with FilePond in various ways, such as dropping files, selecting files from the file system, adding files using the API, or copying and pasting files. This flexibility makes it easy to incorporate into your project.
Here are the different ways you can send files to the server:
- XMLHttpRequest
- Form post as base64 using the File Encode plugin
Feature Overview
FilePond's feature set is quite impressive, and it's worth taking a closer look. It accepts directories, files, blobs, local URLs, remote URLs, and Data URIs, making it a versatile tool for handling various file types.
You can interact with FilePond in several ways, including dropping files, selecting files from the file system, adding files using the API, or even copying and pasting files.
One of the standout features of FilePond is its ability to automatically resize and crop images on the client-side, which not only saves server bandwidth but also dramatically increases upload speed.
If you're concerned about accessibility, FilePond has been tested with AT software like VoiceOver and JAWS, and its user interface is navigable by keyboard.
FilePond's interface is also highly adaptable, automatically scaling to available space on both mobile and desktop devices.
What is Form Data
Form data is a special encoding used by HTML forms to send data to the server, it's different from JSON.
This encoding is called "application/x-www-form-urlencoded" when the form doesn't include files.
If a form includes files, it's encoded as "multipart/form-data", which is a different type of encoding.
You can declare multiple File and Form parameters in a path operation, but not Body fields that expect JSON.
This is because the request will have the body encoded using "multipart/form-data" instead of "application/json", which is part of the HTTP protocol.
Related reading: Html Form File Upload
Featured Images: pexels.com