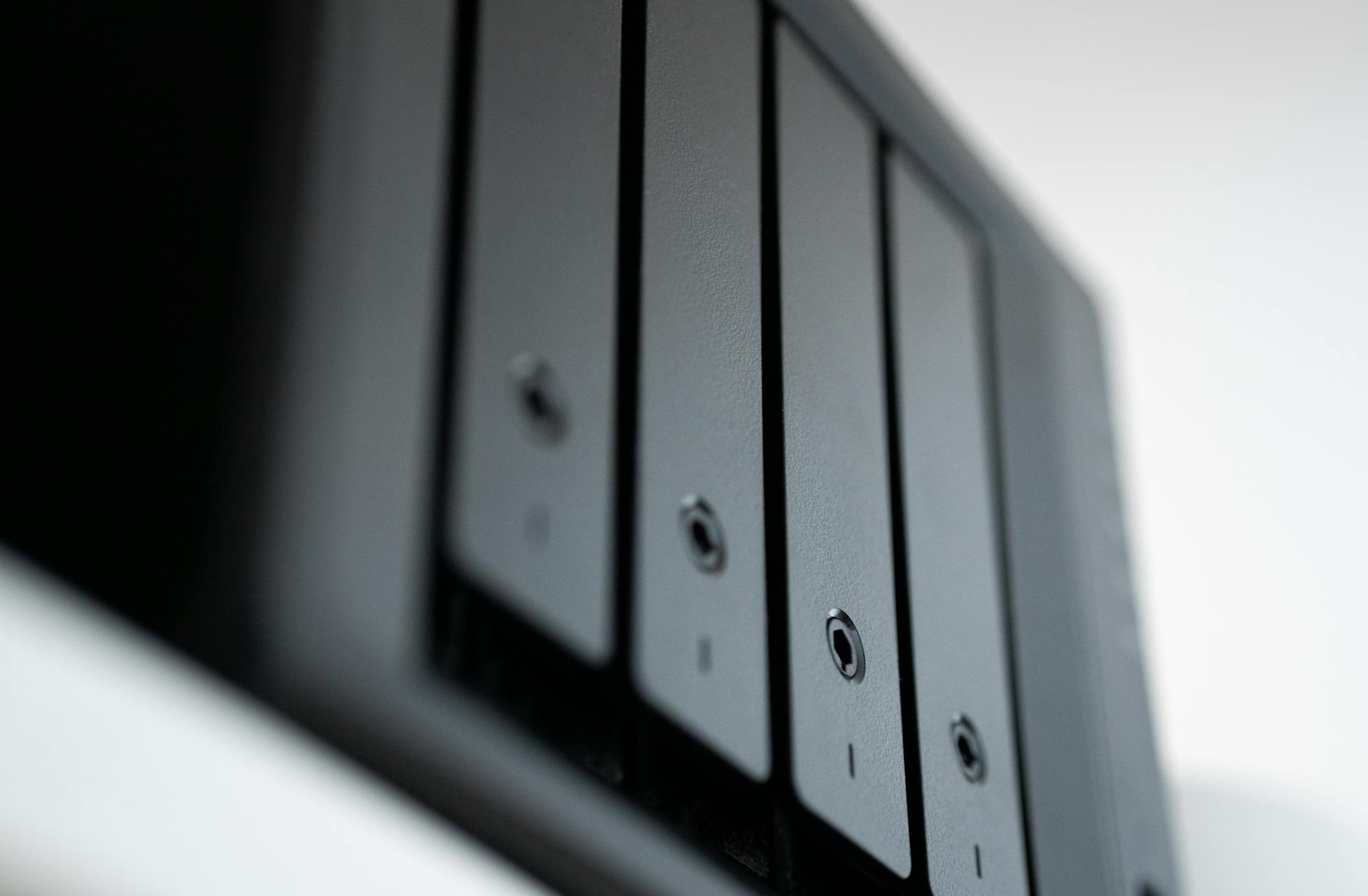
To upload a file to Google Cloud Storage using ReactJS with a NodeJS backend, you'll need to create a service account and enable the Cloud Storage API in the Google Cloud Console. This will provide you with a key file that you'll use to authenticate your API requests.
First, create a new project in the Google Cloud Console and navigate to the API Library page. Select the Cloud Storage API and click on the Enable button to enable it. This will allow you to use the Cloud Storage API in your NodeJS backend.
You'll also need to install the Google Cloud Client Library for NodeJS, which will handle the authentication and API requests for you. This library is available on npm and can be installed using the command `npm install @google-cloud/storage`.
To set up authentication for your NodeJS backend, you'll need to create a service account key file and import it into your NodeJS application. This key file will be used to authenticate your API requests to Google Cloud Storage.
A fresh viewpoint: Google Storage Api
Prerequisites
To upload files to Google Cloud Storage using ReactJS, you need to have knowledge about JavaScript and NodeJS. This is a fundamental requirement to move forward with the process.
Resumable upload is a feature that allows users to upload large files by breaking them down into smaller chunks. This is particularly useful when dealing with large files, as it eliminates the need to re-upload the entire file in case of an error.
You'll need to create a signed URL, which is a type of URL that is valid for a restricted time before it expires. Service providers like GCP and AWS offer this feature.
To get started, you'll need to obtain a service account from the Google Developer Console. This will involve filling out a form with your service account details and downloading a JSON file that will serve as your secret for authentication.
A different take: Upload Url Google Cloud Storage
Google Cloud Buckets
Google Cloud Buckets are a cost-effective solution for storing large binary data, such as images. They prevent database performance degradation as the number and size of files increase.
To use Google Cloud Buckets, you can create a key file that contains confidential keys and links for accessibility, which you should store securely within the root of your application.
You'll need to download the required dependencies necessary for the demo, which will enable you to start programming your import feature.
Google Cloud Buckets can be used as a Cloud Service, and there are several options available in the market, such as Amazon S3, Azure Storage, and Google Cloud Storage. We use Google Cloud Storage as a Cloud Service for our use case.
To upload files to Google Cloud Buckets, you can use Firebase Cloud Functions to authenticate the user and generate a Signed URL, which addresses security concerns and prevents direct upload from the front-end.
Here's an interesting read: Does More Airflow Use More Juice?
Backend Part:
To upload a file to Google Cloud Storage using ReactJS, you'll need to set up your backend correctly. First, install the necessary dependencies, including @google-cloud/storage, cors, and multer. These packages will help you interact with the storage bucket, enable CORS, and handle multipart/form-data for file uploads.
A unique perspective: Azure Files vs Blob
To configure CORS, you'll need to specify the responseHeader and method in your bucket's CORS configuration. This is a security measure to prevent unauthorized access. You can follow the code snippet in Example 2 to configure your bucket's CORS.
Here are the dependencies you'll need to install for your backend:
- @google-cloud/storage
- cors
- multer
These dependencies will help you create a robust backend for uploading files to Google Cloud Storage.
Prepare the Backend
To prepare the backend, we need to install some dependencies. We'll be using multer, a middleware for Express that helps with file uploads, and @google-cloud/storage, our client for uploading a file to Google Cloud Storage.
First, let's create our Express application. We'll then create a multer instance, which will handle the file uploads. We'll also configure our cloud storage client by providing a path to the service account key and the project ID.
To configure our cloud storage client, we need to provide the key filename, project ID, and bucket name. We'll use the Storage class from @google-cloud/storage to create a new storage instance.
A different take: Upload File to Azure Blob Storage
Here's an example of how to configure our cloud storage client:
```javascript
const cloudStorage = new Storage({
keyFilename: __dirname + '/service_account_key.json',
projectId: 'PROJECT_ID',
});
```
Next, we'll create a bucket instance using the bucketName variable. We'll then create a POST route to handle file uploads, which will use the multer middleware to handle the file uploads.
Here's an example of how to create the POST route:
```javascript
app.post('/upload-file-to-cloud-storage', multer.single('file'), (req, res, next) => {
// ...
});
```
We'll also need to configure CORS for our Express application. This will allow our frontend to make requests to our backend.
Here's an example of how to configure CORS:
```javascript
app.use(cors());
```
Finally, we'll need to start our Express server using the listen method. We'll specify the port number and a callback function that will be called when the server is listening.
Here's an example of how to start the server:
```javascript
app.listen(port, () => {
console.log(`Listening at http://localhost:${port}`);
});
```
With these steps, we've prepared our backend to handle file uploads to Google Cloud Storage.
Signed URL
A Signed URL is a URL that includes authentication information in the form of a signature. This signature is generated using a secret key and includes parameters such as the expiration time, allowing access to a particular resource or action for a limited duration.
Signed URLs are commonly used for granting temporary and secure access to resources without requiring the requester to have permanent credentials. For our use case, Signed URLs grant temporary access to upload a file to a specific location in the cloud storage bucket.
Here are the four steps involved in generating and using a Signed URL:
- Authenticate the user
- Generate the signed URL and return
- F fetch the Signed URL from frontend
- Use the Signed URL to upload the image to GCS
Using a Signed URL enhances security and control over the file upload process. Since this cloud function authenticates the users, only authorized users can use this Signed URL to upload the image to Google Cloud Bucket.
Broaden your view: Whatsapp Is Ending Complimentary Google Drive Storage for Android Users
Sources
- https://levelup.gitconnected.com/uploading-images-from-react-frontend-to-google-cloud-storage-using-firebase-cloud-functions-ee1fd5079717
- https://plainenglish.io/blog/upload-files-to-google-cloud-storage-from-react-cf839d7361a5
- https://oluwaponnle.medium.com/how-to-upload-files-to-google-cloud-storage-buckets-using-nodejs-1744f747a99f
- https://benyoss4.medium.com/image-uploading-with-google-cloud-storage-react-express-axios-8517376fb3a0
- https://dev.to/moinakh22885547/gcp-resumable-upload-with-reactjs-and-nodejs-1dd1
Featured Images: pexels.com