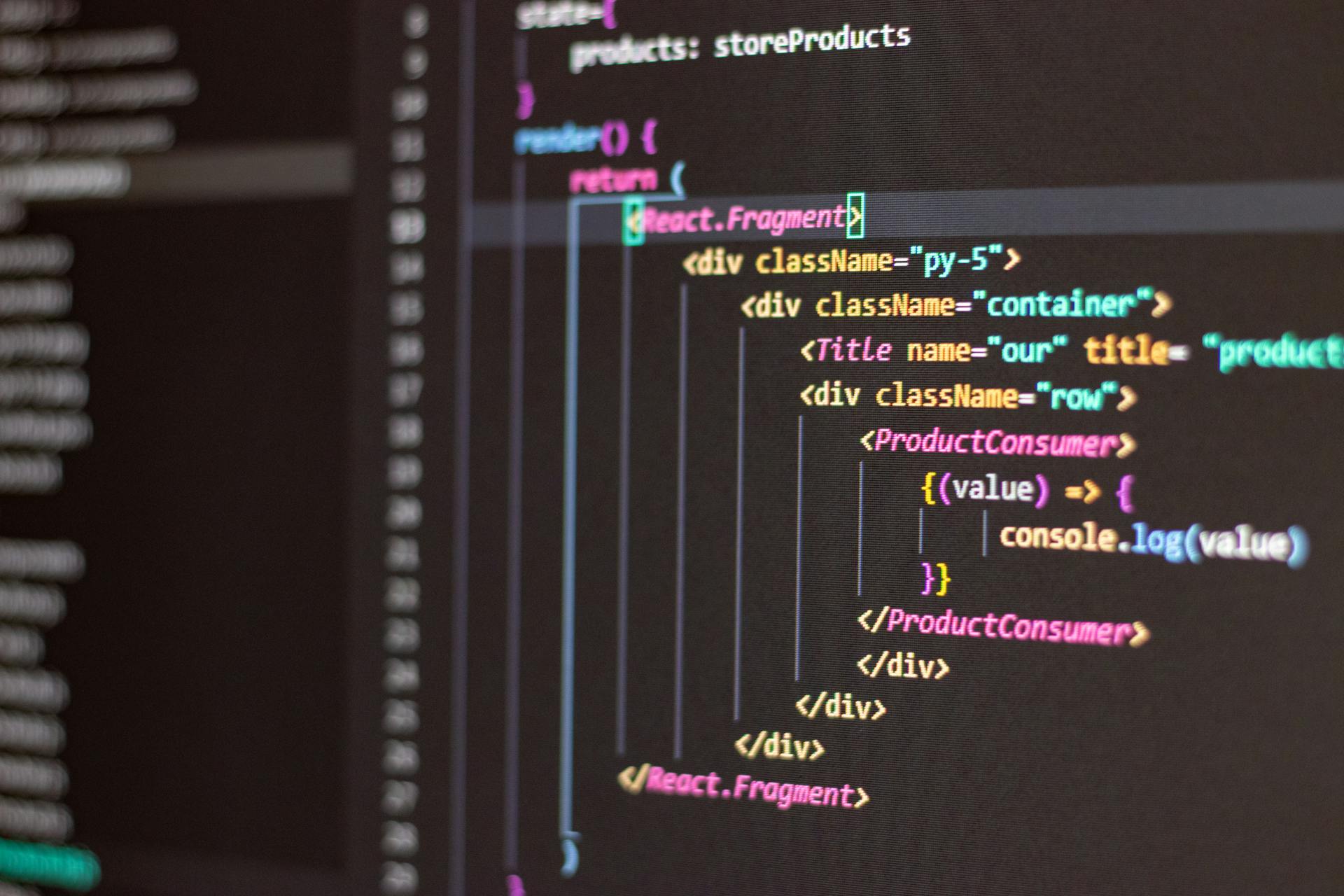
Implementing Next.js navigation in your project is easier than you think. You can start by creating a new Next.js project using the `npx create-next-app` command.
To set up client-side routing, you'll need to use the `Link` component from `next/link`. This component allows you to create links between pages without causing a full page reload.
The `Link` component works by rendering a `a` element with the `href` attribute set to the page's URL. However, it also adds some extra attributes to support client-side routing.
Next.js also provides a `useRouter` hook that allows you to access the router's context and navigate between pages programmatically.
For more insights, see: Next Js Client Portal
Fundamentals
Next.js navigation is built on top of the React Router library, which provides a simple and intuitive API for client-side routing.
The default layout in Next.js is the _app.js file, which is a special file that serves as the top-level component for your application.
In Next.js, you can use the Link component to navigate between pages, which is a replacement for the traditional anchor tag.
Expand your knowledge: Nextjs Pathname Server Component
The Link component is a wrapper around the anchor tag, and it allows you to navigate between pages without a full page reload.
Next.js uses a concept called "static site generation" to pre-render pages at build time, which improves performance and SEO.
This means that when a user navigates to a page, Next.js can serve a pre-rendered version of the page, rather than having to render it on the fly.
Next.js also provides a built-in support for internationalization (i18n) and localization (L10n), which allows you to easily support multiple languages and regions.
To enable i18n and L10n in Next.js, you need to create a directory for each language or region, and then use the next/intl module to manage the translations.
Take a look at this: Nextjs 404
Routing Basics
Routing in a Next.js app is a fundamental concept that allows users to navigate between different pages. It's a crucial part of building a user-friendly interface.
To create routes in Next.js, you can use predefined paths or dynamic routing. Dynamic routing is particularly useful for complex applications.
Explore further: Nextjs Dynamic
Here are the different types of routes you can create in Next.js:
- Route with pages
- Nested routes
- Dynamic routes
- Catch-all routes
- Link component navigation
- Programatically navigate between pages
Dynamic routes are especially useful when you need to handle complex scenarios, such as displaying a list of books or a single book's details. To create a dynamic route, you can add brackets to a page file name, like this: [bookid].js.
The Link component is another essential tool for navigation in Next.js. To use it, you first need to import it from the next/link package.
Link Component Basics
The Link component is one of the components in Next.js, used to create links between pages in a Next.js app. It's a powerful tool for client-side navigation, allowing you to control the navigation behavior with props.
To use the Link component, you need to import it from the next/link package. This is a straightforward step that gets you started with navigation in Next.js.
The Link component has a href property to specify a navigation route. This property is crucial in determining where the link will take you.
On a similar theme: Nextjs Ui Library
Here's a basic example of using the Link component:
In this example, href="/about" specifies the path we want to navigate to. This is a simple yet effective way to create links in Next.js.
The Link component is the best option to navigate to a route, but sometimes you may need to change routes programmatically. This is where the useRouter hook comes in, allowing you to access the router object and navigate to a custom URL.
Navigating Programmatically
Navigating programmatically is a key aspect of next js navigation. You can use the Link component from next js to set routes, but sometimes you need to change routes programmatically, for example, by clicking on a button.
In these cases, you can use the useRouter hook from the next/navigation package. This hook gives you access to the router object, which you can use to navigate to another route by clicking a button and pushing a new route segment to the router. To use the useRouter hook, you must declare the component as a client component by adding the 'use client' directive at the top of the page.
Check this out: Next Js Fetch Data save in Context and Next Route
Here are some key points to keep in mind when using the useRouter hook:
- The useRouter hook must be used in a client component.
- The hook gives you access to the router object, which you can use to navigate to another route.
You can also execute client-side navigations without using the Link component, but the useRouter hook is a more convenient and efficient way to handle routing needs.
Navigating Programmatically
In Next.js, you can navigate programmatically by using the useRouter hook, which gives you access to the router object. This allows you to change routes programmatically, for example, by clicking on a button.
To use the useRouter hook, you must declare the component as a client component by adding the 'use client' directive at the top of the page.
The useRouter hook can be used to navigate another route by clicking a button and pushing a new route segment to the router. For instance, clicking a button might navigate to a route like '/blog'.
If you want to access the router object inside any function component in your app, you can use the useRouter hook with the following snippet.
On a similar theme: How to Use Reducer Api in Next Js 14
Navigating programmatically is useful when you need to change routes based on user interactions, such as clicking a button or submitting a form.
Here are some ways to navigate programmatically in Next.js:
- Navigate to a new route by clicking a button and pushing a new route segment to the router.
- Use the useRouter hook to access the router object and navigate to a new route.
- Use the 'use client' directive to declare the component as a client component and use the useRouter hook.
Shallow
Shallow routing is a game-changer for altering URLs without re-running data fetching methods. This means you can update the URL without having to re-fetch data, which can be a huge time-saver.
You can enable shallow routing by setting the shallow option to true, which will allow you to modify the route's state without changing the page itself. This is especially useful for scenarios where you need to update a specific part of the URL.
The URL will be changed to /?counter=10 as a result of enabling shallow routing, and the page will remain the same. The router object, added by useRouter or withRouter, will provide the modified path name and query.
You can also use componentDidUpdate to monitor URL changes, which is a useful technique for keeping track of updates in real-time. This will allow you to react to changes in the URL and update your application accordingly.
Intriguing read: Next Js Page Transition
Scroll:
Scrolling to the top of the page can be controlled using the scroll property in Next/link. By default, it is set to true, which means the page will scroll to the top after a navigation.
The scroll property can be set to false to prevent the page from scrolling to the top. This is useful when you want to preserve the current scroll position after a navigation.
If you define a hash in your Link, it will scroll to the specific id, just like a normal a tag. To prevent this behavior, you can add scroll={false} to your Link.
Intriguing read: Next Js 14 Redirect to Another Page Loading Indicator
Sources
Featured Images: pexels.com