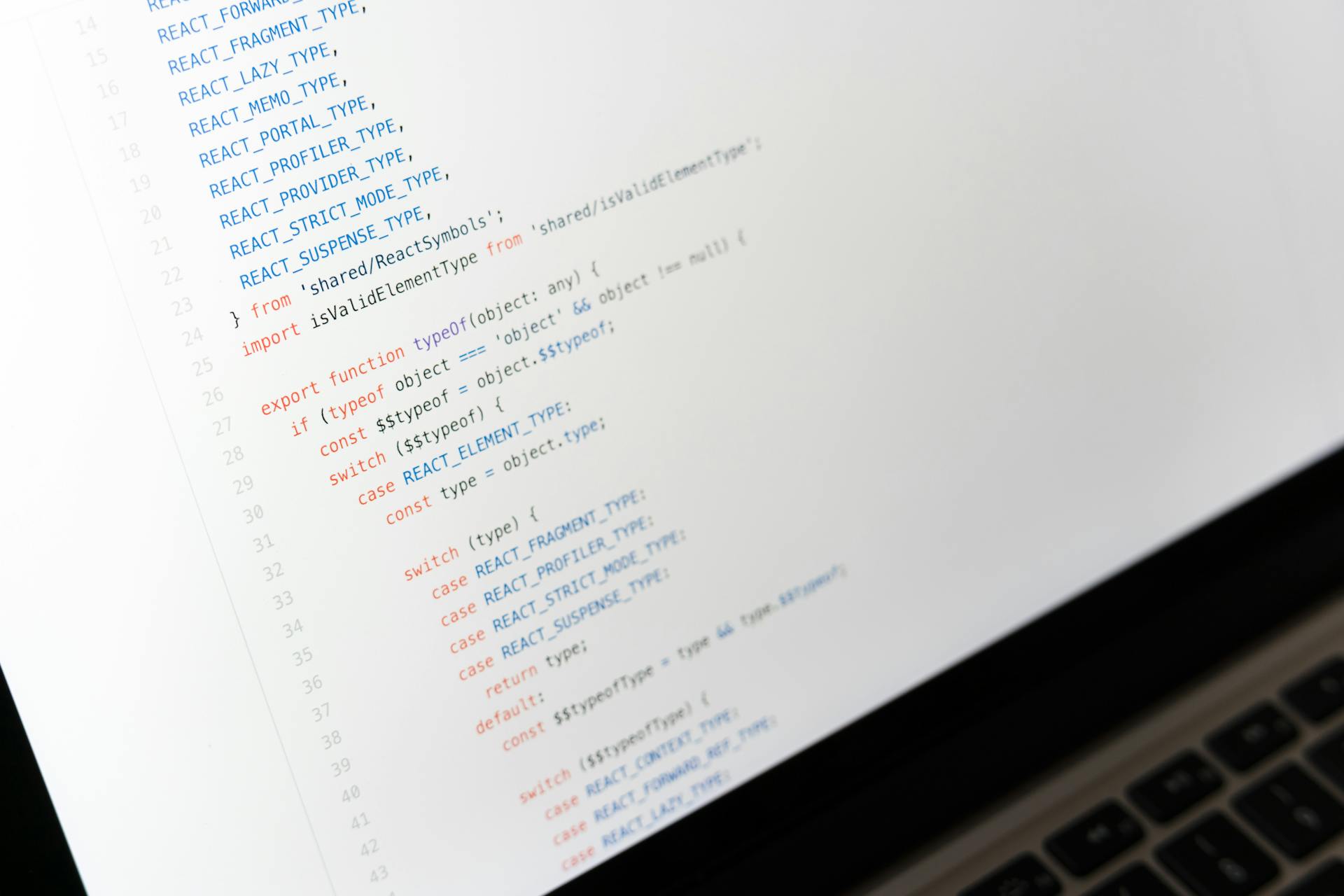
Using the `fetch` API in Next.js can be as simple as making a GET request to a URL. This is demonstrated in the example where a GET request is made to a URL using the `fetch` API.
The `fetch` API can also be used to make POST requests, which is useful when sending data to a server. For instance, sending a JSON object to a server can be achieved with a single line of code using the `fetch` API.
Making multiple requests at once can be achieved using the `Promise.all` method. This is especially useful when making multiple API calls to different servers.
Using the `fetch` API in Next.js can also be used to handle errors, such as when a server returns a 404 status code. This can be achieved by checking the status code of the response.
A fresh viewpoint: Next Js Fetch Data save in Context and Next Route
Features and Methods
Next.js offers several features and methods for fetching data, each with its own strengths and use cases.
Client-side data fetching is one of the methods available in Next.js, which fetches data on the client-side after the UI has been sent to the user. This reduces SEO because the UI is sent without the layout markup.
Server-side data fetching, on the other hand, fetches data while the UI is still on the local machine before rendering it to the user. This approach is achieved using getServerSideProps to fetch data from a database or an API as a backend.
Static-site generation is the fastest way to fetch data and send it to the user, pre-fetching data from the backend and sending it to the UI before the UI is sent to the user.
Next.js provides several methods to fetch data, including using the useEffect and useState hook for client-side data fetching, getServerSideProps for server-side data fetching, getStaticProps for static-site generation, and getStaticPath for static-site generation with dynamic routes.
To fetch data when statically rendering, Next.js recommends using getStaticProps() and getStaticPaths() in page files for the Pages Directory, or using async/await in page.(js|jsx|ts|tsx) files for the App Router.
Here are the data fetching methods in Next.js, each tailored to different scenarios and rendering strategies:
- useEffect and useState hook for client-side data fetching.
- getServerSideProps for server-side data fetching
- getStaticProps for static-site generation
- getStaticPath for static-site generation with dynamic routes.
GetStaticProps and Related
You can use getStaticProps to fetch data at build time, which generates static HTML and JSON files that a CDN can cache. This method is ideal when your page content doesn't change frequently.
getStaticProps is executed only once during build time, and the data is cached and rendered each time the page is requested. This approach leads to faster page loads and reduces the number of data requests to your data source.
Here's a basic example of using getStaticProps:
- Fetch data from an API during build time
- Pass the information as props to the page where it's rendered
- Reuse the cached file instead of fetching data on every request
To handle changes in data, you can use Incremental Static Regeneration (ISR), which rebuilds the project only when the data changes. This approach eliminates the need to rebuild the entire project every time the data changes.
GetStaticProps
GetStaticProps is a powerful function in Next.js that allows you to fetch data at build time. This method is ideal when your page content doesn't change frequently.
By using GetStaticProps, you can ensure that every request serves the same data, cached at build time, leading to faster page loads and reducing the number of data requests to your data source.
For another approach, see: Next Js 14 Redirect to Another Page Loading Indicator
GetStaticProps is executed only once during build time, and the data is cached and rendered each time the page is requested. This is in contrast to getServerSideProps, which is executed on every request.
Here are some key benefits of using GetStaticProps:
- Faster page loads
- Reduced data requests to your data source
- Improved performance
However, if the data has changed since you last built your project, you'll need to use Incremental Static Regeneration (ISR) to update the static pages. This involves adding a property called `revalidate` to the `getStaticProps` function.
For example, you might use the following code to revalidate your data every 20 seconds:
```javascript
getStaticProps = async () => {
// ...
revalidate: 20
}
```
This will trigger a revalidation of the data every 20 seconds, and the page will be regenerated with the new data.
You might like: Next Js Revalidate
GetInitialProps for Initial Loading
getInitialProps is an older Next.js feature that enables server-side data fetching for initial page loads.
It can be used in both server-rendered and statically exported Next.js applications, but it's recommended to use getStaticProps or getServerSideProps for more granular control over rendering and fetching strategies.
For your interest: Nextjs Pathname Server Component
The choice of method depends on the nature of your data and the user experience you aim to deliver, so it's essential to consider your specific needs when deciding which approach to take.
getInitialProps is still supported, but using getStaticProps or getServerSideProps can optimize parallel data fetching, minimize request time, and improve the overall performance of your Next.js applications.
Remember, the goal is to deliver a seamless user experience, and selecting the right data fetching method is a crucial step in achieving that.
GetServerSideProps and Related
GetServerSideProps is a function in Next.js that fetches data on each request, ensuring the rendered result always includes the latest data.
It's executed on each request and pre-renders the page on the server side with the data passed from the getServerSideProps method.
Unlike getStaticProps, the code inside getServerSideProps gets called each time the page is requested and pre-rendered on the server.
This function is particularly useful for pages that require fresh data for each request.
Broaden your view: Nextjs 404
Here are some key points to consider when using getServerSideProps:
- It fetches data on each request, ensuring the latest data is included in the rendered result.
- The function is executed on each request and pre-renders the page on the server side.
- It's useful for pages that require fresh data for each request.
In Next.js, you can create a folder in the pages folder and a file called index.js to use the getServerSideProps function to fetch data.
The getServerSideProps function is not written in the component function, but instead, the data is passed as a property to the component function through the return object.
Here's an example of how to use getServerSideProps to fetch data from a URL:
- Create a folder in the pages folder and a file called index.js.
- Write the code to fetch data from a URL using the getServerSideProps function.
- Set a limit by using ?limit=8 to limit the data fetched.
- Pass the data as a property to the component function through the return object.
In the component function, you can map the data using the map function to get each item.
You might like: Next Js Function Handlers to Filter Data
Next.js's flexible architecture allows for both server and client components, each playing a distinct role in the data fetching process.
Server components handle the data fetching on the server, while client components handle the data rendering on the client.
Here's a summary of the key differences between server and client components:
This architecture allows for efficient data fetching and rendering, making it a powerful tool for building fast and scalable applications.
API Calls and Handling
The fetch API is asynchronous, so you need to await it in Next.js. It's a function that takes the API endpoint as its first parameter, with the second parameter being an object containing data like HTTP method, request headers, and request body.
To make a GET request, you simply call the fetch API function with the endpoint, like this: fetch('https://api.example.com/data'). If you want to make a POST request, you can replace GET with POST and add a body key holding an object with the request body.
Next.js streamlines API calls with built-in functions and best practices, ensuring efficient and effective API calls. Whether you're dealing with user information, product details, or any other data.
You can use the fetch API to make asynchronous API calls directly from your Next.js pages or components. This function can be called within your Next.js page to retrieve data and pass it to your components.
Handling the response and potential errors is crucial when making a fetch request. Next.js allows you to elegantly manage both successful and erroneous responses by using try-catch blocks.
Incorporating await fetch within async functions is a common pattern in Next.js for server-side data fetching. This function fetches data on the server every time the page is requested, which is particularly useful for dynamic content that changes often.
Additional reading: Next Js Components Folder
Optimizing Performance
Optimizing performance is crucial for a smooth user experience. Performance is key in web applications, and optimizing data fetching is a significant part of ensuring your app is fast and responsive.
A different take: Nextjs Performance
To achieve this, consider using parallel data fetching, which allows you to send multiple fetch requests at the same time. This can be achieved using Promise.all, significantly reducing the total time it takes to load all necessary data for your page.
Parallel data fetching can be a game-changer for large datasets. By fetching data in parallel, you can reduce the total time it takes to load all the necessary data for your page.
Caching is another powerful strategy to improve loading times. By storing fetched data in a cache, you can serve repeated requests without having to make new API calls each time. Next.js provides several caching strategies, including the built-in cache for getStaticProps and custom caching solutions that can be implemented with third-party libraries like react-query.
Implementing caching strategies can make a significant difference in how quickly your pages render.
You might enjoy: Next Js Page Transitions
Frequently Asked Questions
How to fetch data in Next.js without useEffect?
Fetch data in Next.js without useEffect by using async/await syntax with Server Components, which execute on the server and send results to the client
Sources
- https://blog.openreplay.com/data-fetching-in-next-js/
- https://jscrambler.com/blog/understanding-data-fetching-in-next-js
- https://rapidapi.com/guides/how-to-use-fetch-api-in-next-js
- https://prismic.io/docs/fetch-data-nextjs
- https://www.dhiwise.com/post/nextjs-data-fetching-how-to-efficiently-load-and-manage-data
Featured Images: pexels.com