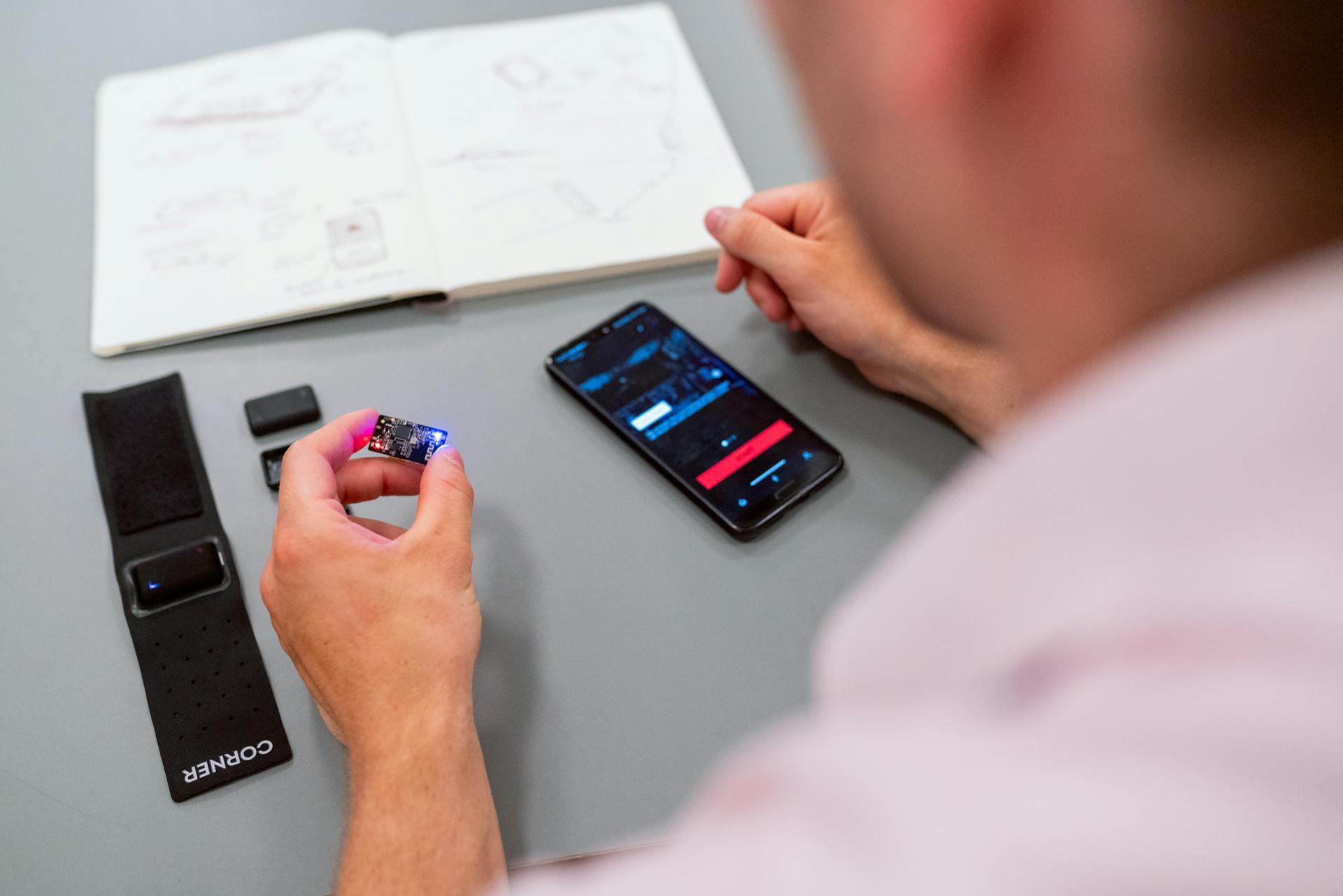
Nextjs 14 introduces a new WebSocket API, which allows developers to build real-time applications with ease. This API enables bidirectional communication between the client and server, making it perfect for applications that require live updates.
With the WebSocket API, developers can create real-time features such as live updates, chat applications, and gaming platforms. The API provides a simple and efficient way to establish and maintain WebSocket connections.
Nextjs 14 also provides a built-in WebSocket library, which simplifies the process of setting up and managing WebSocket connections. This library provides a range of features, including connection management, message sending, and error handling.
On a similar theme: Hire Next Js Developers
Getting Started
Getting Started with Next.js 14 and WebSockets is a breeze. You can create a Next.js application using the Create Next App package.
To begin, run npx create-next-app websocket-example, where websocket-example is the name of your project. This will generate a boilerplate app that you can build upon.
You can then start exploring the features of Next.js 14 and WebSockets by diving into the code and experimenting with different configurations.
The Create Next App package will give you a solid foundation to work from, allowing you to focus on building your WebSocket-powered application.
Check this out: Next Js Spa
Creating the App
We'll be building a very simple chat application that uses WebSocket to allow multiple clients to send and receive messages in real-time.
Our chat app will have an input where users can send a message for all clients to see, and we'll be making it dead simple with no authentication or users.
To initiate a WebSocket connection, we'll use a custom server for Next.js, which will handle WebSocket requests.
In fact, we won't be creating a file-based route in Next.js like you normally would, but instead, we'll use a route that doesn't matter what it is.
We'll need to install the dependencies for our custom server, which will enable us to make this route usable.
Now that we have our Next.js app running on a custom server, we can finally build our chat app.
Explore further: Next Js Custom Server
Integrating WebSocket
Integrating WebSocket into your Next.js 14 application requires a few key steps. To start, you'll need to import the io instance from the WebSocket server into your pages/_app.js file.
Take a look at this: Nextjs Ssr Websocket
This is done by importing the io function from the socket.io-client library and establishing a connection to your WebSocket server when the application loads. You'll also want to log a message when the connection is established and disconnect the socket when the component is unmounted.
To set up the API route, ensure you have an API route in the pages/api directory that initializes the Socket.io connection if it doesn't already exist. The file's contents should be similar to the socket.js file discussed earlier.
On the client-side, you can connect to the WebSocket server using the Socket.io client in your Next.js pages. This is done by importing the io function and using the useEffect hook to establish a connection when the component mounts.
Here's a step-by-step guide to setting up the client-side connection:
- Import the io function from the socket.io-client library.
- Use the useEffect hook to establish a connection to the WebSocket server when the component mounts.
- Log a message when the connection is established.
- Disconnect the socket when the component is unmounted.
Keep in mind that if you're deploying your Next.js app on serverless architecture, WebSockets may not work out of the box. However, you can use third-party services to add WebSocket support or set up the API route and client-side connection as described above.
Adding WebSocket Features
To integrate WebSocket with NEXT.js, you need to import the io instance from the WebSocket server in the pages/_app.js file.
You can establish a connection to the WebSocket server when the application loads and log a message when the connection is established. However, this doesn't work out of the box on serverless architecture, so you might need to use third-party services or another option.
To set up the client-side connection, you can use the Socket.io client in your Next.js pages. This involves importing the io function and establishing a connection to the WebSocket server using the useEffect hook.
Here's how you can set it up in pages/index.js:
- Import the io function and establish a connection to the WebSocket server using the useEffect hook.
- Log a message when the connection is established.
- Disconnect the socket when the component is unmounted.
By following these steps, you can enable WebSocket features in your Next.js application and create real-time connections between clients and the server.
Notifications
Notifications are a crucial part of real-time communication between the server and clients.
With a WebSocket connection established, we can now emit and receive notifications between the server and clients. This allows for seamless communication and updates in real-time.
We can emit notifications whenever a certain event occurs, as we did in our example where we emitted a sample notification to the connected client after 5 seconds.
To receive notifications, we use the useState hook to manage an array of notifications. This helps us update the state to include new notifications when they are received from the WebSocket server.
Notifications are then displayed in a list on the webpage, making it easy for users to see updates and changes in real-time.
Emitting Messages
Emitting messages with WebSocket is a powerful feature that enables real-time communication between clients and the server. This is achieved by sending events from the client to the server and vice versa.
To emit messages, you need to use the socket.emit() function. This function takes two parameters: the event name and the message. For example, in the server-side code, we emit a sample notification to the connected client after 5 seconds.
Curious to learn more? Check out: Next Js Client Side Rendering
Here's an example of how to emit a message from the client to the server:
- Event name: input-change
- Message: the value of the input field
The server then receives this event and broadcasts it to all clients except the one that emitted the event. This is achieved using the socket.broadcast.emit() function.
Here's an example of how to handle the broadcasted event on the client-side:
- Subscribe to the event using socket.on()
- Update the input value with the value sent from the server using the setInput function
By using these techniques, you can create a real-time messaging system that enables clients to communicate with each other and the server.
Enhancing the Implementation
You can take your real-time notification system to the next level by implementing authentication and authorization mechanisms to ensure that notifications are delivered only to authorized users.
Authentication and authorization will help prevent unauthorized access to sensitive information and keep your users' data secure.
To extend the notification payload, consider including different types of notifications, such as messages, friend requests, or activity updates.
This will enable you to provide a more comprehensive and engaging experience for your users.
If you want to replace the sample notifications with real-time updates from a database, you can integrate your application with a real database to fetch new comments, likes, or posts.
This will make your application feel more dynamic and responsive.
Customize the notification display to match your application's design and branding for a seamless user experience.
Error handling is also crucial to implement, as it will help your application gracefully handle situations where the WebSocket connection is lost or encounters errors.
Here are some ways to enhance the implementation:
- Implement authentication and authorization
- Extend the notification payload
- Integrate with a real database
- Customize the notification display
- Error handling
Sources
- https://dev.to/franciscomendes10866/building-real-time-applications-with-nextjs-and-websockets-588j
- https://clouddevs.com/next/real-time-notifications-with-websockets/
- https://firebase.google.com/docs/hosting/frameworks/nextjs
- https://fly.io/javascript-journal/websockets-with-nextjs/
- https://blog.logrocket.com/implementing-websocket-communication-next-js/
Featured Images: pexels.com