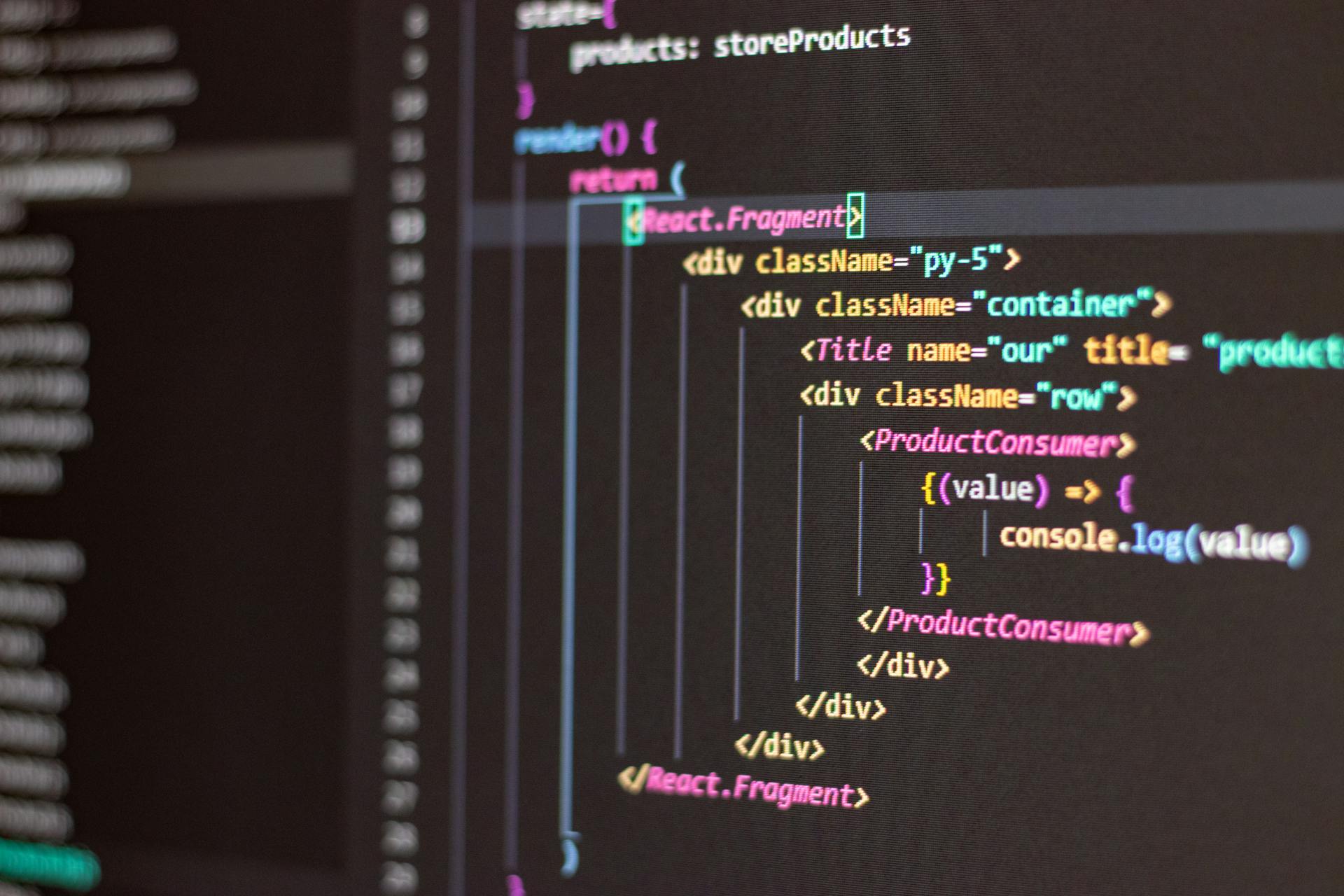
To set up ESLint with Next.js, TypeScript, and Tailwind, we need to create a new Next.js project using the `create-next-app` command with the `--ts` flag to enable TypeScript support.
In the project directory, we can initialize a new ESLint configuration using the `npx eslint --init` command, which will guide us through the setup process.
Next, we need to install the required ESLint plugins, including `@typescript-eslint/parser` and `eslint-plugin-tailwindcss`, to support TypeScript and TailwindCSS respectively.
We can then configure ESLint to use the `@typescript-eslint/parser` parser and enable the `tailwindcss` rule in the ESLint configuration file.
Check this out: New Nextjs Project Typescript
Install Prettier
To install Prettier, you'll need to install it along with some useful plugins. Prettier automatically formats your code, making it a great addition to your Next.js project.
Here's a list of the packages you'll need to install:
- eslint: Lints your JavaScript/TypeScript code.
- prettier: Automatically formats your code.
- eslint-config-prettier: Disables ESLint rules that would conflict with Prettier.
- eslint-plugin-prettier: Integrates Prettier into ESLint so that Prettier errors show up as ESLint errors.
You can install these packages using npm by running the following command: npm install --save-dev eslint-config-prettier.
A fresh viewpoint: Next Js Npm Install
Configuring Prettier
Configuring Prettier is a crucial step in maintaining clean and consistent code quality in your Next.js project. To set up Prettier, you'll need to install it along with some useful plugins.
You might like: Next Js Prettier
Install ESLint, Prettier, and the necessary plugins to get them working together. You'll need eslint, prettier, eslint-config-prettier, and eslint-plugin-prettier.
Once you've installed the necessary plugins, you can configure Prettier in your ESLint setup. Add "prettier" to the END of the extends property in your ESLint config, like this: "extends": ["next/core-web-vitals", ..., "prettier"]. This switches off ESLint rules that conflict with Prettier's code formatting.
A fresh viewpoint: Nextjs Eslint 9
Override Version
You can override the ESLint version used by your dependencies by modifying your package.json file. This can silence conflicts that arise from different ESLint versions.
To do this, run npm install to confirm the issue is fixed. ESLint 9 is properly installed after running npm install.
However, running the linter with npm run lint will still produce errors. These errors are due to the Next.js integrated ESLint configuration when using next link.
Explore further: Npm Next Js
Configure
To configure Prettier, you need to modify the .eslintrc.json file to integrate Prettier. Update it to include the "prettier" rule and display formatting issues as ESLint errors.
You can also use the ESLint config from the eslint-config-prettier package. This package disables ESLint rules that conflict with Prettier. To use it, install the package with npm install --save-dev eslint-config-prettier.
In the ESLint config, add "prettier" to the end of the "extends" array, like this: "extends": ["next/core-web-vitals", ..., "prettier"]. This config will switch off ESLint rules that conflict with Prettier.
Here's a summary of the steps:
- Install eslint-config-prettier with npm install --save-dev eslint-config-prettier
- Add "prettier" to the end of the "extends" array in your ESLint config
By following these steps, you'll be able to integrate Prettier into your ESLint config and start formatting your code automatically.
Setup for Tailwind
To set up Tailwind with ESLint, you'll need to install the eslint-plugin-tailwindcss package. Run npm install --save-dev eslint-plugin-tailwindcss in your terminal.
In your ESLint config, add "plugin:tailwindcss/recommended" to the end of the extends list. This will enable the recommended configuration provided by the plugin.
You'll also need to add "tailwindcss" to the plugins object and a rules object to disable the rule for checking the sequence of Tailwind class names. The rule is named tailwindcss/classnames-order.
See what others are reading: Next Js Tailwind Spinner Loading Page
Here's a summary of the steps:
- Install eslint-plugin-tailwindcss with npm install --save-dev eslint-plugin-tailwindcss
- Add "plugin:tailwindcss/recommended" to the end of the extends list in your ESLint config
- Add "tailwindcss" to the plugins object and a rules object to disable the tailwindcss/classnames-order rule
- If your app uses TypeScript, duplicate the rules object inside overrides and add "plugin:tailwindcss/recommended" to the inner extends list
The eslint-plugin-tailwindcss plugin provides useful linting rules for Tailwind CSS classes. It checks if a class used in code is a Tailwind class, which is a good practice when using Tailwind.
A fresh viewpoint: Nextjs App Router Tailwind Loading Page
Set Up
You'll also need to set up ESLint and Prettier in your Next.js project. Run the command npm install --save-dev eslint-config-prettier to install the necessary plugin. In your ESLint config, add "prettier" to the END of extends: { "extends": ["next/core-web-vitals", ..., "prettier"] }.
Create a .eslintignore file in the project root to ignore certain files or folders that should not be linted by ESLint. This file doesn't need to have any content for now, but it will come in handy in the future if you need to add folders or files to ignore.
Set up your package.json scripts to run ESLint and Prettier correctly. The most important script is "build", which runs ESLint but not Prettier or Stylelint by default. Modify the "build" script in your package.json file to run Prettier in check mode: "build": "next build && prettier --check .".
A fresh viewpoint: Nextjs Script
Prettier in VS Code
To get Prettier working in VS Code, you'll need to install the ESLint and Prettier VS Code extensions. This will enable automatic linting and formatting on save.
You can install these extensions by searching for them in the Extensions panel in VS Code. Once installed, you'll need to modify your workspace settings by adding the following configuration to your .vscode/settings.json file:
- This configuration will run ESLint and Prettier every time you save a file.
To set up Prettier in VS Code, you'll also need to install the eslint-config-prettier package by running the following command in your terminal: npm install --save-dev eslint-config-prettier.
You'll also need to add "prettier" to the end of the extends array in your ESLint config, like so: "extends": ["next/core-web-vitals", ..., "prettier"].
Here's a quick rundown of the necessary VS Code extensions:
- ESLint extension
- Prettier extension
- Stylelint extension (if you're using SCSS and not Tailwind)
- TailwindCSS extension (if you are using Tailwind and not SCSS)
These extensions will provide linting and formatting on file save and syntax highlight on linting errors.
Support for TypeScript
To support TypeScript in Next.js, you can use the `--typescript` flag when creating your app. This will enable Next.js ESLint rules along with TypeScript-specific lint rules.
You can also add the necessary configurations manually. To do this, you'll need to add the following code to your `.eslintrc.json` file: "next/typescript". This will enable TypeScript support for ESLint.
Here's a summary of the key points to keep in mind when setting up ESLint with TypeScript:
With these configurations in place, you'll be able to take advantage of the benefits of using TypeScript with Next.js and ESLint.
Lint Staged: Check Code Only When Necessary
Lint staged is a tool that allows you to run your lint scripts only when necessary, and only on the necessary files. This is a game-changer for large projects where checking code takes time.
You can use lint staged to check your code when you make changes, but only on the files that have actually changed. This saves you time and makes your workflow more efficient.
To configure lint staged, you need to create a configuration file called lint-staged.config.js. You can configure it directly in package.json, but a dedicated file gives you more options.
Suggestion: Nextjs Code Block
In this file, you specify the file matchers and the commands that will be run against the changed files. For example, you can separate TypeScript checks and ESLint checks into two matchers, so they can be run in parallel.
Here's an example of how you can configure lint staged:
```html
// lint-staged.config.js
module.exports = {
'**/*.ts': ['tsc', 'eslint'],
'**/*.{js,jsx,ts,tsx}': ['prettier --write'],
};
```
In this example, we're running TypeScript checks and ESLint checks on all TypeScript files, and running Prettier format on all JavaScript and TypeScript files.
By configuring lint staged, you can make your code checking process more efficient and save yourself time in the long run.
A different take: Running Json Nextjs
Troubleshooting and Checks
Building and committing is a good sanity check for your setup, and you might catch errors during this process.
If you already have code in your project, you might get errors when committing. This can be resolved by adding folders or files to one of the ignore files, such as .stylelintignore, .eslintignore, and .prettierignore.
Typically, you'll need to add specific folders like .storybook and storybook-static to these files. For example, if you have Storybook installed, you'll need to add these folders to each of the ignore files.
You might also need to install plugins for specific file types, like Prettier-plugin-gherkin for Gherkin .feature files. Running npm install prettier-plugin-gherkin --save-dev will resolve this issue.
ESLint might also complain about specific file types, like .cy.ts files containing Cypress interaction tests. To resolve this, you'll need to install the NPM package for Cypress ESLint plugin and configure it as described.
The typescript config might be too strict, causing a lot of errors when building. If you don't want to fix individual errors, you can disable the @typescript-eslint/recommended-type-checked config by commenting it out in .eslintrc.js and uncommenting @typescript-eslint/recommended.
Sometimes, it's safe to turn off a linting rule at a specific line or for a whole file. You can do this by pressing Ctrl + . to Show Code Actions and selecting "Disable @typescript/no-non-null-assertion for the entire file".
See what others are reading: Storybook Nextjs
Frequently Asked Questions
Does NextJS use ESLint?
Yes, NextJS uses ESLint through its bundled eslint-plugin-next, which helps catch common issues in NextJS applications. This integration enables developers to catch errors and improve code quality.
What is ESLint used for?
ESLint helps maintain consistent code formatting and structure across projects, making code easier to share and reuse. It also prevents coding conflicts and issues that can arise from different styles and approaches.
Sources
- https://staticmania.com/blog/how-to-integrate-eslint-and-prettier-with-next-js-for-code-quality
- https://www.freecodecamp.org/news/how-to-set-up-eslint-prettier-stylelint-and-lint-staged-in-nextjs/
- https://medium.com/@kimperria/so-linters-what-are-they-why-and-how-to-set-up-in-nextjs-698ef58480d4
- https://blog.linotte.dev/eslint-9-next-js-935c2b6d0371
- https://paulintrognon.fr/blog/typescript-prettier-eslint-next-js
Featured Images: pexels.com