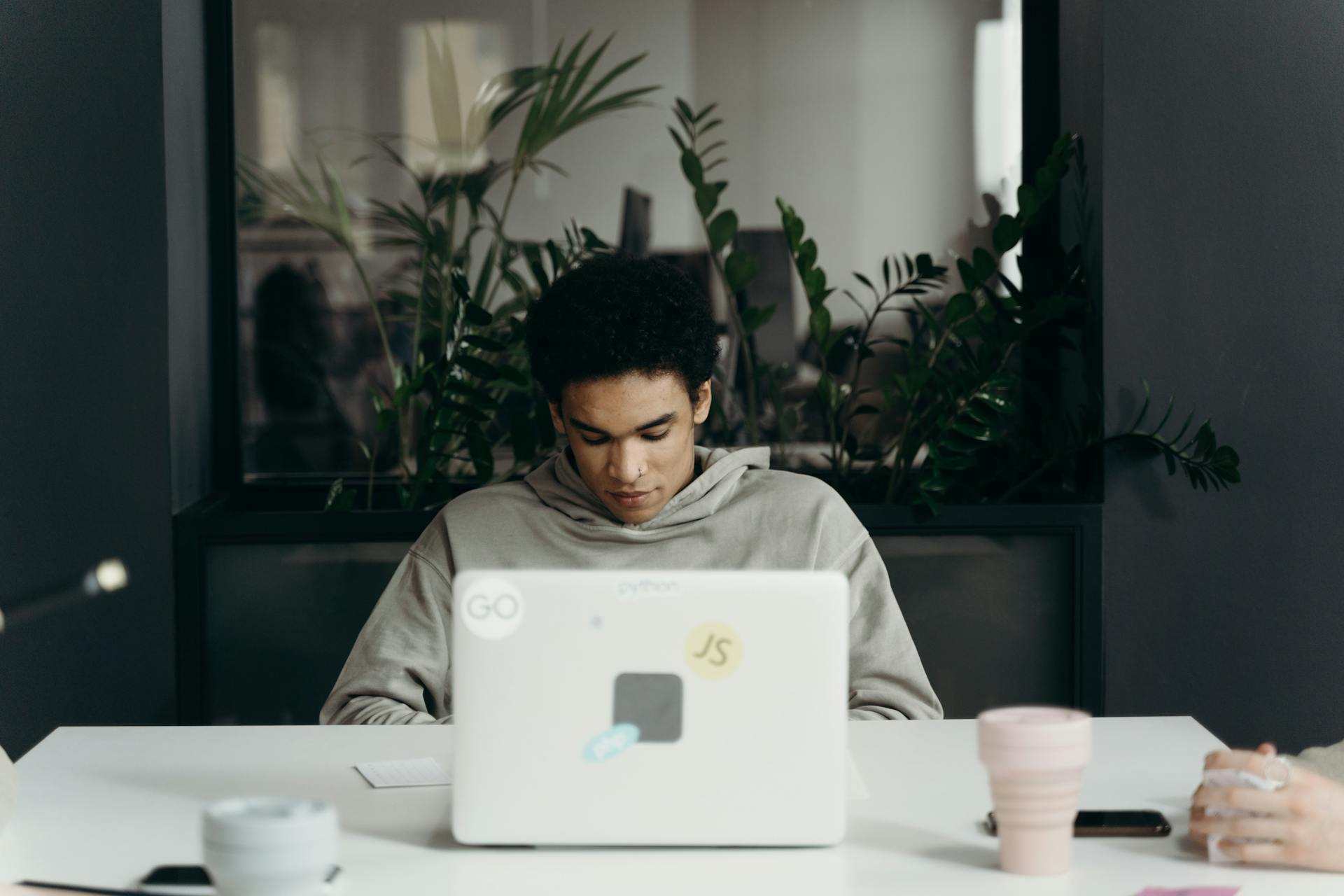
Having a production-ready starter Next.js for real-world projects is crucial for developers who want to build scalable and maintainable applications. This starter kit includes a robust configuration for internationalization, which allows for easy translation of text and dates.
The starter kit also includes a robust authentication system, which uses Next.js's built-in API routes and OAuth 2.0 to handle login and registration functionality. This system is customizable and can be easily integrated with popular authentication services like Google and Facebook.
With this starter kit, you can quickly set up a project that is ready for production, without having to spend hours configuring and setting up individual components. This is especially helpful for developers who are new to Next.js or are working on a tight deadline.
Related reading: Next Js Cms
Getting Started
To get started with a production-ready starter Next.js app, you'll want to initialize a new app using create-next-app. This will create a basic Next.js project with all the necessary files and configurations.
If this caught your attention, see: Cookies Nextjs
Create a new app by running the command `create-next-app supabase-nextjs` in your terminal. This will create a new Next.js project with the name `supabase-nextjs`.
Next, create a `.env.local` file at the root of your project and paste the API URL and anon key that you copied earlier from Supabase. This will store your environment variables securely.
If this caught your attention, see: File Upload Next Js Supabase
Initialize an App
To initialize a Next.js app, use create-next-app to create a new app called supabase-nextjs. This command will get you started with a basic Next.js project.
You can run npm run dev to start the development server, which will allow you to see your app in action. This command is a great way to test and refine your app before moving it to production.
Next, you'll want to save your environment variables in a .env.local file. This file should be created at the root of your project and contain the API URL and anon key that you copied earlier.
See what others are reading: Create Next Js App
What Does 'Next' Mean?
So you're wondering what 'Next' means in the context of web development? Next.js is a popular framework for building server-rendered, statically generated, and performance-optimized websites and applications.
Next.js is specifically designed for React, which is a JavaScript library for building user interfaces. Next.js simplifies the process of building server-side rendered applications.
Next.js provides a lot of built-in features, including automatic code splitting, server-side rendering, and static site generation. This means you can focus on writing code without worrying about the underlying infrastructure.
To run a Next.js application in production mode, you need to use the 'next start' command. This command requires you to first run 'next build' to bundle all the necessary assets.
You might like: How to Run Next Js App
Authentication
Authentication is a crucial aspect of any production-ready application, and Next.js makes it easy to implement. Next.js is a highly versatile framework that offers pre-rendering at build time (SSG), server-side rendering at request time (SSR), API routes, and middleware edge-functions.
To integrate authentication with your Supabase project, you can use the @supabase/ssr package. This package has all the functionalities to quickly configure your Supabase project to use cookies for storing user sessions.
Next.js Server-Side Auth guide provides more information on how to use this package effectively.
Suggestion: Nextjs App Route Get Ssr Data
Supabase Utilities
In a production-ready Next.js starter, Supabase utilities play a crucial role in accessing Supabase from both client and server components.
You'll need to create two essential utilities files: client.js and server.js, and organize them within the utils/supabase directory at the root of your project.
These utilities files will provide the necessary functionalities for client-side and server-side Supabase access.
To create these utilities files, you'll need to follow the recommendations outlined in the example code, which includes creating the following files with specific functionalities.
Here's a summary of the required functionalities for each file:
- Client Component client - To access Supabase from Client Components, which run in the browser.
- Server Component client - To access Supabase from Server Components, Server Actions, and Route Handlers, which run only on the server.
By following these guidelines and organizing your utilities files correctly, you'll be well on your way to setting up a robust Supabase integration in your Next.js starter.
Middleware
Middleware plays a crucial role in ensuring your Next.js app is production-ready.
To refresh expired Auth tokens and store them, you'll need middleware that accomplishes three things: refreshing the Auth token with a call to supabase.auth.getUser, passing the refreshed token to Server Components through request.cookies.set, and passing it to the browser through response.cookies.set.
Readers also liked: Middleware Next Js
You can add a matcher to run the middleware only on routes that access Supabase, which is a good practice. For more information, check out the documentation.
Protecting pages requires careful consideration, as the server gets the user session from cookies, which can be spoofed by anyone.
Always use supabase.auth.getUser() to protect pages and user data, as it sends a request to the Supabase Auth server every time to revalidate the Auth token, making it safe to trust.
Here are the steps to create middleware:
- Create a middleware.js file at the project root.
- Create another middleware.js file within the utils/supabase folder.
- The utils/supabase file contains the logic for updating the session, which is used by the middleware.js file.
Never trust supabase.auth.getSession() inside server code such as middleware, as it isn't guaranteed to revalidate the Auth token.
See what others are reading: Nextjs Middleware Firebase Auth
File Upload
In a production-ready Next.js application, file uploads are a crucial feature. We can create an upload widget using the example of an avatar widget that allows users to upload a profile photo.
To start, we create a new component, just like we did in the avatar widget example. This component will handle the file upload functionality.
Recommended read: Next Js Typescript Example Reducer
A file upload widget typically includes a form with an input field that accepts file uploads. We can use the `input` element with a `type` attribute set to `file` to create this form.
The `onChange` event handler is used to handle the file upload, just like in the avatar widget example. This event handler will be responsible for processing the uploaded file.
When a file is uploaded, we can use the `FileReader` API to read the file contents. This API is used in the avatar widget example to display the uploaded image.
By following these steps, we can create a file upload widget that is production-ready and integrates seamlessly with our Next.js application.
See what others are reading: Next Js Upload File
Setup and Configuration
To get your Next.js project ready for production, you'll want to set up ESLint to catch errors early. Run the yarn lint command to install eslint and eslint-config-next as dependencies.
ESLint will also create an .eslintrc.json file with default settings, including a rule that warns against using the Script tag without an id attribute inside JSX.
You can customize the Next.js start command with options like specifying a custom port or hostname. For example, you can use the -p option to run the server on a specific port, or the -H or --hostname option to set a custom hostname.
A different take: Next Js Linter
Requires Running First
To get your Next.js app up and running, you'll need to run the next build command first.
This step bundles and optimizes all the necessary assets for production.
The full workflow is actually quite straightforward: develop your app with npm run dev, build production assets with next build, and then start the production server with next start.
Here's a quick rundown of the process:
- Develop your app with npm run dev
- Build production assets with next build
- Start the production server with next start
next start won't work until you've run next build, so make sure to do that first.
5. Setup ESLint
Setting up ESLint is a crucial step in catching errors early. You'll want to run the existing yarn lint command to see if it's already set up.
If you don't have a yarn lint command, you'll need to add it. This will install ESLint and eslint-config-next as dependencies.
An .eslintrc.json file will be created with a specific warning about using the Script tag without an id attribute inside JSX.
This is a common error that ESLint can help you avoid.
For your interest: Nextjs Eslint 9
Custom Config Options
Custom Config Options are a great way to tailor your setup to your needs. You can specify a custom port to run the server on.
The next start command has some nice customization options. For example, you can use the -p flag to specify a custom port.
You can also use the -H or --hostname flag to set a custom hostname. This is the same as using the -H flag.
Here are the custom config options in a nutshell:
- -p - Specify custom port to run the server on
- -H - Set a custom hostname
- --hostname - Same as above
Checklist
Before you start setting up your system, make sure you have the following:
First, gather all the necessary hardware components, such as the main unit, power supply, and any additional peripherals.
Have a clear understanding of the system's architecture and how the different components interact with each other.
Check the system's documentation for specific setup instructions and requirements.
Verify that the system's firmware is up-to-date and compatible with the hardware and software components.
Ensure that the system's power supply is sufficient to handle the load of all the connected devices.
Make sure all cables are securely connected and not damaged.
Double-check the system's configuration settings to ensure they are set correctly.
For more insights, see: How to Check Nextjs Version
Static Site Generation
Static Site Generation is a powerful feature in Next.js starters. It allows you to build static HTML pages at build time, resulting in extremely fast page loads.
This is achieved through the use of getStaticProps, a function that fetches data at build time and generates static HTML pages. For example, you can use getStaticProps to fetch data for a React component, like this:
Static Site Generation is particularly useful for SEO, as it allows search engines to index your pages more easily.
With Next.js starters, you can quickly integrate popular tools like Styled Components, Redux, and React Query, and get started with Static Site Generation right away.
Here are some key benefits of using Static Site Generation with Next.js starters:
- Fast page loads: Static HTML pages load extremely fast, making for a better user experience.
- Improved SEO: Search engines can index your pages more easily, improving your website's visibility.
- Easy integration: Next.js starters make it easy to integrate popular tools and libraries.
Routing and API
Routing in Next.js is incredibly straightforward. You can create pages by simply creating React component files, and they'll automatically be available at the corresponding URL.
For example, if you create a file called pages/about.js, it's instantly available at /about. This file-system based routing makes it easy to organize and manage your pages.
API routes are also a breeze to set up. You can create backend API endpoints with zero extra configuration by simply creating files under the /pages/api directory.
Take a look at this: Next Js Pages
Built-in Routing
Next.js has a built-in routing system that makes it easy to create pages by simply creating React component files. This means you can create a page like about.js and it's automatically available at /about.
With file-system based routing, you don't need to configure a separate routing system, it's all taken care of by Next.js. This approach is straightforward and makes it easy to set up routing in your application.
By creating a new file for each page, you can quickly add new routes to your application without having to write a lot of code.
A unique perspective: Creating Shop Page Next Js
API Routes
API Routes are a powerful tool in Next.js, allowing you to create backend API endpoints with ease.
In Next.js, you can create API routes by simply creating files under the /pages/api directory. No extra configuration is required.
This makes it easy to get started with API development, even if you're new to it. I've seen many developers quickly create and deploy API routes using this method.
You can create API routes in a matter of seconds by creating a new file under /pages/api and writing your API endpoint code inside it.
For example, if you create a file called myapi.js under /pages/api, you can define an API endpoint like this:
Just create files under /pages/api to start creating backend API endpoints in Next.js.
You might like: Nextjs App vs Pages
Sources
- https://supabase.com/docs/guides/getting-started/tutorials/with-nextjs
- https://nextjs.org/docs/app/building-your-application/deploying/production-checklist
- https://dev.to/jobyjoseph/setup-production-ready-nextjs-app-5a2a
- https://nextjsstarter.com/blog/next-starter-code-examples-for-immediate-use/
- https://blog.stackademic.com/future-web-with-production-ready-next-js-applications-44aa6079679a
Featured Images: pexels.com