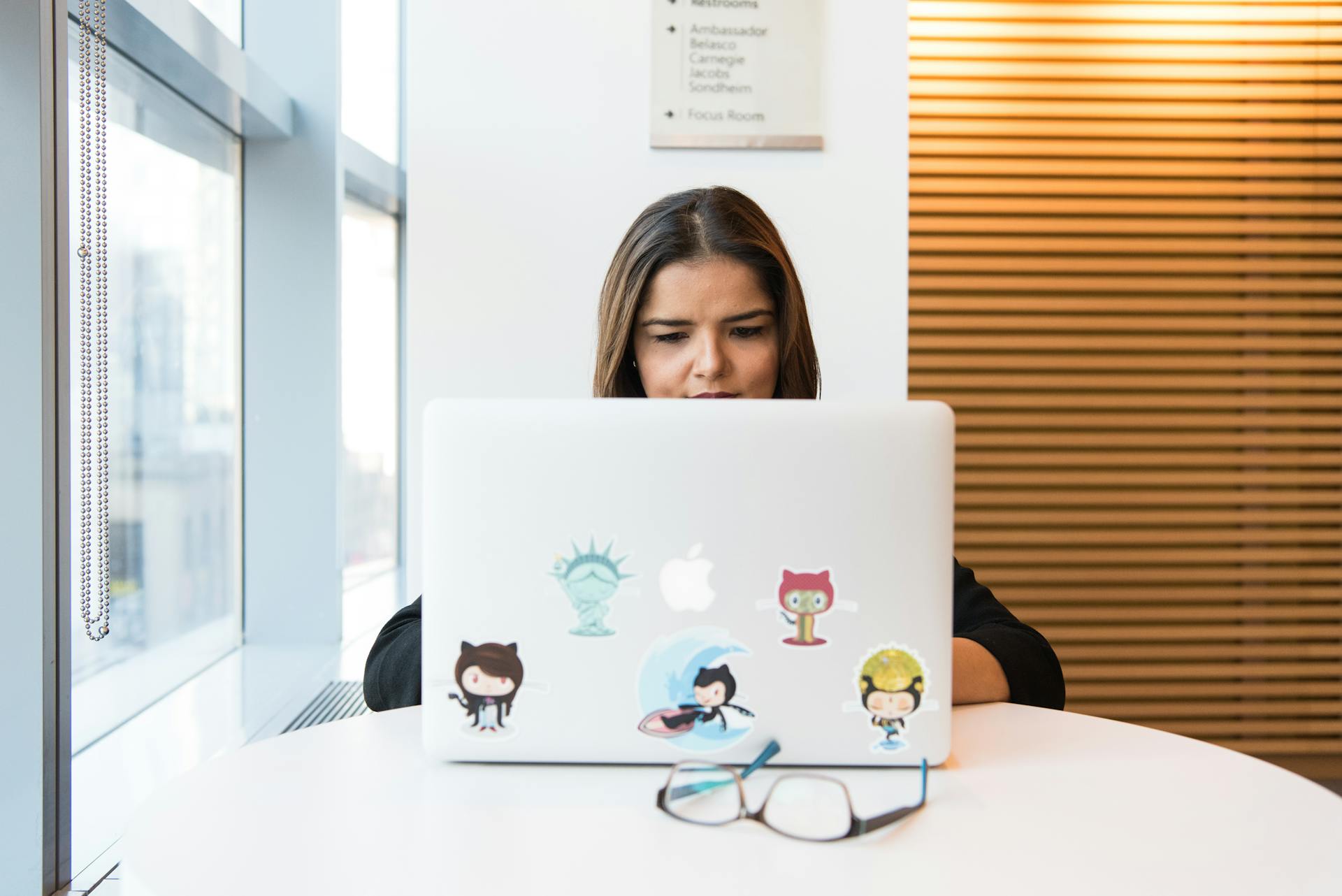
As a MERN stack developer, you're likely no stranger to the importance of being prepared for an interview. In fact, according to the MERN stack interview questions, having a solid understanding of the technology stack is a must-have.
To ace your interview, you'll want to be familiar with the key components of the MERN stack, including MongoDB, Express.js, React.js, and Node.js. These are the building blocks of your application, and being able to speak to their strengths and weaknesses will make you a more attractive candidate.
In terms of specific questions, be prepared to discuss the differences between MongoDB and other databases, as well as the role of Express.js in handling server-side logic. You should also be able to explain how React.js is used to create reusable UI components and how Node.js handles server-side rendering.
Here's an interesting read: Npm Next Js
JavaScript Foundations
JavaScript Foundations are a crucial part of the MERN stack, and understanding them is essential for success in the field.
JavaScript ES6 introduces several features that improve development, including improved syntax and new methods like map, filter, and reduce, which make coding more efficient and enjoyable.
Here are some key features of JavaScript ES6:
Node.js is a JavaScript runtime environment that allows developers to run JavaScript on the server-side, making it a key component of the MERN stack.
What Are Pure Components?
Pure components in JavaScript are primarily tasked with conducting a comparison of props and state whenever there is a change in either props or state.
In the MERN stack, pure components can be viewed as standard components, differing primarily in their engagement with the shouldComponentUpdate method.
Pure components are standard components that are designed to optimize performance by minimizing unnecessary re-renders.
JavaScript Foundations
JavaScript is a versatile programming language that's widely used for web development. It's the backbone of many modern websites and applications.
JavaScript ES6 has introduced several features that improve development, such as let and const variables, which replace the need for var. This makes it easier to write more secure and efficient code.
Consider reading: Mean Stack Development Company
The MERN stack is a popular framework that combines JavaScript with other technologies like MongoDB and Express.js. It's a great choice for building scalable and robust web applications.
Node.js is a JavaScript runtime environment that allows developers to run JavaScript on the server-side. It's used in the MERN stack because it provides a fast and efficient way to handle requests and responses.
Event-driven programming in Node.js is a key concept that allows developers to write asynchronous code. This means that instead of waiting for a task to complete, the code can move on to the next task, making it more efficient and scalable.
Here are some key features of Node.js:
Express.js (Backend Framework)
Express.js is a popular backend framework used in MERN stack development, providing a lightweight and powerful solution for building APIs and web applications. It's an essential tool in the backend of MERN applications.
Express.js supports middleware, allowing for seamless integration of different functionalities like authentication and error handling. Middleware in Express.js refers to functions that are executed during the request-response cycle, modifying requests, handling errors, or terminating the request-response cycle.
Here are some key aspects of Express.js:
- What is Express.js?
- How do you handle errors in Express.js?
- What are routes in Express.js?
- How do you manage authentication in Express.js?
- How do you structure large-scale Express.js applications?
Express.js
Express.js is a popular backend framework that simplifies web development by providing a lightweight and powerful solution for building APIs and web applications. It's an essential tool in the backend of MERN applications.
Express.js can be used in conjunction with Node.js to create scalable web APIs, making it an ideal choice for larger web apps. This synergy is seen in popular stacks like MEAN and MERN.
Express.js provides a range of features, including middleware support, which allows for seamless integration of different functionalities like authentication and error handling. Middleware can modify requests, handle errors, or terminate the request-response cycle.
Here are some key aspects of Express.js:
- Handling errors in Express.js involves using try-catch blocks and error-handling middleware.
- Routes in Express.js are used to handle different HTTP requests and responses.
- Authentication in Express.js can be managed using middleware like Passport.js.
- Structuring large-scale Express.js applications involves using modular design and separating concerns.
Express.js can be used to create RESTful APIs, which follow the principles of resource-based architecture and use standard HTTP methods like GET, POST, PUT, and DELETE. CORS (Cross-Origin Resource Sharing) is also an important aspect of Express.js development, as it allows for secure cross-origin requests.
In a MERN Stack application, Express.js can be used to replace REST APIs with GraphQL, which allows for more efficient data fetching and reduces over-fetching.
Readers also liked: Next Js Stack
What Are WebSockets and How to Implement Real-Time Communication?
WebSockets allow real-time, bi-directional communication between a client and server. This means you can send and receive data simultaneously, making it perfect for applications that require real-time updates.
You can use libraries like Socket.io in your Node.js server to implement WebSockets. This will enable bi-directional communication with your clients.
Real-time communication is especially useful in applications that require instant feedback or updates. With WebSockets, you can create a seamless experience for your users.
To implement real-time communication, you'll need to use a library like Socket.io in your Node.js server and React client. This will allow you to establish a WebSocket connection and start sending and receiving data in real-time.
WebSockets are a game-changer for applications that require real-time updates. By using WebSockets, you can create a more engaging and interactive experience for your users.
A fresh viewpoint: Bootstrap and Node Js
Connecting to Databases
Connecting to databases is a crucial aspect of building a robust MERN stack application. You can connect Node.js to a MongoDB database by following a few simple steps.
Connecting to MongoDB involves using a driver that allows Node.js to interact with the database. This can be done with the help of the 'mongodb' package.
The 'mongodb' package is a popular choice for connecting Node.js to MongoDB, but other options like 'mongoose' can also be used. Mongoose is an Object Data Modeling (ODM) library that provides a more intuitive way of interacting with MongoDB.
To connect to a MongoDB database using the 'mongodb' package, you need to import the package and create a new instance of the MongoDB client. This involves passing the connection string to the client.
Connecting to a database is an essential step in building a MERN stack application. In a real-world scenario, you would replace the connection string with your actual MongoDB connection details.
The connection string is used to establish a connection to the MongoDB database. You can obtain the connection string from your MongoDB Atlas cluster or from your local MongoDB instance.
In the case of MongoDB Atlas, you can find the connection string in the "Connect" tab of your cluster's settings. This connection string includes the username, password, and other details required to connect to the database.
Authentication and Security
Authentication and Security is a crucial aspect of building a robust MERN Stack application. You may need to implement user authentication using JWT (JSON Web Token) or OAuth.
To protect certain routes or data, you can use authorization. This involves creating secure login routes in Express.js and storing tokens in the client for subsequent requests.
Input validation is a common security practice in MERN Stack applications. This helps prevent injection attacks by ensuring that user input is sanitized and validated.
HTTPS is another essential security practice. It enables secure communication between the client and server, protecting sensitive data from interception.
Rate limiting is also a vital security measure. By limiting the number of requests from a single IP address, you can prevent brute-force attacks on your application.
Here are some common security practices in MERN Stack applications:
- Input validation: To prevent injection attacks.
- HTTPS: For secure communication.
- Rate limiting: To prevent brute-force attacks.
- Environment variables: To secure API keys and sensitive information.
How to Optimize Application Performance
Optimizing the performance of a MERN stack application is crucial for a smooth user experience. To achieve this, consider implementing lazy loading in React, a technique that delays loading of components until they are actually needed.
Lazy loading can significantly reduce the initial load time of your application. By loading components on demand, you can minimize the amount of code that needs to be executed, resulting in faster page loads.
Another technique to optimize performance is to use caching mechanisms. This can be achieved through various means, including React's built-in caching features or third-party libraries like Redis.
In addition to these techniques, optimizing database queries is also essential. This can be done by minimizing the number of database calls, using efficient query techniques, and optimizing the database schema.
Here are some key takeaways to keep in mind when optimizing application performance:
- Lazy loading in React can significantly reduce initial load times.
- Caching mechanisms can help minimize the number of database calls.
- Optimizing database queries is essential for efficient performance.
Advanced Topics
In a MERN app, WebSockets can be used to enable real-time communication between the client and server.
WebSockets allow for bi-directional communication, enabling the server to push updates to the client without the client needing to make a request.
To handle asynchronous operations in React, you can use higher-order components (HOCs) to abstract away the complexity of managing state changes.
Higher-order components can be used to handle side effects, such as API calls or timer management, in a more manageable way.
Server-side rendering (SSR) in React can improve page load times by pre-rendering the initial HTML on the server.
SSR can also improve SEO by providing a static HTML snapshot of the page to search engines.
To handle asynchronous operations in React, you can use the following techniques:
- Use async/await with promises
- Use callbacks or event handlers
- Use libraries like Redux or MobX to manage state changes
Environment variables in Node.js can be used to store sensitive information, such as API keys or database credentials.
Using environment variables can help keep your code secure by not hardcoding sensitive information.
Development and Best Practices
As you dive into the world of MERN stack development, it's essential to understand the best practices and development techniques that will make your projects shine.
To interact with the backend in a MERN application, you need to understand how the frontend and backend communicate with each other. This is typically done through RESTful APIs.
You might like: Front-end Web Development
RESTful APIs are a crucial part of the MERN stack, and they help to separate the frontend and backend logic. In a MERN application, RESTful APIs are used to handle requests and responses between the frontend and backend.
One of the key challenges in MERN development is securing the application. To secure a MERN stack application, you need to implement authentication and authorization mechanisms.
CORS (Cross-Origin Resource Sharing) is a crucial aspect of MERN development, and it helps to handle requests between different domains. In a MERN stack application, CORS is handled through the use of headers and middleware.
To deploy a MERN stack application, you need to use a combination of tools such as Node.js, Express.js, and MongoDB. The deployment process typically involves setting up a production environment, configuring the application to use the correct ports, and ensuring that the application is scalable and secure.
Here are some key development best practices to keep in mind:
- Use a modular approach to development, breaking down the application into smaller modules and components.
- Implement a robust testing framework to ensure that the application is thoroughly tested.
- Use a consistent coding style and follow established coding standards.
- Keep the application organized and maintainable by using a clear and logical directory structure.
- Use a version control system to track changes and collaborate with team members.
By following these best practices and development techniques, you'll be well on your way to creating robust and scalable MERN stack applications.
Sources
- https://www.geeksforgeeks.org/top-mern-stack-interview-questions/
- https://softspacesolutions.com/blog/mern-stack-developer-interview-questions/
- https://www.linkedin.com/pulse/top-questions-from-my-first-mern-stack-interview-mahesh-pawar-lpelf
- https://blog.imocha.io/mern-stack-developer-interview-questions
- https://futuristiccodingacademy.com/mern-stack-interview-questions/
Featured Images: pexels.com