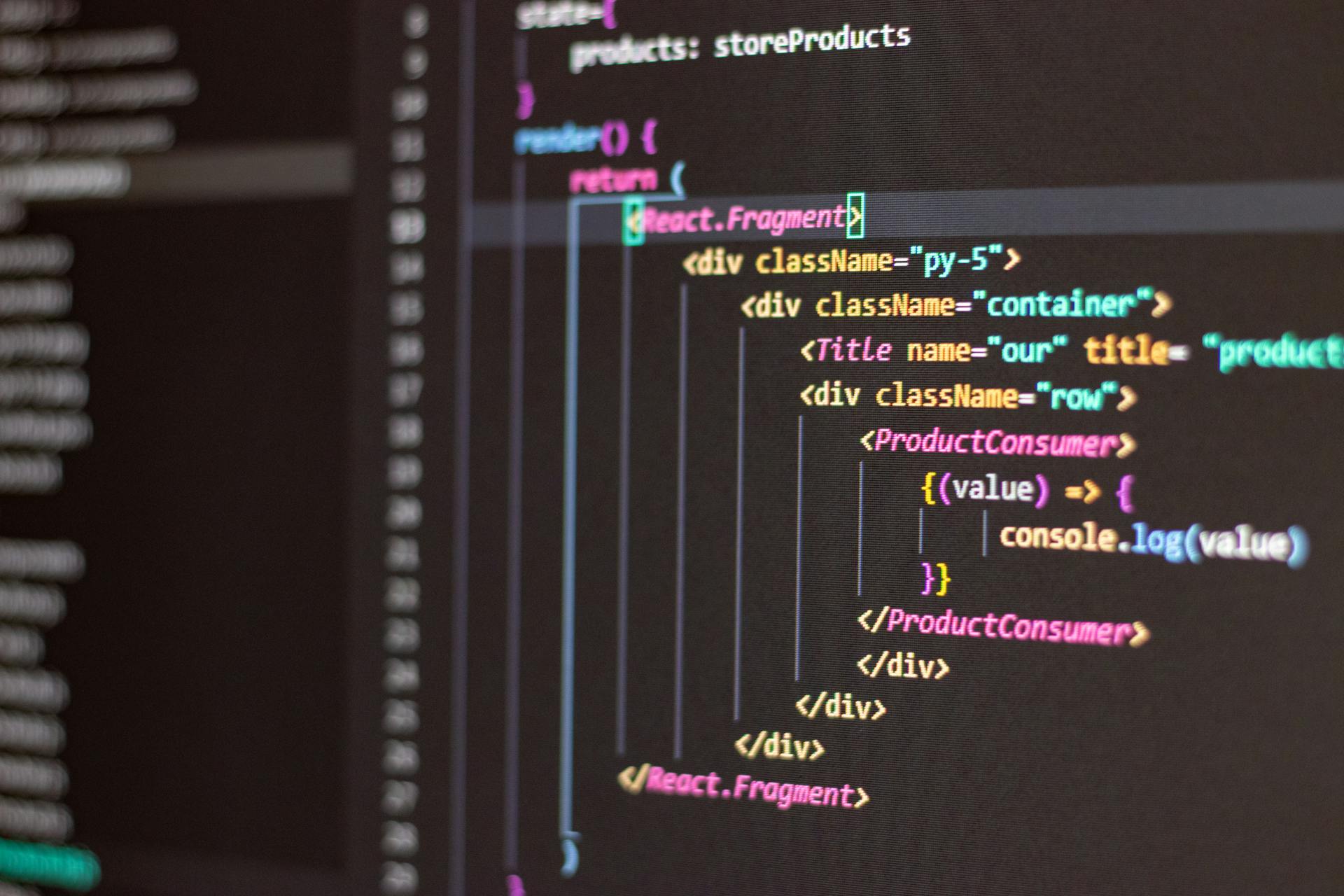
Enabling strict mode in Next Js can significantly improve performance by catching type errors at compile time.
Strict mode helps catch type errors at compile time, preventing runtime errors and improving overall performance.
By default, Next Js uses a hybrid mode that is a mix of strict and loose modes. To enable strict mode, you need to add the 'strictMode' option in the next.config.js file.
Strict mode is enabled by default in Next Js version 12 and later.
Strict Mode Warnings
Strict Mode in Next.js warns you about potential issues in your application. It's like having a personal quality control manager that checks your code for you.
One of the main warnings you'll see is related to deprecated APIs. These are older ways of doing things that are no longer recommended.
- findDOMNode is deprecated and should be replaced with a different approach.
- UNSAFE_ class lifecycle methods like UNSAFE_componentWillMount are also deprecated and should be replaced.
- Legacy context (childContextTypes, contextTypes, and getChildContext) is error-prone and will be removed in a future major version.
- Legacy string refs (this.refs) are also deprecated and should be replaced with a different approach.
You can enable Strict Mode for a specific part of your application, like a Header or Footer component, without affecting the rest of your app.
React 16.3 introduced a new way to manage refs called createRef, which is a replacement for the legacy string ref API. This new API is more convenient and doesn't have the downsides of the old one.
If you're using legacy context API, you'll see a warning message in Strict Mode. This is because the new context API is more robust and will be the recommended way of doing things in the future.
You can learn more about the new context API and how to migrate to it by reading the documentation.
You might like: Nextjs Usecontext
Detecting and Fixing Issues
Strict mode in Next.js helps you detect and fix issues early on, making your development process more efficient. It does this by intentionally double-invoking certain methods, making it easier to spot problems.
This includes class component constructor, render, and shouldComponentUpdate methods, class component static getDerivedStateFromProps method, function component bodies, state updater functions, and functions passed to useState, useMemo, or useReducer. These methods will be double-invoked in development mode only.
Broaden your view: Next Js Development Company
In React 18, React DevTools will dim the logs from the second call, and you can also choose to suppress them completely.
Strict mode can also help find bugs in Effects. Every Effect has some setup code and may have some cleanup code. React will run one extra setup+cleanup cycle in development for every Effect, which can help reveal subtle bugs that are hard to catch manually.
Here are some common issues that strict mode can help you detect:
- Missing cleanup logic in Effects
- Incorrect usage of legacy string ref API
- Unsafe lifecycles in class components
To fix these issues, you can follow these steps:
- Add cleanup logic to your Effects
- Replace legacy string ref API with the new createRef API
- Remove unsafe lifecycles from your class components
By following these steps and enabling strict mode in your Next.js app, you can catch issues early on and make your development process more efficient.
Enabling and Configuring Strict Mode
You can enable Strict Mode for specific parts of your application, giving you more control over where checks run.
Strict Mode will not run against components like Header and Footer, but it will run on Sidebar and Content, as well as all components inside them.
This means you can choose which parts of your app to prioritize for optimization and error checking.
Check this out: How to Run Nextjs to Build
Resolving Deprecation Warnings
You're likely to see deprecation warnings in your Next.js app when using Strict Mode. findDOMNode is one of the deprecated APIs that can cause warnings.
To fix deprecation warnings, you can replace findDOMNode with a ref passed to your custom component and then forwarded to the DOM using ref forwarding.
This approach makes it explicit and avoids refactoring hazards. Alternatively, you can add a wrapper DOM node in your component and attach a ref directly to it.
If you're getting warnings about UNSAFE_ class lifecycle methods, you can replace them with alternative methods. Legacy context is another deprecated API that can cause warnings, and you can replace it with the new context API.
Legacy string refs can also cause warnings, and you can replace them with the new ref API.
You might like: Using State in Next Js
Featured Images: pexels.com