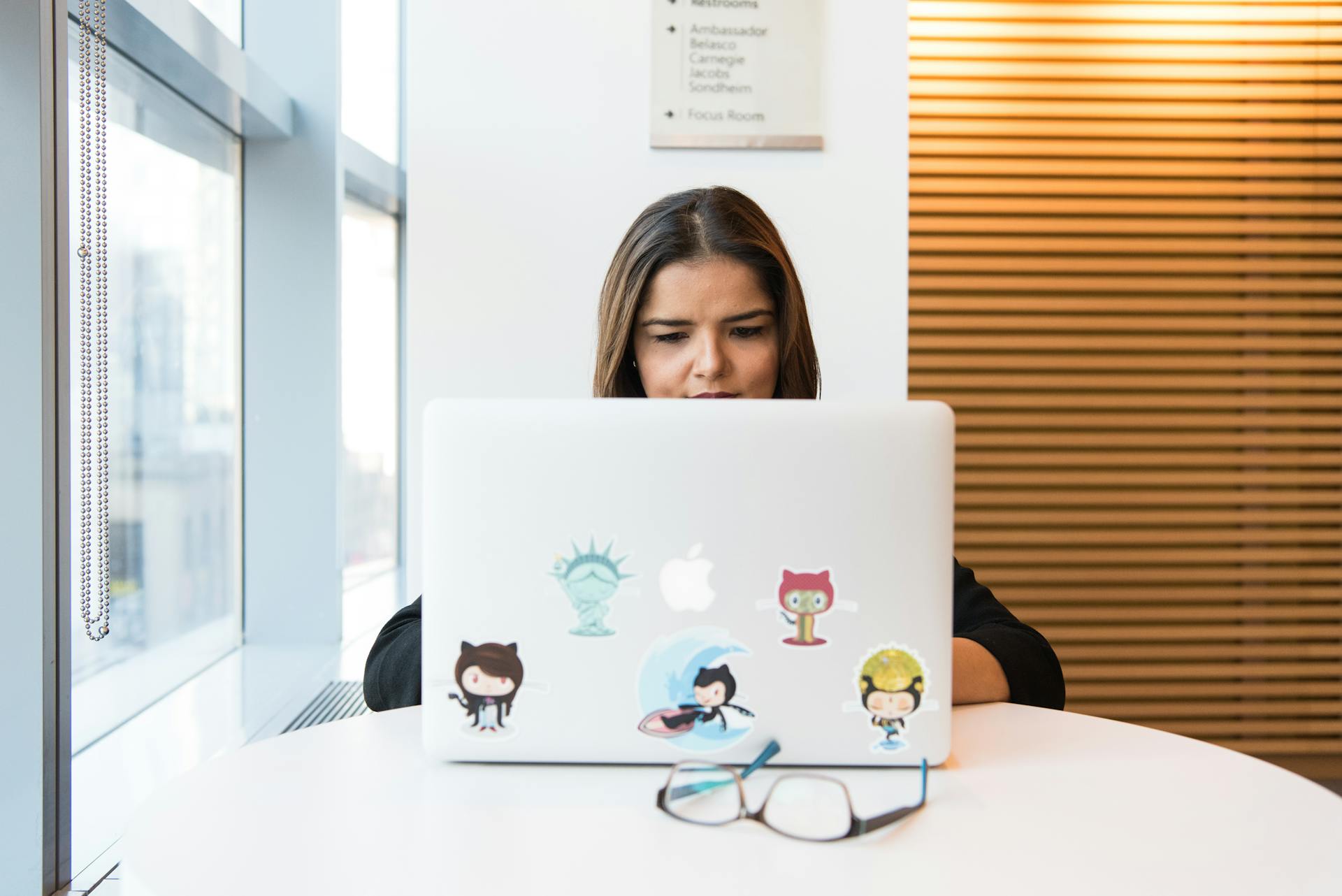
Next.js Server Components are revolutionizing the way we build frontend applications. They provide a new way to render components on the server, which can lead to significant performance improvements and better SEO.
Server Components are built on top of React, which means you can use all your existing React knowledge and skills. This makes it easy to integrate Server Components into your existing projects.
One of the key benefits of Server Components is that they can render on the server, which means that the initial HTML can be sent to the client without any JavaScript code. This can lead to faster page loads and a better user experience.
Server Components also provide a way to reuse code and share data between the server and the client. This can make your code more maintainable and easier to manage.
Discover more: Next Js Debug
Rendering and State
With Next.js Server Components, you can simplify your client-side logic by handling data fetching and rendering on the server. This is known as Server-Side Rendering (SSR).
Related reading: Next Js Client Side Rendering
SSR pre-renders each page on the server at request time, sending the fully rendered page to the client. This reduces the need for client-side state management to handle data fetching and rendering.
The initial page load can display data without the need to fetch it client-side, reducing the need for initial state setup and client-side data fetching logic. Here are some key benefits of using SSR:
- Initial Load: Display data without client-side fetching
- Interactivity: Still need to manage state on the client side for updates
- Reduced Client-Side Complexity: Simplify client-side logic by handling data on the server
By handling data fetching and rendering on the server, you can rely less on complex state management solutions or use simpler state management patterns. This can make your code easier to maintain and update.
You might like: Next Js State Management
Rendering
Rendering is a crucial aspect of web development that determines how a page is loaded and displayed to the user.
Server-Side Rendering (SSR) pre-renders each page on the server at request time, making it a more efficient approach.
Since the data fetching and initial rendering are done on the server, there is less immediate need for client-side state management to handle data fetching and rendering.
The page arrives at the client ready to be displayed, eliminating the need for additional rendering on the client-side.
This approach is particularly useful for applications that require a seamless user experience, as the page is already fully rendered and ready to be displayed.
You might like: Nextjs Server Rendering Tailwind
Impact on State
Server-side rendering (SSR) has a significant impact on state management. It can reduce the need for initial state setup and client-side data fetching logic.
The initial page load with SSR can display data without the need to fetch it client-side. This reduces the complexity of client-side state management for initial data fetching and rendering.
With SSR, you can simplify your client-side logic by handling data fetching and rendering on the server. This might reduce the reliance on complex state management solutions.
State is still crucial for managing interactivity and updates based on user actions after the initial page load. You'll still need to manage state on the client side for interactive elements.
Here are some key differences in state management with SSR:
- Initial Load: Reduced need for initial state setup and client-side data fetching logic.
- Interactivity: State is still crucial for managing updates based on user actions.
- Reduced Client-Side Complexity: Simplified client-side logic due to server-side data handling.
Fetching Data
Fetching data is a crucial aspect of building dynamic web applications with Next.js Server Components. You can directly use data fetching libraries or native fetch within your Server Components.
Direct fetching allows you to fetch data during render time on the server and pass it down to client components as props. This approach is particularly useful when you need to fetch data that's required for a specific page or component.
Readers also liked: Nextjs Fetch
Server Components also enable streaming capabilities, which can improve perceived performance for end-users. This means content can be streamed to the client as it's being generated, reducing load times and improving user experience.
To fetch data in Layout Components, you can use the new use hook, an experimental hook in React that uses Suspense to fetch data on the server. This hook allows you to fetch data in a seemingly synchronous way, making it easier to write asynchronous code.
Here are the key benefits of using the use hook for data fetching in Layout Components:
- Fetch data on the server using the use hook
- Conditionally render components based on the fetched data
The new data fetching system in Next.js also adds automatic request deduping, caching, and cache re-validation to the JavaScript fetch API. This means you can make the same fetch request in multiple React components, and only one request will be made.
You can use async/await in Server Components to simplify data fetching. This makes it easier to write asynchronous code and handle errors. For example, you can use async/await to fetch data from an API or database and render it on the page.
Broaden your view: Nextjs Script Async
API and Webhooks
API and Webhooks are two powerful features in Next.js Server Components. API routes are now supported in the new app directory, and the convention to define them is to create a file named route.tsx in the app directory.
API routes use the standard Request object, rather than the express-like req and res objects. This makes it easier to work with API routes.
You can export the handler for the methods you want to support, such as GET and POST methods. For example, you can export the GET and POST functions.
To set cookies in API routes and Server Actions, you can use the function cookies().set. However, you cannot set a cookie from a Server Component.
Handling webhooks is also a breeze in Next.js App Router. You can get the raw body request by using the request.text() method.
Here are the ways to call a server action from a client component:
- Defining the action as the action property of a form component
- Calling the action from a button component using the formAction property
- Calling the action using the useTransition hook (if it mutates data)
- Simply calling the action like a normal function (if it does not mutate data)
API Routes
API Routes are now supported in the new app directory, and they use the standard Request object, not the express-like req and res objects.
To define an API route, create a file named route.tsx in the app directory and export the handler for the methods you want to support, such as GET and POST functions.
You can also use the function cookies().set to set cookies in API routes.
API routes are different from Server Components, which cannot set cookies and will throw an error if you try.
Recommended read: Nextjs Client Side Cookies
Handling Webhooks
Handling webhooks is a common use case for API routes. You can get the raw body request by using the request.text() method, which is much simpler than in the Pages routing system.
In Next.js App Router, this method makes it easier to retrieve the raw body from the request. This is a significant improvement over the previous system.
To call a server action from a client component, you have multiple options. Here are a few ways to do it:
- Defining the action as the action property of a form component
- Calling the action from a button component using the formAction property
- Calling the action using the useTransition hook (if it mutates data)
- Simply calling the action like a normal function (if it does not mutate data)
If you're defining a server action from a client component, it needs to be exported from a separate file and imported in the client component. This file should have the keyword use server at the top.
Intriguing read: Nextjs Server Actions File Upload
Reading HTTP Cookies and Headers
Reading HTTP cookies and headers is a crucial part of building robust APIs and webhooks. You can use the next/headers package to read cookies and headers if you're working with a Server Component.
The next/headers package allows you to read the values of cookies and headers, but it's currently limited to reading only, and you can't set or delete them. This is a known limitation, and it's not just you who's feeling it.
To access cookies and headers, you'll need to use the functions provided by the next/headers package. This is because, unlike getServerSideProps, you don't have direct access to the request object.
One thing to keep in mind is that Next.js is exposing these utilities to help you read data from the request. This is a deliberate design choice, and it's not something that will be changing anytime soon.
Related reading: Nextjs Headers
Migration and Best Practices
Migration to Server Components can simplify your data fetching process, making it more intuitive to integrate with the component lifecycle.
By incorporating data fetching logic within Server Components, you can streamline your application's architecture and potentially reduce complexity.
Server Components can directly replace getStaticProps and getServerSideProps for static generation and server-side rendering, respectively.
This approach can make your application's architecture more intuitive and easier to maintain.
It's essential to understand when and how to use Server Components effectively to avoid sacrificing the user experience.
Incorporating Server Components with interactive elements requires separating concerns between Server Components for fetching and displaying data, and Client Components for interactive elements like buttons.
Server Components can handle fetching and displaying data, while Client Components can handle interactive elements like update and delete buttons.
See what others are reading: Nextjs App Route Get Ssr Data
Advanced Topics
Server components in Next.js allow for true separation of concerns between client and server-side rendering. This means you can write components that only render on the server, reducing the amount of data sent to the client.
With server components, you can write code that only runs on the server, without having to worry about it being executed on the client-side. For example, you can use the `useEffect` hook to fetch data on the server, without it being re-run on each client-side render.
If this caught your attention, see: Use Client Nextjs
Server components can also be reused across multiple pages, making it easier to manage shared functionality. For instance, a component that fetches data from an API can be reused on multiple pages, reducing code duplication.
Server components are also more secure, as they don't expose sensitive data to the client. This is especially important when dealing with sensitive data, like user authentication or payment information.
Server components can be used to implement API routes, which can be used to handle requests and return data to the client. This allows for a more robust and scalable API implementation.
By using server components, you can take advantage of the benefits of server-side rendering, while still being able to write client-side code that takes advantage of modern web APIs. This allows for a more flexible and efficient development workflow.
Expand your knowledge: Next Js Pages
Get and Client-Side
Server components in Next.js allow for rendering on the server and client-side. This is made possible by the use of the `useEffect` hook.
You can use the `useEffect` hook to fetch data on the server, making it available to the client-side render. This can be useful for reducing the amount of data transferred over the network.
By leveraging the server-side rendering capabilities of Next.js, you can improve the performance and user experience of your application.
Get
Get is a crucial concept in the context of Client-Side, as it refers to the process of retrieving data or resources from a server or another source.
The GET method is a fundamental HTTP request method that allows clients to request data from a server without modifying it.
In the context of Client-Side, GET is often used to fetch data from a server, such as retrieving user information or loading content.
A GET request typically includes query parameters, which are used to pass data to the server.
For instance, a GET request to retrieve a user's profile might include parameters such as the user's ID or username.
The GET method is also idempotent, meaning that making the same request multiple times will have the same effect as making it once.
This property makes GET requests safe for use in situations where the same request is made repeatedly, such as when a user refreshes a page.
You might enjoy: Next Js Get Url Params
Client
Client components are the components as we know them today, but they have some restrictions when used with server components.
You can use a client component inside a server component, but it's best to keep your client component at the leaves of your component tree.
For example, if you have a webpage with a logo, sidebar, blog posts, and dynamic navigation and search, you should make the whole page a server component and make just the navbar and search as client components.
Server components can import client components, and you should pass the server component as a child prop to the child component, and wrap both components in another server component.
This pattern allows React to render the server component on the backend and then send the generated HTML to the client component.
Worth a look: Nextjs Redirect from Server Component
Sources
- https://medium.com/@sassenthusiast/my-epiphany-with-next-js-14s-server-components-08f69a2c1414
- https://nextjs.org/docs/app/building-your-application/rendering/server-components
- https://makerkit.dev/blog/tutorials/nextjs13
- https://deadsimplechat.com/blog/react-server-components-with-next-js/
- https://nextjs.org/docs/app/building-your-application/rendering
Featured Images: pexels.com