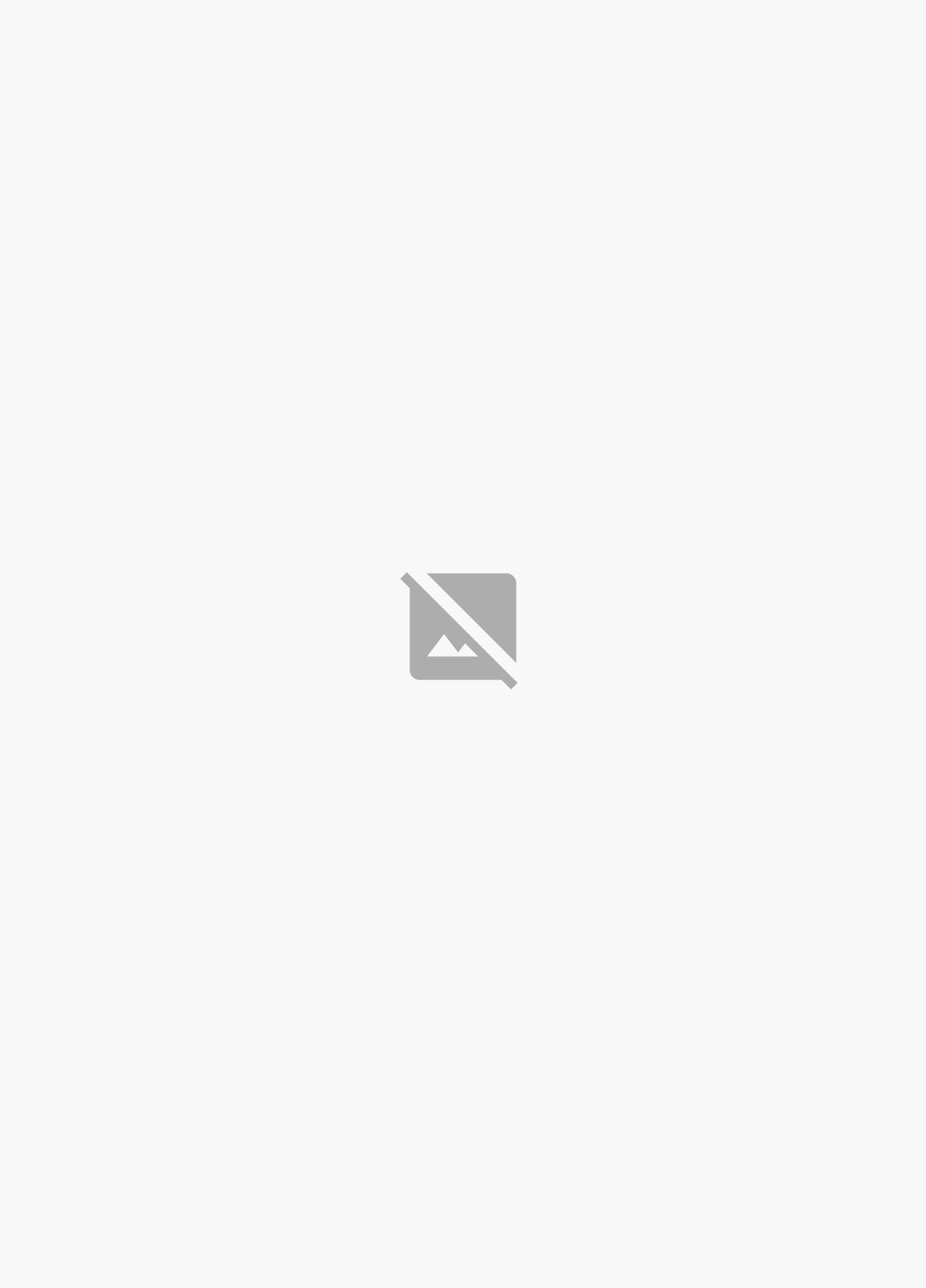
Next.js headers are a crucial aspect of securing your application. They help protect your site from common web vulnerabilities like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF).
To set up Next.js headers, you can use the `next.config.js` file, which is where you'll configure most of your site's settings. This file is where you'll find the `headers` property, which is used to set custom HTTP headers.
Custom headers can be used to add security features like Content Security Policy (CSP) and Cross-Origin Resource Sharing (CORS). These features help protect your site from malicious requests and unauthorized access.
By configuring your Next.js headers correctly, you can significantly improve the security of your application and protect your users' data.
Expand your knowledge: Nextjs Set Hostname
Setting Up Secure Headers
To set up secure headers in Next.js, you'll want to use the `createSecureHeaders` function in your `next.config.js` file. This is recommended for projects that don't use servers or have just been created.
A different take: Why Use Nextjs
You can import `createSecureHeaders` from `next-secure-headers` and use it in the `headers` async function in `next.config.js`. By default, `next-secure-headers` applies some rules, but you can give options to the first argument of the function to enable or disable rules.
Here are some common security headers you can set up using `createSecureHeaders`:
Configure Secure Settings in Next.js
To configure secure settings in Next.js, you'll want to use the `createSecureHeaders` function in your `next.config.js` file. This function is available in Next.js 9.5 or higher.
You can import `createSecureHeaders` from `next-secure-headers` and use it in the `headers` function in `next.config.js`. By default, `next-secure-headers` applies some rules, but you can give options to the first argument of the function to enable or disable rules.
You can also configure different headers by URLs following the official documents. To do this, you'll need to pass options for rules as the first argument to `createSecureHeaders`.
To harden security for your application, you can use the `X-Frame-Options` header to prevent click-jacking. This tells browsers not to embed your application inside another frame. You can also use the `X-Content-Type-Options` header to prevent browsers from interpreting a response as any other content-type than what is defined with the `Content-Type` header.
The `Referrer-Policy` header allows you to customize how much information visitors give about where they are coming from when they navigate away from your page. You can also use the `Permissions-Policy` header to disable browser features to varying degrees.
For another approach, see: Next Js Disable Ssr
Setup
You have two main options for setting up secure headers in your Next.js project: using createSecureHeaders in next.config.js or using withSecureHeaders in page components. You can't go wrong with the recommended approach of using createSecureHeaders in next.config.js, which is supported in Next.js 9.5 or higher.
This method is serverless, works well with static pages, and retains Automatic Static Optimization. If you're just starting out with Next.js, I recommend sticking with this approach.
You can import createSecureHeaders from next-secure-headers and use it in the headers async function in next.config.js. By default, next-secure-headers applies some rules, but you can customize these rules by giving options to the first argument of the function.
If you're using Cloudflare Pages, you can also attach headers to responses by creating a _headers plain text file in the output folder of your project. This file is usually where you'll find the deploy-ready HTML files and assets generated by the build.
See what others are reading: Next.js
Here are some key things to keep in mind when using the _headers file:
Managing Headers
To attach headers to Cloudflare Pages responses, create a _headers plain text file in the output folder of your project. It's usually the folder that contains the deploy-ready HTML files and assets generated by the build.
The _headers file is where you define header rules in multi-line blocks. The first line of a block is the URL or URL pattern where the rule's headers should be applied. You can use absolute URLs, but be aware that they must begin with https and specifying a port is not supported.
A rule like https://example.com/path would match against requests to other://example.com:1234/path. This means that using absolute URLs is flexible, but it's essential to follow the rules.
You can define as many [name]: [value] pairs as you require on subsequent lines. For example:
An incoming request which matches multiple rules' URL patterns will inherit all rules' headers. This is a powerful feature, as it allows you to apply different headers to different parts of your website.
Check this out: Nextjs Public Folder
Here's an example of what the _headers file might look like:
A project is limited to 100 header rules. Each line in the _headers file has a 2,000 character limit. The entire line, including spacing, header name, and value, counts towards this limit.
If a header is applied twice in the _headers file, the values are joined with a comma separator. This means you can stack multiple headers for a single URL pattern.
Worth a look: Next Js Header
Security Features
To harden security for your application, you can use the next-secure-headers package, which is recommended for Next.js 9.5 or higher. This approach is serverless, making it perfect for static pages and Static Site Generation (SSG).
The package provides a function called createSecureHeaders that returns headers as an object in a specific format. You can customize the rules applied by giving options to the first argument of the function.
One of the security features you can enable using next-secure-headers is the X-Frame-Options header, which prevents browsers from embedding your application inside another frame. This is particularly useful for preventing click-jacking attacks.
The Permissions-Policy header allows you to customize the browser features that can be disabled, giving you fine-grained control over your application's content. This is especially useful for developers who need to configure security settings for their application.
You can also use the Content-Security-Policy header to configure a range of security settings, including controls similar to the X-Frame-Options header. This header is particularly useful for developers who need to configure security settings for their application.
To implement these security features, you can use the createSecureHeaders function and pass in your desired options. This function is available in the next-secure-headers package and can be used in conjunction with the headers function in next.config.js.
CORS and Cross-Site Security
Cross-Origin Resource Sharing (CORS) is a security feature that allows other domains to fetch assets from your Next.js project.
To enable CORS, you can add the following to the _headers file: This applies the Access-Control-Allow-Origin header to any incoming URL.
You can be more restrictive by defining a URL pattern that applies to a *.pages.dev subdomain, which then only allows access from its staging branch's subdomain.
Next.js also provides a way to harden security for your application, which includes preventing click-jacking with a X-Frame-Options header.
Expand your knowledge: Nextjs Url Parameter Is Not Allowed
Frame Guard
Frame Guard is a crucial aspect of cross-site security. It's a feature that prevents clickjacking attacks by informing browsers not to embed your application inside another.
To set the "X-Frame-Options" header, you can use the "frameGuard" directive. The default value is "deny", which is highly recommended if you don't use frame elements such as iframe.
This setting is documented on the MDN website, which provides more information on the X-Frame-Options header.
The "deny" value is particularly effective in preventing clickjacking attacks, as it blocks any attempts to embed your application within another frame.
Referrer Policy
The Referrer Policy is a crucial aspect of maintaining cross-site security. It's used to control how much information about the current page is sent to other servers.
The default value for the Referrer Policy is false. This can be found in the MDN documentation on the Referrer-Policy header.
You can specify one or more values for legacy browsers that don't support a specific value. This helps ensure that older browsers don't break when encountering a new policy.
The Referrer Policy is set using the Referrer-Policy header, which prevents other servers from getting the referrer information.
Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing (CORS) is a technique that allows other domains to fetch assets from your Pages project. To enable this, you can add a specific configuration to the _headers file.
To apply the Access-Control-Allow-Origin header to any incoming URL, you can simply add this to the _headers file. This will allow any domain to fetch assets from your Pages project.
If you want to be more restrictive, you can define a URL pattern that applies to a *.pages.dev subdomain, which then only allows access from its staging branch's subdomain. This is a great way to control who can access your assets.
Take a look at this: Next Js Fetch Data
Search Engine Optimization (SEO)
SEO is a crucial aspect of Next.js development, and it's essential to understand how it works.
Next.js provides built-in support for SEO through its use of HTML head tags, which can be customized using the `next/head` component.
Custom HTML head tags are used to specify metadata about a page, such as its title, description, and keywords.
A different take: Next Js Head
The `next/head` component allows developers to add custom HTML head tags to their pages, making it easier to manage SEO metadata.
By using the `next/head` component, developers can ensure that their pages are properly optimized for search engines.
Custom HTML head tags can also be used to specify the language of a page, which is important for search engines that cater to users who speak different languages.
Specifying the language of a page can also improve the user experience for non-English speakers.
SEO metadata can also be generated automatically using Next.js's built-in support for internationalization (i18n) and localization (L10n).
Automatic SEO metadata generation can save developers a significant amount of time and effort.
Next.js's built-in support for i18n and L10n allows developers to easily create multilingual pages that are optimized for search engines.
By using Next.js's built-in support for SEO, developers can focus on building a great user experience without worrying about the technical details of SEO.
Take a look at this: Nextjs Redirect from Server Component
Application Security
Application security is crucial for any Next.js application. You can prevent click-jacking by informing browsers not to embed your application inside another with a X-Frame-Options header.
To harden security for your application, you can use the X-Content-Type-Options: nosniff header to prevent browsers from interpreting a response as any other content-type than what is defined with the Content-Type header. This is especially useful when serving static files.
The Referrer-Policy header allows you to customize how much information visitors give about where they are coming from when they navigate away from your page. You can also use the Permissions-Policy header to disable browser features to varying degrees.
Here are some security settings you can configure with the Content-Security-Policy header:
- X-Frame-Options takes priority over frameAncestors in some browsers.
- frameAncestors should be set to false when setting frameGuard to false.
By implementing these security settings, you can significantly improve the security of your Next.js application.
Content Security Policy
Content Security Policy is a crucial aspect of application security that helps prevent loading and executing non-allowed resources. It's a header that can be set to prevent click-jacking by informing browsers not to embed your application inside another.
To set Content Security Policy, you can use the `contentSecurityPolicy` option in your `next.config.js` file. By default, it's set to `false`, but you can change it to `true` to enable the policy. You can also specify directives using chain-case names, such as `child-src` instead of `childSrc`.
Here's a breakdown of the default values for Content Security Policy:
Keep in mind that when setting `frameAncestors`, you should also set `frameGuard` to `false`, as Chrome 40 and Firefox 35 will ignore the `frame-ancestors` directive and follow the `X-Frame-Options` header instead.
By implementing Content Security Policy, you can have fine-grained control over your application's content and prevent various types of security threats.
Secure Application
To secure your application, you can start by using the createSecureHeaders function in next.config.js. This is recommended for Next.js 9.5 or higher, as it provides a built-in way to configure headers without requiring any servers.
This approach is ideal for static pages or Server-Side Generation (SSG) projects, and it can retain Automatic Static Optimization. You can import createSecureHeaders from next-secure-headers and use it in the headers async function in next.config.js.
You can also use the withSecureHeaders HOC to specify headers using getServerSideProps. This is available for application and page components, but not in next.config.js.
To harden security for your application, you can use headers like X-Frame-Options, X-Content-Type-Options, Referrer-Policy, Permissions-Policy, and Content-Security-Policy. These headers can prevent click-jacking, browser features from being disabled, and fine-grained control over your application's content.
To prevent man-in-the-middle attacks during redirects from HTTP to HTTPS, you can use the Strict-Transport-Security (HSTS) header. This is highly recommended if you use HTTPS (SSL) on your servers.
Here's a summary of some of the key headers you can use to secure your application:
Featured Images: pexels.com