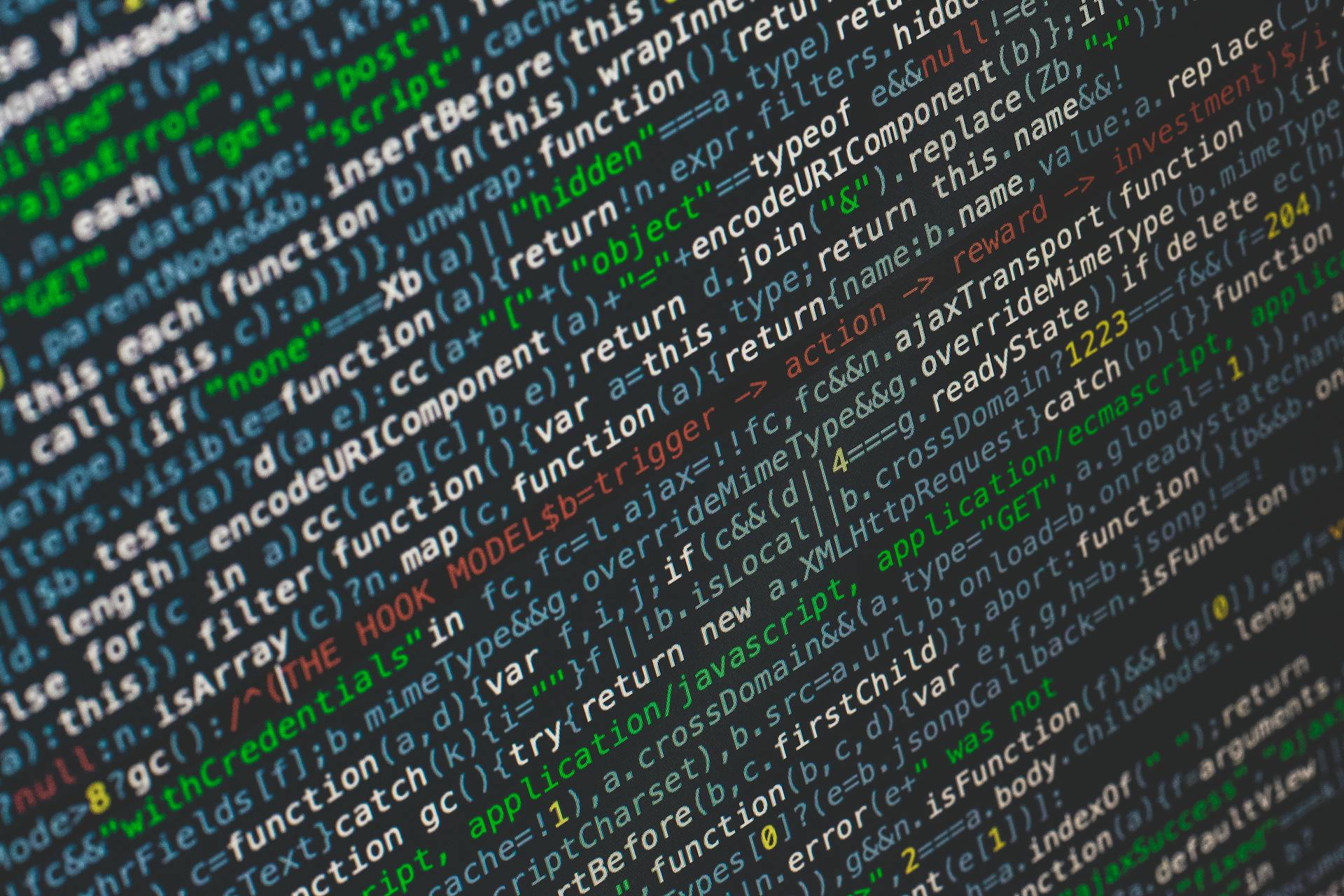
Using the Reducer API in Next JS 14 can simplify state management by allowing you to handle complex state updates in a more predictable and scalable way.
One of the key benefits of using the Reducer API is that it helps you avoid unnecessary re-renders by only updating the state when the reducer function returns a new state.
To get started with the Reducer API in Next JS 14, you need to create a reducer function that takes the current state and an action as arguments and returns a new state based on the action.
The reducer function is the heart of the state management system, and it's where you'll write the logic for updating the state in response to different actions.
Broaden your view: Next Js Using State Context
Benefits and Advantages
Using the reducer API in Next.js 14 can bring numerous benefits to your application. One of the key advantages is predictable state updates, which ensures centralized and consistent state updates.
Consider reading: Using State in Next Js
By leveraging useReducer, you can create more maintainable applications. It encapsulates complex state logic, making your codebase easier to understand and manage.
With useReducer, you can handle complex state transitions with ease. This makes it suitable for complex state management, allowing you to build robust applications.
UseReducer minimizes unnecessary re-renders, leading to performance optimization. This results in a smoother user experience and faster application loading times.
Here are the benefits of using useReducer in a concise list:
By mastering useReducer, you can create more efficient, scalable, and maintainable Next.js applications. This will save you time and effort in the long run, allowing you to focus on building innovative features and improving your users' experience.
Understanding and Setup
The useReducer hook is a powerful tool for managing state in Next.js 14. It's ideal for handling complex state logic, such as multiple states or actions.
To get started with useReducer, you'll need to define a reducer function and an initial state. The reducer function takes the current state and an action, and returns a new state. useReducer is particularly useful when you need to manage multiple states or actions.
The useReducer hook returns an array with the current state value and a dispatch function to update it. This makes it easy to manage complex state logic and keep your code organized.
Check this out: Next Js Function Handlers to Filter Data
Understanding
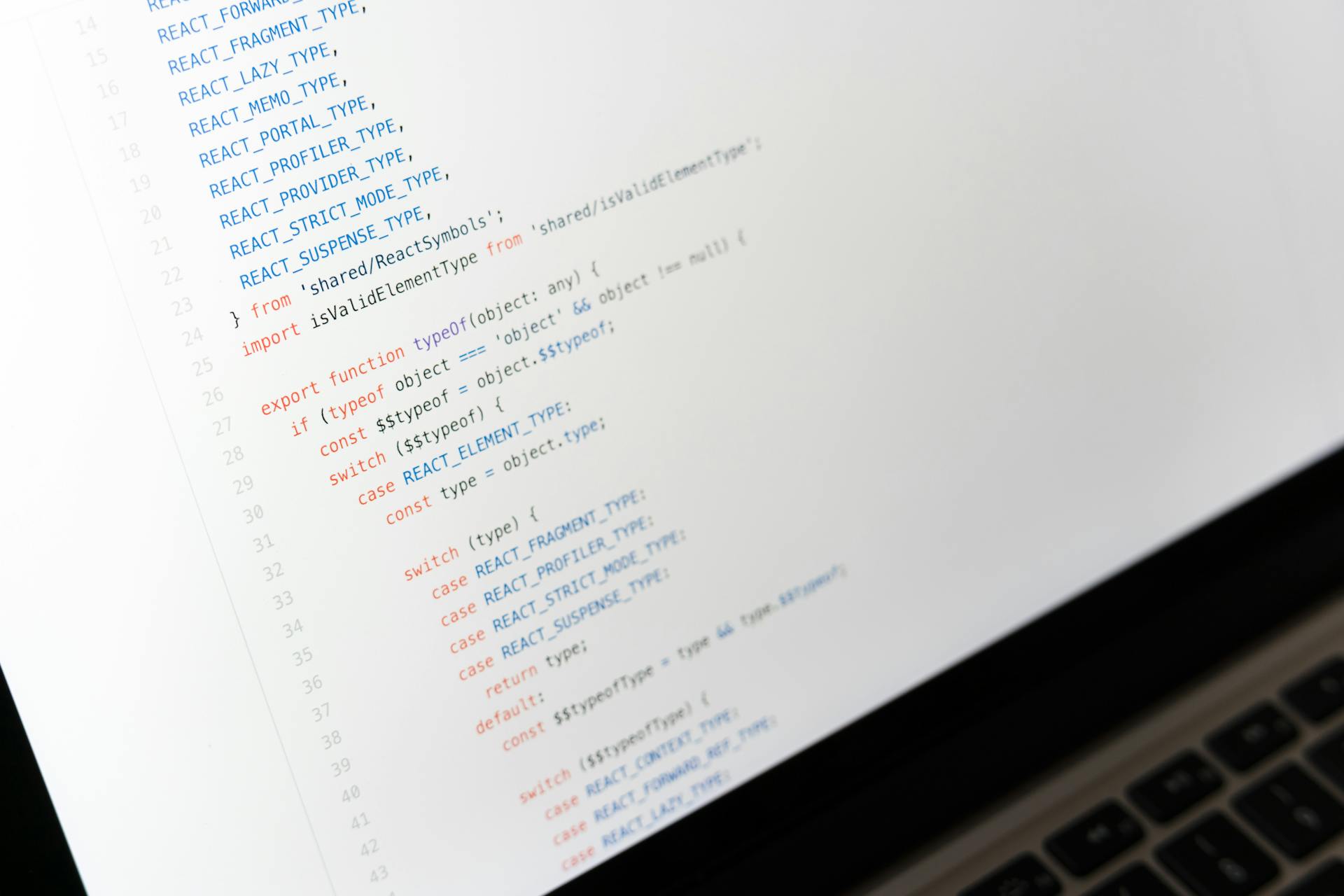
The useReducer hook is a powerful tool for managing state in React. It takes a reducer function and an initial state as arguments and returns an array with the current state value and a dispatch function to update it.
useReducer is ideal for handling complex state logic, such as managing multiple states or actions. This is particularly useful when you need to update multiple pieces of state at once.
The complexity of your state management needs is a key factor in deciding between useState and useReducer. If your needs are simple, useState might be sufficient. However, if you're dealing with complex logic, useReducer is the way to go.
useReducer is particularly useful in scenarios where you need to manage multiple states or actions. This includes situations where you have a lot of interdependent state variables.
A fresh viewpoint: Nextjs Multiple Middleware
Setting Initial
Setting Initial State is a crucial step in using the useReducer hook. The initial state is the default state of your component when it's first rendered.
The initial state should be set as follows: let's set the initial state as follows. This is where you define the starting point for your state management.
The useReducer hook returns an array with the current state value and a dispatch function to update it. The initial state should be a value that makes sense for your application, and it will be used as the starting point for your state management.
In simple terms, the initial state is like the starting point of a game - it's where everything begins.
Complex Transitions and Management
Complex transitions and management are where useReducer truly shines. It's particularly useful when dealing with complex state transitions, such as form validation or multi-step wizards.
One of the key benefits of useReducer is its ability to handle complex state transitions that depend on previous state values. This makes it easier to manage intricate data manipulation.
To understand how useReducer handles complex state transitions, consider the following rules:
By defining clear rules for state updates based on specific actions, you can ensure predictable and manageable state changes. This makes useReducer an excellent choice for complex state transitions that involve multiple related values.
Creating and Refactoring
You can refactor your component to use useReducer after creating the reducer function and setting the initial state.
By following the steps in the previous section, you can migrate from useState to useReducer and simplify your state management.
Refactoring the counter component is a great way to experience the benefits of using useReducer firsthand.
A different take: Nextjs Pathname Server Component
Creating a Function
A reducer function is a pure function that takes the current state and an action as arguments, and returns a new state. This is the first step in migrating to useReducer.
To create a reducer function, consider an example of a counter component that increments or decrements the count based on user input. A reducer function can be created as follows.
A pure reducer function is essential for optimizing useReducer performance. A pure function always returns the same output given the same inputs and has no side effects.
To write a pure reducer function, avoid mutating the state object directly. This means you should not change the existing state, but instead return a new state object with the updated values.
Here are some key principles to keep in mind when writing a pure reducer function:
- Avoid mutating the state object directly.
- Return a new state object with the updated values.
- Avoid using useCallback or useMemo inside your reducer function.
Refactoring
Refactoring is an essential part of the development process, and in this section, we'll explore how to refactor your component to use useReducer.
Refactoring with useReducer can simplify your state management and improve your application's maintainability. By following the steps outlined in the article, you can migrate from useState to useReducer and simplify your state management.
One of the key benefits of using useReducer is that it offers several advantages when managing state in NextJS applications. Here are some of the key benefits:
- Predictable state updates
- Centralized logic
- Improved maintainability
To create a reducer function, you need to write a pure function that takes the current state and an action as arguments, and returns a new state. A pure function always returns the same output given the same inputs and has no side effects.
To write a pure reducer function, avoid mutating the state object directly and return a new state object with the updated values. This ensures that your state updates are predictable and easier to debug.
See what others are reading: Nextjs 14 New Features
Refactoring your component to use useReducer can also help you encapsulate complex state logic within the reducer function, making your components more focused and maintainable. This approach enables better code organization and reusability.
By using useReducer, you can trigger state updates by dispatching actions to the reducer function. You can dispatch actions using the dispatch function returned by the useReducer hook.
See what others are reading: Next Js Typescript Example Reducer
Performance and Optimization
Minimizing unnecessary re-renders is crucial for better performance in NextJS applications, especially when dealing with large datasets or complex computations.
By using useReducer, you can optimize performance and ensure a seamless user experience in your NextJS applications. Optimizing useReducer performance is essential when using this state management approach.
UseReducer can lead to better performance by reducing unnecessary re-renders, making it a valuable tool for handling large datasets or complex computations.
Scalability
Scalability is a key aspect of building high-performance applications, and useReducer is a powerful tool that makes it easier to manage state across multiple components. By using useReducer, you can build complex applications with confidence.
One of the benefits of using useReducer is that it provides predictable state updates, which means that your application's state will always be in a consistent and centralized state. This is especially important for complex applications that require precise control over state transitions.
Here are some of the key benefits of using useReducer for scalability:
By leveraging these benefits, you can create more maintainable, efficient, and scalable applications. In fact, useReducer can help you eliminate code duplication and ensure consistent state management throughout your application.
Performance Optimization
Minimizing unnecessary re-renders is key to better performance in NextJS applications.
UseReducer can lead to significant performance improvements, especially when dealing with large datasets or complex computations.
Unnecessary re-renders can cause a significant slowdown in your application, making it essential to optimize useReducer performance.
Optimizing useReducer performance is crucial to ensure a seamless user experience in NextJS applications.
By following best practices and tips, you can significantly improve the performance of your useReducer in NextJS.
Best Practices and Guidelines
To get the most out of the Reducer API in Next.js 14, it's essential to follow best practices and guidelines.
Use the `useReducer` hook to manage complex state changes, as it provides a more predictable and efficient way to handle state updates.
When dealing with nested data, use the `immer` library to simplify state updates and make your code more maintainable.
Always pass an initial state to the `useReducer` hook to ensure your application starts with a clean slate.
Use the `dispatch` function to trigger state updates, and make sure to handle any potential errors that may occur.
In Next.js 14, you can use the `useReducer` hook in conjunction with the `useEffect` hook to handle side effects and optimize your application's performance.
Keep your reducer functions pure and free of side effects to ensure that your application remains predictable and efficient.
Use the `useReducer` hook in combination with other hooks, like `useState` and `useContext`, to create a robust and scalable application architecture.
A unique perspective: Next Js Context
Migration and Integration
Migrating to useReducer can simplify your state management and improve your application's maintainability.
You can migrate from useState to useReducer with a clear understanding of the process. This involves breaking down complex state logic into smaller, more manageable pieces.
Migrating to useReducer can seem overwhelming, but with a step-by-step guide, you can make the process smoother. This guide will walk you through the process of migrating to useReducer.
Breaking down complex state logic into smaller pieces can make your codebase more maintainable. This is especially true when dealing with complex state management scenarios.
By using useReducer, you can improve the maintainability of your application and make it easier to debug and test. This is a key benefit of using the Reducer API in Next.js 14.
For more insights, see: Can Nextjs Be Used on Traditional Web Application
Managing Async Operations
Managing async operations with useReducer is a bit tricky, but don't worry, I've got you covered.
Use the useReducer hook to manage state in your async operation handlers. This is a key best practice to keep in mind.
Related reading: Next Js 14 Layout Async
Avoid using useState or other state management hooks to update state in async operation handlers. This can lead to unpredictable behavior and bugs.
Use the dispatch function provided by useReducer to update state in a centralized manner. This is the most efficient way to manage state changes in async operations.
Here's a quick rundown of the best practices to keep in mind:
- Use the useReducer hook to manage state in async operation handlers.
- Avoid using useState or other state management hooks to update state in async operation handlers.
- Use the dispatch function provided by useReducer to update state in a centralized manner.
Sources
- https://nextjsstarter.com/blog/usestate-to-usereducer-nextjs-state-management/
- https://blog.logrocket.com/guide-state-management-next-js/
- https://focusreactive.com/breaking-down-next-js-14/
- https://nextjs.org/docs/app/building-your-application/data-fetching/server-actions-and-mutations
- https://blog.greenroots.info/understanding-nextjs-server-actions-with-examples
Featured Images: pexels.com