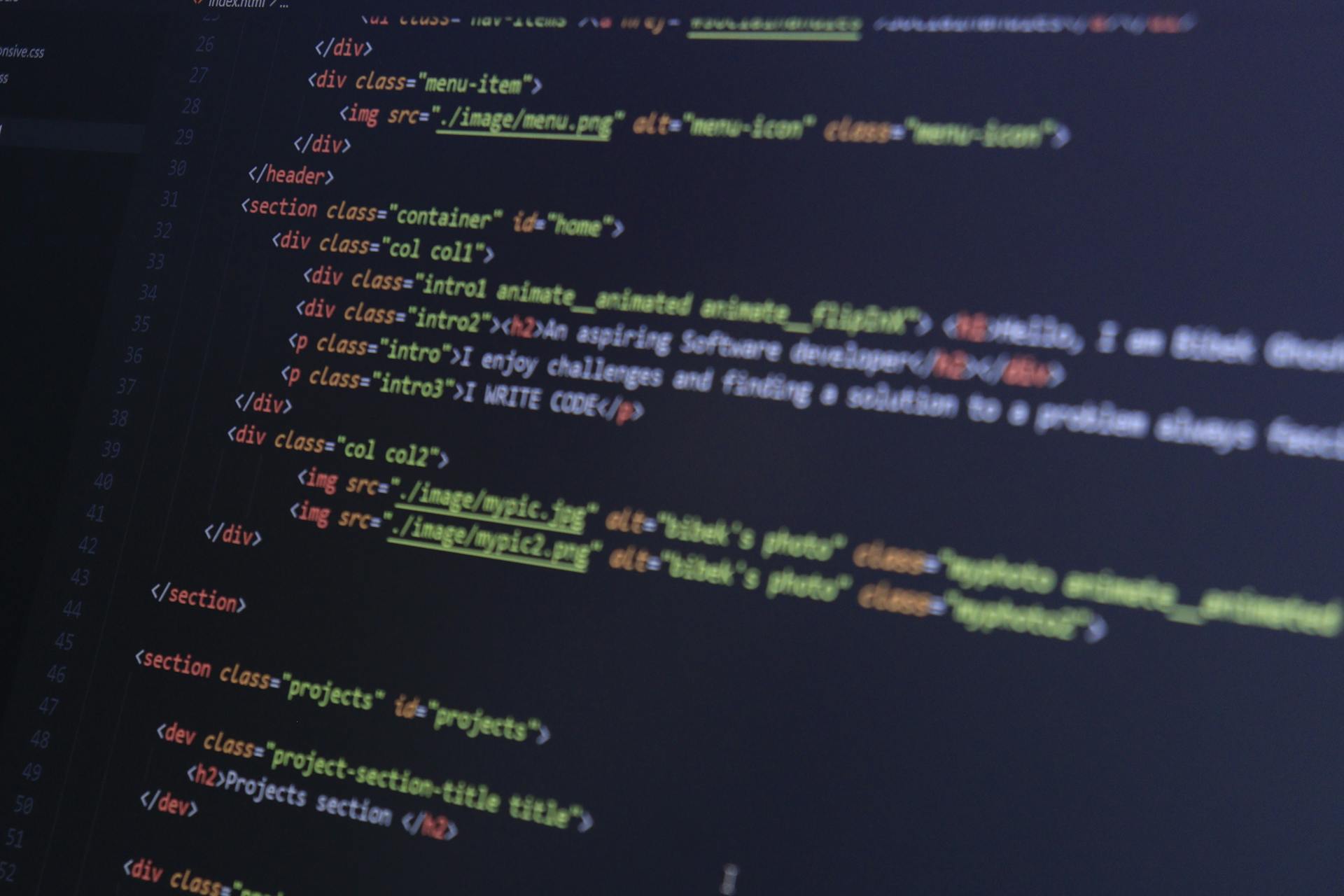
To start a Next.js project, you'll need to create a new project folder and initialize a new Node.js project using the command `npx create-next-app my-app`. This will create a basic Next.js project structure.
You'll then need to navigate into the project folder and install the dependencies using `npm install`. Next.js requires a few specific packages to be installed, including `react` and `react-dom`.
Start by creating a new file called `pages/index.js` and add some basic HTML to get started. This is where your website's homepage will be rendered.
Next, you'll need to create a new file called `components/Layout.js` to define the layout of your website. This will include the header, footer, and any other repeating elements.
Configure Your Environment
To configure your environment for a Next.js project, start by initializing a new app using the CLI with the command `create-next-app`. This will generate a bunch of starter files for you.
You can also use the `create-next-app` command to create a project directory, install necessary dependencies, initialize a new Git repository, and create an initial commit with all the added files. This gives you a good starting point for managing your project's source code history.
To install and configure Transformers.js, use the command `npm install transformers`. You'll also need to update the `next.config.js` file to ignore node-specific modules when bundling for the browser.
Creating a new Web Worker script is essential for ensuring the main thread isn't blocked while the model is loading and performing inference. You can place all ML-related code in this script.
For this application, you'll be using a ~67M parameter model finetuned on the Stanford Sentiment Treebank dataset. Add the necessary code to your `worker.js` file to get started.
Page Structure
In a Next.js project, your page structure is crucial for organizing your code and making it easy to maintain.
The public folder is where you'll store static assets and pages that don't need server-side rendering.
You can create a new page by creating a new file in the pages directory, such as pages/about.js. This file will be treated as a separate page by Next.js.
Components
Components are a crucial part of organizing your project's structure.
You can add a custom folder to create components to use in your project, such as a /components folder where you can store your reusable components. Later, you can use them in your Next.js pages.
Routing
Routing is easy with Next.js. You can create a route for any page by creating a file within the pages folder.
If you create a file called about.js, it will automatically become a route for the /about page. The exception is index.js, which routes to the root /. You can also create folders to create routes like /blog/my-post-title.
To dynamically create pages for each item in an array, create a file called [color].js. This will allow you to create routes for each item in the array, like 'Classic Blue' and 'Ultra Violet', all at once.
Design and UI
Designing the user interface is where the magic happens. You can add a simple UI to the Home component with an input textbox and a preformatted text element to display the classification result.
This is a crucial step, as it's where you'll start to see your app take shape. The input textbox will allow users to interact with your app, while the preformatted text element will display the results of their input.
To create a clean and organized design, remove all the content in app/globals.css and replace it with the basic styles provided.
Link Component
The Link component is a powerful tool in Next.js that allows for client-side route transitions, making page transitions smoother for users. This is especially useful when you want to create a seamless user experience.
You can use the Link component in place of an anchor tag (a tag) to link to other pages. This is exactly what we did in our example, where we updated the index.js file with the list of colors.
The Link component is specifically designed for Next.js, making it a great choice for building fast and efficient web applications.
Design UI
Design UI is a crucial step in building a user-friendly app. In the Next.js starter app, you can add a simple UI to the Home component by including an input textbox and a preformatted text element to display the classification result.
The input textbox allows users to interact with your app, but you need to design it in a way that's intuitive and easy to use. To do this, you can draw inspiration from other apps and websites that have a similar functionality.
To create a clean slate for your styles, remove all the content in app/globals.css and replace it with basic styles. This will ensure that your UI is consistent throughout the app.
Deployment
Deployment is a crucial step in getting your Next.js app live online. AWS Amplify supports deploying both SSR and SSG Next.js apps without any additional configuration.
If you're creating a statically generated Next.js app, change your build script in the package.json file to next build && next export. This will prepare your app for deployment.
To deploy your app, create a repository on your git provider of choice, and push your code to it. You'll also need an AWS account, which you can create if you don't already have one.
Here's a step-by-step guide to deploying your app with AWS Amplify:
- Create an AWS account if you don't already have one.
- Navigate to the Amplify Console.
- Click on the orange connect app button.
- Choose GitHub in the From your existing code menu, and click continue.
Once you've connected your app, you'll need to configure the build settings. The build settings will auto-populate, so you can just click next on the Configure build settings.
Finally, click Save and deploy to get your app live online.
Alternatively, you can deploy your app to Vercel using the vercel CLI. This will install the CLI, log you in to Vercel, create the app, and deploy it.
Backend and Stripe
To set up your backend for a Next.js project, you'll need to integrate Stripe Checkout. This can be done using Next.js with Stripe Checkout and TypeScript.
You can get started with this combination by following a tutorial like "Next.js with Stripe Checkout and Typescript".
Stripe Checkout provides a secure and seamless payment experience for your users.
Build the Backend
Building the backend is a crucial step in creating a seamless user experience. You'll need to create two server-side endpoints: one for running the model and one for polling the status of that request until it's complete.
Create a directory for these endpoints, and within it, create two files: `app/api/predictions/route.js` and `app/api/predictions/[id]/route.js`. The `[id]` in the directory structure is a feature of Next.js called dynamic routing, which treats the `id` part of the URL as a variable.
To handle prediction creation requests, add the following code to `app/api/predictions/route.js`. This will enable you to create a new prediction and store its ID.
To handle requests to poll for the prediction's status, add the following code to `app/api/predictions/[id]/route.js`. This will allow you to check the status of a prediction by its ID.
Here's a breakdown of the key components involved in server-side inference:
Note that `pipeline.js` uses the Singleton pattern to enable lazy construction of the pipeline, which is a common pattern in JavaScript. This ensures that the pipeline is only created once and reused throughout the application.
Next.js with Stripe
Next.js with Stripe is a popular combination for building e-commerce websites.
You can integrate Stripe Checkout with Next.js using a library like next-stripe.js, which makes it easy to add payment functionality to your site.
Next.js with Stripe Checkout and Typescript is a more advanced setup that requires some additional configuration, but it provides a lot of flexibility and customization options.
To get started with Next.js and Stripe, you'll need to install the required packages and set up a Stripe account.
Stripe Checkout can be easily integrated into your Next.js site using a simple API call, allowing you to accept payments in a seamless and secure way.
Next.js with Stripe Checkout and Typescript requires you to set up a Stripe Checkout session, which involves creating a secret key and a publishable key.
You can use the Stripe Checkout library to customize the payment form and make it fit your site's branding and design.
By following the steps outlined in the example "Next.js with Stripe Checkout and Typescript", you can successfully integrate Stripe Checkout into your Next.js site.
Stripe's robust documentation and support make it easy to troubleshoot any issues that arise during the integration process.
The example "Next.js with Stripe Checkout and Typescript" shows how to use TypeScript to type-check your Stripe API calls and ensure that your code is robust and maintainable.
Frequently Asked Questions
What is the next start command?
The next start command starts the application in production mode, requiring a previous compilation with next build. It also accepts an optional directory parameter to specify where to start the application.
How do I start a Next.js development server?
To start a Next.js development server, run `npm run dev` in your terminal. This will launch the server at http://localhost:3000, where you can view and interact with your application.
Featured Images: pexels.com