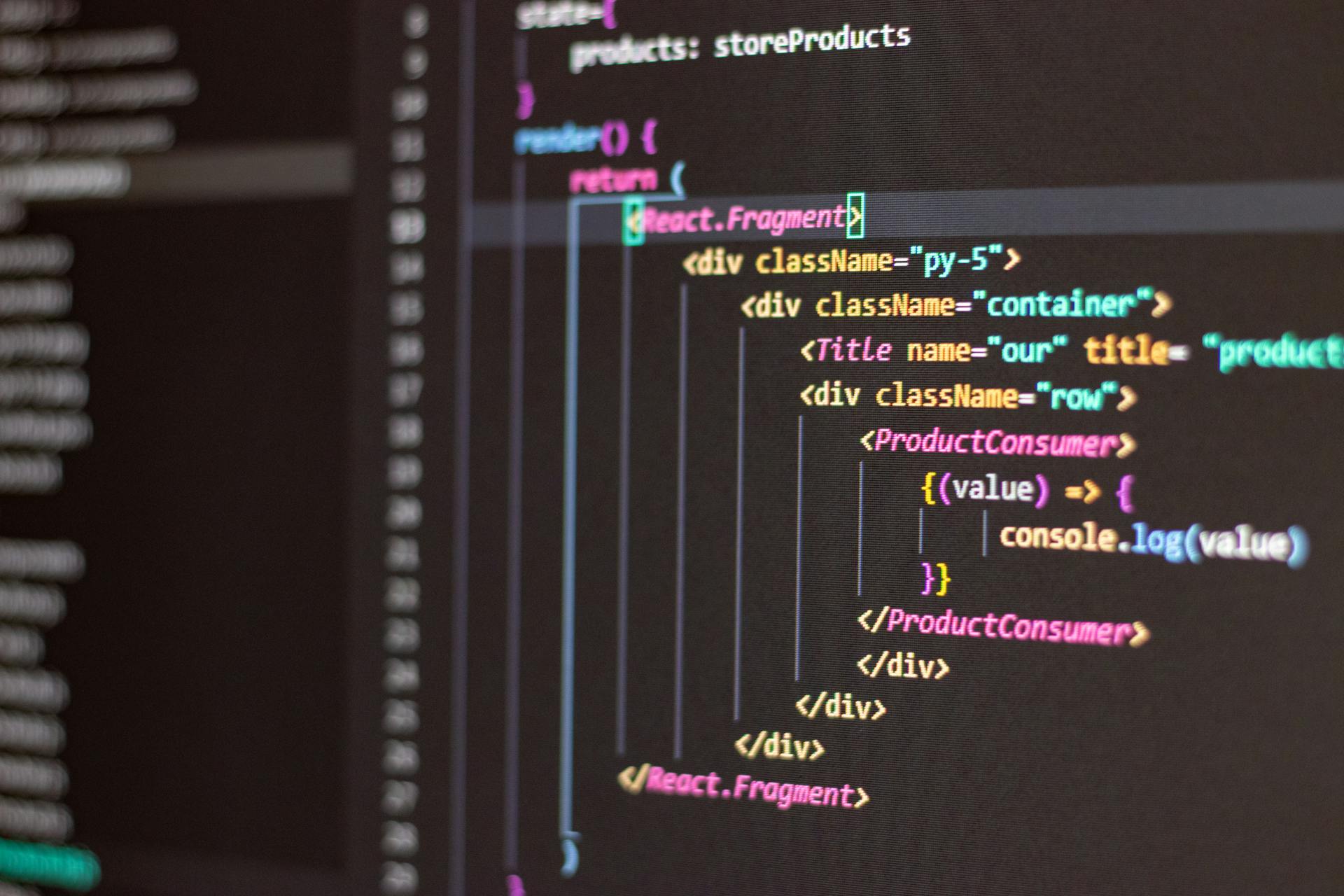
Setting up a Next.js client portal requires careful planning to ensure security and functionality.
To begin, create a new Next.js project using the command `npx create-next-app my-app`. This will set up a basic project structure for your client portal.
When it comes to authentication, Next.js provides built-in support for popular libraries like Next-Auth and Okta. These libraries can be integrated into your project to handle user authentication and authorization.
For example, Next-Auth allows you to easily add authentication to your Next.js app using popular authentication providers like Google, Facebook, and GitHub.
Recommended read: Adding Stripe to My Nextjs App
Setting Up the Project
To set up your Next.js client portal, start by creating a new Next.js application. This will be the foundation of your project.
Next, you'll need to install Storyblok's React SDK, which allows you to interact with the Storyblok API and enables real-time editing inside the Visual Editor.
Before you can start building your app, create a clone of the starter kit repo in your Git provider account. This will give you a copy of the code to work with.
Setup the Project
To set up a new Next.js application, start by creating a new one using the Next.js application. You'll also need to install Storyblok's React SDK, which allows you to interact with the Storyblok API and enables real-time editing inside the Visual Editor.
This package is essential for a seamless editing experience, and I've found it to be a game-changer for collaborative projects. With Storyblok's React SDK, you can easily manage content and make changes in real-time, without needing to restart the application.
Next.js makes it easy to get started, and with Storyblok's SDK, you'll be up and running in no time.
Readers also liked: Next Js and React
Create Git Repo
Create a clone of the starter kit repo to build your app on top of our project. This creates a copy of the code in a repo in your Git provider account.
To connect your Git provider to Vercel, click the Continue with GitHub, Continue with GitLab, or Continue with Bitbucket buttons. If you haven't already connected your provider, you'll need to do so first.
Enter a name for your repo. This name becomes the name of your project in Vercel and is also used for deploy preview URLs.
Worth a look: Next Js Provider
Authentication and Authorization
Authentication and Authorization is a crucial aspect of building a secure Next.js client portal. You can use the Auth0 Next.js SDK to implement user authentication in Next.js applications.
To get started, you'll need to install the Auth0 Next.js SDK using the command `npm install @auth0/nextjs-auth0`. This will enable you to handle user authentication on the server-side of your Next.js application.
Create a dynamic API route to handle the authentication flow by following these steps: create an `api` directory under `src/app`, then an `auth` directory under `src/app/api`, and finally a `[auth0]` directory under `src/app/api/auth`. Populate the `route.ts` file in this directory with the necessary code to handle user authentication.
The Auth0 Next.js SDK exposes a `UserProvider` component that provides a `UserContext` to its child components. You can wrap your App Router Root Layout component with `UserProvider` to integrate Auth0 with your Next.js app.
Here are the API routes that are automatically created when you run the `handleAuth()` method:
- `/api/auth/login`
- `/api/auth/callback`
- `/api/auth/logout`
- `/api/auth/me`
To configure an authentication provider for Next.js, update the `src/app/layout.tsx` file to wrap your App Router Root Layout component with `UserProvider`.
Worth a look: Nextjs Multi Tenant App
You can also render Next.js components conditionally based on the authentication status of your users. This can be done by using the `useSession` hook provided by the Auth0 Next.js SDK.
Here are the steps to add user login to Next.js:
1. Create a button that redirects users to the `/api/auth/login` API route.
2. Update the `src/app/api/auth/[auth0]/route.ts` file to customize the default login handler.
3. Use the `handleLogin()` method to override the default return path.
To add user sign-up to Next.js, follow these steps:
1. Create an API route that takes users from your Next.js application to the sign-up page.
2. Update the `src/app/api/auth/[auth0]/route.ts` file to customize the behavior of the `handleLogin()` method.
3. Use the `authorizationParams` object to specify query parameters for the call to the Auth0 `/authorize` endpoint.
Here's an example of how you can customize the login experience:
- Set the `screen_hint` query parameter to `signup` to redirect users to the sign-up page.
- Use the `handleLogin()` method to customize the default login handler and override the default return path.
By following these steps and using the Auth0 Next.js SDK, you can implement user authentication and authorization in your Next.js client portal and provide a secure and seamless user experience.
Authentication and Middleware
Authentication is a crucial aspect of any Next.js client portal, ensuring that only authorized users can access sensitive data. To achieve this, you can leverage Next.js middleware to protect specific routes.
You can create a middleware file, such as `middleware.ts`, to set up authentication. This file will contain the logic to refresh expired Auth tokens and store them using `request.cookies.set` and `response.cookies.set`. Be careful not to trust `supabase.auth.getSession()` inside server code, as it's not guaranteed to revalidate the Auth token.
To protect specific routes, you can use a matcher, such as `next { 'api/auth/[auth0]': ['auth0'], }`. This will ensure that only authenticated users can access the `/protected` and `/admin` routes. You can also customize the logout behavior by using the `handleLogout()` property in the configuration object of the `handleAuth()` method.
Related reading: Nextjs File Upload Api
Configure Authentication Provider
To configure an authentication provider for Next.js, you can use the Auth0 Next.js SDK. This SDK uses React Context to manage the authentication state of your users on the client side. You can wrap your App Router Root Layout component with the UserProvider component to integrate Auth0 with your Next.js app.
Check this out: Next Js Chat
The UserProvider component provides a UserContext to its child components, which you can use to manage the authentication state of your users. To use the UserProvider component, you need to update the src/app/layout.tsx file as follows:
You can then create a login-button.tsx file under the src/components/buttons directory to trigger the authentication flow.
Here are the steps to configure an authentication provider for Next.js:
1. Install the Auth0 Next.js SDK using npm or yarn.
2. Update the src/app/layout.tsx file to wrap the App Router Root Layout component with the UserProvider component.
3. Create a login-button.tsx file under the src/components/buttons directory to trigger the authentication flow.
By following these steps, you can configure an authentication provider for Next.js using the Auth0 Next.js SDK.
Worth a look: Can Amazon S3 Take in Nextjs File
User Profile and API Integration
In a Next.js client portal, user profile information can be retrieved on the server side using the Auth0 session. This allows you to personalize the user interface with properties such as picture, name, and email.
To do this, you need to update the src/services/profile.service.ts file to call the getSession() method, which gets the user's session from the server. If the session is not defined, you throw an error that the nearest error bounder should catch.
You can also retrieve user profile information on the client side by using the useUser() React hook, which is only available on the client side or browser. This hook allows you to get the user object and access its properties.
To use the useUser() hook, you need to add the "use client" directive at the top of the client component file, such as the src/app/profile/page.tsx file. This transforms the component from a server component to a client component.
Here are the API routes that can be used to handle the essential features of user authentication in Next.js:
- /api/auth/login
- /api/auth/callback
- /api/auth/logout
- /api/auth/me
Consume User Profile Information
You can retrieve user profile information in Next.js in two ways: on the server side or on the client side. To get server-side user profile information, you need to update the src/services/profile.service.ts file to use the getSession() method, which gets the user's session from the server.
You might like: Next Js Server Side Api Call
The getSession() method returns the user object, which you can then use to personalize the user interface. You can also display the full content of the user object within a code box.
On the client side, you can use the useUser React Hook to access the user profile information. This hook is only available on the client side or browser, so you need to add the "use client" directive at the top of the page.tsx file to transform it into a client component.
Here are the essential API routes for handling user authentication in Next.js:
- /api/auth/login
- /api/auth/callback
- /api/auth/logout
- /api/auth/me
These API routes are automatically created when you run the handleAuth() method, which is the main way to use the server-side features of the Auth0 Next.js SDK.
API Server Integration
To integrate an API server with your Next.js application, you can use Auth0 to protect your API and delegate the authorization process to a centralized service. This ensures only approved client applications can access protected resources on behalf of a user.
You can use Auth0 to get an access token in your Next.js application and use it to make API calls to protected API endpoints. This token is then passed to your API as a credential.
Your Next.js application can authenticate the user and request an access token from Auth0, which it can then use to interact with your API server. This setup allows you to test the authentication and authorization flow between the client and the server without creating an API from scratch.
To integrate your Next.js application with an API server, you can pair it with an API server that matches your technology stack. You can pick an API code sample from the Auth0 Developer Center, set it up, and then return to this page to learn how to integrate it with your Next.js application.
The AUTH0_AUDIENCE and API_SERVER_URL values represent the audience and URL of your API server, respectively.
You might like: How to Use Reducer Api in Next Js 14
Conditional Rendering and Navigation
Conditional rendering is a powerful feature in Next.js that allows you to render components conditionally based on the authentication status of your users.
To render Next.js components conditionally, you can check if the user object returned by the useUser() React Hook is defined. This allows you to render UI elements depending on the authentication state of your users.
In the desktop navigation user experience, you'll show both the login and sign-up buttons on the navigation bar when the user is not logged in. When the user is logged in, you'll show the logout button instead.
The mobile navigation experience works similarly, but the mobile menu modal hides the authentication-related buttons while closed.
You can use the isLoading value from the useUser() React Hook to render the PageLayout component once the Auth0 Next.js SDK has finished loading. This prevents the UI from flashing when the user is redirected from the Auth0 login page.
For your interest: Next Js Components Folder
To implement this, open the src/components/page-layout.tsx file and update it with the PageLoader component, which shows an animation while the SDK is loading.
Only authenticated users should see the navigation tabs to access the /protected and /admin pages. To implement this, rely on the value of the user object from the useUser() React Hook.
Logging out from your Next.js application will hide the navigation tabs for the /protected and /admin pages, and only show the tabs for the /profile and /public pages, along with the login and sign-up buttons.
Expand your knowledge: Next Js Pages
Sources
- https://supabase.com/docs/guides/getting-started/tutorials/with-nextjs
- https://nextjstemplates.com/templates
- https://www.storyblok.com/tp/add-a-headless-cms-to-next-js-in-5-minutes
- https://developer.paddle.com/build/nextjs-supabase-vercel-starter-kit
- https://developer.auth0.com/resources/guides/web-app/nextjs/basic-authentication
Featured Images: pexels.com