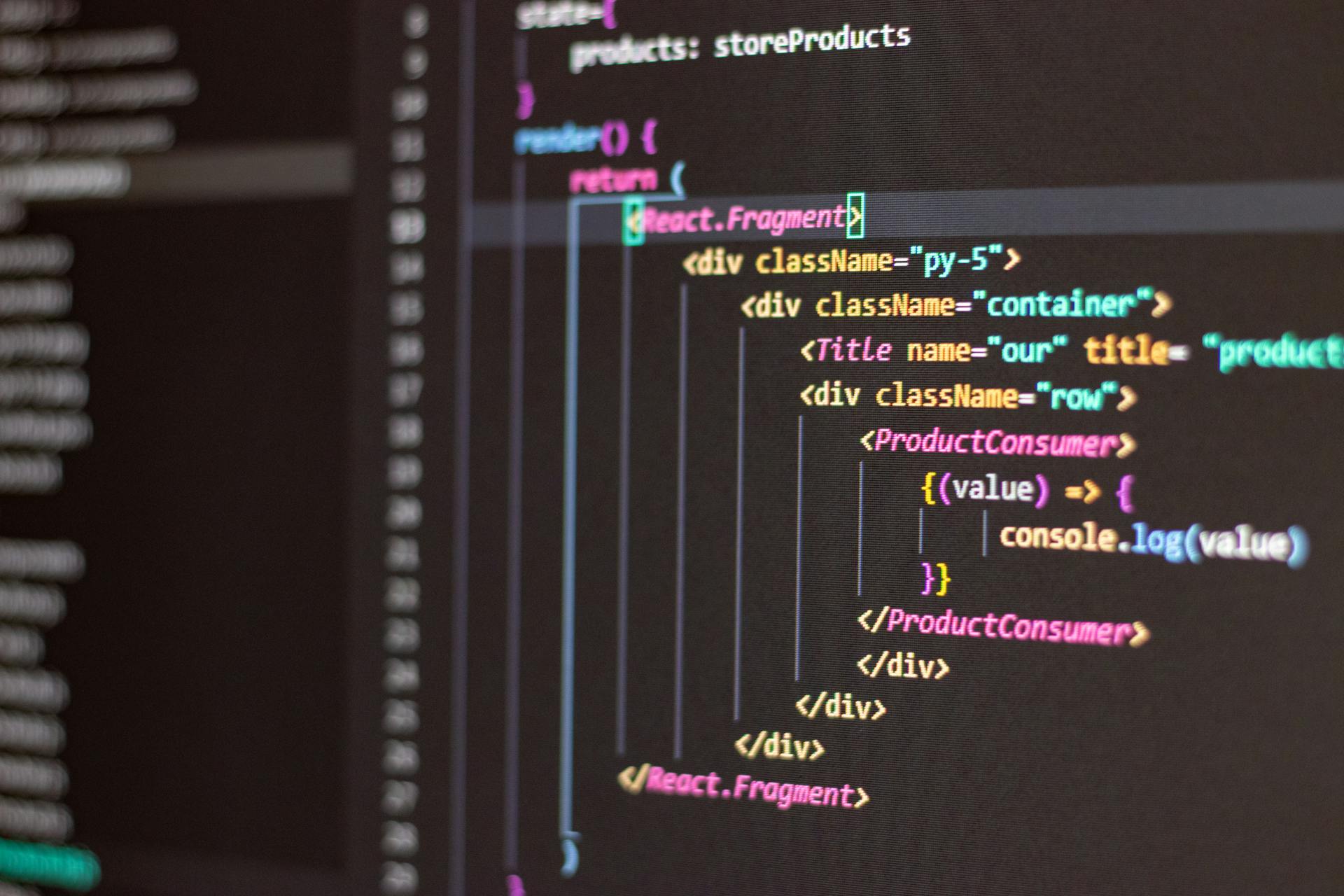
Disabling Server-Side Rendering (SSR) in Next.js can be a game-changer for enhanced security.
To start, you'll need to understand that SSR is enabled by default in Next.js, and it's what allows your application to pre-render pages on the server. However, this also means that your server is handling sensitive data, which can be a security risk.
You can disable SSR in your Next.js project by setting the `target` option in your `next.config.js` file to `'serverless'`. This will switch your app to client-side rendering, which can help prevent server-side vulnerabilities.
By disabling SSR, you'll also avoid the risk of server-side code execution, which can be exploited by attackers.
On a similar theme: Nextjs Redirect from Server Component
Verify Server-Side Rendering
To confirm whether Server-Side Rendering (SSR) is disabled, you can check if the window object is undefined. If SSR is disabled, the window object will be undefined.
You can create a utility function to check this. A simple function could be:
```javascript
function isClient() {
return typeof window !== 'undefined';
}
```
This function returns true if the window object is defined, indicating that SSR is enabled.
Disabling Server-Side Rendering in Next.js
Disabling Server-Side Rendering in Next.js can be a bit tricky, but don't worry, I've got you covered.
To disable SSR in Next.js, you'll need to go through some steps. You can start by creating a fresh Next.js application using npx create-next-app.
To disable SSR for page content, you need to add a specific code to pages/_app.js. This code wraps your page content in a component called SafeHydrate, which prevents the page content from rendering on the server.
When checking if we're on the server, we use the window object. However, simply wrapping our code in an if-statement won't work, as it'll produce a hydration mismatch warning in the console.
To hide this warning, we can render a div with the prop suppressHydrationWarning. This can be done by creating a separate SafeHydrate component and wrapping our page component into it.
You can verify if Server-Side Rendering is disabled by checking if the window object is undefined. A utility function can be created to check this.
If SSR is disabled, you should be able to see the Main component.
Worth a look: Nextjs Components Library
Why
You might wonder why you'd want to disable Server-Side Rendering (SSR) in Next.js, but it's actually quite simple. The main reason is that SSR has multiple trade-offs and challenges, particularly if you don't need it.
For example, using SSR requires a complicated hosting environment, which adds complexity and costs more. You also need to make sure your code works both on the browser and on the server, which makes debugging harder and restricts you in some cases.
Here are some specific reasons why you might want to disable SSR:
- The app's content doesn't need to rank high on search results (SEO).
- You don't need social-media previews (Facebook, Twitter, Slack, etc.).
- You don't need the additional speed optimizations it can provide for your users.
Opting out of NextJS
Opting out of NextJS can be a good decision, especially if your app is behind a login. This is because server-side rendering (SSR) has several trade-offs and challenges that might not be worth it for certain types of applications.
You'll need a complicated hosting environment to use SSR, which adds complexity and costs more. This includes using Node.js servers for server-side rendering, which is not as straightforward as uploading your app to a server or a CDN.
Take a look at this: Nextjs Url Parameter Is Not Allowed
Disabling SSR can simplify your application architecture, as you won't need to fetch all the data for the page in a single location. This can be a relief if you're using libraries like Redux or React Query for data fetching.
If your app is behind a login, you likely should disable SSR, as it's not worth the trade-offs and challenges. For example, you can't use localStorage to store authorization information, but you need to pass it in a cookie and use a cookie library that works on the server and the browser.
You might enjoy: Next Js Fetch Data save in Context and Next Route
Csr, Ssg: Definitions
Next.js supports three forms of rendering: Client-Side Rendering, Static Site Generation, and Server-Side Rendering. Static Site Generation and Server-Side Rendering are two forms of pre-rendering methods.
Client-Side Rendering generates HTML for a page on the client's browser.
The significant difference between these renderings is when it generates the HTML for a page.
Static Site Generation generates HTML at build time, making it a pre-rendering method.
This means that Static Site Generation can be used to improve page load times and SEO.
Disabling SSR Methods
You can disable Server-Side Rendering (SSR) in Next.js by using the npx create-next-app command to create a fresh Next.js application.
There are several methods to disable SSR, including dynamic import.
Dynamic import allows you to create a wrapper component, such as NoSSRWrapper, that wraps any page where you want SSR disabled.
next/dynamic is a Next.js function that enables dynamic importing of components, which can be useful for controlling SSR or optimizing initial page loads.
To use next/dynamic, you'll need to ensure you have Next.js installed in your project, as it's a built-in feature.
By using dynamic, you can import components asynchronously at runtime, rather than during the initial server render.
The dynamic function takes two arguments: a function that imports the component and an options object that can include properties like ssr.
You can explicitly instruct Next.js to exclude a component from server-side rendering by setting the ssr property to false in the options object.
This approach allows for fine-grained control over SSR, helping you balance rendering components on the server for SEO and initial page loads with offloading others for better client-side interactivity.
Related reading: Disable Hydration Nextjs
Alternative Approaches
Dynamic import is a viable alternative to disabling server-side rendering (SSR) in Next.js. You can create a wrapper component and wrap any page where you want SSR disabled with that component.
Using next/dynamic is another approach to disable SSR. This function allows for dynamic importing of components, enabling loading components asynchronously.
next/dynamic is a built-in Next.js feature, so no separate installation is required. It takes two arguments: a function that imports the component and an options object that can include properties like ssr.
To use dynamic, you can employ it by asynchronously importing components at runtime rather than during the initial server render. This ensures that certain components are not bundled with the server-rendered HTML.
The dynamic function operates by including a property like ssr, which explicitly instructs Next.js to exclude the component from server-side rendering. This helps in managing the balance between rendering components on the server for SEO and initial page loads while offloading others for better client-side interactivity.
For more insights, see: Next Js Dynamic
A string 'use client' at the top of a component file can also be used as a workaround to trigger client-side rendering for that component. This technique exploits Next.js behavior to exclude certain components from server-side rendering.
Note that this technique is considered more of a workaround and might not be officially documented or supported. It's essential to test thoroughly and ensure it aligns with the application's requirements.
Sources
- https://blogs.perficient.com/2022/09/19/how-to-disable-server-side-rendering-in-next-js/
- https://dev.to/apkoponen/how-to-disable-server-side-rendering-ssr-in-next-js-1563
- https://dev.to/apkoponen/how-to-disable-server-side-rendering-ssr-in-next-js-1563/comments
- https://sourcefreeze.com/how-to-disable-server-side-rendering-in-nextjs/
- https://sourcefreeze.com/tag/nextjs/
Featured Images: pexels.com