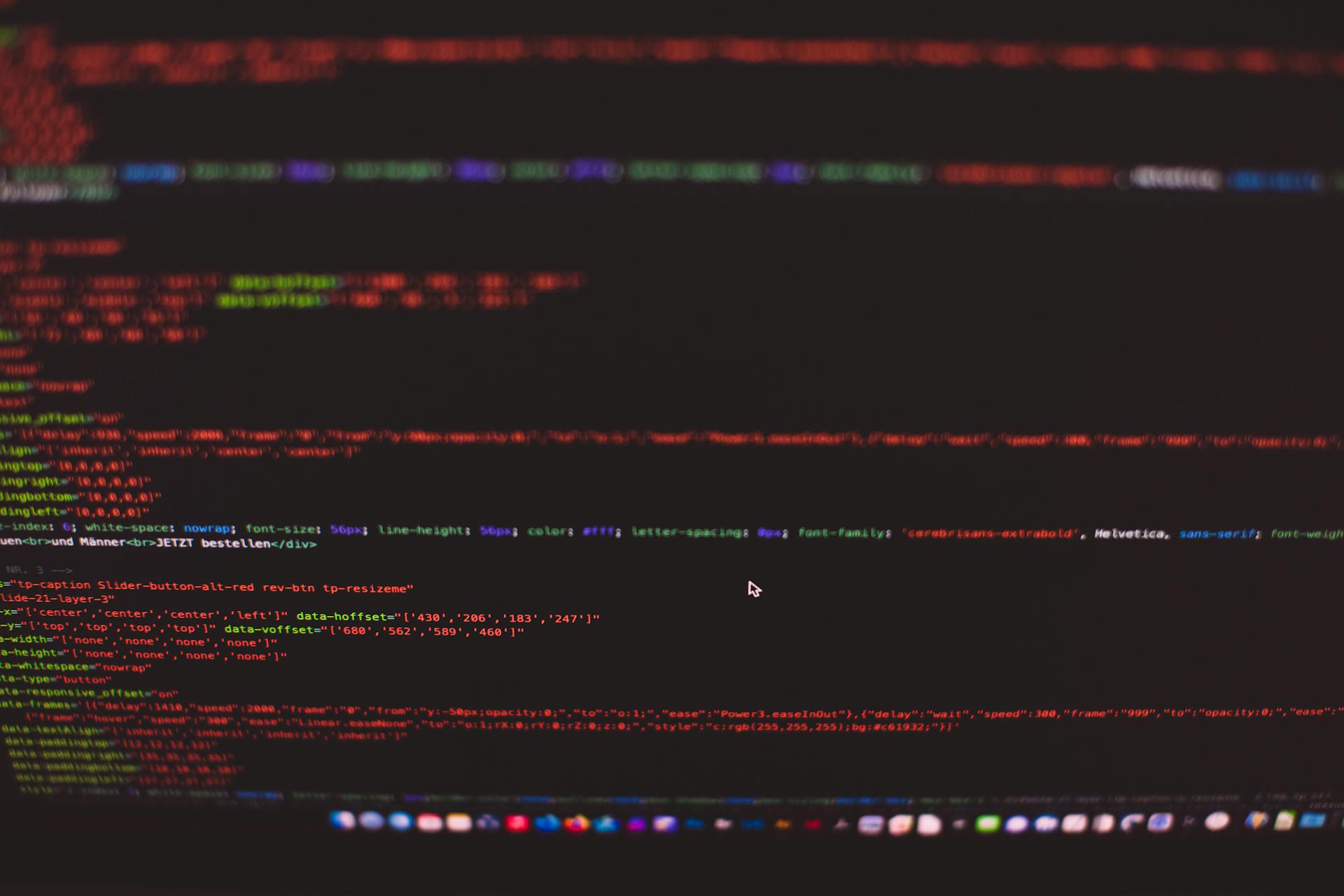
Applying CSS classes with jQuery is a breeze. You can use the `addClass()` method to add a CSS class to an element.
It's as simple as calling the method with the class name as an argument. For example, `$("p").addClass("highlight")` will add the `highlight` class to all paragraph elements.
The `addClass()` method is chainable, meaning you can string together multiple method calls. This can be useful when you need to perform multiple operations on an element.
CSS Basics
CSS classes are a fundamental part of web development, allowing you to define a style once and apply it to multiple elements across your website.
They ensure consistency and reduce repetition by enabling you to reuse the same style across different elements. For instance, a .highlight class can change the background color of an element to yellow.
You can apply this style to desired elements in your HTML by simply adding the class. This is a great way to style your website without having to repeat the same code over and over.
Vanilla JavaScript
Vanilla JavaScript is a great option for adding or removing classes from an element.
You can use the classList property of an element to achieve this, which returns a live DOMTokenList collection of the class attributes of the element.
The classList property is a live collection, meaning it updates dynamically as you add or remove classes.
This makes it easy to toggle classes on and off, which can be useful for responsive design or other interactive elements.
Adding and Removing Classes
Adding a class to an HTML element with jQuery is as simple as calling the .addClass() method. You can add multiple classes at once by passing multiple arguments to the method.
To remove a class, you can use the .removeClass() method, which also accepts multiple arguments. If a specified class doesn't exist on the element, it will be ignored, providing some built-in safety and no error will be thrown.
You can use the add method of classList to add multiple classes to an element. This method can accept multiple arguments, each one being a class you want to add. If a specified class already exists on the element, it will not be added again.
Removing multiple classes can be done using the remove method of classList. This method also accepts multiple arguments, each one a class to be removed. If a specified class does not exist on the element, it will be ignored.
The .attr() method in jQuery can be used to add or alter style properties to any element. This method allows you to dynamically change the style of an element during runtime.
The .addClass() method in jQuery will not replace any previously defined class, it simply adds another class to the element.
jQuery
If you're already using jQuery in your project, you can use its own methods for adding and removing classes, which provide a more concise syntax for class manipulation.
These methods include addClass() and removeClass(), which allow you to add or remove multiple classes at once. The addClass() method takes a single string argument with individual class names separated by spaces.
For example, to add classes to an element with the ID myElement, you would use the code: $( "#myElement" ).addClass( "class1 class2 class3" );. This code adds the classes class1, class2, and class3 to the element.
Unlike vanilla JavaScript, the addClass() method in jQuery doesn't require you to iterate over the classList or use the classList.add() method. This makes it a convenient choice if you're already using jQuery in your project.
The removeClass() method in jQuery also takes a space-separated string of class names, allowing you to remove multiple classes at once. For example, to remove classes from an element with the id "myElement", you would use the code: $( "#myElement" ).removeClass( "class1 class2 class3" );.
Use Cases and Applications
Adding dynamic behavior to websites is a common use case for manipulating CSS classes with JavaScript. This technique can be used to change the style of a navigation menu when the user scrolls down.
A navigation menu can change style by adding or removing classes that control its appearance. For example, adding a class to change the background color or font size.
Form validation is another common use case. You can add a class to an input field to change its border color if the user inputs an invalid email address.
Interactive web applications can also benefit from manipulating CSS classes. A button can have different styles depending on whether it's enabled or disabled by adding or removing classes.
Sources
- https://api.jquery.com/css/
- https://stackabuse.com/bytes/how-to-add-remove-multiple-classes-to-an-element-in-javascript/
- https://www.encodedna.com/jquery/add-important-style-using-jquery.htm
- https://www.bennadel.com/blog/2442-clearing-inline-css-properties-with-jquery.htm
- https://www.htmlgoodies.com/css/jquery-css-change/
Featured Images: pexels.com