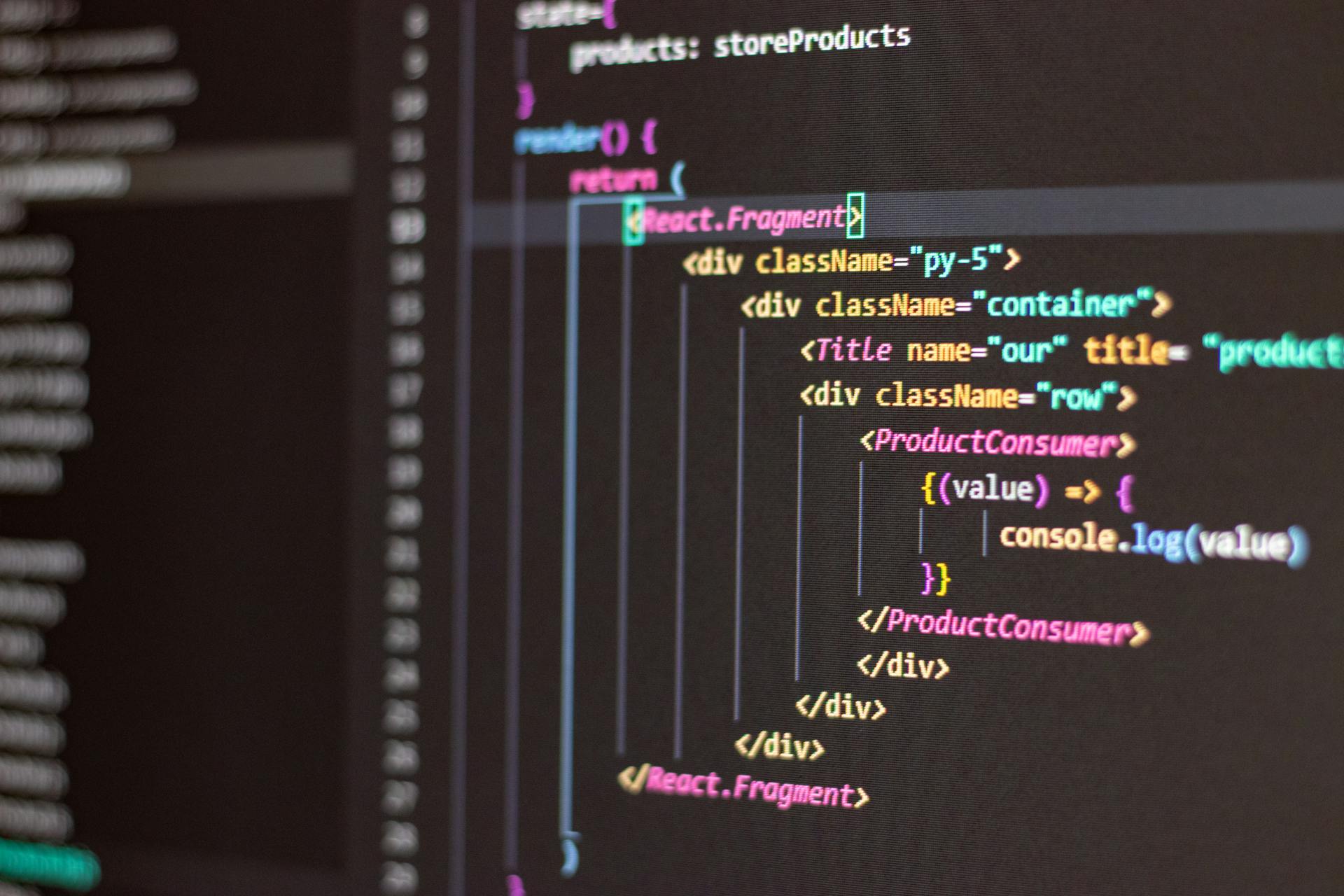
Redirecting to another page in Next.js 14 can be a seamless experience for users with a well-implemented loading indicator.
By default, Next.js 14 provides a built-in loading indicator that appears when a user is redirected to another page.
This built-in loading indicator is a circular progress bar that indicates to the user that the page is loading.
You can customize the loading indicator to suit your application's design and branding.
To customize the loading indicator, you can use the `getStaticProps` method and return a `loading` object with the desired properties.
For example, you can change the color and size of the loading indicator by passing a `style` object to the `loading` object.
This allows you to tailor the loading indicator to your application's visual identity and create a cohesive user experience.
By implementing a customized loading indicator, you can improve the user experience and make your application feel more polished and professional.
You might like: Can Nextjs Be Used on Traditional Web Application
Redirecting
Redirecting is a crucial aspect of Next.js development, and there are several ways to achieve it. You can define Next.js redirects in the next.config.js file, which applies to the entirety of your application and all users accessing it.
This method is best for permanent redirects on the server side, where you need to inform search engines of a changed URL. It's ideal for correcting misspelled URLs or updating outdated links.
For client-side redirects, you can use the useRouter hook and call the push method to send the user to a given path. This method is suitable for loading a new page in response to a user action.
The App Router and Pages Router in Next.js 14 have similarities, including customization options like push and replace, and the ability to target specific routes or paths. However, they differ in usage, flexibility, and data-fetching capabilities.
Here are the key differences between App Router and Pages Router redirects:
To avoid issues with redirects in API routes, make sure to use the correct syntax for res.redirect() and call it before sending any response back. This will ensure that the redirect is executed correctly and the response is not modified after it has been finalized.
Loading UI
Loading UI is a crucial aspect of creating a seamless user experience. In Next.js 14, you can use the built-in `useRouter` hook to navigate between pages and display a loading indicator.
Next.js 14 provides a simple way to display a loading indicator when navigating between pages using the `useRouter` hook. You can use the `isFallback` property to determine if the page is still loading.
The `isFallback` property returns `true` when the page is still loading, allowing you to conditionally render a loading indicator. For example, you can use a simple `div` element with a loading animation to display a loading indicator.
A simple example of a loading indicator can be achieved by using a CSS animation. Next.js 14 provides a built-in `Loading` component that you can use to display a loading indicator.
See what others are reading: Nextjs Pages
Next.js Redirects
Next.js redirects are a powerful feature that allows you to control how users navigate your application. You can define redirects in the next.config.js file, which applies to the entirety of your application and all users accessing it.
For your interest: Next Js Single Page Application
To create redirects in next.config.js, you can add them to the return value of the redirects function exported by the module. This is best for permanent redirects on the server side, where you need to inform search engines of a changed URL.
For example, if you have a page with a misspelled URL, you can create a permanent redirect in next.config.js to redirect the old URL. This way, links to the old mistyped URL still work. You can also use regex to redirect multiple URLs at once.
Redirects in Next.js can be used for SEO purposes, as permanently redirected URLs will pass their SEO score on to the new page. Temporary redirects, on the other hand, will not pass on their SEO score, making them suitable only for short-lived redirects.
Here are the key differences between App Router redirects and Pages Router redirects:
In API routes, you can use the res.redirect() method to perform a redirect. However, make sure to call res.redirect() before any response is sent back, and provide the correct syntax for the status code and destination URL.
You can also use the useRouter hook to redirect pages on the client side based on user actions. This method is best used when a new page needs to load in response to a user action, such as when a button is clicked.
Next.js Redirect
Next.js Redirect is a crucial aspect of building a seamless user experience. You can define Next.js redirects in the next.config.js file, located in your project directory, to apply to the entirety of your application and all users accessing it.
This method is best for permanent redirects on the server side, where you need to inform search engines of a changed URL. For example, if you have a page with a misspelled URL, you can correct the URL and create a permanent redirect in next.config.js to redirect the old URL.
There are different ways to redirect, including basic redirects from one page to another, redirects using regex, and redirects for specific paths. You can also use the useRouter hook to redirect pages on the client side based on user actions.
Here are some key differences between App Router and Pages Router redirects:
Remember to use the correct syntax for res.redirect() when performing redirects in API routes, and make sure to call res.redirect() before sending any response back to the client.
Next.js Best Practices
To get the best out of Next.js, you should follow its best practices. For SEO purposes, pages in your app should have unique URLs and not just load content dynamically without updating the current page address.
Permanently redirected URLs will pass their SEO score on to the new page, which is good for maintaining SEO. Temporary redirects will not pass on their SEO score, making them suitable only for short-lived redirects like temporarily hiding a page while it is updated. This means you should use permanent redirects for pages you want to rank in search engines.
Each redirect requires reloading content, which will disrupt your users’ experience. To get the best performance for your Next.js apps, use a composable content platform to deliver optimized media from a high-speed CDN.
You might like: Nextjs Router Not Mounted
UI Best Practices
For a seamless user experience, it's essential to keep redirects to a minimum. Each redirect requires reloading content, which can disrupt your users' experience.
To maintain SEO, permanently redirected URLs will pass their SEO score on to the new page. Temporary redirects, on the other hand, will not pass on their SEO score and are best suited for short-lived redirects.
Use unique URLs for your pages to avoid any confusion and ensure that they can be properly indexed by search engines. This will help you maintain a strong SEO score.
For more insights, see: Next Js Seo
Security Considerations
To ensure the security of your Next.js application, you should enable the Content Security Policy (CSP) to prevent cross-site scripting (XSS) attacks. This can be done by adding the `Content-Security-Policy` header in your `next.config.js` file.
Always validate user input to prevent XSS attacks, as demonstrated in the "Input Validation" section. This can be achieved by using a library like `sanitize-html` to sanitize user input before rendering it on the page.
Use a secure protocol like HTTPS to protect sensitive data transmitted between the client and server. This can be achieved by setting `secure` to `true` in your `next.config.js` file.
Here's an interesting read: Next Js File Upload
Always validate user input to prevent SQL injection attacks, as demonstrated in the "Input Validation" section. This can be achieved by using a library like `sanitize-html` to sanitize user input before rendering it on the page.
Use a Web Application Firewall (WAF) to protect your application from common web attacks, such as SQL injection and cross-site scripting. This can be done by integrating a WAF like Cloudflare into your Next.js application.
Always keep your dependencies up to date to prevent known vulnerabilities, as demonstrated in the "Dependency Management" section. This can be achieved by using a tool like `npm audit` to scan for known vulnerabilities.
Curious to learn more? Check out: Next Js Sql
Sources
- https://nextjs.org/docs/app/building-your-application/routing/redirecting
- https://nextjs.org/docs/pages/building-your-application/routing/redirecting
- https://nextjs.org/docs/app/building-your-application/routing/loading-ui-and-streaming
- https://www.contentful.com/blog/next-js-redirect/
- https://blog.logrocket.com/redirects-next-js/
Featured Images: pexels.com