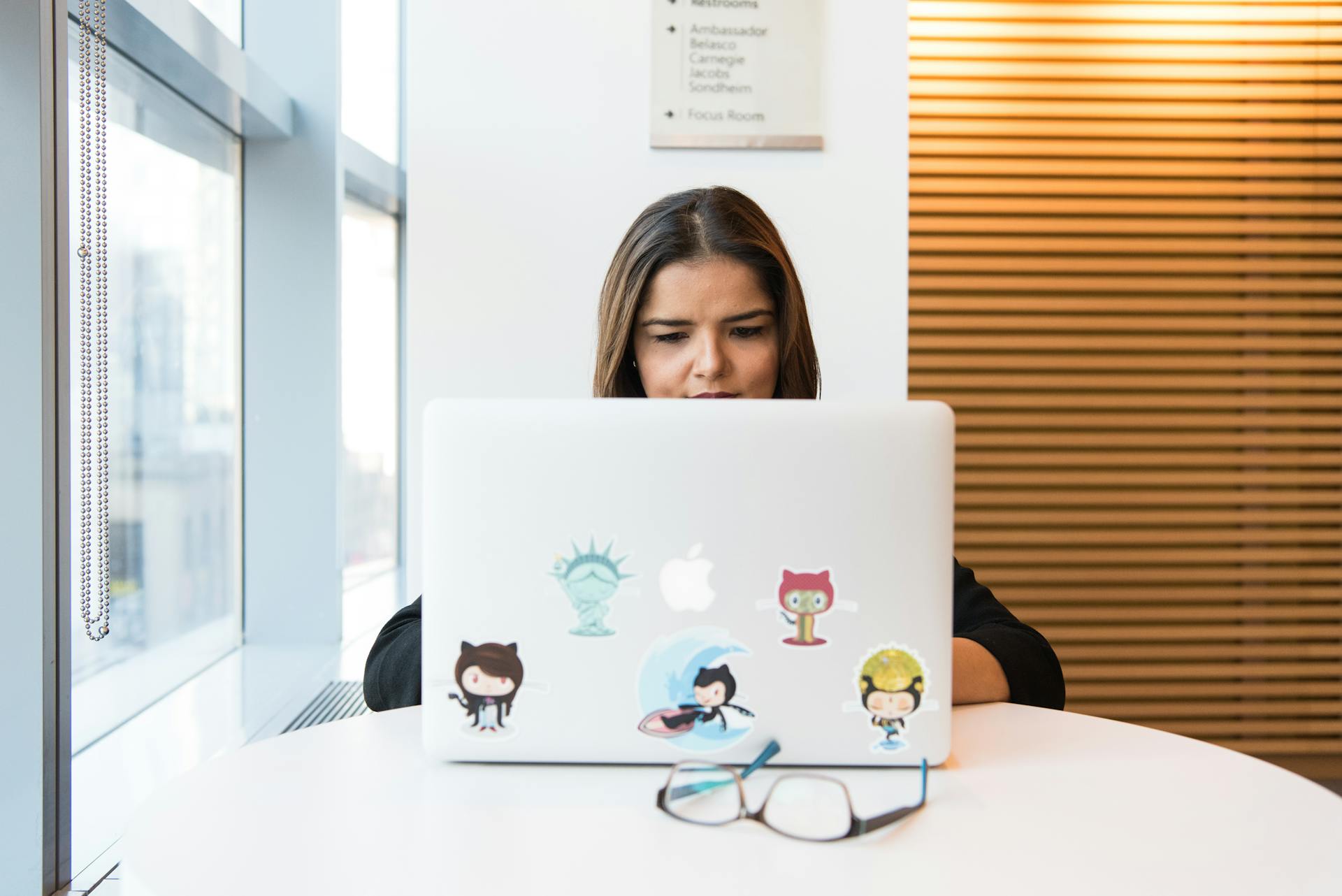
To run Next.js, you'll need to install it as a dependency in your project. This can be done using npm or yarn by running the command `npm install next` or `yarn add next`.
Next.js is built on top of React, so if you're already familiar with React, you'll find it relatively easy to pick up. The Next.js documentation provides a comprehensive guide to getting started.
To create a new Next.js project, you can use the `create-next-app` command, which will set up a basic project structure for you. This includes the necessary configuration files and a basic layout for your application.
With Next.js, you can use the `next build` command to build your project for production, which will optimize your code and bundle it for deployment. This is especially useful for large applications where performance is critical.
Readers also liked: Installing Next Js
What Is Nextjs
Next.js is a robust open-source framework built on React, a popular JavaScript library for developing interactive user interfaces.
Next.js has grown in popularity among developers and businesses due to its out-of-the-box functionalities, great performance, and seamless developer experience.
It makes it simple for developers to create modern, scalable online apps, such as static sites, server-rendered pages, and single-page applications.
Next.js' focus on simplicity and developer productivity is one of its primary strengths.
The framework automates several complex web development activities, including server-side rendering, static site building, and code splitting.
Next.js also has built-in support for API routes, dynamic imports, and incremental static regeneration, all of which considerably speed up the development process.
As a result, Next.js has emerged as the go-to framework for developers seeking to build high-performance online applications with little configuration and setup.
Here's an interesting read: Hire Next Js Developer
Setting Up Nextjs
To set up Next.js, you'll need to start by creating a new project using the `npx create-next-app` command in your terminal.
Next.js uses a server-side rendering (SSR) approach by default, which means it will render pages on the server before sending them to the client's web browser.
First, navigate to your project directory in the terminal and run `npm install` to install the required dependencies.
Next.js uses a file-based routing system, which means you can create pages in a directory structure that mirrors your app's URL structure.
To get started with routing, create a new file called `pages/index.js` and add a simple React component to it.
Suggestion: Next Js App Router Project Structure
Creating a Project
Creating a Next.js project is a straightforward process. You can create a new project using the command `npx create-next-app your-app-name` or `yarn create next-app your-app-name`.
To create a new project, you'll need to navigate to the directory where you want to create your project. You can do this by running `cd your-desired-directory` in your terminal or command prompt.
If you don't want to create a new directory, you can create a new Next.js app in the current working directory by running `npx create-next-app ./.` or `yarn create next-app ./`.
Here are the steps to create a new Next.js project:
Broaden your view: Next Js Src Directory
1. Open your terminal or command prompt.
2. Navigate to the directory where you want to create your project.
3. Run `npx create-next-app your-app-name` or `yarn create next-app your-app-name`.
4. Change to your new project directory by running `cd your-app-name`.
That's it! You now have a new Next.js project set up and ready to go.
Recommended read: Create Next Js App
Running Nextjs
To run Next.js, you can use a development server that comes with the framework. This server supports hot module replacement, which means you can see your changes in real-time without having to manually reload the page.
To serve your Next.js app, open your terminal or command prompt, and navigate to the root directory of your project. Run the command `npm run dev` or `yarn dev`, depending on your preferred package manager. This command will start the development server on a default port, usually 3000.
You can also use other commands to serve your Next.js app, such as `nx dev my-new-app` or `bun --bun run dev`. These commands will start the server at `http://localhost:3000` by default.
Here are some common commands to run Next.js:
- `npm run dev` or `yarn dev`
- `nx dev my-new-app`
- `bun --bun run dev`
These commands will start the development server and serve your Next.js app at `http://localhost:3000`.
Running Locally
To run your Next.js app locally, you need to start the development server. This can be done by running the command npm run dev or yarn dev in your terminal or command prompt.
The development server will start on a default port, usually 3000. You can then visit your Next.js app by going to http://localhost:3000 in your web browser.
To stop the development server, simply press Ctrl+C in your terminal or command prompt. This will allow you to make changes to your code and see the results in real-time in your browser.
You can also serve your Next.js application for development using the command nx dev my-new-app. This will start the server at http://localhost:3000 by default.
To serve your Next.js application for production, you can use the nx command, but the exact command is not specified in the article.
To run your Next.js build locally, you can use the development server. First, add a new script to your package.json file called "start": "next start". Then, run the start script using the command npm run start or yarn start.
If this caught your attention, see: Nextjs Script Async
App with Bun
To build an app with Next.js and Bun, initialize a Next.js app with create-next-app, which will scaffold a new Next.js project and automatically install dependencies.
You can start the dev server with Bun by running `bun --bun run dev` from the project root. This will hot-reload your code in the browser as you make changes.
To run the dev server with Node.js instead, simply omit the `--bun` flag.
Open http://localhost:3000 with your browser to see the result, and any changes you make to `pages/app/index.tsx` will be hot-reloaded in the browser.
You can use various frameworks and libraries with Bun, such as EdgeDB, Prisma, and Drizzle ORM, to build a robust and scalable app.
To run Bun as a daemon with PM2, use the `pm2` command with the `bun` executable.
If you're looking to deploy your Bun application on Render, you can follow the instructions outlined in the article section.
Remember to add dependencies, trusted dependencies, and development dependencies as needed to your project.
If this caught your attention, see: Next Js Bun
Debugging and Deployment
Debugging and Deployment are two crucial steps in building a Next.js application. If you experience problems while executing your Next.js build locally, you must understand how to debug them efficiently.
To debug your Next.js build, check your browser's developer console for error messages or warnings. Review the terminal or command prompt output for any error messages or stack traces. Ensure that all dependencies are installed and up to date by running npm install or yarn install. Verify that your build configuration in next.config.js (if present) is correct and compatible with your local environment.
Here are some common debugging methods to keep in mind:
- Check your browser's developer console for error messages or warnings
- Review the terminal or command prompt output for any error messages or stack traces
- Ensure that all dependencies are installed and up to date
- Verify your build configuration in next.config.js (if present)
After debugging, you're ready to deploy your Next.js application. You can choose any hosting provider that fits your needs, but AWS Amplify supports deploying both SSR and SSG Next.js apps without any additional configuration.
Debugging Your
Debugging Your Next.js build can be a challenge, but it doesn't have to be. To start, check your browser's developer console for error messages or warnings that may provide clues about the issue.
Reviewing the terminal or command prompt output is also crucial. Look for any error messages or stack traces that might indicate what's going wrong.
Make sure all dependencies are installed and up to date by running npm install or yarn install. This simple step can often resolve issues.
Verify that your build configuration in next.config.js (if present) is correct and compatible with your local environment. This will help you catch any configuration-related errors.
Here are some key steps to follow:
- Check your browser's developer console for error messages or warnings.
- Review the terminal or command prompt output for any error messages or stack traces.
- Ensure that all dependencies are installed and up to date.
- Verify that your build configuration in next.config.js is correct.
Deployment
Deployment is a crucial step in getting your Next.js app live online. You have the freedom to choose any hosting provider that fits your needs.
AWS Amplify supports deploying both SSR and SSG Next.js apps without any additional configuration on your end. If you're creating a statically generated Next.js app, go to your package.json file and change your build script to next build && next export.
To deploy your app, follow these simple steps:
- The build settings will auto-populate, and so you can just click next on the Configure build settings
- Click Save and deploy.
You also have the option to deploy your app to Vercel, the hosting platform developed in tandem with Next.js. This offers a great overall developer and user experience.
To deploy to Vercel, you can use the vercel CLI. Start by installing the CLI and running it: The command above installs the CLI, then walks you through the process of logging in to Vercel, creating the app, and deploying it.
Intriguing read: Nextjs by Vercel Meaning
Frontend Development
Building the frontend involves creating the code that renders a form, which posts data to the server-side endpoint. This endpoint runs the model with Replicate and returns a prediction.
To get started, you'll need to create a file called app/page.js that renders the default "Welcome to Next.js" home route. Remove all the existing content in this file and replace it with specific code.
Curious to learn more? Check out: Next Js Directory Structure
Testing in Browser
Testing your frontend app in a browser is a crucial step in the development process. You can test your Next.js build locally by opening your preferred web browser.
Navigate to the URL provided by the start command, usually http://localhost:3000. This is where you'll see your Next.js app running locally.
You can interact with it just like you would with a live deployment, exploring your app, testing its functionality, and ensuring that everything works as planned.
Add Basic Styles
As you start building your frontend, it's essential to create a clean slate for your styles. Remove all the content in app/globals.css.
The Next.js starter app includes some CSS styles, but they're not intended for reuse in a real app.
Replace the existing content with basic styles to establish a solid foundation for your project. The basic styles to add are the ones mentioned in the Next.js starter app documentation.
These styles will help you create a clean and organized structure for your app.
Recommended read: Production Ready Starter Next Js
Link Component
The Link component is a powerful tool in Next.js that allows for client-side route transitions, making page transitions smoother for users. This means you can use it to navigate between pages without a full page reload.
To use the Link component, you simply replace regular anchor tags with it in your code.
You can use the Link component in place of a tag, making it a direct replacement for traditional navigation links.
For more insights, see: What Is Link Building in Search Engine Optimization
Generating Pages and Components
You can quickly generate new pages and components for your application using Nx's commands.
Nx provides a command to generate a new page, nx g @nx/next:page, which adds a new page to your project in the specified directory.
To generate a new page with tests, you can pass the --withTests option, like this: nx g @nx/next:page --withTests.
Nx also generates components with tests by default, so you don't need to specify anything extra.
You can generate a new component using the command nx g @nx/next:component, which adds a new component to your project in the specified directory.
The new component will have tests created for it automatically, so you can start working on it right away.
Nx makes it easy to add new pages and components to your application, saving you time and effort in the development process.
Take a look at this: New Nextjs Project Typescript
Featured Images: pexels.com