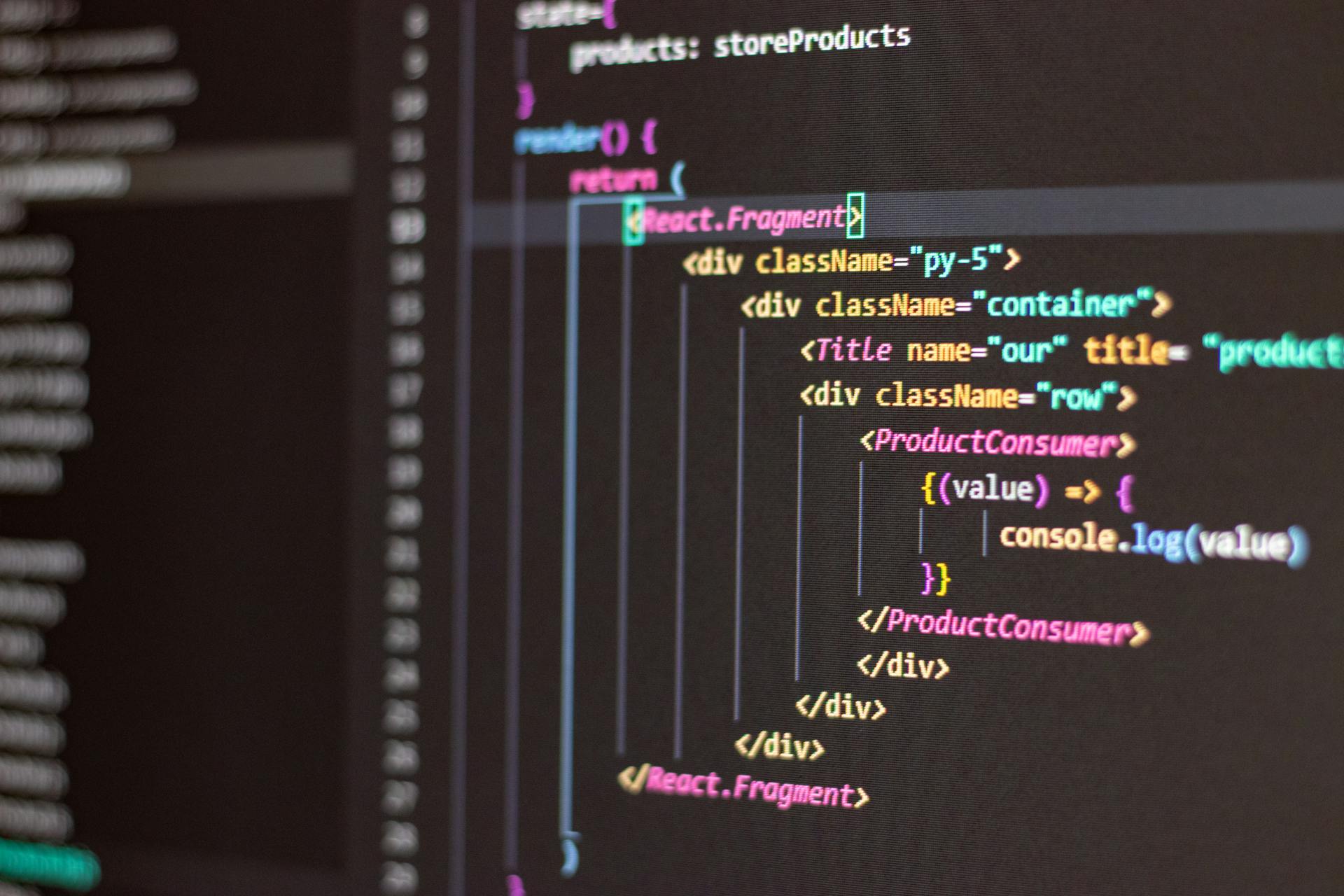
Next Js and Apollo are a powerful combination for building full-stack applications using npm. Next Js is a popular React-based framework for building server-side rendered (SSR) and statically generated websites and applications.
With Next Js, you can create a robust and scalable full-stack application that integrates with Apollo, a graph-based data query language. This integration enables you to manage complex data relationships and fetch data from multiple sources efficiently.
By leveraging the strengths of both Next Js and Apollo, you can build a fast, secure, and maintainable application that meets the needs of your users. This approach also enables you to take advantage of the latest web development trends and best practices.
A unique perspective: Mern Stack Interview Questions
Project Setup
To set up your Next.js project, you'll need to ensure Node.js is installed on your computer. This will enable you to install the necessary dependencies using npx and npm commands.
First, install Node.js on your computer. Once you have Node.js installed, you can proceed with the setup.
For more insights, see: Install Next Js
Requirements
To set up your project, you'll need to have a few things in place first. Make sure you have Node.js installed on your computer.
Having Node.js installed will allow you to install other necessary tools like Next.js, Tailwind CSS, and Flowbite React using npx and npm commands.
A unique perspective: Bootstrap and Node Js
Install
To install the necessary tools for your project, start by ensuring you have Node.js installed on your computer, as this is a requirement for installing Next.js, Tailwind CSS, and Flowbite React.
You can check if Node.js is installed by running a command in your terminal, but for the sake of this tutorial, we'll assume it's already installed.
To install the authentication library NextAuth, see the options documentation for more details on how to configure providers, databases, and other options.
Here are some key things to note when installing NextAuth:
- Read the documentation for more details on configuration.
- Learn how to add authentication providers.
To create a new Next.js app, you'll use the `create-next-app` command, which will scaffold your app and install dependencies.
Upgrade Status
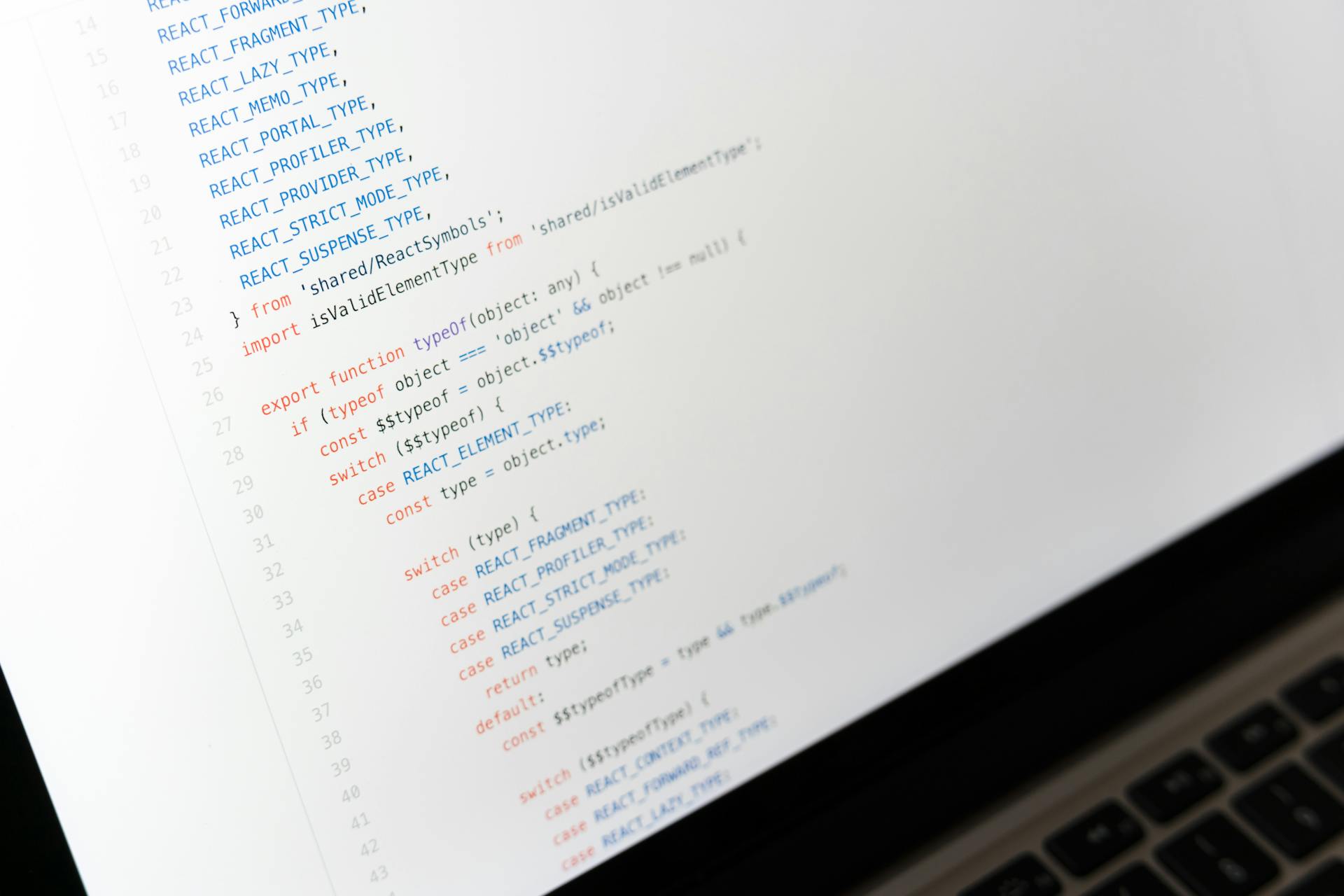
To track the progress of your project's upgrade, it's essential to know which dependencies support React 19. I've created a table to help you out.
The package recharts is supported but has a note that the react-is version needs to match the version of React 19 you're using.
Intriguing read: Tailwindcss React Native
Components and Routing
Components in Next.js are essentially reusable pieces of code that can be used to build pages and applications. They can contain React components, HTML, and even server-side rendered (SSR) code.
Routing in Next.js is managed using the `pages` directory, where each file in the directory corresponds to a route in the application. For example, a file named `[id].js` in the `pages` directory would match any URL that has an `id` parameter.
The `pages` directory is the central hub for routing in Next.js, making it easy to manage and organize routes in a large application.
Recommended read: Next Js Pages
Using Shadcn/UI
You'll need to run npx shadcn@latest init -d to resolve peer dependency issues.
This command will prompt you to select an option to fix the issues.
By running this command, you'll be able to resolve the peer dependency issues that can arise when using Shadcn/UI.
The process is straightforward and will guide you through the necessary steps to get your project up and running smoothly.
Adding Components
Adding new components to your app can be a great way to enhance its functionality. Remember to always test your app after installing new dependencies.
To avoid any potential issues, it's essential to follow a structured approach when adding components. This will help you ensure that everything works as expected.
When you add a new component, take the time to review its documentation and understand how it fits into your app's architecture.
For more insights, see: Next Js Component
Add API Route
To add an API route for authentication, create a file called [...nextauth].js in pages/api/auth. This file will contain the dynamic route handler for NextAuth.js.
All requests to /api/auth/* will automatically be handled by NextAuth.js, including signIn, callback, signOut, and more.
If you're using Next.js 13.2 or above with the new App Router, you can initialize the configuration using the new Route Handlers, but this is not required.
Recommended read: Next Js Fetch Data save in Context and Next Route
Add React Hook
Adding a React Hook to your application is a straightforward process. The useSession() React Hook in the NextAuth.js client is the easiest way to check if someone is signed in.
You can use the useSession hook from anywhere in your application, such as in a header component.
To leverage this hook, you don't need to write any complex code or worry about authentication logic. The useSession hook takes care of it for you.
The useSession hook is a powerful tool that simplifies authentication and authorization in your application.
Suggestion: React + Next Js
Data Fetching and Rendering
You can fetch data for server-side rendered pages using the getServerSideProps method in Next.js. This method is used during each page request to get any data passed into the page component as props.
Next.js will fetch the country codes for each page request instead of only at build time. This is the major difference between fetching data for server-side rendered pages and statically generated pages.
To set up Apollo Client for client-side data, you need to use ApolloProvider in your _app.js file. This is necessary because you'll be in React and want to use hooks.
ApolloProvider wraps your root component, allowing you to use hooks anywhere within your app. However, you still need to ensure that components using hooks are only rendered on the client, not on the server.
To accomplish this, you create a component that only renders its children in the browser and not on the server. This component is called ClientOnly and is responsible for fetching the countries.
You can use the ClientOnly component to wrap your page components and ensure that data is fetched only when the page is rendered in the browser. This is particularly useful for pages that don't need data at build time, like the client-side.js page in the example.
You might enjoy: Next Js Hook
Featured Images: pexels.com