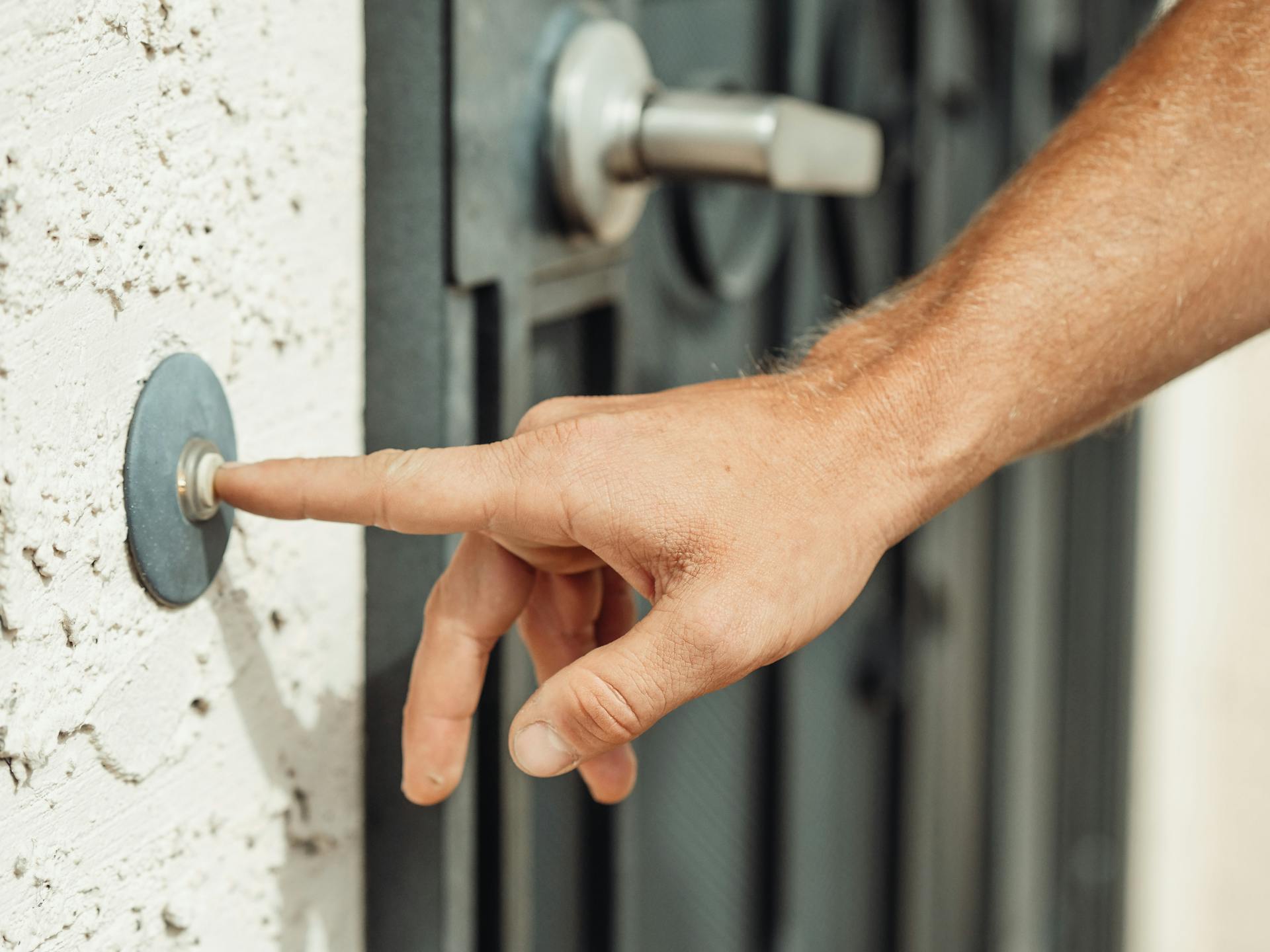
Changing a CSS class with JavaScript is a fundamental skill for any web developer. It allows you to dynamically update the appearance and behavior of HTML elements on your website.
You can use the `document.querySelector()` method to select an HTML element and then use the `classList` property to add or remove CSS classes. For example, `document.querySelector('.container').classList.add('active');` adds the `active` class to the element with the class `container`.
With this method, you can change the CSS class of an HTML element in a single line of code. This is particularly useful for responsive design, where you need to adapt the layout of your website based on different screen sizes.
Consider reading: Hide a Class Css
Prerequisites to JavaScript
To change a CSS class with JavaScript, you need to have a solid foundation in JavaScript basics. This includes understanding how to access and interact with the Document Object Model (DOM) using methods like getElementById, querySelector, and getElementsByClassName.
You should have a good grasp of JavaScript fundamentals, including variables, data types, functions, loops, and conditionals. This will help you write efficient and effective code.
To change an element's class based on user interactions, such as a click or mouseover event, you need to be familiar with event handling in JavaScript. This involves understanding how to attach event listeners and respond to events.
To manipulate the classList property, you'll need to use methods like add(), remove(), and toggle(). These methods are part of the DOM API and are essential for changing an element's class.
To get started, you'll need to learn about the following JavaScript methods:
- getElementById
- getElementsByClassName
- getElementsByTagName
- querySelector
- querySelectorAll
- getElementByName
Understanding the context in which to use querySelector and Parent/Child/Descendant Selectors will also be helpful in your journey to change a CSS class with JavaScript.
Changing an Element
Changing an element's class with JavaScript is a common technique used to dynamically update the appearance and behavior of elements on a web page.
The classList interface in JavaScript provides several methods for modifying an element's class attribute.
The classList interface offers methods for add, remove, toggle, and replace classes on elements.
For more insights, see: Inspect Element Javascript
This makes it easy to apply CSS styles or trigger specific behaviors associated with those classes.
The classList interface is a simple and efficient way to change an element's class.
The classList interface's methods allow developers to dynamically update the appearance and behavior of elements.
The classList interface is a powerful tool for modifying an element's class attribute.
Removing a CSS Class
Removing a CSS class is a straightforward process with JavaScript. You can use the classList.remove method to remove one or more CSS classes from the list of classes of the selected element.
This method provides a way to dynamically modify the class attribute of an element and consequently remove CSS styles or behavior associated with those classes. It won't produce an error if you try to remove a class that doesn't exist in the class list; it simply ignores the class name if it's not found.
To remove a single class, you can use the classList.remove method, like in the example where the class "box-style" is removed from the element with the ID "box." The element will no longer have the "box-style" class, and any associated CSS styles with that class will be removed.
Explore further: Css How to Override Style Class Using Stylesheet
You can also remove multiple classes by passing multiple class names to the classList.remove method, separated by commas. For instance, to remove the classes "class1" and "class2" from the element with the ID "box", you can use the classList.remove method like this.
Here are some examples of how to remove a single class and multiple classes using the classList.remove method:
Toggling a CSS Class
Toggling a CSS Class is a powerful technique that allows you to switch between different styles on an element with just one line of code.
The classList.toggle() method is the key to this technique. It adds or removes a CSS class from an element based on its current state. If the class exists, it's removed; if it doesn't, it's added.
You can use classList.toggle() to create interactive elements like dropdowns or buttons that change their appearance when clicked. For example, you can toggle the "highlight" class on an element when it's clicked.
Here are the parameters you can pass to classList.toggle():
- className: The string representing the name of the class to be toggled.
- force (optional): A Boolean value. If true, the class is added; if false, the class is removed.
The force parameter is useful when you want to override the default behavior of classList.toggle(). If you set force to true, the class will be added even if it already exists. If you set it to false, the class will be removed even if it doesn't exist.
For instance, you can use classList.toggle() to toggle the "new-style" class on an element with the ID "box" when a button is clicked.
Remember, classList.toggle() is a convenient way to simplify the process of adding or removing classes, which is a common workflow in styling scenarios.
Replacing a CSS Class
Replacing a CSS Class is a breeze with JavaScript. You can use the classList.replace method to swap one CSS class with another in the list of classes of the selected element.
The classList.replace method takes two parameters: oldClass and newClass. If the oldClass is not found in the class list, the method behaves like classList.add and simply adds the newClass.
On a similar theme: Changing Text Css
To replace a single class, you can use the classList.replace method like this: element.classList.replace('old-class', 'new-class'). This will replace the class "old-class" with the class "new-class" in the element.
If you want to replace a class conditionally, you can use a function that checks if the element has a certain class, and if it does, replaces it with another class. For example: if (element.classList.contains('new-style')) { element.classList.replace('new-style', 'highlight'); } else { element.classList.replace('old-class', 'highlight'); }
Here's a summary of the classList.replace method:
Setting CSS Styles
Setting CSS styles using JavaScript is a powerful technique that lets developers interact with HTML elements in an interesting way. JavaScript provides a bunch of methods to do this, all aimed at targeting specific elements in the Document Object Model (DOM).
You can change content, modify styles, and even update attributes by selecting elements. This becomes possible with element selection. Think about form validation, event handling, generating dynamic content, and getting real-time updates – all of these become possible with element selection.
There are two awesome ways to style your content using JavaScript: creating a style rule and having its selector target an element or elements, and directly setting a style on an element. A style rule would look as follows: An element that would be affected by this style rule could look like this.
Ignoring inline styles, the other approach involves JavaScript. We can use JavaScript to directly set a style on an element, and we can also use JavaScript to add or remove class values on elements which will alter which style rules get applied.
1. Adding and Removing
Adding and removing CSS classes with JavaScript is a breeze. The classList API provides a handful of methods to make working with class values easy.
You can add a class value to an element by calling the add method on its classList. For example, classList.add("className") adds the class "className" to the element. This method will not add a class if it already exists in the class list.
To add multiple classes, separate the class names with commas. So, classList.add("className1", "className2") adds both classes to the element.
Here's a summary of the add method:
You can remove a class value from an element by calling the remove method on its classList. For example, classList.remove("className") removes the class "className" from the element. This method will not produce an error if you try to remove a class that does not exist in the class list.
To remove multiple classes, separate the class names with commas. So, classList.remove("className1", "className2") removes both classes from the element.
Here's a summary of the remove method:
Remember, the classList API takes care of ensuring spaces are added between class values, so you don't have to worry about that.
Toggling a CSS Class
Toggling a CSS class is a fundamental concept in JavaScript that allows you to add or remove a class from an element with a single function call.
The classList.toggle method is a convenient way to toggle the presence of a CSS class in the list of classes of the selected element.
This method automatically determines whether to add or remove the class based on its current presence. If the class exists, it will be removed; if it does not exist, it will be added.
The classList.toggle method is particularly useful for creating elements that can be toggled on and off with a single function call, such as showing/hiding dropdowns or changing the appearance of an element based on user interactions.
The method takes two parameters: className and force. The className parameter represents the name of the class to be toggled, and the force parameter is an optional Boolean value that determines whether to add or remove the class.
Here's a summary of the classList.toggle method:
To use the classList.toggle method, simply call it on the classList of the element you want to toggle, passing in the name of the class and the force parameter if needed. For example, if you want to toggle the presence of the "highlight" class on an element, you can call classList.toggle("highlight") on the element's classList.
You might like: Css Text Highlight
Sources
- https://blog.replaybird.com/change-an-elements-class-with-javascript-js-add-remove-toggle-replace-contains/
- https://post.bytes.com/forum/topic/javascript/118988-change-css-class-property-using-javascript
- https://developer.chrome.com/docs/devtools/css
- https://www.kirupa.com/html5/setting_css_styles_using_javascript.htm
- https://www.geeksforgeeks.org/how-to-change-style-class-of-an-element-using-javascript/
Featured Images: pexels.com