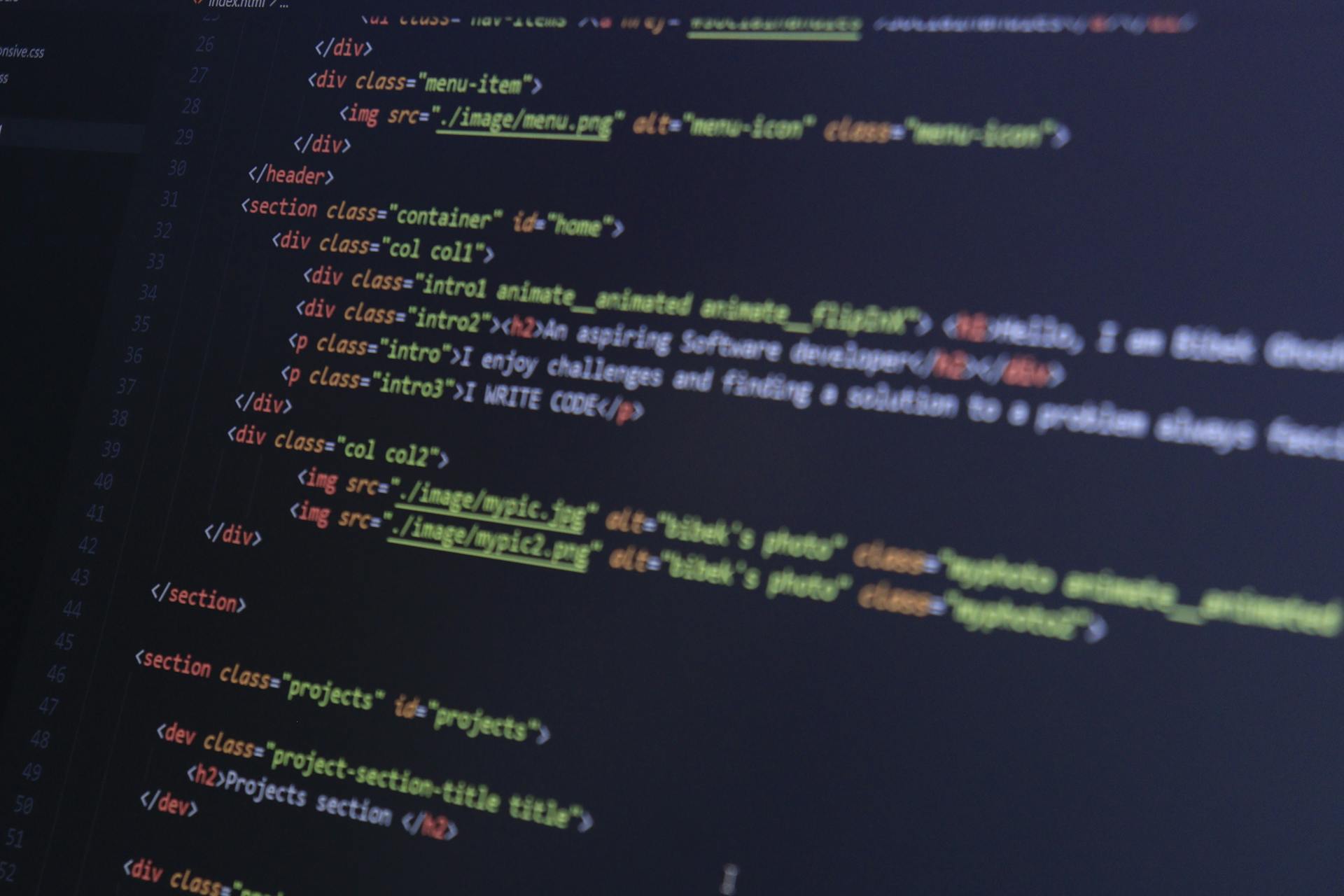
Connect RPC Nextjs is a powerful tool for building real-time applications. It enables bidirectional communication between clients and servers, allowing for seamless data exchange.
With Connect RPC Nextjs, you can handle a large number of concurrent connections efficiently. This is achieved through the use of WebSockets, which provide a low-latency communication channel between clients and servers.
By leveraging WebSockets, Connect RPC Nextjs reduces the overhead associated with traditional HTTP requests, resulting in a significant improvement in application performance. This is particularly beneficial for applications that require real-time updates, such as live updates, live scores, or live chat.
Setting Up RPC in Next.js
To set up RPC in Next.js, you'll need to install the `@rpc-nextjs` package and its dependencies.
The `@rpc-nextjs` package provides a simple way to create RPC endpoints in Next.js.
You'll need to create a new file in the `pages/api` directory, for example, `rpc.js`, to define your RPC endpoint.
In this file, you can use the `rpc` function to create a new RPC endpoint, which will be available at the `/api/rpc` route.
Here's an interesting read: New Nextjs Project Typescript
Creating a Server
Creating a server is a crucial step in setting up RPC in Next.js. To create a server, you'll need to install the `express` package, which is a popular Node.js web framework.
You can install `express` using npm by running the command `npm install express`. This will add the package to your project's dependencies.
For example, if you're using a project created with `npx create-next-app my-app`, you can install `express` in the project root directory.
Next.js provides a built-in development server that you can use to test your RPC endpoint. To use the built-in development server, you can run the command `npm run dev` in your project root directory.
This will start the development server, which you can use to test your RPC endpoint.
Related reading: Next Js Npm Install
Configuring RPC
Configuring RPC is a crucial step in setting up RPC in Next.js. You need to create a new file in the root of your project called `rpc.json` to store your RPC configuration.
In the `rpc.json` file, you'll define the RPC endpoints for your application. For example, if you want to set up a new RPC endpoint, you can add a new key-value pair to the `rpc` object, like this: `"getPosts": "/api/posts"`
The `rpc.json` file is used to map RPC methods to API routes in your Next.js application. This allows you to easily switch between different RPC methods and routes.
To enable RPC in your Next.js application, you'll need to create a new API route in your `pages/api` directory. For example, you can create a new file called `posts.js` to handle RPC requests for the `getPosts` endpoint.
In the `posts.js` file, you can use the `rpc` object to handle RPC requests and return data to the client. For example, you can use the `getPosts` method to fetch data from an external API and return it to the client.
Curious to learn more? Check out: Next Js Client Side Rendering
Server Actions and Mutations
Server Actions and Mutations are the foundation of our Connect RPC Next.js application. They define the entry points for our API.
We've already set up our server actions in the `pages/api` directory, where we created a new file called `users.js`. This file exports a single function, `createUser`, which will be called when a client makes a request to the `/api/users` endpoint.
To add a new server action, we simply create a new file in the `pages/api` directory and export a function that matches the endpoint we want to create. For example, we could create a file called `posts.js` and export a function called `createPost`.
Server mutations, on the other hand, are used to update existing data in our application. We've already seen an example of a server mutation in the `users.js` file, where we used the `updateUser` function to update a user's information.
In order to add a new server mutation, we need to create a new function in our server action file that takes the updated data as an argument. For instance, in our `users.js` file, we could add a new function called `updateUserAddress` that takes the updated address as an argument.
By following these simple steps, we can easily add new server actions and mutations to our Connect RPC Next.js application. This will allow us to create a robust and scalable API that meets the needs of our application.
Suggestion: Nextjs Server Actions File Upload
Sources
- https://firebase.google.com/docs/hosting/frameworks/nextjs
- https://nextjs.org/docs/app/building-your-application/data-fetching/server-actions-and-mutations
- https://nextjs.org/blog/next-15-rc
- https://supabase.com/blog/fetching-and-caching-supabase-data-in-next-js-server-components
- https://scastiel.dev/simplest-example-server-actions-nextjs
Featured Images: pexels.com