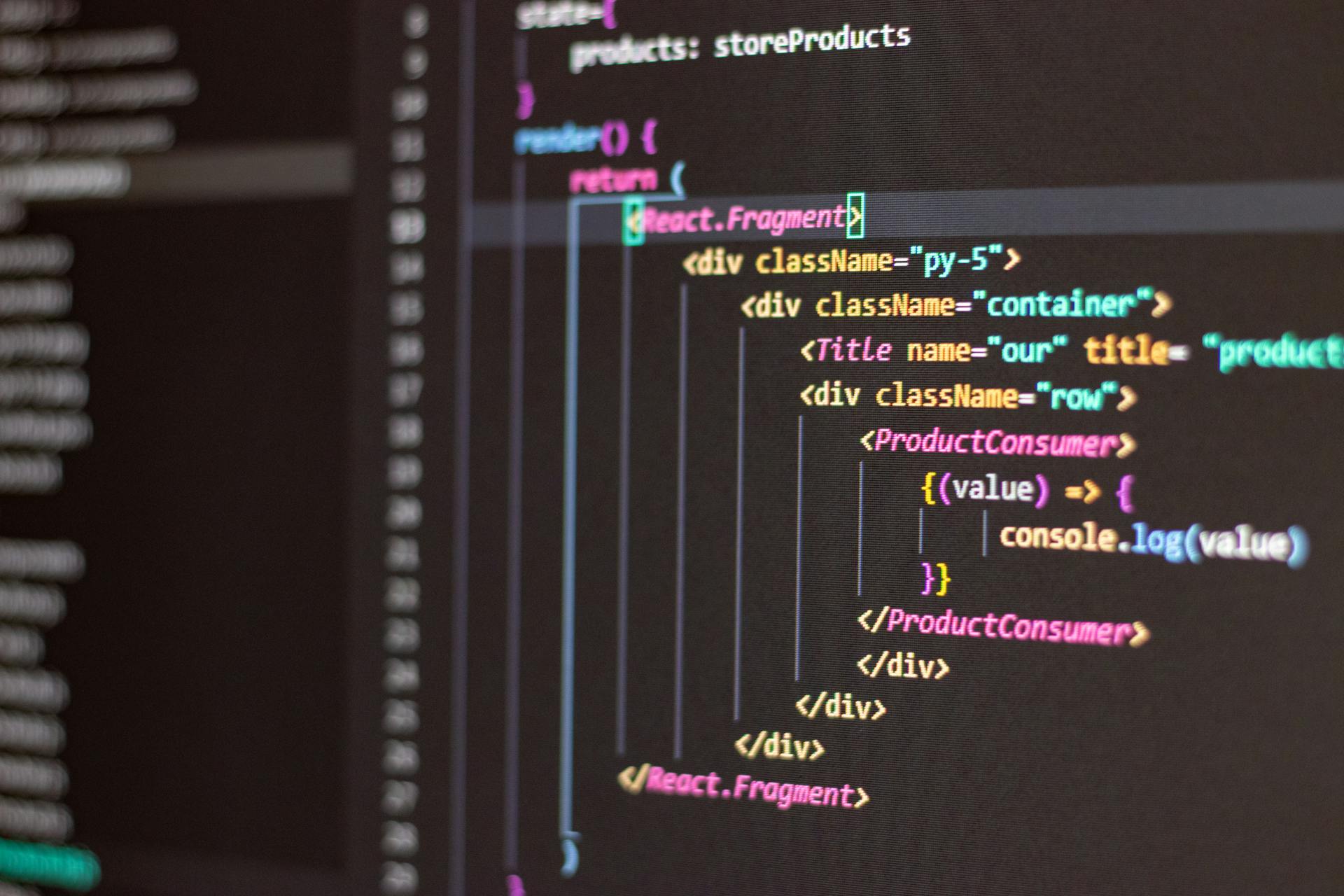
Next Js components are the building blocks of your application, and optimizing them is crucial for a seamless user experience. By utilizing the built-in features of Next Js, such as server-side rendering and static site generation, you can significantly improve the performance of your application.
In Next Js, components are rendered on the server by default, which means that the initial HTML is generated on the server and sent to the client. This approach is known as server-side rendering, or SSR. SSR can improve the performance of your application by reducing the amount of data that needs to be transferred between the client and server.
By using static site generation, Next Js can pre-render pages at build time, which can improve the performance of your application by reducing the amount of data that needs to be transferred between the client and server. Static site generation can also improve SEO by providing search engines with a static version of your site.
Using Next Js components with dynamic imports can also improve the performance of your application. By importing components on demand, you can reduce the amount of code that needs to be loaded upfront, which can improve the initial load time of your application.
On a similar theme: Next Js Debugging
Next.js Version 14
Next.js Version 14 introduces Server Components, which run exclusively on the server, fetching data directly within the component without sending code to the client. This reduces the JavaScript bundle size that needs to be downloaded and parsed by the browser.
Server Components use a .server.js extension, while Client Components, designed for handling client-side interactivity, run in the browser and use a .client.js extension. They are crucial for dynamic functionalities like handling user inputs, clicks, and other interactions.
Streaming SSR is enhanced in Next.js 14, allowing parts of a page to be sent to the client as soon as they’re ready. This approach improves the time-to-first-byte (TTFB) and the overall user experience by progressively rendering the page.
Migrating from the traditional getServerSideProps approach to using Server Components and Client Components offers several advantages, including reduced JavaScript bundle size and improved flexibility. Developers gain more control and flexibility by mixing server and client components, enabling optimized data fetching strategies and rendering paths.
Check this out: Nextjs App Route Get Ssr Data
Here are some key benefits of adopting Next.js 14’s Server Components and Client Components:
- Reduced JavaScript Bundle Size
- Improved Flexibility
- Enhanced User Experience
- Simplified Development Process
The transition to Server Components and Client Components involves rethinking how components are structured and data is fetched, but the benefits make it a compelling upgrade for Next.js applications.
Rendering and Performance
Server-side rendering (SSR) pre-renders each page on the server at request time, sending a fully rendered page to the client.
This approach minimizes the need for client-side state management to handle data fetching and rendering.
Server Components are rendered through a server-client coordination process, which involves segmenting rendering work into smaller units, or chunks, based on route segments.
The server sends the HTML and React Server Component Payload (RSC Payload) to the client, which React uses to update the browser's DOM and synchronize server-rendered components with their client-side counterparts.
Client Components are loaded and made interactive through JavaScript hydration.
Next.js offers three rendering strategies: static, dynamic, and streaming, which enable efficient content distribution, real-time content generation, and improved load times, respectively.
Developers can adjust settings to balance between serving static and dynamic responses.
For your interest: Next Js React Fundamentals
State Management and Updates
State management is a crucial part of building interactive web applications, and it's especially important when using Next.js components. With Server-Side Rendering (SSR), the initial page load can display data without the need to fetch it client-side, reducing the need for initial state setup and client-side data fetching logic.
To make the page interactive or to update the content based on user actions, you still need to manage state on the client side. This is because the initial state is more complete from the server, but you still need to handle user interactions.
The good news is that SSR can simplify your client-side logic by handling data fetching and rendering on the server. This means you might rely less on complex state management solutions or use simpler state management patterns since the bulk of data handling is performed server-side.
However, state is still crucial for managing interactivity and updates based on user actions after the initial page load. This is where things get a bit more complicated.
Discover more: Nextjs Usecontext
Here's a quick rundown of what you need to consider:
- Interactivity: You'll still need to manage state on the client side for user interactions.
- Reduced Client-Side Complexity: SSR can simplify your client-side logic by handling data fetching and rendering on the server.
In a Next.js application using Server Components along with Client Components, you'll need to employ a strategy to refresh the data shown to the user after an action like deletion. This might involve using client-side state management, possibly with React hooks like useState and useEffect, in a Client Component.
Intriguing read: Using State in Next Js
Migration and Upgrades
Migration to Server Components can simplify the data fetching process, making it more intuitive to integrate with the component lifecycle. This approach streamlines your application's architecture.
You can now directly incorporate data fetching logic within Server Components, eliminating the need for getStaticProps and getServerSideProps. This change makes it easier to build Next.js applications.
Separating concerns is key when migrating to Server Components with Interactions. Use a Server Component for fetching and displaying data, and Client Components for interactive elements.
Migration from GetStaticProps and GetServerSideProps
Migration from GetStaticProps and GetServerSideProps can be a game-changer for Next.js applications. This approach simplifies the data fetching process, making it more intuitive to integrate with the component lifecycle.
You can now directly incorporate data fetching logic within Server Components, which streamlines your application's architecture. This means you can ditch getStaticProps for static generation and getServerSideProps for server-side rendering.
Server Components offer a powerful new model for building Next.js applications, but understanding when and how to use them effectively is key to leveraging their benefits without sacrificing the user experience.
Migrating
Migration can be a complex process, but with the right approach, it can also be a great opportunity to simplify and streamline your application's architecture.
You can now directly incorporate data fetching logic within Server Components, making it more intuitive to integrate with the component lifecycle.
Server Components offer a powerful new model for building Next.js applications, but understanding when and how to use them effectively is key to leveraging their benefits without sacrificing the user experience.
To migrate code to Server Components, you can directly fetch data within the component, and files that contain Server Components should have a .server.js extension.
Worth a look: Nextjs Ui Library
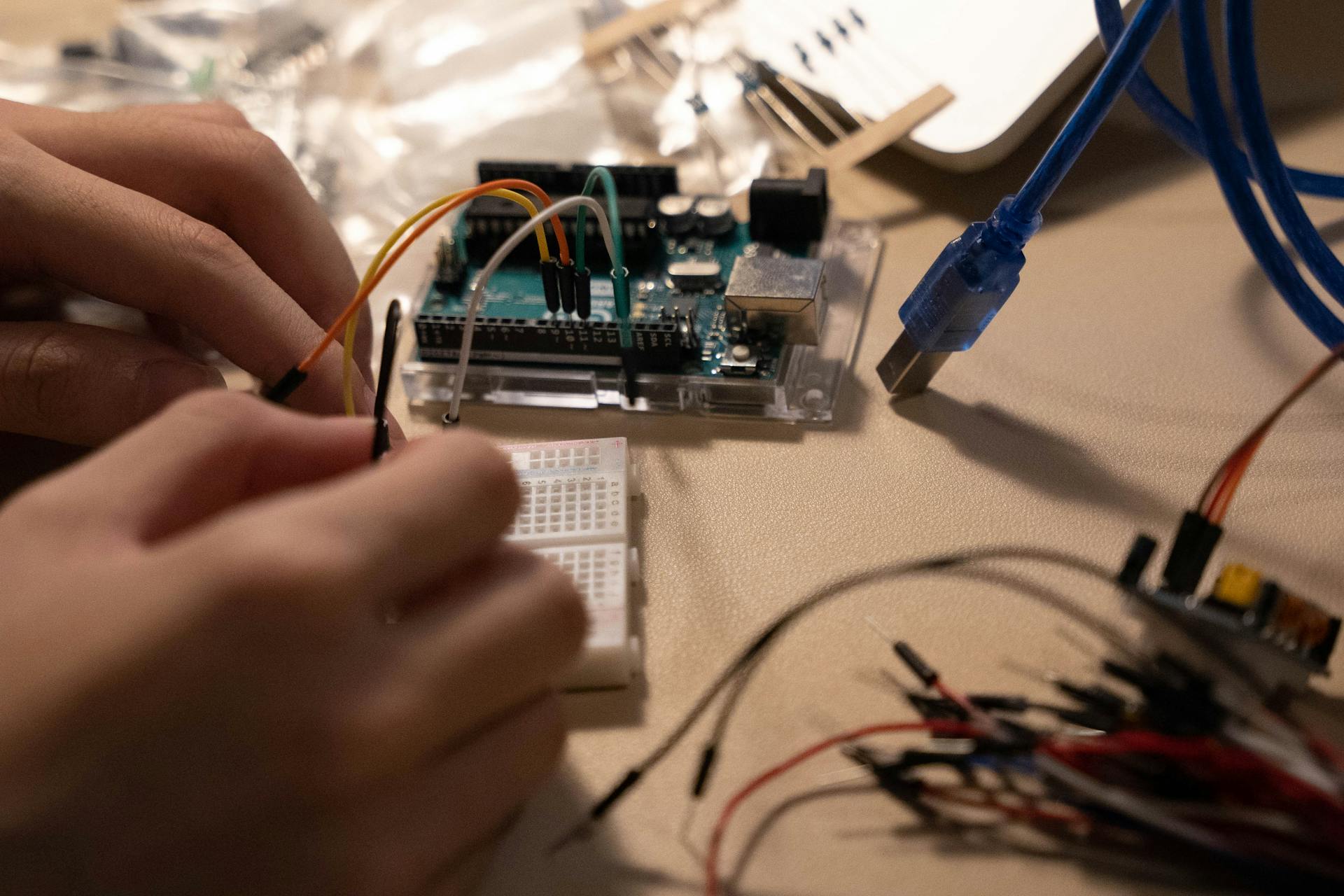
In version 14 with Server Components, you'll separate the concerns by using a Server Component for fetching and displaying data, and Client Components for interactive elements.
Server Components can be used for fetching and displaying data, such as a list of posts, and then use Client Components for interactive elements like update and delete buttons.
Curious to learn more? Check out: Next Js Client Side Rendering
Components and Structure
Components in Next.js are self-contained modules that encapsulate all the code necessary to render a part of the user interface. They manage their own state and lifecycle, and can be composed together to create rich page layouts.
A well-organized components folder in a Next.js project might look something like this: Layout components arrange other components on the page.UI components are responsible for general user interface elements.Form components are specific to form functionality. These components help maintain a consistent layout throughout the application.
Components can be reused across multiple pages, promoting reusability and modularity. A consistent naming and organization scheme across the entire project makes it easier to find and import components. This can be achieved by keeping the components folder flat, using index files to re-export components, and documenting components to explain their purpose and usage.
Take a look at this: Next Js Form
Understanding V14
Understanding V14 is a significant upgrade in Next.js, introducing Server Components as a unified and flexible approach to building web applications.
This change replaces traditional data fetching methods like getStaticProps and getServerSideProps with a more streamlined process.
Server Components run on the server, allowing for direct data fetching using await, eliminating the need for getServerSideProps.
The file extension for Server Components is now .server.js, indicating it's a Server Component, and data fetching is done directly inside the component.
The use of getServerSideProps is eliminated, and the component itself handles the responsibility of fetching data and rendering based on that data.
Server Components provide a more flexible architecture by allowing you to mix server-side and client-side components seamlessly.
Here are some key benefits of using Server Components:
- Flexibility: You can optimize your application by deciding which components need to be server-side based on data requirements and which can be client-side.
- Performance: Server Components can significantly reduce the amount of code the client needs to download, parse, and execute, leading to faster load times and improved performance.
- Incremental Adoption: Server Components can be adopted incrementally, meaning you can start using them in parts of your application without needing to refactor everything at once.
This flexibility and performance boost make Server Components a powerful tool in your Next.js toolkit.
Rendering Strategies
Next.js offers three rendering strategies that help developers balance between serving static and dynamic responses. These strategies are designed to optimize performance and improve load times.
Static rendering is ideal for static content that doesn't change often, and it can be used with getStaticProps to pre-generate pages at build time. This approach ensures fast load times and can be served from a CDN.
Dynamic rendering, on the other hand, is better suited for dynamic content that changes regularly or depends on the user session. getServerSideProps can be used to generate pages on-the-fly, ensuring up-to-date content.
Next.js also supports streaming rendering, which enables real-time content generation. This strategy is particularly useful for applications that require continuous updates or live data.
Here's a brief summary of the three rendering strategies:
Key Considerations
When you're structuring your application with components, there are some key considerations to keep in mind.
Server Components are not interactive, so they're perfect for handling pre-rendered parts of your application, like fetching and displaying data.
Direct data fetching should be done within Server Components, as we saw in the PostsList.server.js example.
For more insights, see: Next Js Spa
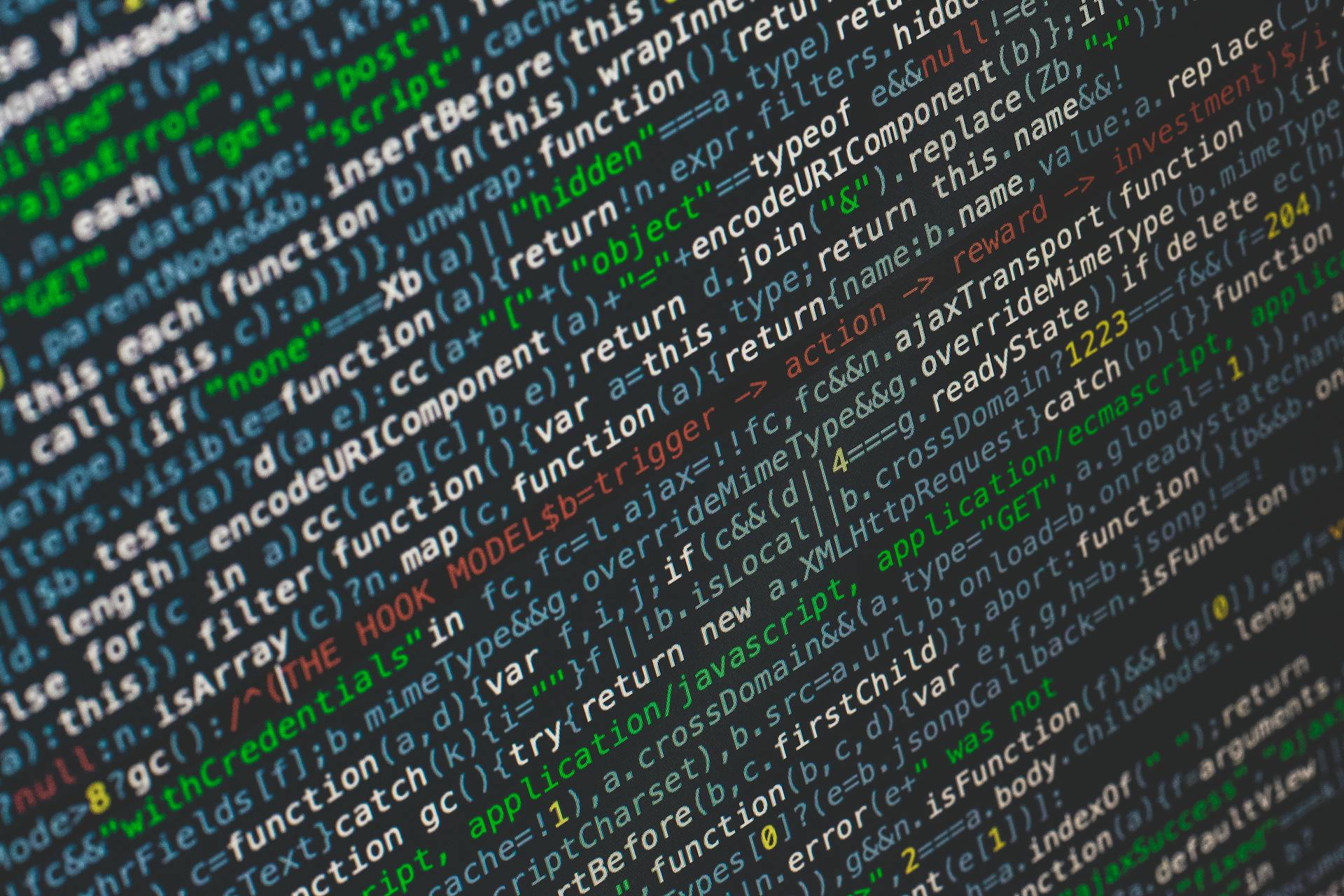
Interactivity, on the other hand, is best managed by Client Components, like PostActions.client.js, which handles update and delete buttons.
Here are some key differences between Server and Client Components to keep in mind:
- Server Components handle data fetching and pre-rendering.
- Client Components handle interactivity, like update and delete functionality.
- Client Components must be regular .client.js files to run on the client side.
This setup allows you to leverage the efficiency and performance benefits of Server Components while still providing a dynamic user experience where necessary.
What is a React Component?
A React component is a self-contained module that encapsulates all the code necessary to render a part of the user interface.
It's like a Lego block in your application code, which you can use and reuse to build complex UIs. Each component manages its own state and lifecycle.
Components in React are typically written in JSX, which allows you to write HTML-like syntax directly within your JavaScript code.
This makes it easier to visualize the UI structure within the component's code.
Take a look at this: Next Js Component Rendering Analyzer
Reusability and Composition
Reusability and composition are two fundamental principles of React and Next.js components. A component is a self-contained module that encapsulates all the code necessary to render a part of the user interface. Components in Next.js are typically written in JSX, which allows you to write HTML-like syntax directly within your JavaScript code.
Readers also liked: Nextjs Code Block
Components in Next.js are designed to be reusable, which means you can significantly reduce the amount of code you need to write and maintain. This leads to a more consistent user experience and speeds up the development process. By designing components to be reusable, you can create a more modular codebase that's easier to manage.
Components can be composed together to create rich page layouts. For example, you might have a Button component that you can use on its own, but you can also incorporate it into a Form component to create a complete interactive form. This is made possible by the compositional nature of React and Next.js components.
Here are some key benefits of reusability and composition:
- Flexibility in designing and structuring your application
- Reduced code duplication and maintenance
- Easier collaboration and knowledge sharing among team members
By applying these principles, you can create a more efficient, scalable, and maintainable codebase that's easier to work with.
Functional vs Class
Functional components are simpler and are just JavaScript functions that return JSX, making them perfect for components that don't require state management or lifecycle methods.
In fact, with the introduction of hooks in React, functional components have become even more powerful, allowing you to use state and other React features without writing a class.
Functional components are typically used for components that don't require state management or lifecycle methods, which makes them a great choice for simple UI elements or presentational components.
Class components, on the other hand, are more complex and are defined by extending React.Component, providing more features like lifecycle methods that allow you to run code at specific points in the component's lifecycle.
However, with the growing popularity of hooks, class components are becoming less common, making functional components a more widely used choice.
Readers also liked: React and Next Js
Headings
Server Components in Next.js allow you to write components that render exclusively on the server, leading to smaller bundle sizes and potentially faster load times.
Server Components can fetch data directly within the component itself, removing the strict separation between data fetching and UI rendering.
In Next.js, implementing Server Components involves creating a React component, with Next.js automatically handling the server-side rendering process.
Worth a look: Nextjs Server Rendering Tailwind
Roles
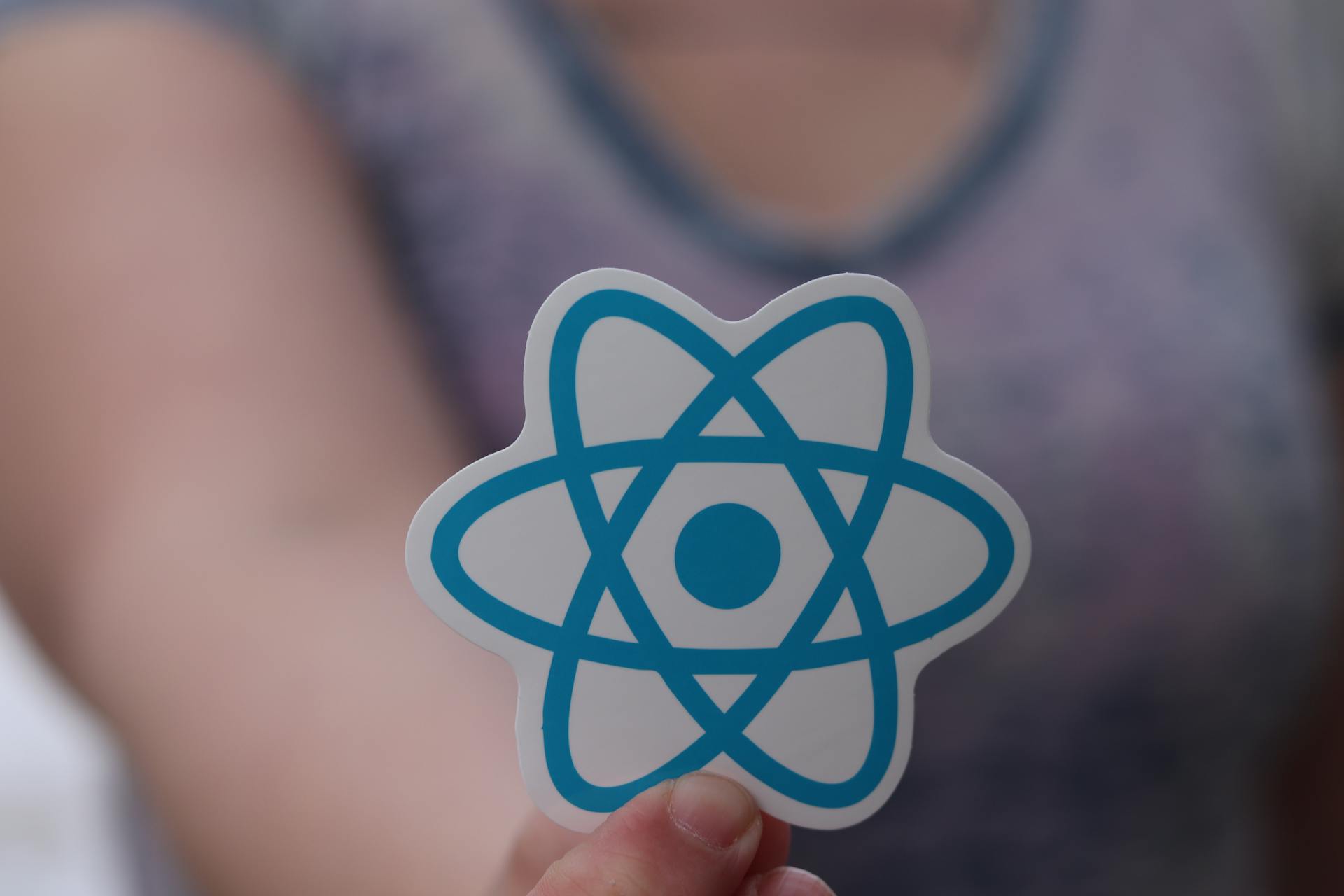
In Next.js applications, understanding the roles of different components is crucial for optimizing server-side efficiency and user experience.
Server Components efficiently handle data and backend interactions, while Client Components focus on interactivity and state management.
The distinct roles of these components allow for a seamless user experience, where the server handles the heavy lifting and the client provides a responsive interface.
On a similar theme: Next Js Use Client
Sources
- https://medium.com/@sassenthusiast/my-epiphany-with-next-js-14s-server-components-08f69a2c1414
- https://nextjs.org/learn/react-foundations/server-and-client-components
- https://scastiel.dev/server-components-actions-react-nextjs
- https://www.codecademy.com/learn/learn-next-js/modules/next-js-server-components/cheatsheet
- https://www.dhiwise.com/post/ultimate-guide-to-organizing-your-nextjs-components-folder
Featured Images: pexels.com