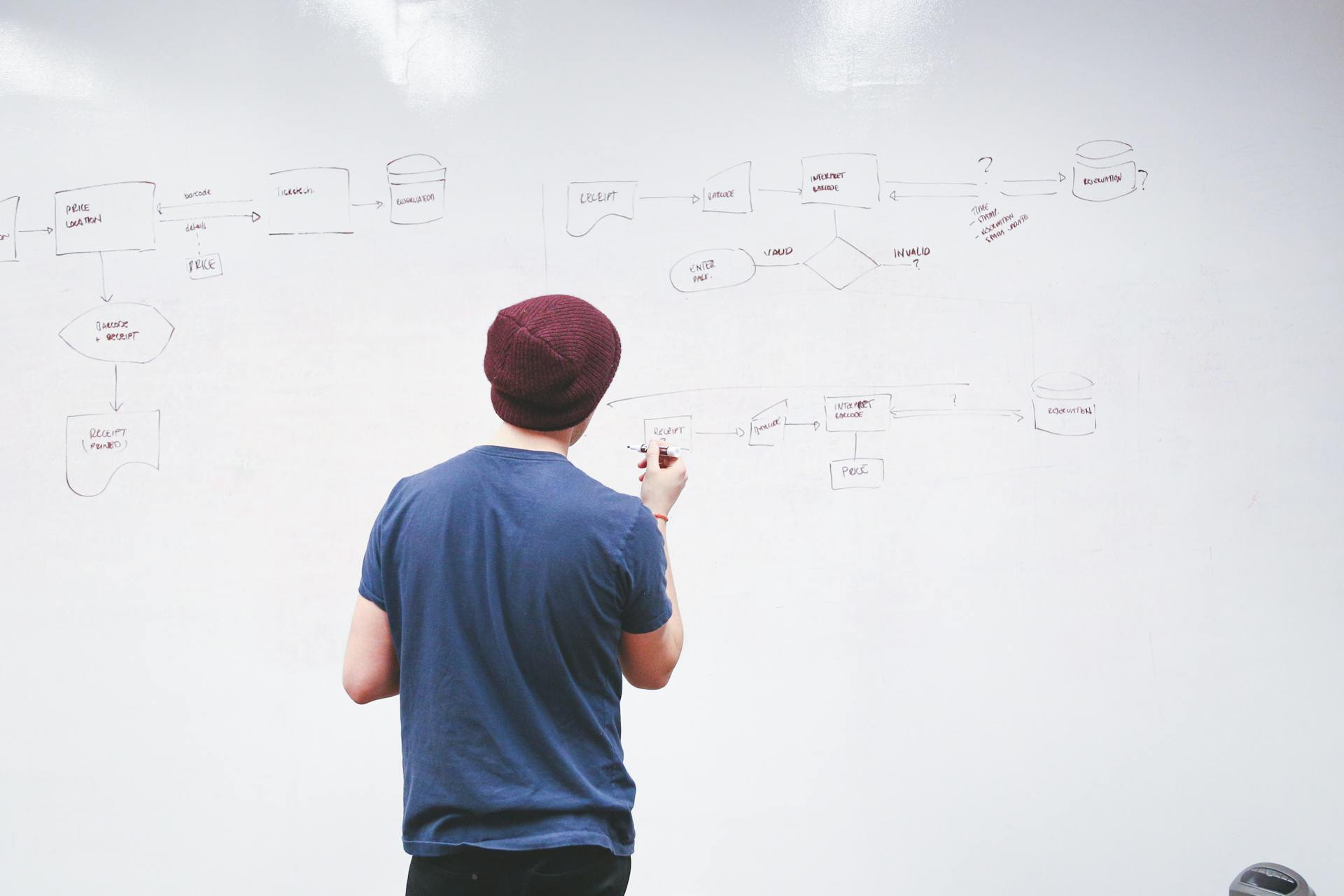
As a beginner, setting up a Next JS App Router project structure can seem daunting, but it's actually quite straightforward. You can create a new Next JS project using the `npx create-next-app` command.
The basic structure of a Next JS App Router project includes the `app` directory, which contains the main application files. This directory is where you'll find the `router` and `pages` directories.
In a Next JS App Router project, the `router` directory is used to define routes for the application. You can create a new route by adding a new file to this directory.
The `pages` directory is where you'll find the individual pages of your application. Each page is represented by a separate file, and you can use the `getStaticProps` method to fetch data for each page.
A unique perspective: Nextjs Client Side Cookies
Directories and Files
The app directory is the heart of your Next.js project, and understanding its structure is key to building a robust application. The app directory contains all the routes and layouts leveraging the App Router.
For your interest: Src Directory Nextjs
By default, the app directory has a specific file structure. The page.js file serves as a route or path definition, taking its name from the folder within which it resides. This means that if you place a page.js file in the root (app) directory, it maps to the homepage (/) of the website.
The layout.js file defines the layout for a particular route if placed in a folder, but when placed in the root (app) directory, it becomes the layout for the entire web application. This means that all descendant routes inherit the layout specified in this file.
Here's a breakdown of the key directories and files you'll find in the app directory:
- app/: Contains all the routes and layouts leveraging the App Router.
- components/: Reusable UI components.
- styles/: Global and component-specific styles.
- public/: Static assets like images, fonts, etc.
- lib/: Utility functions and libraries.
- hooks/: Custom React hooks.
- tests/: Test suites for various parts of the application.
To create specific routes, such as /blog, create a folder named blog within the app directory. Inside this folder, include a page.js file that exports a function component. This will allow you to create a custom layout for your blog page.
Take a look at this: Next Js Project
Routing Fundamentals
Routing Fundamentals are crucial in Next.js App Router project structure. The App Router is a new routing system in Next.js that allows for more flexibility and customization.
A key concept in App Router is the use of pages as routes. This means that each page in your application can be a route, and you can use the `use` directive to define routes for your pages.
The `use` directive is used to define routes for your pages, and it takes a path as an argument. The path is used to match URLs and determine which page to render.
In the App Router, routes are defined at the top level of your application. This is different from the traditional Next.js routing system, where routes were defined in a separate file called `pages/_app.js`.
The `use` directive can be used to define routes for individual pages, or for groups of pages. For example, you can use it to define a route for all pages in a specific directory.
For another approach, see: How to Use Loading in Next Js
The App Router also supports route parameters, which allow you to capture values from the URL and pass them to your page components. This is done using the `$` symbol in the path.
Route parameters can be used to customize the behavior of your page components based on the values passed from the URL. This is a powerful feature that allows you to create more dynamic and interactive applications.
In the App Router, route parameters are defined using the `$` symbol in the path, and they are passed to your page components as props. This allows you to access the values of the route parameters in your page components.
The App Router also supports nested routes, which allow you to define routes for subdirectories. This is done using the `use` directive with a nested path.
Nested routes are useful for creating complex applications with multiple levels of navigation. They allow you to define routes for subdirectories and still maintain a flat structure in your page components.
Intriguing read: Using State in Next Js
Page Organization
Organizing your pages and routes effectively is crucial for a Next.js app router project. This means structuring your directory to mirror your URL structure.
A file-based routing system is encouraged by Next.js, where the directory structure directly maps to the URL structure. This makes it easier to navigate and understand your project.
To organize your pages and routes, keep in mind that consistent naming conventions improve readability and maintainability. Here are some guidelines to follow:
By following these conventions, you'll make it easier for yourself and others to understand your code. This is especially important when working with components, where clear and consistent naming conventions are vital. For example, use a separate file for styles, like Button.module.css, to keep your code organized.
Intriguing read: Nextjs Code Block
Next.js Configuration
When building a Next.js app, it's essential to consider how you'll manage your build settings and environment variables. This is crucial for deployment.
To store sensitive data like API keys and database credentials, use environment variables. This is a best practice for securing your app's data.
Curious to learn more? Check out: Nextjs Build Fails Environment Variables
You can use a .env file to store and load environment variables. This is a common approach that makes it easy to manage your app's configuration.
Here's a quick rundown of how you can configure your build settings and environment variables in Next.js:
Build Settings and Environment Variables
Managing build settings and environment variables is crucial for deployment. You want to store sensitive data like API keys and database credentials securely, so environment variables are the way to go.
Store sensitive data like API keys and database credentials in environment variables.
A .env file is a great tool for storing and loading environment variables. This keeps your sensitive data out of your codebase and makes it easy to switch between different environments.
Use a .env file to store and load environment variables, and keep your sensitive data safe.
Next.js allows you to configure build settings through the next.config.js file. This is where you can control things like the output directory and runtime environment.
Check this out: Nextjs Server Actions File Upload
Configure build settings like output directory and runtime environment through the next.config.js file.
Here's a quick rundown of the key players:
API Routes and Middleware
API routes in Next.js 14 are files that handle HTTP requests. You can organize them in an api folder or with your page components. Middleware functions can handle tasks like authentication and caching.
To keep things tidy, it's a good idea to keep API routes organized and easy to find. This will save you time and frustration when you need to make changes or debug issues.
Middleware is a great way to handle common tasks, such as authentication and caching. By using middleware, you can keep your API routes clean and focused on the task at hand.
Implementing authentication and authorization is crucial for any API. This will help you control who has access to your API and what they can do once they're logged in.
Here are some best practices to keep in mind:
- Keep API routes organized and easy to find.
- Use middleware for common tasks.
- Implement authentication and authorization.
Advanced Features
In a Next.js app router project, you can utilize the built-in support for internationalization (i18n) to easily manage multiple languages. This feature is particularly useful for global applications.
The `app/[...params].js` file is a key component in the app router project structure, as it serves as a catch-all route for dynamic routes.
With the app router, you can create nested routes by using the `app/[...params].js` file as a parent route and then defining child routes within it.
The `app/[...params].js` file can also be used to define a catch-all route that renders a default page when no other route matches the URL.
By utilizing the `app/[...params].js` file, you can create a flexible and scalable route structure that adapts to different URL patterns.
You might like: Next Js File Structure
Frequently Asked Questions
Where do you put components in Next.js app router?
Place components in a directory at the root of your project, or in the src directory, alongside the routes
What is an app router?
The App Router is a new model for building React applications that leverages cutting-edge features like Server Components and Server Actions. It's a powerful tool for creating fast and efficient web applications, and getting started is as simple as creating your first page.
Sources
- https://staticmania.com/blog/how-to-start-with-nextjs-app-from-scratch
- https://nextjs.org/docs/app/building-your-application/routing
- https://thiraphat-ps-dev.medium.com/mastering-next-js-app-router-best-practices-for-structuring-your-application-3f8cf0c76580
- https://nextjs.org/docs/app/getting-started/project-structure
- https://nextjsstarter.com/blog/nextjs-14-project-structure-best-practices/
Featured Images: pexels.com