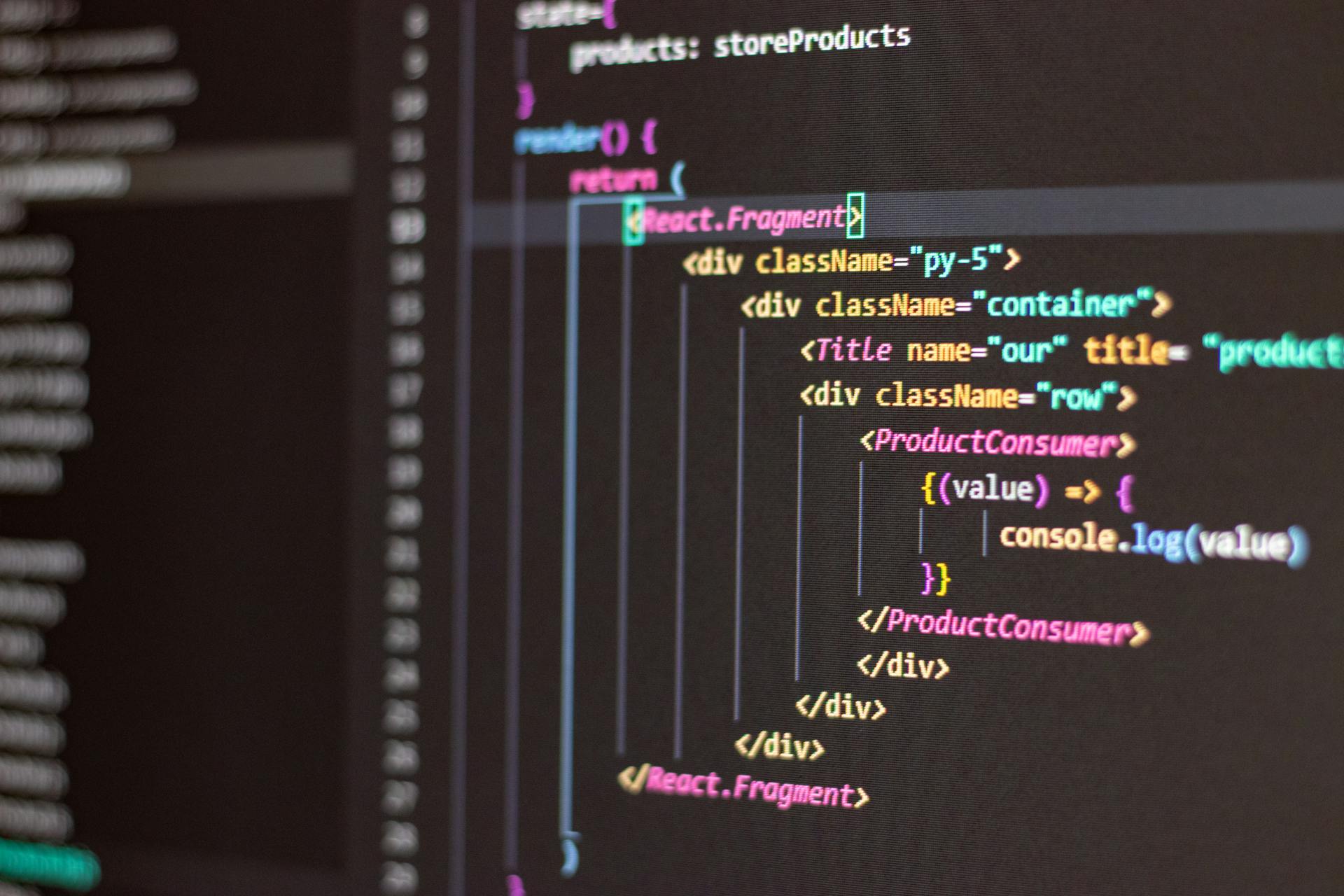
Working with databases in Next.js can be a challenge, but Prisma makes it a whole lot easier. Prisma is an ORM (Object-Relational Mapping) tool that allows us to interact with our database using a more intuitive and code-friendly approach.
Prisma simplifies database operations by providing a more declarative way of defining our database schema and performing CRUD (Create, Read, Update, Delete) operations. This means we can focus on writing our application logic without getting bogged down in the details of database queries.
By using Prisma, we can write database-agnostic code that works seamlessly with different database systems, including PostgreSQL and MySQL.
Curious to learn more? Check out: Next Js Db
Setting Up the Environment
To set up the environment for your Next.js app, you'll need to start by installing Node.js and npm, the foundation for server-side application development. This is the first step in creating a solid foundation for your project.
You'll also want to initialize a new project using the Next.js framework, which enables you to extend the latest React capabilities seamlessly. This will give you a head start on building your app.
Here are the key tools and technologies you'll need to get started:
- Node.js and npm
- Next.js framework
- TiDB Serverless cluster
- Yarn package manager
By setting up these tools and technologies, you'll be well on your way to connecting your Next.js app to a database securely and efficiently.
Installing Necessary Dependencies
Installing the necessary dependencies is a crucial step in setting up your Next.js project. You'll want to start by installing the required npm packages to establish the connection between Next.js and PostgreSQL.
The pg package is a PostgreSQL client for Node.js that you'll need to install. It's a must-have for connecting to your PostgreSQL database.
To get started, create a new file named db.js within your Next.js project. This file will handle database connections.
Next, you'll need to install the pg package using npm. You can do this by running the command 'npm install pg' in your terminal.
Alternatively, you can also use the 'next js connect to postgres' guide for setup, which mentions the pg package as a required dependency.
Here's a quick rundown of the necessary packages to install:
By installing these packages, you'll be well on your way to connecting your Next.js app to a PostgreSQL database securely and efficiently.
Configuring Connection Settings
Configuring connection settings is a crucial step in setting up your Next.js environment. You'll want to store sensitive database connection details securely by creating a .env file in the root of your Next.js project.
To do this, populate the .env file with environment variables specifying the database host, port, user, password, and name. This will help you keep your database credentials safe from prying eyes.
For a smooth development journey, start by installing Node.js and npm, the foundation for your server-side application development. This will provide the necessary tools for your server-side application development.
You'll also want to initialize a new project using the Next.js framework, enabling you to extend the latest React capabilities seamlessly. This will give you a solid foundation for building your Next.js application.
To manage your project effectively, consider utilizing the benefits of the Yarn package manager, offering a fast and secure alternative to npm.
Here's a quick rundown of the essential tools and technologies you'll need to set up:
By setting up these tools and technologies meticulously, you'll be well on your way to a smooth development journey, connecting your Next.js app to a database securely and efficiently.
Database Connection
Connecting your Next.js application to a database is a crucial step in building a robust and scalable web application. This can be achieved by integrating Next.js with a powerful database system like PostgreSQL.
To store sensitive database connection details securely, create a .env file in the root of your Next.js project and populate it with environment variables specifying the database host, port, user, password, and name.
A connection pool manages and reuses database connections, improving overall performance. This can be implemented by creating a function responsible for creating a connection pool to the PostgreSQL database inside a db.js file.
Crafting tailored connection logic within your Next.js application allows you to interface with the MySQL2 driver effectively. By customizing this logic, you optimize database interactions and enhance overall performance.
In the realm of database connectivity, encountering connection errors can impede the seamless operation of your Next.js application. By proactively addressing these issues through systematic debugging techniques, you can swiftly identify root causes, rectify connection discrepancies, and ensure uninterrupted data flow.
Intriguing read: Why Database Is Important
To establish a seamless connection between your Next.js application and the TiDB Serverless cluster, leverage the robust capabilities of the MySQL2 driver. This driver ensures efficient data retrieval and manipulation within your web application.
Here's a step-by-step guide to connecting to the database using the MySQL2 driver:
- Populate the connection parameters object using the GetDBSettings() function to get the environment variables corresponding to the database connection.
- Connect to the database using the mysql.createConnection() method.
- Create the SQL query to fetch data.
- Execute the query and retrieve the results using the mysql.execute() method.
- Close the connection when done using the mysql.end() method.
- Return the results as a JSON API response using NextResponse.
Prisma and ORM
Prisma Client is a crucial component in a Next.js application, and it's essential to initialize a Prisma Client instance to read and write from/to the database.
To avoid issues with hot reloading and multiple instances of the Prisma Client in development mode, use the singleton pattern to create a Prisma Client instance. This can be achieved using the following code snippet.
Sequelize is a reliable Node.js Object-Relational Mapper (ORM) that can help you define models and schemas effectively within your Next.js application. Its robust features include SOLID transaction support, relations management, and read replication capabilities.
Discover more: Next Js Client Portal
Prisma Migrations
Prisma Migrations are a crucial part of working with Prisma, as they allow you to introduce new data in the database.
To create a new table in the database, you can add it to the Prisma schema file. For example, you can create a Post table with specific properties.
You can then migrate the database by running a command, which will update the database schema accordingly.
Prisma Studio is a useful tool for checking the database and seeing the changes you've made. You can use it to verify that the new table has been created.
Seed the database with some initial data to see it later in your Next.js application, if you want to test it out.
Recommended read: Nextjs 14 New Features
Defining Models with ORM
Defining models is a crucial step in structuring your data effectively, and Sequelize is a reliable Node.js Object-Relational Mapper (ORM) that can help you achieve this.
Sequelize offers robust features like SOLID transaction support, relations management, and read replication capabilities, making it a popular choice for defining models and schemas within Next.js applications.
To define models using Sequelize, you can leverage its robust features to streamline the process. You can create models that align with your application's data model, ensuring that your database schema is well-structured and efficient.
Sequelize's robust features make it an ideal choice for defining models, and its popularity in the Next.js community is a testament to its effectiveness.
Here are some key benefits of using Sequelize to define models:
By leveraging Sequelize's features, you can define models that are well-structured, efficient, and scalable, ultimately improving the performance and maintainability of your Next.js application.
API and CRUD Operations
Next.js makes it easy to create API routes for handling CRUD operations, which include data retrieval, insertion, updating, and deletion endpoints.
With Next.js, you can create API routes for CRUD operations, making it a great choice for building server-side logic.
Integrating database connection logic from db.js into your API routes ensures each API request has a dedicated and managed database connection, preventing connection leaks and improving overall performance.
In Next.js, handling database connections in API routes is crucial for performance and security.
By managing database connections in API routes, you can prevent connection leaks and improve overall performance, which is essential for a smooth user experience.
Additional reading: Best Next Js Database with Drizzle
Error Handling and Deployment
Error handling is crucial to ensure a seamless user experience. Verify that your application seamlessly connects to the production database after deployment.
When deploying your application and database, follow the provider-specific deployment instructions to ensure a successful deployment. This will help you avoid common pitfalls and ensure your application is up and running smoothly.
Discover more: Can Nextjs Be Used on Traditional Web Application
Connection Errors
Connection errors can impede the seamless operation of your Next.js application.
Encountering connection errors in the realm of database connectivity can be frustrating, but proactive debugging techniques can help you identify root causes quickly.
By embracing a proactive approach to debugging connection errors, you can rectify connection discrepancies and ensure uninterrupted data flow between your app and the TiDB Serverless cluster.
Systematic debugging techniques can help you swiftly identify and address connection errors, enhancing the stability and efficiency of your database integration.
In fact, addressing connection errors proactively can prevent future issues and ensure a smoother experience for your users.
Deploying Next.js App
Deploying your Next.js app involves several key steps. First, you'll need to deploy your application and database to a hosting provider, following their specific deployment instructions to ensure a seamless deployment.
To deploy your application, you'll need to use a hosting provider that supports your js application. Make sure to follow the provider-specific deployment instructions to ensure a successful deployment.
Once deployed, verify that your application connects to the production database. This is crucial to ensure that your application functions as expected.
You might like: Nextjs Hosting
Development and Tools
To develop a Next.js app that connects to a database, you'll need to set up a solid foundation. Begin by installing Node.js and npm, the foundation for your server-side application development.
Installing Node.js and npm will give you the tools you need to create a Next.js project. Initialize a new project using the Next.js framework, which enables you to extend the latest React capabilities seamlessly.
A TiDB Serverless cluster is a powerful tool for optimizing performance. Integrate it with Vercel Functions for efficient data processing.
On a similar theme: Npm Next Js
For managing your project effectively, consider using the Yarn package manager. It offers a fast and secure alternative to npm.
Here are the essential tools and technologies you'll need:
- Node.js and npm
- Next.js framework
- TiDB Serverless cluster with Vercel Functions
- Yarn package manager
By setting up these tools and technologies, you'll be well on your way to connecting your Next.js app to a database securely and efficiently.
Frequently Asked Questions
Does Next.js have a database?
No, Next.js itself does not have a built-in database, but it can be used with external databases like MySQL, PostgreSQL, and SQL Server through Prisma. Next.js is a framework for building server-side rendered and statically generated websites, and Prisma helps you connect it to your database of choice.
Can Next.js be used as a backend?
Yes, Next.js can be used as a backend framework for server-side rendering and dynamic content management. Its API routes and middleware enable seamless backend functionality alongside frontend duties.
Sources
- https://www.robinwieruch.de/next-prisma-sqlite/
- https://www.saffrontech.net/blog/how-to-connect-nextjs-with-postgres-sql
- https://www.pingcap.com/article/how-to-connect-your-next-js-app-to-a-database/
- https://medium.com/@asishpanda444/next-js-api-connect-to-mysql-database-using-next-js-c020fa17a299
- https://stackoverflow.com/questions/74709716/connect-sql-server-with-next-js
Featured Images: pexels.com