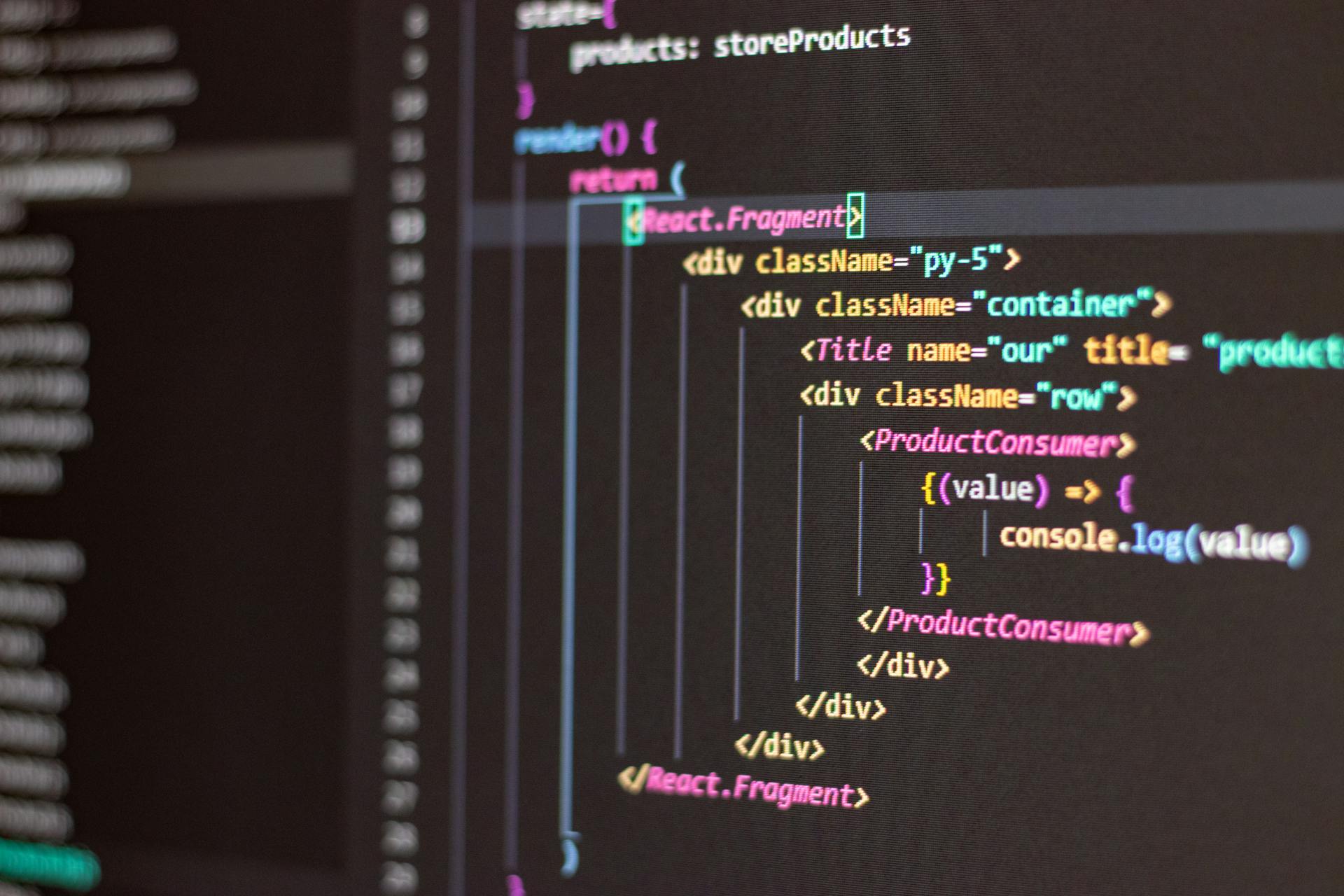
In a Next.js project, we need to create a reducer to manage the state of our application. A reducer is a pure function that takes the current state and an action, and returns a new state.
We'll use the `createSlice` function from the `@reduxjs/toolkit` package to create our reducer. This function takes an initial state and a reducer function as arguments.
To create a reducer, we need to define the initial state and the actions that can be performed on it. For example, if we're building a counter app, our initial state might look like this: `const initialState = { count: 0 };`.
Take a look at this: How to Use Reducer Api in Next Js 14
Setup Store
In the /redux folder, create a file called store.ts to configure the store. This is where we define the store and its associated types.
We export the store so it can be used in the Redux provider, which provides the store throughout the application. This is crucial for accessing the store in our components.
We also export RootState to inject the Redux state variables into our components easily. This helps ensure that our components are using the Redux store correctly.
Additionally, we export AppDispatch to use actions directly into our components using props. This allows us to dispatch actions from our components without worrying about the underlying implementation.
Finally, we export AppThunk, which represents a thunk action that can contain side effects. Thunk actions are often used with the redux-thunk middleware.
Here are the types we export in the store.ts file:
- store: The Redux store instance
- RootState: The root state type of the application
- AppDispatch: The dispatch type for the application
- AppThunk: The thunk action type for the application
These exports provide TypeScript types/interfaces for better type safety and code documentation in our application. They help ensure that our components and functions are using the Redux store, dispatch, and thunks in a type-safe manner, reducing the chance of runtime errors.
Connecting Store and Components
To connect your store and components in a Next.js TypeScript project, start by defining a component and gaining access to the store variables and actions. You'll need to add mapStateToProps and mapDispatchToProps to establish the connection between your component and the Redux store and actions.
Suggestion: Server Actions Nextjs
The mapStateToProps function is used to map the store's state to the component's props. To utilize this function, you'll need to export RootState from store.tsx. This is because TypeScript mandates providing variable types, and RootState is required to utilize the "state" variable.
MapDispatchToProps is used to map the store's actions to the component's props. To utilize this function, you'll need to export AppDispatch from store.tsx. This is because TypeScript mandates providing variable types, and AppDispatch is required to dispatch actions.
Once you have mapStateToProps and mapDispatchToProps defined, you can use the connect function from Redux to establish the connection between your component and the store. This will allow you to access the store's state and actions within your component.
In the component function, you can then use the destructured props to invoke the login action upon clicking the login button. This will update the loginInProgress state variable to true and subsequently set it to false once the login process is completed.
To integrate the Redux store into your application, you'll need to create a StoreProvider component and wrap your _app.tsx component with it. This will provide the store to your components.
In each of the reducers that you create, you'll need to add the HYDRATE action handler to the switch case. This action is triggered every time a new component that connects with Redux is opened, and it's used to sync the values that will stay in the Store state.
For more insights, see: Next Js Typescript Example Props
Once you've wrapped your _app.tsx component with the StoreProvider, you can create a component for a page and connect it with Redux using the connect function. This will allow you to access the store's state and actions within your component.
To get the static props from the wrapper, you'll need to place the following code in the component. This will inject the static props from the wrapper into your component.
You might enjoy: Nextjs Static Site
Managing State
Defining initial states is crucial in a Next.js application, as it helps the program run correctly on the first render. Create a new file called authInitialState.ts in the /initialStates folder to define the initial state, which is required when using TypeScript.
To update the state, you need to utilize the dispatch function to execute your actions. The dispatch function is used to update the state by passing the reducer function to it.
Here's a list of the steps to update the state:
- Utilize the dispatch function to execute your actions.
- Pass the reducer function to the dispatch function to update the state.
- For example, to update the floor field, you can use the updateFloor function with a number as the payload.
Defining Initial States
Defining initial states is a crucial step in setting up a Next.js application, helping the program run correctly on the first render.
In Next.js, you can create a new file called authInitialState.ts in the /initialStates folder to define the initial state.
Defining an interface for the initial state is necessary when using TypeScript, as seen in our example of authentication.
In this file, you can define the structure of your initial state, ensuring it's correctly formatted for your application.
A well-defined initial state helps prevent errors and ensures your application functions as expected from the start.
If this caught your attention, see: Nextjs Server Actions File Upload
Updating the State
To update the state, we need to utilize the dispatch function to execute our actions. This involves utilizing the reducer functions, such as updateFloor and updateUnit, to make changes to the state.
The updateFloor function, for instance, requires a number as its payload. So, to update the floor field, we would use updateFloor(5).
In our React component, we can create a function to execute this action, which we would then pass to the dispatch function to affect the store.
Check this out: Next Js State Management
We can also update the state by using the dispatch function to execute actions, such as login and logout, which are defined in the actions file. This involves utilizing the action creators, such as login and logout, to make changes to the state.
To update the state, we need to make use of the reducer functions, which are defined in the reducers object. This allows us to update the state in a controlled and predictable way.
The reducer functions, such as updateFloor, are designed to update specific fields in the state. By using these functions, we can ensure that the state is updated correctly and consistently.
In our MyComponent.tsx file, we can create a function to execute the updateFloor action, which would then be passed to the dispatch function to update the state. This allows us to decouple the action from the dispatch function, making the code more modular and reusable.
By utilizing the dispatch function and the reducer functions, we can update the state in a controlled and predictable way, ensuring that the application behaves as expected.
You might like: Using State in Next Js
Sources
- https://medium.com/@himanshu.sharma.for.work/setting-up-redux-in-next-js-with-typescript-65eda6decdbf
- https://victorbruce82.medium.com/setup-redux-in-your-nextjs-application-with-typescript-dcbbb60c3e96
- https://dev.to/juniorbatistadev/how-to-use-nextjs-13-with-redux-toolkit-and-typescript-2anb
- https://dev.to/dguglielmigit/redux-toolkit-with-typescript-and-nextjs-14-4k0c
- https://github.com/felipemeriga/next-typescript-redux-template
Featured Images: pexels.com