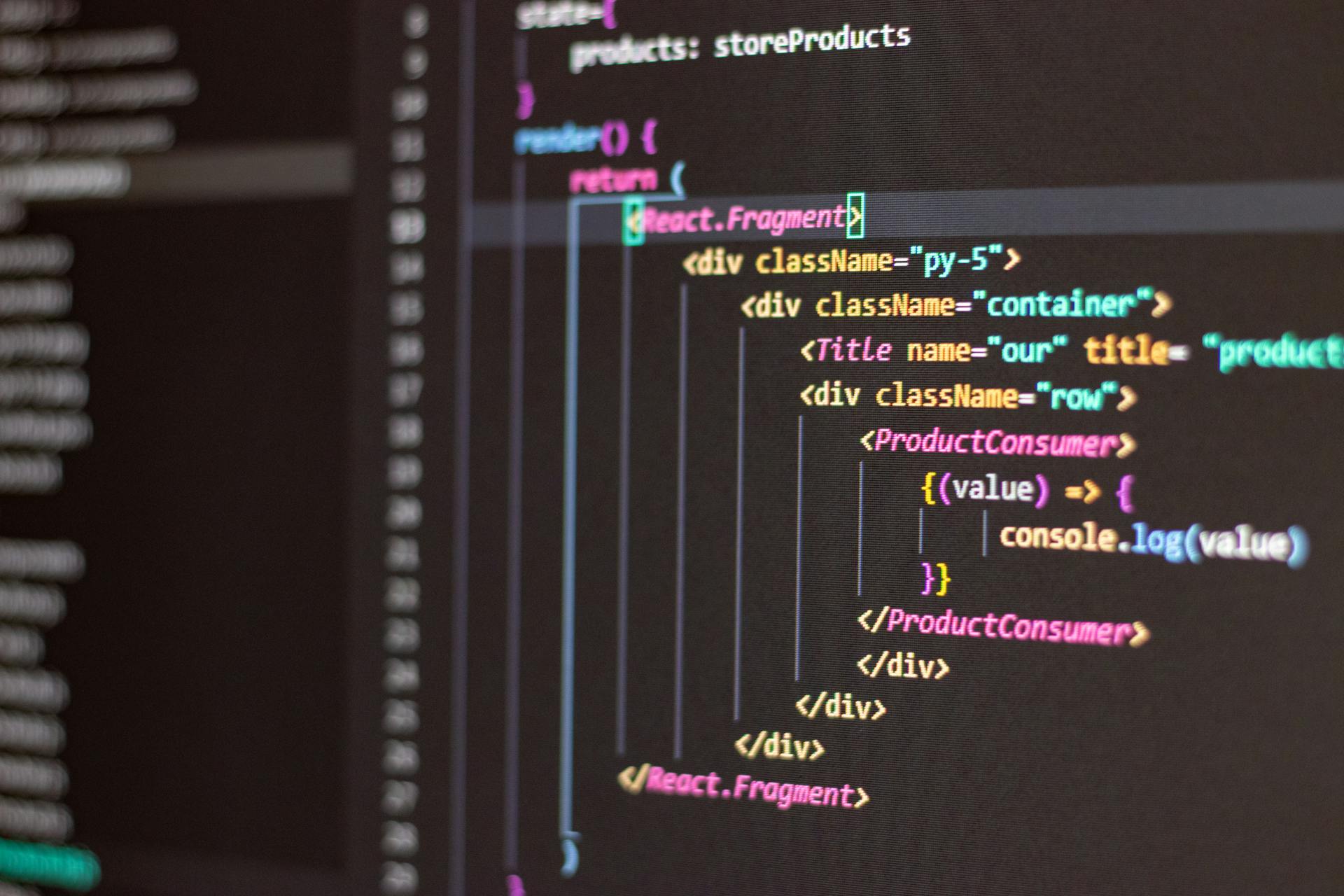
Setting up a custom server with Next.js can be a game-changer for your application's performance and scalability. By integrating a custom server, you can take advantage of features like dynamic routing, server-side rendering, and improved SEO.
To get started, you'll need to create a new file for your custom server, typically named `server.js`. This file will contain the code for your server, which will be executed by the `next start` command. The file should export a function that returns a new instance of the Next.js server.
Next, you'll need to configure your server to serve your Next.js application. This can be done by importing the `createServer` function from the `http` module and passing it a callback function that sets up the server. The callback function should be responsible for setting up the server's routing and handling requests.
For another approach, see: Nextjs Server Actions File Upload
Setting Up the Environment
To set up the environment for your Next.js custom server, it's essential to establish a proper development environment. This setup ensures that all necessary tools and dependencies are in place to facilitate a smooth integration process.
A different take: Nextjs Build Fails Environment Variables
You'll need to clone the Next.js Starter Project from GitHub, specifically the nextjsssl project. This will give you a solid foundation to work from.
To get started, follow these initial steps:
- Clone the nextjsssl Next.js Starter Project from my GitHub
- Install Chocolatey
- Install OpenSSL
By completing these steps, you'll be well on your way to setting up a development environment that's ready for your Next.js custom server.
Starting with NPM
To start setting up your Next.js and Express.js integration, you'll need to configure your npm start command. This involves changing the command from next start to node server.js.
You'll also need to make this change in your packages.json file. Specifically, you'll replace the command "next start" with "node server.js". This will ensure that your Next.js application starts correctly.
You can start your Next.js application by running "npm run serve". This command will kickstart your development environment and get you ready to integrate Next.js and Express.js.
Take a look at this: How to Start Next Js Project
Plan of Action
To set up your environment, you'll need to follow a specific plan of action. First, you'll want to clone the Next.js Starter Project from GitHub. This will give you a solid foundation to work from.
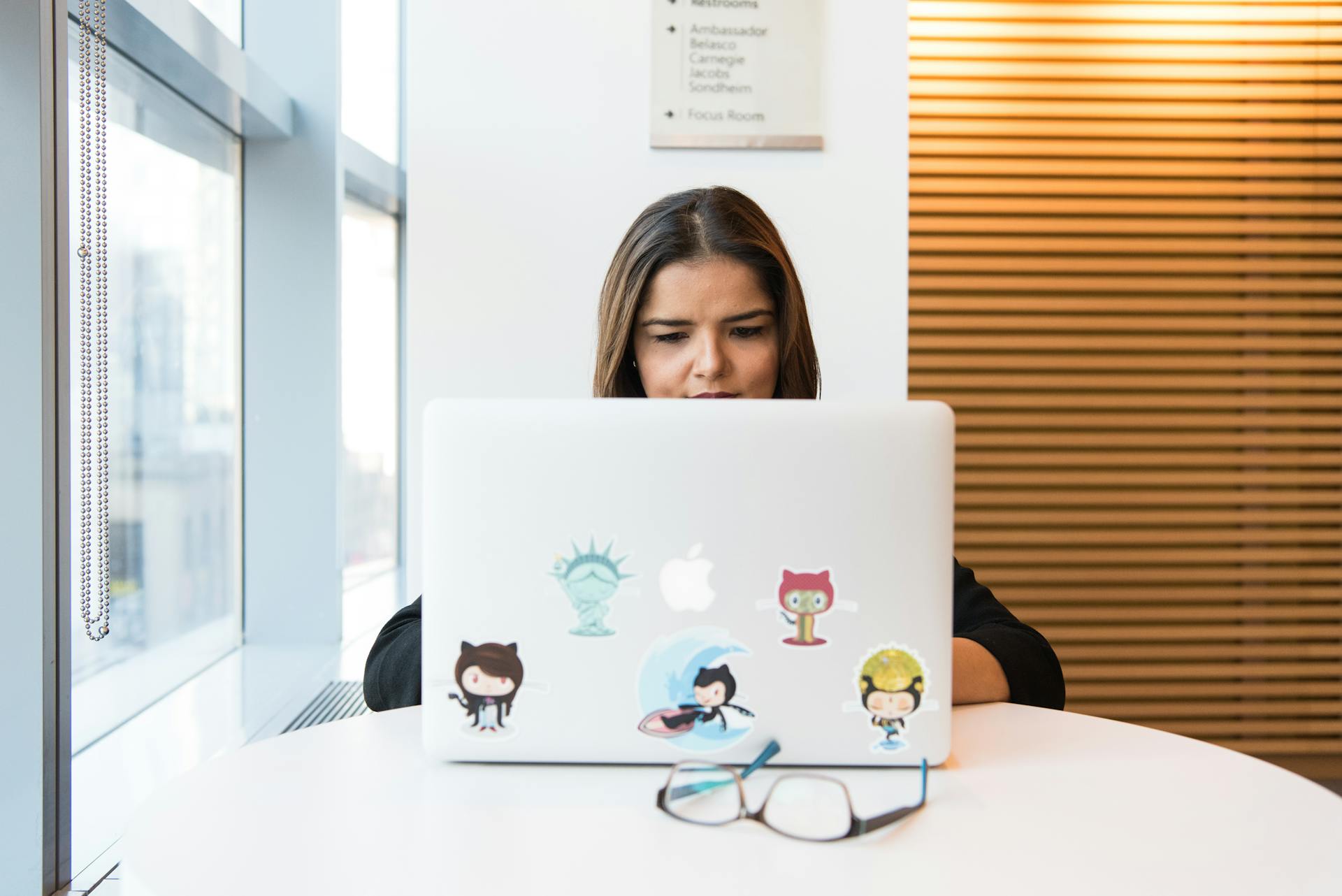
To get started, you'll need to install some essential tools. You'll need to install Chocolatey, which will make it easy to install other tools and software.
Next, you'll need to install OpenSSL, a crucial tool for creating and managing certificates. I've found that having a reliable certificate creation process is essential for a smooth development experience.
Here's a step-by-step guide to help you stay on track:
- Clone the Next.js Starter Project from my GitHub
- Install Chocolatey
- Install OpenSSL
- OpenSSL Certificate Creation
- Certificate Installation
- Update npm run-script
By following these steps, you'll have a solid foundation for your environment, and you'll be well on your way to setting up your project for success.
Prerequisites
To get started with a Next.js custom server, you'll need to have Node.js installed on your machine. Ensure you have the latest stable version installed.
You'll also need a package manager like npm or Yarn to install and manage JavaScript libraries and tools.
A code editor like Visual Studio Code, Sublime Text, or Atom is necessary for writing and editing your JavaScript code.
Having basic knowledge of JavaScript and React is crucial, including familiarity with JavaScript syntax and React concepts like components and state management.
Some experience with backend development, particularly with Express.js or another JS backend framework, will be helpful.
Additional reading: Next Js React Fundamentals
Collaboration and Integration
Combining Next.js and Express.js allows developers to leverage the strengths of both frameworks. Express.js takes charge of the backend development, managing API routes and server-side logic.
This integration enables the creation of custom server patterns, where Express.js acts as the custom server, handling all the server-side logic. Next.js manages the rendering of React components and static generation, resulting in a seamless client-side navigation experience.
The collaboration between Next.js and Express.js also provides a scalable and performance-oriented server-side infrastructure.
You might like: Google Fonts Nextjs
Setting Up the Integration Environment
Before diving into the integration of Next.js and Express.js, it's essential to establish a proper development environment. This setup ensures that all necessary tools and dependencies are in place to facilitate a smooth integration process.
You'll need Node.js, the runtime environment for running JavaScript code server-side, installed on your machine. Ensure you have the latest stable version installed.
A code editor is also a must-have, such as Visual Studio Code, Sublime Text, or Atom, for writing and editing your JavaScript code.
Curious to learn more? Check out: Nextjs Code Block
To get started, make sure you have basic knowledge of JavaScript and React, including familiarity with JavaScript syntax and React concepts like components and state management.
Some experience with backend development, particularly with Express.js or another JS backend framework, will be helpful in this process.
Here are the prerequisites you'll need to install on your machine:
- Node.js
- npm or Yarn
- A code editor
- Basic knowledge of JavaScript and React
- Understanding of backend concepts
Once you have these prerequisites installed, you can navigate to your project directory and start preparing Next.js to handle requests.
Collaboration Between Express.js
Collaboration Between Express.js and Next.js is a powerful combination that allows developers to leverage the strengths of both frameworks. This integration enables the creation of custom server patterns, where Express.js acts as the custom server, handling all the server-side logic, and Next.js manages the rendering of React components and static generation.
By combining Next.js and Express.js, developers can create a seamless client-side navigation experience, with the added benefit of a scalable and performance-oriented server-side infrastructure. This is achieved through the use of React components and server-side rendering capabilities of Next.js, while Express.js handles API routes and server-side logic.
For another approach, see: React + Next Js
Express.js can be configured to work alongside Next.js by creating a new JS file in the Next.js project, often named server.js. This file serves as the entry point for the server, where Express.js is configured to handle server-side logic.
Here are the key benefits of collaborating between Express.js and Next.js:
- Seamless client-side navigation experience
- Scalable and performance-oriented server-side infrastructure
- Custom server patterns
With this powerful combination, developers can build high-quality web applications that are both performance-oriented and feature-rich. By integrating Express.js and Next.js, developers can take advantage of the strengths of both frameworks, resulting in a robust and efficient web application.
Consider reading: Hire Next Js Developer
Express.js Configuration
To configure a custom server with Next.js, you can use the same options as in a next.config.js file. These options include setting up API routes and middleware.
You can implement API routes and middleware to extend the functionality of your Next.js application. For example, you can add custom backend features such as user authentication, data processing, and more. By implementing API routes and middleware, you can leverage the strengths of both Next.js and Express.js.
On a similar theme: Nextjs Middleware Firebase Auth
Here are some key options to consider when configuring your custom server:
By configuring your custom server with these options, you can create a seamless client-side navigation experience with a scalable and performance-oriented server-side infrastructure.
For your interest: Next Js Client Side Rendering
When to Use?
When to use a custom Express.js configuration? You should only use it when the built-in configuration can't meet your app's requirements. If you need to customize the routing behavior, a custom configuration is the way to go.
Customizing routing behavior is crucial for complex applications. For instance, if you need to add middleware for authentication, a custom configuration can help. This is especially true if you're dealing with sensitive user data.
Adding middleware for authentication or error handling is a common reason to use a custom configuration. This allows you to fine-tune your app's security and performance.
Intriguing read: 'use Server' Nextjs
Setting Up Express.js
To set up Express.js, you'll need to create a new JS file in your project, often named server.js. This file will be the entry point for your server, where you'll configure Express.js to work alongside Next.js.
On a similar theme: Next Js Directory Structure
You'll need to install Express and its Typescript definitions using npm. Create a server folder and a server entry point, typically named index.ts.
In the index.ts file, you'll need to populate it with code that imports Express and sets up your server. However, you'll encounter an issue with the tsconfig.json file, which is set to "module": "esnext" by default.
To resolve this, you can create another config file, tsconfig.server.json, that extends the tsconfig.json file and sets the "module" option to "commonjs". This will allow both Express and Next.js to work together.
Here's a breakdown of the options you'll need to set in the tsconfig.server.json file:
- "extends": "./tsconfig.json" - Gets all options from tsconfig.json
- "compilerOptions.module": "commonjs" - Use commonjs as the module pattern
- "compilerOptions.outDir": "dist" - Compile all .ts files in the server directory to .js and send to the dist directory
- "compilerOptions.noEmit": true - Override the value to tell TS compiler compile and output our server files
- "include": ["server"] - Let the TS Compiler know only compiling .ts files in the server/ directory
Frequently Asked Questions
Does Next.js have its own server?
Yes, Next.js includes its own server, which can be started with the command `next start`.
How to host Next.js app on server?
To host a Next.js app on a server, you can deploy it to a platform like Vercel, which offers a seamless integration with Next.js, or explore other hosting options such as GitHub Pages or Netlify. Learn more about deploying your Next.js app to a server in our comprehensive guide.
Sources
- https://www.dhiwise.com/post/the-developer-guide-to-nextjs-express-integration
- https://www.bymoji.com/blog/Custom_Server_in_Nextjs_How_to_Create_Your_Own_Server_for_Complete_Customization
- https://lukman.hashnode.dev/how-to-create-a-custom-server-in-nextjs-using-vhost-and-express
- https://levelup.gitconnected.com/set-up-next-js-with-a-custom-express-server-typescript-9096d819da1c
- https://www.briangetsbinary.com/nextjs/software%20engineering/2023/04/26/nextjs-configuring-localhost-ssl.html
Featured Images: pexels.com