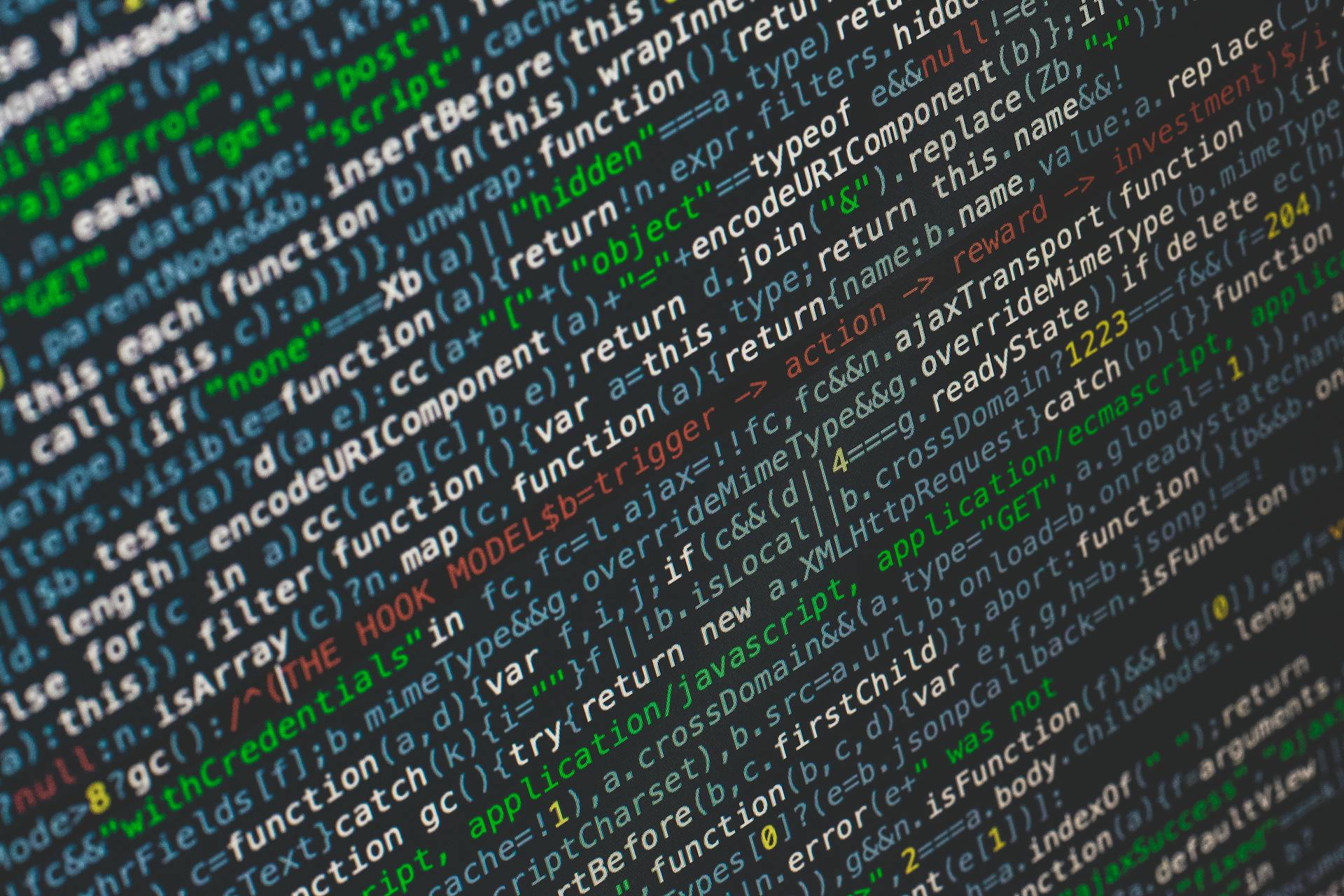
In Next.js 14, local storage is a powerful tool for storing small amounts of data in the browser. This can be particularly useful for caching data that doesn't change frequently.
Next.js 14 introduces a new API called `useSessionStorage` which allows you to store data in session storage instead of local storage. This is useful for storing sensitive data that needs to be cleared when the user closes their browser.
To use local storage effectively, it's essential to understand its limitations. Local storage has a maximum storage capacity of 5MB, which can be exceeded if you store large amounts of data.
By using local storage strategically, you can improve the performance and user experience of your Next.js 14 application.
See what others are reading: Nextjs App Route Get Ssr Data
Storage Basics
LocalStorage is a way to store data locally on a user's browser, allowing us to save and retrieve data even when the user closes their browser or comes back later. It's a simple and effective way to persist data.
Next.js 14 apps can use LocalStorage to store data, making it a great tool for storing user preferences, settings, or other types of data that don't need to be synced with a server.
Custom Storage Solutions
Custom Storage Solutions can make our app interactions smoother by reusing logic across the app.
Creating a custom hook is a great way to achieve this, and it's as simple as using a custom hook like `useLocalStorage`.
This hook can be reused in various parts of our app, making it easier to manage LocalStorage interactions.
Related reading: Next Js Custom Server
Advanced Storage Techniques
Advanced Storage Techniques can be a game-changer for your Next.js 14 app.
Batching operations is a great way to improve performance, and it's as simple as updating a single object instead of individual items.
Batching can be especially helpful if you're doing a lot of read/write operations with LocalStorage.
By batching operations, you can reduce the number of requests made to LocalStorage, which can lead to faster page loads and a better user experience.
Consider using advanced LocalStorage techniques to level up your storage game.
LocalStorage can be used to store complex data structures, such as objects and arrays, which can be updated in bulk using batching.
For another approach, see: Next Js Using State Context
Error Handling and Troubleshooting
You might run into some issues when working with LocalStorage in Next.js, but don't worry, they're easily solvable.
Even with careful implementation, you might encounter common problems like the "localStorage is not defined" error, which usually happens when you're trying to access LocalStorage during server-side rendering.
To fix this, simply wrap your LocalStorage calls in a check to ensure it's defined before using it.
Troubleshooting Common Issues
You're likely to encounter some common issues when working with LocalStorage in Next.js, but don't worry, I've got you covered.
Even with careful implementation, you might run into some hiccups.
You can troubleshoot these issues by checking for a few common errors.
One common error is the "localStorage is not defined" error, which usually happens when you're trying to access LocalStorage during server-side rendering.
To fix this, you can wrap your LocalStorage calls in a check.
Additional reading: Nextjs Error Page
Unexpected Data Types
Unexpected Data Types can be frustrating, especially when you're expecting a specific type of data. This issue usually arises because LocalStorage stores everything as strings, so you need to make sure you're parsing your data correctly when retrieving it.
For instance, if you're trying to access a number that's stored as a string, you'll get unexpected results. Make sure to use JSON.parse() to convert the string back into its original data type.
If you're dealing with complex data, remember that LocalStorage stores everything as strings, so you'll need to use JSON.stringify() when setting items and JSON.parse() when getting them. This will ensure you're working with the correct data type.
Suggestion: Nextjs Json Parse
Performance Considerations
Using LocalStorage in Next.js can be a bit tricky, but it's essential to keep your app running smoothly. LocalStorage is super handy, but it's not a magic solution.
To avoid performance issues, you need to use LocalStorage wisely. It's not a good idea to store too much data in LocalStorage, as it can slow down your app.
In Next.js, you can use the `useEffect` hook to optimize LocalStorage usage. This hook allows you to run a function after a component has rendered, which is perfect for cleaning up LocalStorage.
LocalStorage can be a great way to store small amounts of data, like user preferences or settings. Just remember to keep it small and only store what's necessary.
Event Handling and Client-Side
Event handling is a crucial aspect of building a seamless user experience in Next.js 14. LocalStorage can trigger events, allowing different parts of your app to react to changes.
This is super useful, as it enables you to keep your app in sync with the user's preferences and settings.
Handling Events
Handling events is a crucial aspect of client-side development. LocalStorage can trigger events, which is super useful when you want different parts of your app to react to LocalStorage changes.
This allows you to keep your code organized and focused on one task at a time.
In fact, LocalStorage events can be handled in a similar way to other events in JavaScript.
The Client-Side Catch
LocalStorage is a browser API that only exists on the client-side, which means it's not available on the server. This can cause issues in Next.js, especially with server-side rendering.
You need to be careful about when and where you use LocalStorage, as it can lead to "hydration mismatch" errors if used in a component that's rendered on the server.
To use LocalStorage safely in Next.js, you should wrap it in a useEffect hook to ensure it only runs in the browser. This is crucial for preventing hydration mismatch errors.
In Next.js, server-side rendering is the default, so you need to consider this when using LocalStorage.
Explore further: Nextjs Rendering
The Component
In this component, TailwindCSS is used to enable and disable dark mode through a class, making it simple to create a dark mode.
TailwindCSS handles enabling and disabling all classes with the "dark:" prefix, which simplifies the process.
The component uses conditional rendering to display the icon for the current theme at the bottom.
A helper function is created to get the opposite theme, which is then used to update the state when the toggle is clicked.
The toggle switch is connected to the `setStoredMode` function, which updates the state with the opposite theme when clicked.
The component loads the saved value from Local Storage when the page is refreshed.
The state value stays in sync with Local Storage, allowing the component to initialize with the stored value.
Related reading: Next Js Strict Mode
Frequently Asked Questions
How to get LocalStorage value in Next.js 14?
To access LocalStorage in Next.js, use the `useLocalStorage` hook with the desired key and initial value, ensuring it's safely implemented for both client and server-side rendering. This hook provides a reliable way to retrieve and store data in LocalStorage.
Can I use LocalStorage in SSR?
Yes, you can use LocalStorage in Server-Side Rendering (SSR), but you'll need to use Pinia's persisted state plugin or create a custom storage driver to ensure reactivity.
Sources
- https://nextjs.org/docs/app/building-your-application/routing/middleware
- https://upmostly.com/next-js/using-localstorage-in-next-js
- https://bigcodenerd.org/blog/localstorage-nextjs-14/
- https://articles.wesionary.team/securing-sensitive-data-in-a-next-js-application-d7d5cce67f23
- https://nextjs.org/blog/next-14
Featured Images: pexels.com