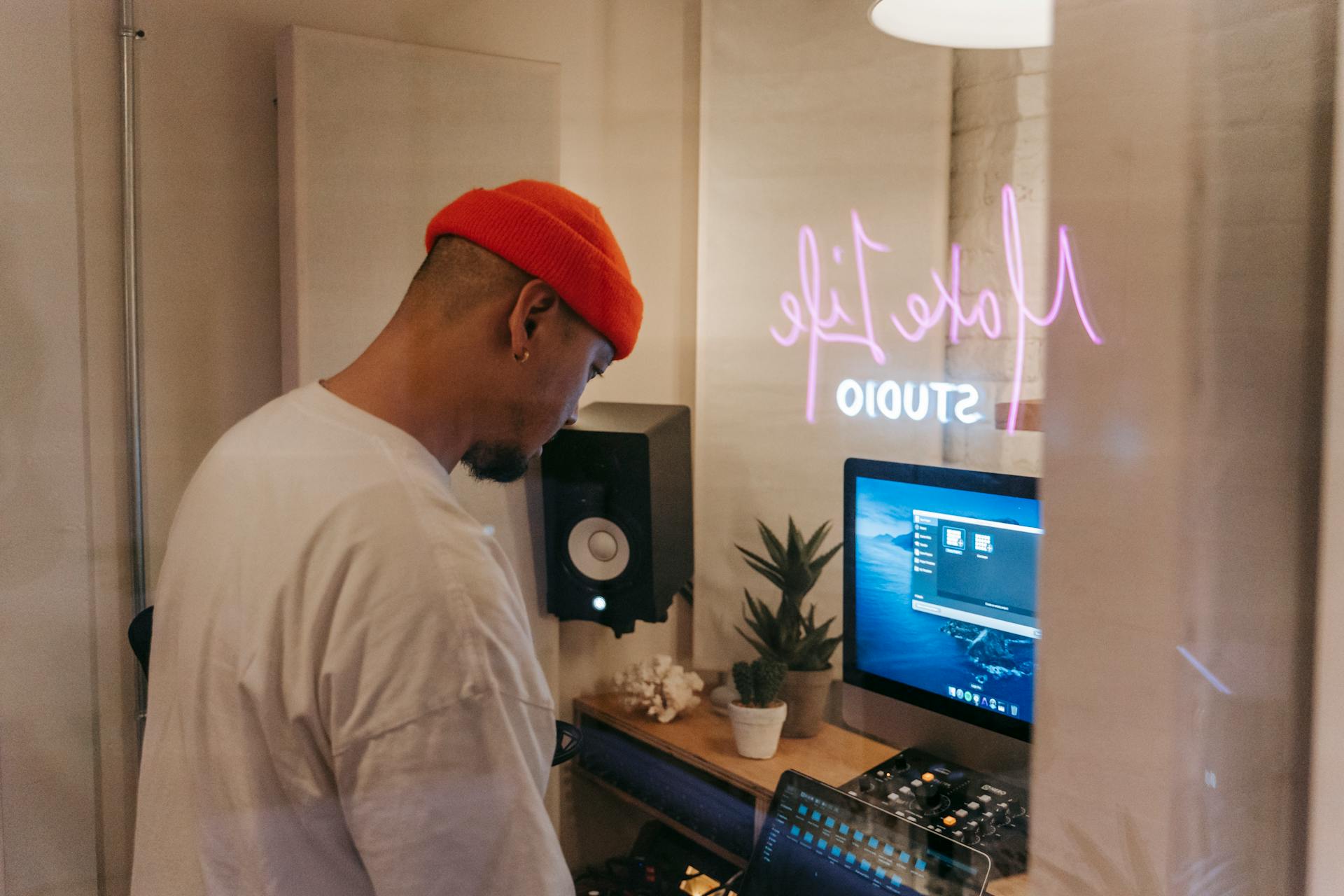
In ASP.NET Core, you can handle file uploads using the IFormFile interface, which provides a way to access the uploaded file.
To use this interface, you need to add the Microsoft.AspNetCore.Mvc.FormFile NuGet package to your project.
To create a form that allows users to upload files, you need to include the enctype attribute in the form tag, which specifies the character encoding for the form data.
The enctype attribute should be set to multipart/form-data, which is the required encoding for file uploads.
This encoding tells the browser to send the form data as a multipart request, which includes the file contents.
Check this out: Data Text Html
HTML Form Basics
To upload files in an HTML form, you'll need to use a form with a special multipart/form-data encoding. This lets you mix standard form data with file attachment data.
The HTTP method used to submit the form must be POST, not GET. This is a crucial detail to keep in mind when setting up your form.
Add a CSRF token to the form, unless you have the CSRF filter disabled. This improves security and avoids potential errors.
The CSRF filter checks the multi-part form in the order the fields are listed, so put the CSRF token before the file input field. This is a good practice to follow for efficiency.
An empty file will be treated just like no file was uploaded at all. This is something to keep in mind when handling file uploads in your application.
Server-Side Handling
To handle file uploads on the server side, you'll need to create a folder named "uploads" on your web server at the same path level as the upload script. This folder is where the uploaded files will be saved.
The PHP code in the upload script checks if the form is posted and if the file is uploaded successfully. It then moves the uploaded file to the uploads sub-folder using the move_uploaded_file() function.
To ensure successful file uploads, you'll need to set up the web server configuration correctly. This includes checking the folder permissions to make sure the web server user has write access to the folder. You can do this using the 'chmod' command if you have shell access, or through your hosting control panel if you don't.
Here are the typical web server configuration settings you'll need to check:
- memory_limit
- upload_max_filesize
- post_max_size
Server Handling
Server Handling is a crucial aspect of file uploads on the server side. To handle file uploads, you need to create a folder named "uploads" on your web server at the same path level as the upload script.
This folder is where you'll save the uploaded files. You'll also need to add PHP code to handle the upload, which involves checking if the form is posted and if the file is uploaded successfully.
To ensure file uploads work fine, the folder where files are saved must be writable by the script. This means the web server user (usually www-data) should have write access to the folder.
You can update folder permissions using the 'chmod' command if you have shell access. If not, you can check your hosting control panel for this feature.
Here are some key configuration parameters to set up for successful file uploads:
- memory_limit
- upload_max_filesize
- post_max_size
These parameters determine whether file uploads are allowed and set a limit on the size of the file upload.
Kestrel Max Request Body Size
The default maximum request body size for apps hosted by Kestrel is 30,000,000 bytes, which is approximately 28.6 MB. This is a pretty big limit, but you can customize it if you need to.
To change the limit, you can use the MaxRequestBodySize Kestrel server option. This is a useful feature if you're dealing with large files.
You can also use the RequestSizeLimitAttribute to set the MaxRequestBodySize for a single page or action. This attribute can be applied to the page handler class or action method, or using the @attribute Razor directive.
Here's a quick rundown of the options:
Security Considerations
Security considerations are crucial when allowing users to upload files to your server. Cyberattackers may attempt to execute denial of service attacks, upload viruses or malware, and compromise networks and servers in other ways.
Use a dedicated file upload area, preferably on a non-system drive, to make it easier to impose security restrictions on uploaded files. Disable execute permissions on the file upload location to prevent malicious scripts from running.
Don't persist uploaded files in the same directory tree as the app, and use a safe file name determined by the app instead of the user-provided file name. HTML encode the untrusted file name when displaying it to prevent XSS attacks.
Only allow approved file extensions for the app's design specification, and check the file format signature to prevent masqueraded files. For example, don't permit a user to upload an *.exe* file with a *.txt* extension.
Verify that client-side checks are performed on the server, as client-side checks are easy to circumvent. Check the size of an uploaded file and set a maximum size limit to prevent large uploads.
Here are some key security steps to reduce the likelihood of a successful attack:
- Upload files to a dedicated file upload area.
- Disable execute permissions on the file upload location.
- Use a safe file name determined by the app.
- Allow only approved file extensions.
- Verify client-side checks on the server.
- Check the size of an uploaded file.
- Run a virus/malware scanner on uploaded content.
Troubleshooting and Error Handling
The server's configured content length can be a major issue when uploading files, and the error message will indicate that the uploaded file exceeds this limit.
If you encounter this error, check the server's configuration to see if it needs to be increased to accommodate larger files.
The error message will be a clear indication of the problem, so be sure to read it carefully to understand the solution.
Race Conditions in URL-Based Systems
Race Conditions in URL-Based Systems can be particularly tricky to deal with. They can occur in functions that allow you to upload a file by providing a URL, where the server has to fetch the file over the internet and create a local copy before it can perform any validation.
In these cases, developers are unable to use their framework's built-in mechanisms for securely validating files. This means they may manually create their own processes for temporarily storing and validating the file, which may not be quite as secure.
See what others are reading: How to Create Index Html File
For example, if the file is loaded into a temporary directory with a randomized name, it should be impossible for an attacker to exploit any race conditions. However, if the randomized directory name is generated using pseudo-random functions like PHP's uniqid(), it can potentially be brute-forced.
Uploading a larger file can make attacks like this easier, by lengthening the window for brute-forcing the directory name. This is because larger files are often processed in chunks, allowing attackers to potentially take advantage of this by creating a malicious file with the payload at the start, followed by a large number of arbitrary padding bytes.
Null Reference Exception
Null Reference Exception is a common issue that can occur when working with file uploads.
This error often arises when the IFormFile is null, which can be caused by a missing enctype attribute in the HTML form.
Make sure the enctype attribute is set to multipart/form-data on the form element, as this is required for file uploads.
Also, verify that the upload naming in form data matches the app's naming, as a mismatch can result in a null IFormFile.
I've seen this issue arise when developers forget to include the enctype attribute, so double-check your form tags to avoid this problem.
Connection Failure
Connection failure can be a real headache. A connection error and a reset server connection probably indicates that the uploaded file exceeds Kestrel's maximum request body size.
If you're experiencing connection failure, check the Kestrel maximum request body size section for more information. You may need to adjust Kestrel client connection limits.
Large files can cause connection issues. Kestrel's maximum request body size may need to be adjusted to accommodate larger files.
Frequently Asked Questions
How will you upload files using forms in HTML?
To upload files using forms in HTML, you can use the `` element within a `
How to store an uploaded file in HTML?
To store an uploaded file in HTML, you can use the `` element to select a file, and then use the `input` event to capture the selected file and store it on your server-side using a programming language like JavaScript or PHP.
Featured Images: pexels.com