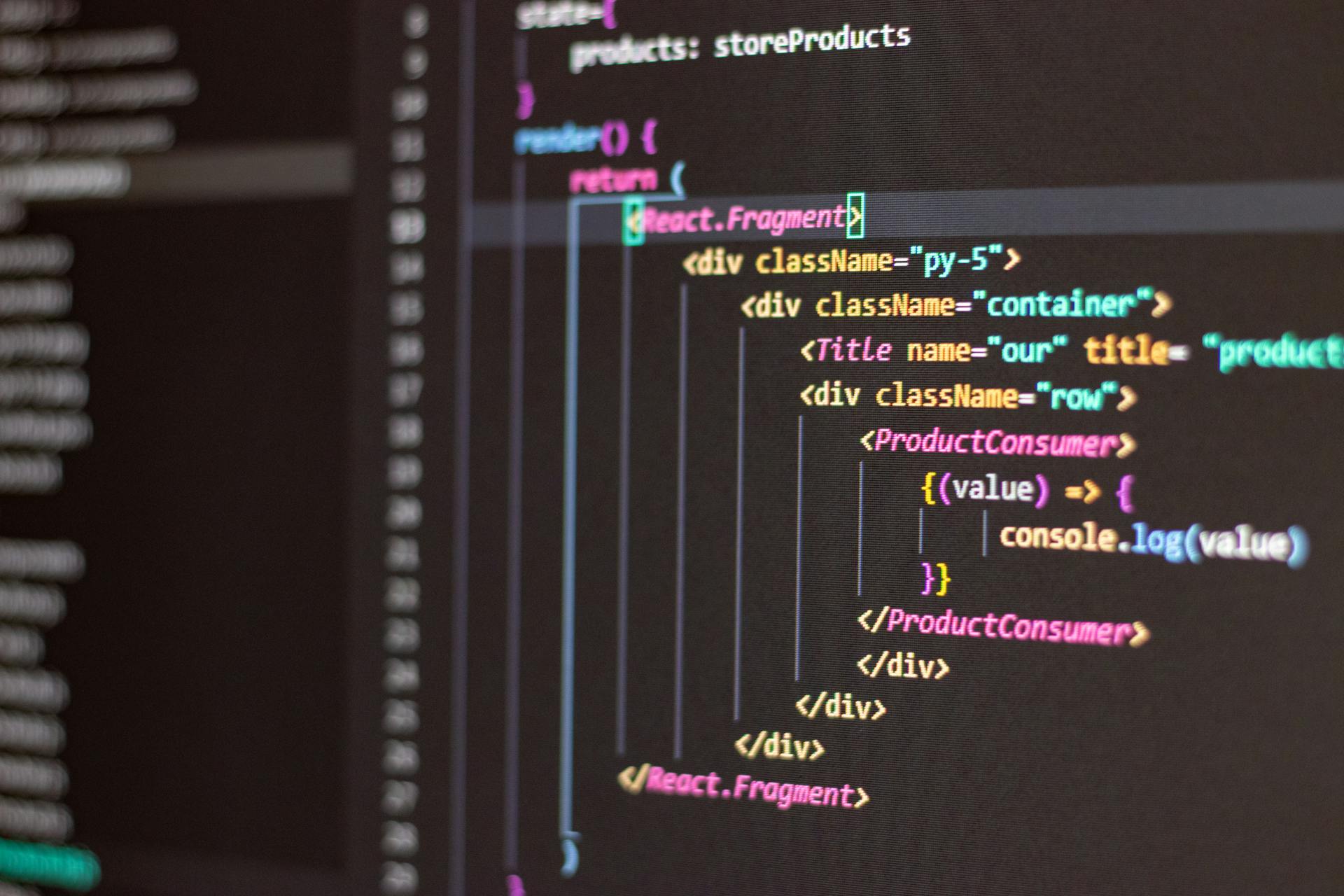
Cloning a Next.js project can be a daunting task, but don't worry, I've got you covered. You'll need to start by creating a new project using the `create-next-app` command.
First, make sure you have Node.js installed on your computer. You can check by running `node -v` in your terminal. If you don't have it installed, download it from the official Node.js website.
Next, run `npx create-next-app my-app` in your terminal to create a new Next.js project. This will create a basic project structure for you to work with.
The project will be created in a directory called `my-app`, and you can navigate into it by running `cd my-app` in your terminal.
If this caught your attention, see: Running Json Nextjs
Project Setup
To set up a new Next.js project, you'll need to create a new directory and initialize a new Node.js project using npm or yarn.
You can use the `npx` command to create a new Next.js project, which will also install the required dependencies. The command is `npx create-next-app my-app`, where `my-app` is the name of your project.
Discover more: Next Js Cookie
Navigate to the project directory using the `cd` command, and then run the command `npm install` or `yarn install` to install the dependencies.
Next.js projects come with a basic configuration file called `next.config.js`. This file contains settings for the project, such as the development server port and the output directory for the built application.
The `next.config.js` file is also where you can configure features like internationalized routing and API routes.
Discover more: Next Js File Structure
Frontend Development
In Next.js project cloning, frontend development is a crucial step. You'll need to create the frontend code that renders a form.
The frontend code should post data to the server-side endpoint, which runs the model with Replicate and returns a prediction. This prediction is an object representing a single model run.
To start building the frontend, remove all the existing content in the app/page.js file and replace it with the following code.
See what others are reading: Next Js Debug
Deploy to Vercel
Deploying your frontend app to Vercel is a straightforward process that can be completed using the vercel CLI.
Check this out: Nextjs by Vercel Meaning
You'll need to install the CLI first, which will then guide you through the process of logging in to Vercel, creating the app, and deploying it.
The CLI will prompt you to enter your API token, which you'll need to paste in for your app to make requests to Replicate.
This is the same token you used earlier in the process.
Build the Frontend
Building the frontend is a crucial step in frontend development. You've finished writing the server-side code that talks to Replicate.
To create the frontend code, you'll need to render a form that allows users to enter a prompt and submit it. The form will post the data to the server-side endpoint that you created, which will then run the model with Replicate and return a prediction.
You'll replace the existing content in the app/page.js file with the new code. This file already exists in your project, and it renders the default "Welcome to Next.js" home route. Remove all the existing content in that file.
The new code will be responsible for rendering the form and handling the submission. It will post the data to the server-side endpoint that you created in the previous step, which will then run the model with Replicate and return a prediction.
A unique perspective: Next Js Client Side Rendering
Add Basic Styles
Adding basic styles is a crucial step in frontend development.
The Next.js starter app includes some CSS styles that are used on the default splash page, but they aren't really intended to be reused for a real app.
To create a clean slate for your styles, remove all the content in app/globals.css.
Styling is a crucial thing in every front-end project.
Always remove existing styles in your CSS file to start from scratch.
You'll want to replace it with basic styles to ensure a clean and consistent look.
Even if you use ready-made frameworks like bootstrap or tailwind, there will be some styling to change.
Intriguing read: Apply Css Nextjs
Components and Features
Cloning a Next.js project is a straightforward process that involves several key components and features.
The project structure is based on a directory with a `pages` directory where all the pages are located. This is a standard structure for Next.js projects.
One of the main features of Next.js is its support for internationalization (i18n) out of the box. This means you can easily add support for multiple languages to your project.
To get started with cloning a Next.js project, you'll need to have Node.js and npm installed on your machine. This is because Next.js is built on top of the React framework and uses npm as its package manager.
You might enjoy: Next Js App Router Project Structure
Components
Components are the building blocks of any Next.js app, and they're essential for creating a user-friendly interface. You'll need to create separate components for the header and footer.
To start, let's create a header component. In Next.js, this is done by creating a single component named header.jsx and adding the necessary template. This will be the element that almost every app needs.
The footer component is created similarly, by making a footer.jsx file and component named footer. You'll then need to add a footer template that will contain the necessary buttons, such as a button that redirects to the homepage and another that redirects to the user's profile.
Components can also include state management, which is useful for tracking user interactions. For example, you can use the useState hook to set a default value of "false" for a "bookmarked" state, and its corresponding setter as "setBookmarked". This is useful for creating dynamic UI elements that respond to user actions.
For more insights, see: Nextjs Metadata Template
Creating a Card Component
Creating a Card Component is a crucial step in building a Next.js application. To create a card component, you need to create a component named "Card" in the file card.jsx.
This component should be placed in the "components/home" path. The app is small and uses a fake API, so we won't be using Redux or handling state anywhere.
To render these cards, you should visit the homepage, which is located in the pages/index.jsx file. Next, create a map function and apply it to your data, then render Card components, passing the photo's data into each of them.
The Card component should be created in the "components/home" path to keep things organized.
For another approach, see: Nextjs Server Actions File Upload
Getting API Data in React
To get data from an API in React, you'll need to make a call to a fake API that returns a JSON file containing mocked data.
You can fetch this data by opening your app's JSX file and calling the fake API at '/mocks/items.json'.
Add the initial API call in the "useEffect" hook to have it fire first.
Fetch data from the API and store it in a variable for later use.
Explore further: Next Js Fetch Data save in Context and Next Route
Data and API
To get data from an API in a Next.js project, you need to call a fake API that will return a JSON file containing mocked data. This can be done by fetching data from '/mocks/items.json'.
To have the first fire call, you can add it in the "useEffect" hook.
Recommended read: Nextjs App Route Get Ssr Data
Authentication and Next Steps
Authentication in Next.js is handled through Route Handlers, a feature introduced in Next.js 13.2. This is the preferred way to handle REST-like requests.
To configure NextAuth.js FusionAuth's provider, create a file within src/app/api/auth/[...nextauth]/route.ts and import the necessary environment variables. These variables are then exported in an object called authOptions, which can be imported on the server to get the session using getServerSession.
The FusionAuthProvider is then provided to NextAuth as a provider for any GET or POST commands sent to the /api/auth/* route.
For a link component, create a new file in src/components/LoginLink.tsx and use the signIn function from next-auth/react. This link is only used on the client side, so be sure to add use client at the top of this file.
See what others are reading: 'use Server' Nextjs
Authentication
Authentication is a crucial part of any application, and Next.js 13.2 introduced Route Handlers to handle REST-like requests.
Route Handlers are the preferred way to handle authentication in Next.js, and you can configure NextAuth.js FusionAuth's provider in a new route handler by creating a file within src/app/api/auth/[...nextauth]/route.ts.
The file will ensure you have the correct environment variables on first load of Next.js, which are then exported in an object called authOptions.
You can import authOptions on the server when you need to get your session using getServerSession.
The FusionAuthProvider is then provided to NextAuth as a provider for any GET or POST commands that are sent to the /api/auth/* route.
To create a login link, you'll need to create a new file in src/components/LoginLink.tsx that will be used for a link component, and add use client at the top of this file.
This link will only be used on the client side, so make sure to use the signIn function from next-auth/react.
You can login to FusionAuth with Email: [email protected] and Password: password, although this is not ideal for production.
Here's an interesting read: Next Js Use Client
Next Steps
Now that you have a proof of concept up and running, it's time to think about taking your application to the next level.
To run your application in production, you'll want to do some additional things that you've been putting off until now. This quickstart is a great starting point, but it's not designed for long-term use.
You'll need to consider implementing authentication in a more robust way to ensure the security of your application and its users. This might involve setting up user accounts, permissions, and other security features.
One thing you can do is scale up your application to handle more traffic and users. This might involve moving to a cloud-based infrastructure or setting up load balancing to distribute traffic more evenly.
You'll also want to think about monitoring and logging to keep an eye on your application's performance and troubleshoot any issues that arise. This will help you identify and fix problems before they become major headaches.
Additional reading: Next Js Single Page Application
Frequently Asked Questions
How do I run a cloned Next.js project?
To run a cloned Next.js project, run 'npm install' to install dependencies, then start the development server with 'npm run dev'. This will get your project up and running for development.
Sources
Featured Images: pexels.com