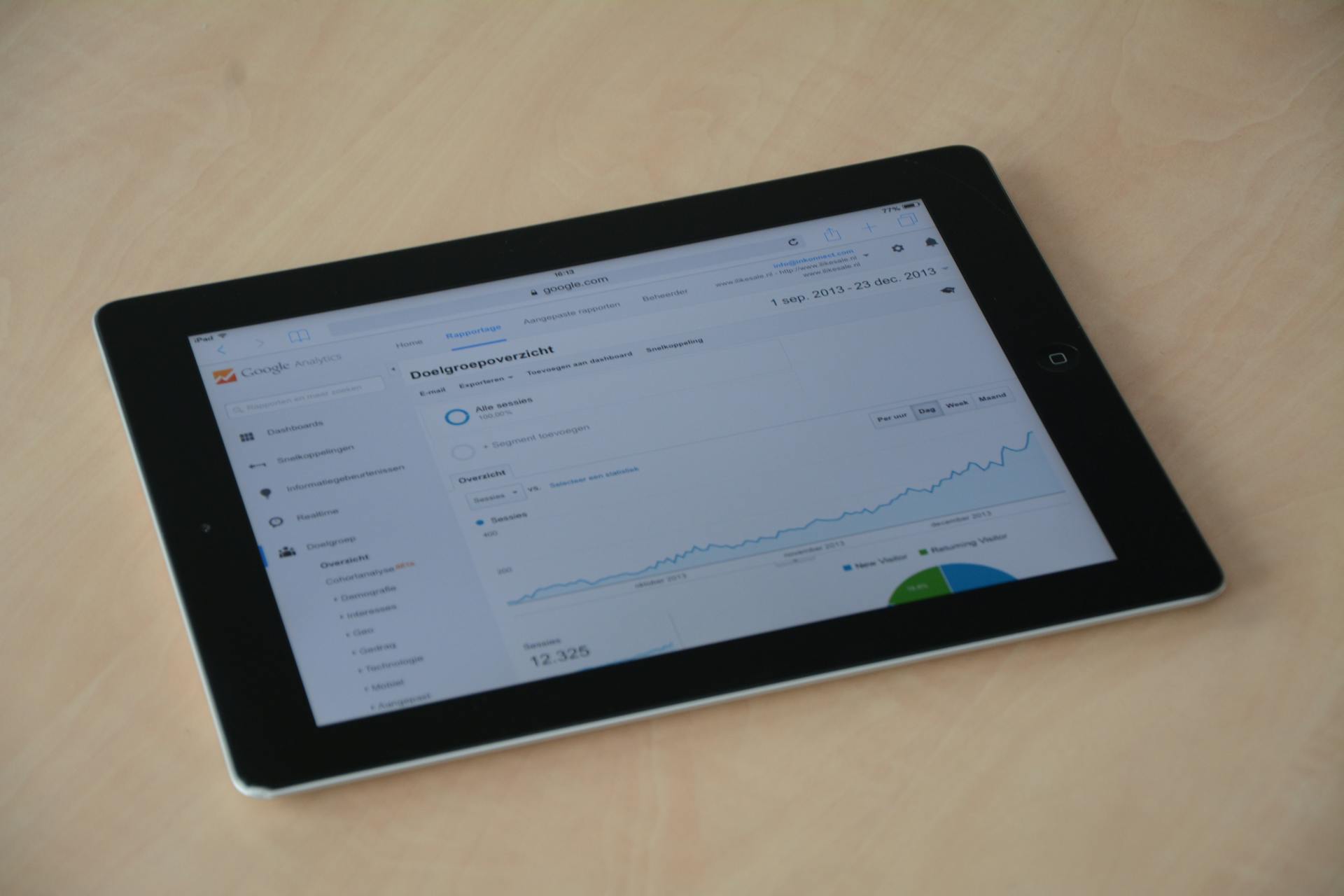
Nextjs 14 has just been released, and it's packed with exciting new features and upgrades. One of the most notable changes is the introduction of Next.js 14's new router.
Next.js 14's new router is built on top of the Edge Runtime and allows for more efficient and scalable routing. This is a game-changer for developers who work with complex routing systems.
With Next.js 14, you can now easily handle client-side routing and server-side rendering in a single application. This makes it easier to build fast and scalable web applications.
Next.js 14 also includes a new feature called "app directory" which allows you to organize your application's code in a more modular way. This feature is particularly useful for large-scale applications.
Broaden your view: Next Js Single Page Application
Routing and Navigation
Routing and Navigation in Next.js 14 is a game-changer. The framework introduces a redesigned routing system that's simpler, yet more powerful than ever before.
This new system is based on specialized files and directory naming conventions, allowing developers to structure their applications in a hierarchical manner that reflects the UI and navigational flow. You can create Route Groups by enclosing directories in braces (...), which simplifies the application's structure and applies a single set of configurations to all routes within the group.
Intriguing read: Next Js Project Structure
Route Groups can be defined by directories enclosed in parentheses (...), allowing developers to apply a common set of layouts or data fetching logic to all routes within the group. This is especially useful for managing complex routing needs.
Here's a breakdown of the specialized files and what they accomplish:
- page.tsx defines a page component, where each page.tsx file corresponds to a route
- layout.ts specifies a layout component that wraps around the page component, useful for defining common UI elements like headers and footers across different pages
- template.tsx similar to layout.tsx, but doesn’t persist state between navigations
- loading.tsx defines a loading component displayed during data fetching or when navigating between pages
- error.tsx custom error component for handling errors within the application
- not-found.tsx specifically for 404 pages, allowing developers to create custom "Page Not Found" responses
- middleware.ts for defining middleware that can run before rendering a page, useful for authentication, redirects, and more
- route.ts manages custom request handling for routes, including fetching and mutations
- default.tsx a fallback component for when no specific page or layout is matched, ensuring a graceful handling of unexpected navigation scenarios
- instrumentation.ts used for monitoring and measuring performance metrics or other custom logging for a route
With Next.js 14, you can also create Dynamic Routes, which allow for the creation of routes that adapt based on the data passed to them, such as user IDs or product names. Enclosed in brackets [...], dynamic routes enable variable paths and parameters, offering flexibility in how applications handle data-driven navigation and rendering.
Take a look at this: Nextjs Dynamic
Routing
Routing is a crucial aspect of building web applications, and Next.js has made significant improvements in this area. The framework introduces a redesigned routing system that provides increased flexibility, better organization, and more control over the routing behavior of your applications.
The new routing system is based on specialized files and directory naming conventions. You can define a page component in a file named `page.tsx`, which corresponds to a route. Layout components can be defined in `layout.ts` files, which wrap around the page component and include common UI elements like headers and footers.
Here's an interesting read: Next Js Cms
The routing system also supports dynamic, parallel, and intercepting routes. Dynamic routes are defined using brackets `[]`, parallel routes use the `@` symbol, and intercepting routes use parentheses `()`. These features enable the creation of complex routing structures and improve the overall user experience.
Here's a breakdown of the specialized files and what they accomplish:
- `page.tsx`: defines a page component
- `layout.ts`: specifies a layout component
- `template.tsx`: similar to `layout.ts`, but doesn’t persist state between navigations
- `loading.tsx`: defines a loading component
- `error.tsx`: custom error component
- `not-found.tsx`: specifically for 404 pages
- `middleware.ts`: for defining middleware that can run before rendering a page
- `route.ts`: manages custom request handling for routes
- `default.tsx`: a fallback component for when no specific page or layout is matched
- `instrumentation.ts`: used for monitoring and measuring performance metrics or other custom logging for a route
Route Groups are a new feature in Next.js that allow you to group routes under a shared configuration. This is accomplished by enclosing directories in braces `{}`, creating a logical grouping of routes that share common attributes or behaviors. This organization simplifies the application's structure and enhances maintainability and consistency across similar routes.
Parallel Routes enable the simultaneous loading of components, which is particularly useful for applications requiring multiple components to be rendered independently but within the same page layout. By creating a directory with a parallel syntax, developers can structure their applications to load components in parallel.
Explore further: Next Js App Router Project Structure
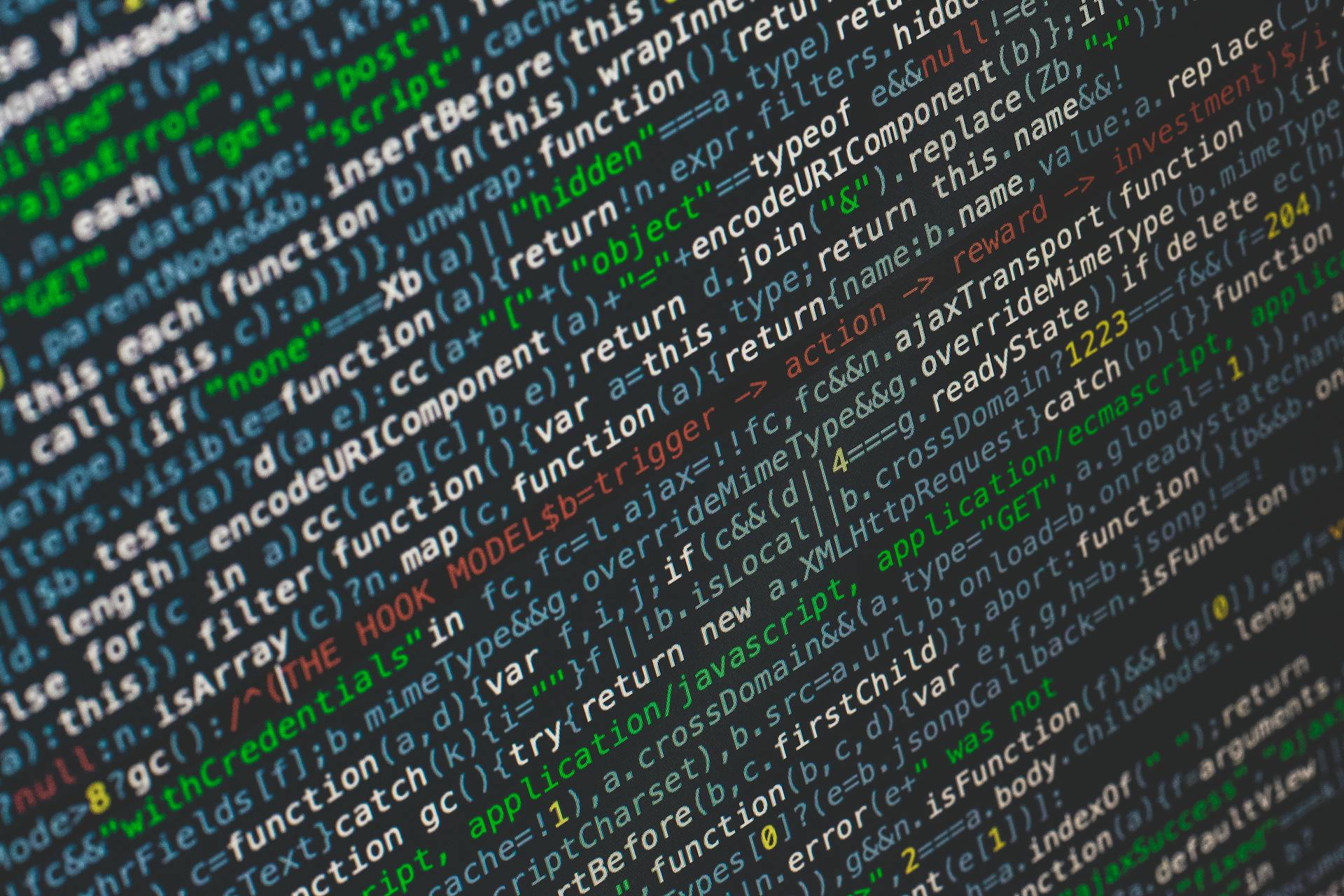
Intercepting Routes offer a sophisticated mechanism for managing route transitions within an application, particularly when aiming to maintain the current layout or context. This feature enables the dynamic loading of content from different parts of the application into the current view, without necessitating a full context switch for the user.
Here's a summary of the routing features in Next.js:
By leveraging these features, you can create more complex and dynamic routing structures in your Next.js applications.
Router Cache
The Router Cache in Next.js is a game-changer for smooth navigation. It caches routing information and component states, ensuring that navigating back to previously visited pages is instant.
This caching strategy eliminates the need for re-rendering components or re-fetching data, making Next.js applications feel more responsive and app-like.
The Router Cache works by storing the state of components and routing information, so when you navigate back to a page, it can quickly restore the page from the cache. This results in faster navigation and a more seamless user experience.
Pages and Layouts
In Next.js 14, Pages and Layouts form the backbone of the routing system. The page.tsx file represents the entry point for a route, rendering the main content.
With the introduction of a new caching system, data fetching has become more efficient, eliminating the concern of request waterfalls. fetch() calls are now cached, allowing for data requests at both the page and layout levels without duplicating network requests.
page.tsx and layout.tsx are essential for any Next.js project, acting as the main files that determine the structure and behavior of pages and their surrounding layouts.
Imagine an application with a project management board where clicking on a task within a project overlays task details without leaving the project context. This can be achieved with Intercepting Routes.
The page component, page.tsx, is the heart of any page within a Next.js application, where the rendering logic begins and components come together to form the page's content.
Additional reading: Nextjs Rendering
Data Fetching
Data fetching is a fundamental aspect of building interactive and dynamic web applications. Next.js 14 enhances the experience of fetching, caching, and revalidating data, aligning closely with React's architectural advancements.
You can fetch data on the server side using the native fetch() function, which simplifies how dynamic data is fetched and used within components. This approach ensures that data fetching logic is colocated with the component that requires the data, promoting better data encapsulation and component reusability.
Next.js extends the capabilities of the traditional fetch API, allowing for configurable caching and revalidation strategies. This integration simplifies the data fetching process within React's component model and significantly improves performance by reducing unnecessary network requests and leveraging smart caching techniques.
You can fetch data on the client side using Route Handlers, which enable the execution of data fetching logic on the server, with the results seamlessly passed back to the client. This mechanism allows for secure data fetching patterns, where sensitive operations are kept on the server, away from the client's reach.
For your interest: Using State in Next Js
The data cache feature in Next.js allows developers to cache the results of data fetching operations at the application level. This speeds up data retrieval and minimizes redundant data fetching operations, contributing to overall application efficiency.
Here are some key benefits of data fetching in Next.js 14:
- Configurable caching and revalidation strategies
- Simplified data fetching process within React's component model
- Improved performance through reduced network requests and smart caching
- Secure data fetching patterns through Route Handlers
- Efficient data retrieval through data caching
Faster Builds and Improved Performance
Next.js 14 is a game-changer for developers, especially when it comes to faster builds and improved performance.
Faster incremental builds are now a reality, thanks to Next.js 14, reducing build times and boosting developer productivity. This is a huge win for large-scale applications that require frequent updates and deployments.
With Next.js 14, you can expect faster local server startup times and code updates, making development a more efficient and enjoyable process.
Turbopack, the innovative compiler introduced in Next.js 14, is built on the Rust programming language and promises to revolutionize the development process with faster build times and code updates.
Turbopack is a substantial leap forward in local development performance, providing tangible speed and reliability benefits.
Additional reading: Nextjs Code Block
Server and Client Components
Server and Client Components are a crucial part of Next.js 14, allowing you to create interactive applications that require data to be fetched and manipulated on both the server and client sides.
To designate a component as a Client Component, you add the "use client" directive at the top of the file, ensuring that it and its dependencies are bundled for client-side execution. This directive serves as a boundary between Server and Client Component modules.
You can fetch data on the client while keeping server-side execution for data retrieval using Route Handlers, which are server-side functions that can be called from client components. Route Handlers execute on the server, fetching data or performing other server-side logic, and then return the data to the client component that initiated the call.
Server Actions and Mutations allow you to define functions that can perform data modifications on the server side, directly from client-side events. These actions are defined in Server Components and can be invoked from Client Components, providing a seamless integration between the client and server.
For more insights, see: Nextjs Server Actions File Upload
Here are some key features of Server Actions:
- Revalidate cached data with revalidatePath() or revalidateTag().
- Redirect to different routes using redirect().
- Set and read cookies through cookies().
- Handle optimistic UI updates with useOptimistic().
- Capture and display server errors with useFormState().
- Show loading states on the client with useFormStatus().
You can use the fetch() function within Server Components to request data from APIs or other data sources during the server rendering process, ensuring that data fetching logic is colocated with the component that requires the data.
A different take: Nextjs App Route Get Ssr Data
Handling Unmatched Slots
Handling Unmatched Slots can be a challenge in Server and Client Components, but there's a solution. You can define a default.tsx file within the /app/admin directory to serve as a fallback for unmatched slots during the initial load or full-page reload scenarios.
This fallback ensures that a meaningful default view is always presented to the user, enhancing the application's usability and navigation experience.
Server Components
Server Components are a game-changer in Next.js 14. They're now the default way to render components, unless explicitly defined otherwise.
Server Components are rendered on the server and don't include any JavaScript that runs in the browser. This approach is optimal for delivering static content, accessing server-side resources, and performing tasks that don't require client-side interactivity.
Additional reading: Next Js Client Side Rendering
To create a Server Component, you simply need to define it as such, either by adding the "use server" directive or by placing it in a file that doesn't have the "use client" directive. This allows you to leverage the server's capabilities for initial rendering, while still allowing for dynamic client-side interactions through Client Components.
You can use Server Components to fetch data on the server side, using the native fetch() function. This approach ensures that data fetching logic is colocated with the component that requires the data, promoting better data encapsulation and component reusability.
Here are some key benefits of using Server Components:
- Optimized performance by leveraging the server's capabilities for initial rendering
- Improved developer experience by simplifying how dynamic data is fetched and used within components
- Reduced page bundle size by not sending JavaScript to the client
- Better data encapsulation and component reusability by colocating data fetching logic with the component
By using Server Components, you can create more efficient and scalable applications that take full advantage of the server's capabilities.
Frequently Asked Questions
Is next 14 production ready?
Yes, Next.js 14 is production-ready, thanks to its enhancements in routing, caching, and bundling. It's now a top choice for building robust and efficient React applications.
What is the difference between Next.js 13 and Next.js 14?
Next.js 14 builds upon the Incremental Static Regeneration (ISR) feature introduced in Next.js 13, taking it to the next level with on-demand ISR support for even faster and more efficient updates. This upgrade enables developers to update static pages even more efficiently.
What is the difference between Next.js 14 and 15?
Next.js 14 and 15 differ in their default caching behavior for GET HTTP methods, with 14 caching them by default and 15 not caching them by default. However, you can still opt into caching in 15 using a static route config option.
Featured Images: pexels.com