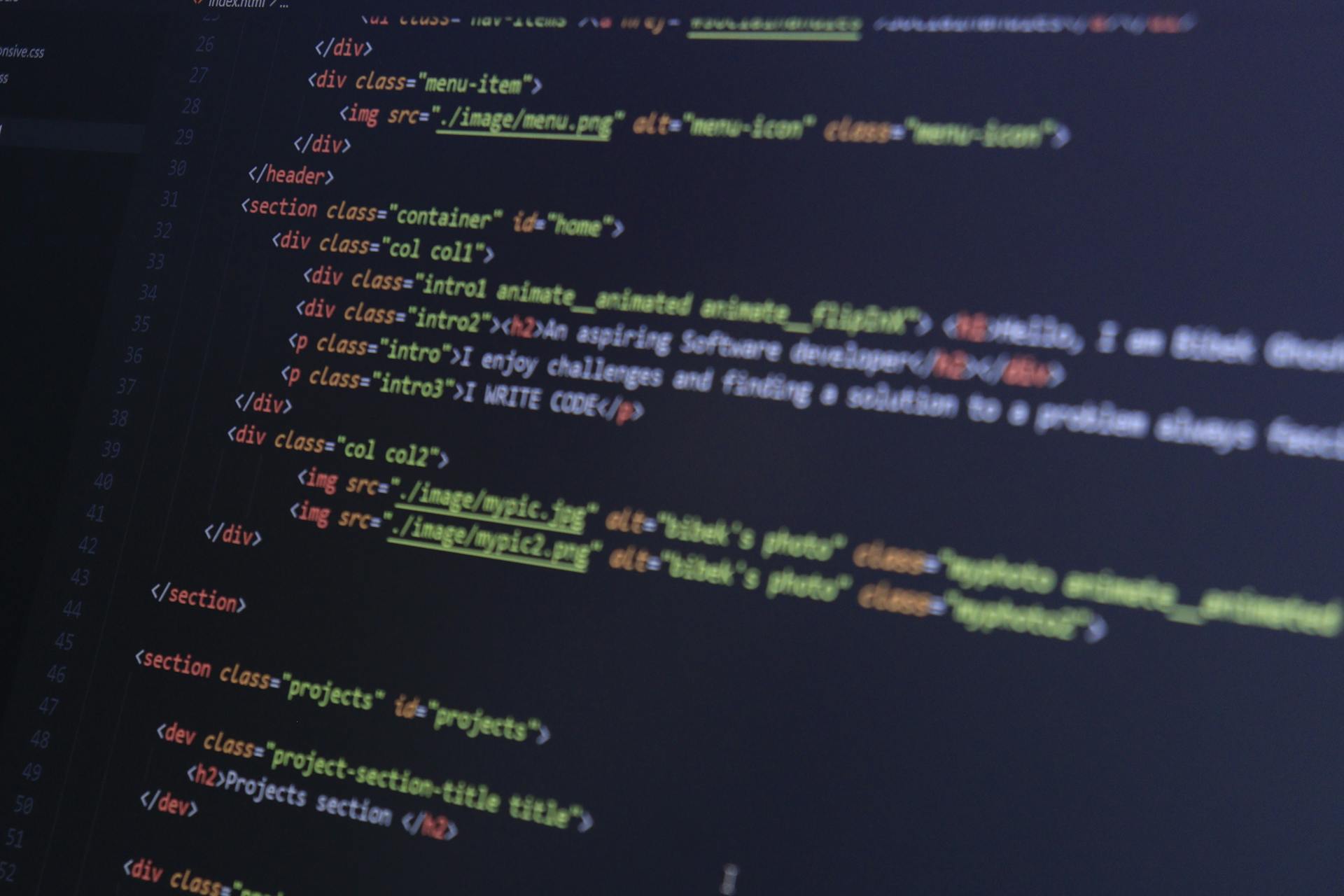
Next.js makes it easy to apply CSS with advanced styling techniques. You can use CSS-in-JS libraries like styled-components or emotion to write more maintainable and efficient CSS.
One of the key benefits of using Next.js is its support for server-side rendering, which allows you to render pages on the server before sending them to the client. This can improve performance and SEO.
To get started with CSS-in-JS, you can install a library like styled-components using npm or yarn. Then, you can import it into your pages and use its functions to create styled components.
Next.js also has built-in support for CSS modules, which allow you to write CSS in the same file as your JavaScript code. This can make it easier to manage your styles and reduce the amount of code you need to write.
Here's an interesting read: Next Js Using State Context
Custom Fonts
Custom fonts can improve the user experience and positively impact Lighthouse scores and SEO, but implementing them carefully is crucial to avoid performance issues.
Implementing custom fonts on your Next.js site can have a positive impact on Lighthouse scores and SEO, but using too many or too large font files can slow down your site's loading time and hurt your Lighthouse performance score.
Using custom fonts in a way that affects the visual stability of the page can result in lower scores for Core Web Vitals, which can impact your SEO. So, it's essential to strike a balance between using custom fonts to enhance the design and ensuring they don't negatively impact performance or user experience.
The next/font package provides an easy way to load fonts in your Next.js app, while maintaining performance and minimizing Cumulative Layout Shift (CLS). It uses the Font Loading API to asynchronously load local font files or from popular font providers like Google Fonts.
To load custom fonts, you can use the next/font/google package to load fonts from Google Fonts, or load font files stored locally in your repo using the next/font/local package.
A different take: Using State in Next Js
SEO Benefits of Custom Fonts on Next.js Sites
Implementing custom fonts on your Next.js site can improve the user experience and boost your Lighthouse scores. This is a win-win for your website and your users.
Using too many or too large font files can slow down your site's loading time, which can hurt your Lighthouse performance score. Performance issues can be a major problem if not addressed.
Incorporating custom fonts in a way that affects the visual stability of the page can result in lower scores for Core Web Vitals. This can have a negative impact on your SEO.
Striking a balance between using custom fonts to enhance the design and ensuring they don't negatively impact performance or user experience is key. It's all about finding that sweet spot.
For another approach, see: Next Js Custom Server
Loading Custom Fonts
Loading custom fonts into your Next.js app is a straightforward process. You can use the next/font/google package to load fonts from Google Fonts, or load font files stored locally in your repo using the next/font/local package.
Broaden your view: Nextjs Font
To load custom fonts from Google Fonts, you can use the variable configuration prop in _app.jsx/tsx to generate a definition for a CSS --var for each font. This will make the font available to any child of the App component, essentially all pages and components on your site.
You can also load custom fonts from a local file using the next/font/local package. This package allows you to map font weights to different files as needed, and it also generates optimized font subsets to reduce the amount of data transferred over the network.
Behind the scenes, next/font generates unique CSS classes for each font permutation, which define a CSS custom property (sometimes called a CSS variable) for each font. These variables are made available to any child of the App component, essentially all pages and components on your site.
The next/font package also provides a convenient way to generate CSS variables for each font, which can be used to create custom font families in your Tailwind config. This makes it easy to use custom fonts throughout your Next.js app with minimal configuration.
You might like: Next Js Pages vs App
Requirements
To get started with custom fonts, you'll need a few essential tools installed on your computer. Make sure you have Node.js installed to be able to install the necessary packages.
Having Node.js on your computer will allow you to use npx and npm commands to install the required packages.
You might enjoy: Nextjs Install
Adding Custom Fonts
Adding custom fonts to your Next.js app can be a game-changer for user experience.
Using custom fonts correctly on your Next.js site can improve the user experience and have a positive impact on your Lighthouse scores and SEO.
To add custom fonts, you'll want to use the next/font package, which is developed by Vercel and provides an easy way to load fonts in your Next.js app while maintaining performance and minimizing Cumulative Layout Shift (CLS).
You can load custom fonts from Google Fonts using the next/font/google package or from a local file using the next/font/local package.
The basic steps are the same for both methods.
To load custom fonts from Google Fonts, you'll need to use the variable configuration prop in your _app.jsx/tsx file to generate a definition for a CSS --var for each font.
This will make the variable available to any child of that element in the DOM, which means your whole app.
To load custom fonts from a local file, you'll need to use the same variable configuration prop and map font weights to different files as needed.
This also applies to font styles like italic and bold.
After loading your custom fonts, the next/font library generates unique CSS classes for each font permutation, which define a CSS custom property (or CSS variable) for each font.
These variables are made available to any child of the App component, essentially all pages and components on your site.
To use these variables in your Tailwind config, you'll need to add an entry to your theme.extend to create a new font family that uses the Google Fonts you just imported.
This will allow you to use the fonts you've added by prefixing the font-family with "font-" in your Tailwind classnames.
Related reading: Next Js Folder Structure
Component Styles
Component styles in Next.js are a breeze to set up. You can use Styled components to automatically vendor prefix CSS rules and avoid worrying about colocation.
One of the advantages of using Styled components is that CSS rules are automatically vendor prefixed. This saves you time and effort in maintaining your CSS.
With Styled components, you don't need to worry about specificity issues, as auto-generated class names solve this problem for you.
If you prefer to write CSS using JavaScript directly inside your components, Next.js has an inbuilt support for styled JSX. This allows you to write CSS as easily as vanilla CSS.
Alternatively, you can use libraries like Chakra, ThemeUI, or Tailwind to implement a component library and design system.
To use CSS Modules for component-level styles, create a CSS file with the .module.css extension for styles scoped to specific components. This approach allows you to apply styles that are specific to individual components without affecting others.
Intriguing read: Nextjs Loader
Here are the key benefits of using CSS Modules:
- CSS rules are automatically vendor prefixed
- CSS is scoped to specific components, avoiding global style conflicts
- Specificity is solved by auto-generated class names
To integrate component-scoped CSS, you can use CSS Modules with Sass by naming your files with the .module.scss extension. This ensures styles are scoped locally to components.
You can also use template literals to dynamically apply styles based on conditions, giving you more flexibility in your styling.
Expand your knowledge: Next Js Use Client
Frequently Asked Questions
How to use a CSS file in NextJS?
To use a CSS file in NextJS, import it from the pages/_app.js file. This is the only location where global CSS imports are allowed in NextJS.
What is the best CSS to use with Nextjs?
For Next.js projects, Tailwind CSS is a popular choice due to its utility-first approach and seamless integration with the framework. However, the best CSS library for Next.js ultimately depends on your project's specific needs and preferences.
Sources
- https://storybook.js.org/docs/configure/styling-and-css
- https://mikebifulco.com/posts/custom-fonts-with-next-font-and-tailwind
- https://www.qed42.com/insights/styling-your-next-js-application
- https://medium.com/@farihatulmaria/how-to-integrate-css-and-sass-in-next-js-6264e75bc268
- https://flowbite.com/docs/getting-started/next-js/
Featured Images: pexels.com